USBHost library. NOTE: This library is only officially supported on the LPC1768 platform. For more information, please see the handbook page.
Dependencies: FATFileSystem mbed-rtos
Dependents: BTstack WallbotWii SD to Flash Data Transfer USBHost-MSD_HelloWorld ... more
USBHostMIDI Class Reference
A class to communicate a USB MIDI device. More...
#include <USBHostMIDI.h>
Inherits IUSBEnumerator.
Public Member Functions | |
USBHostMIDI () | |
Constructor. | |
bool | connected () |
Check if a USB MIDI device is connected. | |
bool | connect () |
Try to connect a midi device. | |
void | attachMiscellaneousFunctionCode (void(*fn)(uint8_t, uint8_t, uint8_t)) |
Attach a callback called when miscellaneous function code is received. | |
void | attachCableEvent (void(*fn)(uint8_t, uint8_t, uint8_t)) |
Attach a callback called when cable event is received. | |
void | attachSystemCommonTwoBytes (void(*fn)(uint8_t, uint8_t)) |
Attach a callback called when system exclusive is received. | |
void | attachSystemCommonThreeBytes (void(*fn)(uint8_t, uint8_t, uint8_t)) |
Attach a callback called when system exclusive is received. | |
void | attachSystemExclusive (void(*fn)(uint8_t *, uint16_t, bool)) |
Attach a callback called when system exclusive is received. | |
void | attachNoteOn (void(*fn)(uint8_t, uint8_t, uint8_t)) |
Attach a callback called when note on is received. | |
void | attachNoteOff (void(*fn)(uint8_t, uint8_t, uint8_t)) |
Attach a callback called when note off is received. | |
void | attachPolyKeyPress (void(*fn)(uint8_t, uint8_t, uint8_t)) |
Attach a callback called when poly keypress is received. | |
void | attachControlChange (void(*fn)(uint8_t, uint8_t, uint8_t)) |
Attach a callback called when control change is received. | |
void | attachProgramChange (void(*fn)(uint8_t, uint8_t)) |
Attach a callback called when program change is received. | |
void | attachChannelPressure (void(*fn)(uint8_t, uint8_t)) |
Attach a callback called when channel pressure is received. | |
void | attachPitchBend (void(*fn)(uint8_t, uint16_t)) |
Attach a callback called when pitch bend is received. | |
void | attachSingleByte (void(*fn)(uint8_t)) |
Attach a callback called when single byte is received. | |
bool | sendMiscellaneousFunctionCode (uint8_t data1, uint8_t data2, uint8_t data3) |
Send a cable event with 3 bytes event. | |
bool | sendCableEvent (uint8_t data1, uint8_t data2, uint8_t data3) |
Send a cable event with 3 bytes event. | |
bool | sendSystemCommmonTwoBytes (uint8_t data1, uint8_t data2) |
Send a system common message with 2 bytes event. | |
bool | sendSystemCommmonThreeBytes (uint8_t data1, uint8_t data2, uint8_t data3) |
Send a system common message with 3 bytes event. | |
bool | sendSystemExclusive (uint8_t *buffer, int length) |
Send a system exclusive event. | |
bool | sendNoteOff (uint8_t channel, uint8_t note, uint8_t velocity) |
Send a note off event. | |
bool | sendNoteOn (uint8_t channel, uint8_t note, uint8_t velocity) |
Send a note on event. | |
bool | sendPolyKeyPress (uint8_t channel, uint8_t note, uint8_t pressure) |
Send a poly keypress event. | |
bool | sendControlChange (uint8_t channel, uint8_t key, uint8_t value) |
Send a control change event. | |
bool | sendProgramChange (uint8_t channel, uint8_t program) |
Send a program change event. | |
bool | sendChannelPressure (uint8_t channel, uint8_t pressure) |
Send a channel pressure event. | |
bool | sendPitchBend (uint8_t channel, uint16_t value) |
Send a control change event. | |
bool | sendSingleByte (uint8_t data) |
Send a single byte event. |
Detailed Description
A class to communicate a USB MIDI device.
Definition at line 31 of file USBHostMIDI.h.
Constructor & Destructor Documentation
USBHostMIDI | ( | ) |
Constructor.
Definition at line 27 of file USBHostMIDI.cpp.
Member Function Documentation
void attachCableEvent | ( | void(*)(uint8_t, uint8_t, uint8_t) | fn ) |
Attach a callback called when cable event is received.
- Parameters:
-
ptr function pointer prototype: void onCableEvent(uint8_t data1, uint8_t data2, uint8_t data3);
Definition at line 68 of file USBHostMIDI.h.
void attachChannelPressure | ( | void(*)(uint8_t, uint8_t) | fn ) |
Attach a callback called when channel pressure is received.
- Parameters:
-
ptr function pointer prototype: void onChannelPressure(uint8_t channel, uint8_t pressure);
Definition at line 158 of file USBHostMIDI.h.
void attachControlChange | ( | void(*)(uint8_t, uint8_t, uint8_t) | fn ) |
Attach a callback called when control change is received.
- Parameters:
-
ptr function pointer prototype: void onControlChange(uint8_t channel, uint8_t key, uint8_t value);
Definition at line 138 of file USBHostMIDI.h.
void attachMiscellaneousFunctionCode | ( | void(*)(uint8_t, uint8_t, uint8_t) | fn ) |
Attach a callback called when miscellaneous function code is received.
