CMSIS DSP library
Dependents: performance_timer Surfboard_ gps2rtty Capstone ... more
arm_cfft_radix2_init_q31.c
00001 /* ---------------------------------------------------------------------- 00002 * Copyright (C) 2010-2014 ARM Limited. All rights reserved. 00003 * 00004 * $Date: 19. March 2015 00005 * $Revision: V.1.4.5 00006 * 00007 * Project: CMSIS DSP Library 00008 * Title: arm_cfft_radix2_init_q31.c 00009 * 00010 * Description: Radix-2 Decimation in Frequency Fixed-point CFFT & CIFFT Initialization function 00011 * 00012 * Target Processor: Cortex-M4/Cortex-M3/Cortex-M0 00013 * 00014 * Redistribution and use in source and binary forms, with or without 00015 * modification, are permitted provided that the following conditions 00016 * are met: 00017 * - Redistributions of source code must retain the above copyright 00018 * notice, this list of conditions and the following disclaimer. 00019 * - Redistributions in binary form must reproduce the above copyright 00020 * notice, this list of conditions and the following disclaimer in 00021 * the documentation and/or other materials provided with the 00022 * distribution. 00023 * - Neither the name of ARM LIMITED nor the names of its contributors 00024 * may be used to endorse or promote products derived from this 00025 * software without specific prior written permission. 00026 * 00027 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS 00028 * "AS IS" AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT 00029 * LIMITED TO, THE IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS 00030 * FOR A PARTICULAR PURPOSE ARE DISCLAIMED. IN NO EVENT SHALL THE 00031 * COPYRIGHT OWNER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, INDIRECT, 00032 * INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, 00033 * BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; 00034 * LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER 00035 * CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT 00036 * LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN 00037 * ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 00038 * POSSIBILITY OF SUCH DAMAGE. 00039 * -------------------------------------------------------------------- */ 00040 00041 00042 #include "arm_math.h" 00043 #include "arm_common_tables.h" 00044 00045 /** 00046 * @ingroup groupTransforms 00047 */ 00048 00049 /** 00050 * @addtogroup ComplexFFT 00051 * @{ 00052 */ 00053 00054 00055 /** 00056 * 00057 * @brief Initialization function for the Q31 CFFT/CIFFT. 00058 * @deprecated Do not use this function. It has been superseded by \ref arm_cfft_q31 and will be removed 00059 * @param[in,out] *S points to an instance of the Q31 CFFT/CIFFT structure. 00060 * @param[in] fftLen length of the FFT. 00061 * @param[in] ifftFlag flag that selects forward (ifftFlag=0) or inverse (ifftFlag=1) transform. 00062 * @param[in] bitReverseFlag flag that enables (bitReverseFlag=1) or disables (bitReverseFlag=0) bit reversal of output. 00063 * @return The function returns ARM_MATH_SUCCESS if initialization is successful or ARM_MATH_ARGUMENT_ERROR if <code>fftLen</code> is not a supported value. 00064 * 00065 * \par Description: 00066 * \par 00067 * The parameter <code>ifftFlag</code> controls whether a forward or inverse transform is computed. 00068 * Set(=1) ifftFlag for calculation of CIFFT otherwise CFFT is calculated 00069 * \par 00070 * The parameter <code>bitReverseFlag</code> controls whether output is in normal order or bit reversed order. 00071 * Set(=1) bitReverseFlag for output to be in normal order otherwise output is in bit reversed order. 00072 * \par 00073 * The parameter <code>fftLen</code> Specifies length of CFFT/CIFFT process. Supported FFT Lengths are 16, 64, 256, 1024. 00074 * \par 00075 * This Function also initializes Twiddle factor table pointer and Bit reversal table pointer. 00076 */ 00077 00078 arm_status arm_cfft_radix2_init_q31( 00079 arm_cfft_radix2_instance_q31 * S, 00080 uint16_t fftLen, 00081 uint8_t ifftFlag, 00082 uint8_t bitReverseFlag) 00083 { 00084 /* Initialise the default arm status */ 00085 arm_status status = ARM_MATH_SUCCESS; 00086 00087 /* Initialise the FFT length */ 00088 S->fftLen = fftLen; 00089 00090 /* Initialise the Twiddle coefficient pointer */ 00091 S->pTwiddle = (q31_t *) twiddleCoef_4096_q31 ; 00092 /* Initialise the Flag for selection of CFFT or CIFFT */ 00093 S->ifftFlag = ifftFlag; 00094 /* Initialise the Flag for calculation Bit reversal or not */ 00095 S->bitReverseFlag = bitReverseFlag; 00096 00097 /* Initializations of Instance structure depending on the FFT length */ 00098 switch (S->fftLen) 00099 { 00100 /* Initializations of structure parameters for 4096 point FFT */ 00101 case 4096u: 00102 /* Initialise the twiddle coef modifier value */ 00103 S->twidCoefModifier = 1u; 00104 /* Initialise the bit reversal table modifier */ 00105 S->bitRevFactor = 1u; 00106 /* Initialise the bit reversal table pointer */ 00107 S->pBitRevTable = (uint16_t *) armBitRevTable ; 00108 break; 00109 00110 /* Initializations of structure parameters for 2048 point FFT */ 00111 case 2048u: 00112 /* Initialise the twiddle coef modifier value */ 00113 S->twidCoefModifier = 2u; 00114 /* Initialise the bit reversal table modifier */ 00115 S->bitRevFactor = 2u; 00116 /* Initialise the bit reversal table pointer */ 00117 S->pBitRevTable = (uint16_t *) & armBitRevTable [1]; 00118 break; 00119 00120 /* Initializations of structure parameters for 1024 point FFT */ 00121 case 1024u: 00122 /* Initialise the twiddle coef modifier value */ 00123 S->twidCoefModifier = 4u; 00124 /* Initialise the bit reversal table modifier */ 00125 S->bitRevFactor = 4u; 00126 /* Initialise the bit reversal table pointer */ 00127 S->pBitRevTable = (uint16_t *) & armBitRevTable [3]; 00128 break; 00129 00130 /* Initializations of structure parameters for 512 point FFT */ 00131 case 512u: 00132 /* Initialise the twiddle coef modifier value */ 00133 S->twidCoefModifier = 8u; 00134 /* Initialise the bit reversal table modifier */ 00135 S->bitRevFactor = 8u; 00136 /* Initialise the bit reversal table pointer */ 00137 S->pBitRevTable = (uint16_t *) & armBitRevTable [7]; 00138 break; 00139 00140 case 256u: 00141 /* Initializations of structure parameters for 256 point FFT */ 00142 S->twidCoefModifier = 16u; 00143 S->bitRevFactor = 16u; 00144 S->pBitRevTable = (uint16_t *) & armBitRevTable [15]; 00145 break; 00146 00147 case 128u: 00148 /* Initializations of structure parameters for 128 point FFT */ 00149 S->twidCoefModifier = 32u; 00150 S->bitRevFactor = 32u; 00151 S->pBitRevTable = (uint16_t *) & armBitRevTable [31]; 00152 break; 00153 00154 case 64u: 00155 /* Initializations of structure parameters for 64 point FFT */ 00156 S->twidCoefModifier = 64u; 00157 S->bitRevFactor = 64u; 00158 S->pBitRevTable = (uint16_t *) & armBitRevTable [63]; 00159 break; 00160 00161 case 32u: 00162 /* Initializations of structure parameters for 32 point FFT */ 00163 S->twidCoefModifier = 128u; 00164 S->bitRevFactor = 128u; 00165 S->pBitRevTable = (uint16_t *) & armBitRevTable [127]; 00166 break; 00167 00168 case 16u: 00169 /* Initializations of structure parameters for 16 point FFT */ 00170 S->twidCoefModifier = 256u; 00171 S->bitRevFactor = 256u; 00172 S->pBitRevTable = (uint16_t *) & armBitRevTable [255]; 00173 break; 00174 00175 00176 default: 00177 /* Reporting argument error if fftSize is not valid value */ 00178 status = ARM_MATH_ARGUMENT_ERROR; 00179 break; 00180 } 00181 00182 return (status); 00183 } 00184 00185 /** 00186 * @} end of ComplexFFT group 00187 */
Generated on Tue Jul 12 2022 11:59:15 by
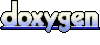