- Parameters:
-
ptr function pointer prototype: void onMiscellaneousFunctionCode(uint8_t data1, uint8_t data2, uint8_t data3);
Definition at line 58 of file USBHostMIDI.h.
void attachNoteOff | ( | void(*)(uint8_t, uint8_t, uint8_t) | fn ) |
Attach a callback called when note off is received.
- Parameters:
-
ptr function pointer prototype: void onNoteOff(uint8_t channel, uint8_t note, uint8_t velocity);
Definition at line 118 of file USBHostMIDI.h.
void attachNoteOn | ( | void(*)(uint8_t, uint8_t, uint8_t) | fn ) |
Attach a callback called when note on is received.
- Parameters:
-
ptr function pointer prototype: void onNoteOn(uint8_t channel, uint8_t note, uint8_t velocity);
Definition at line 108 of file USBHostMIDI.h.
void attachPitchBend | ( | void(*)(uint8_t, uint16_t) | fn ) |
Attach a callback called when pitch bend is received.
- Parameters:
-
ptr function pointer prototype: void onPitchBend(uint8_t channel, uint16_t value);
Definition at line 168 of file USBHostMIDI.h.
void attachPolyKeyPress | ( | void(*)(uint8_t, uint8_t, uint8_t) | fn ) |
Attach a callback called when poly keypress is received.
- Parameters:
-
ptr function pointer prototype: void onPolyKeyPress(uint8_t channel, uint8_t note, uint8_t pressure);
Definition at line 128 of file USBHostMIDI.h.
void attachProgramChange | ( | void(*)(uint8_t, uint8_t) | fn ) |
Attach a callback called when program change is received.
- Parameters:
-
ptr function pointer prototype: void onProgramChange(uint8_t channel, uint8_t program);
Definition at line 148 of file USBHostMIDI.h.
void attachSingleByte | ( | void(*)(uint8_t) | fn ) |
Attach a callback called when single byte is received.
- Parameters:
-
ptr function pointer prototype: void onSingleByte(uint8_t value);
Definition at line 178 of file USBHostMIDI.h.
void attachSystemCommonThreeBytes | ( | void(*)(uint8_t, uint8_t, uint8_t) | fn ) |
Attach a callback called when system exclusive is received.
- Parameters:
-
ptr function pointer prototype: void onSystemCommonThreeBytes(uint8_t data1, uint8_t data2, uint8_t data3);
Definition at line 88 of file USBHostMIDI.h.
void attachSystemCommonTwoBytes | ( | void(*)(uint8_t, uint8_t) | fn ) |
Attach a callback called when system exclusive is received.
- Parameters:
-
ptr function pointer prototype: void onSystemCommonTwoBytes(uint8_t data1, uint8_t data2);
Definition at line 78 of file USBHostMIDI.h.
void attachSystemExclusive | ( | void(*)(uint8_t *, uint16_t, bool) | fn ) |
Attach a callback called when system exclusive is received.
- Parameters:
-
ptr function pointer prototype: void onSystemExclusive(uint8_t *data, uint16_t length, bool hasNextData);
Definition at line 98 of file USBHostMIDI.h.
bool connect | ( | ) |
Try to connect a midi device.
- Returns:
- true if connection was successful
Definition at line 48 of file USBHostMIDI.cpp.
bool connected | ( | ) |
Check if a USB MIDI device is connected.
- Returns:
- true if a midi device is connected
Definition at line 44 of file USBHostMIDI.cpp.
bool sendCableEvent | ( | uint8_t | data1, |
uint8_t | data2, | ||
uint8_t | data3 | ||
) |
Send a cable event with 3 bytes event.
- Parameters:
-
data1 0-255 data2 0-255 data3 0-255
- Returns:
- true if message sent successfully
Definition at line 219 of file USBHostMIDI.cpp.
bool sendChannelPressure | ( | uint8_t | channel, |
uint8_t | pressure | ||
) |
Send a channel pressure event.
- Parameters:
-
channel 0-15 pressure 0-127
- Returns:
- true if message sent successfully
Definition at line 313 of file USBHostMIDI.cpp.
bool sendControlChange | ( | uint8_t | channel, |
uint8_t | key, | ||
uint8_t | value | ||
) |
Send a control change event.
- Parameters:
-
channel 0-15 key 0-127 value 0-127
- Returns:
- true if message sent successfully
Definition at line 305 of file USBHostMIDI.cpp.
bool sendMiscellaneousFunctionCode | ( | uint8_t | data1, |
uint8_t | data2, | ||
uint8_t | data3 | ||
) |
Send a cable event with 3 bytes event.
- Parameters:
-
data1 0-255 data2 0-255 data3 0-255
- Returns:
- true if message sent successfully
Definition at line 215 of file USBHostMIDI.cpp.
bool sendNoteOff | ( | uint8_t | channel, |
uint8_t | note, | ||
uint8_t | velocity | ||
) |
Send a note off event.
- Parameters:
-
channel 0-15 note 0-127 velocity 0-127
- Returns:
- true if message sent successfully
Definition at line 293 of file USBHostMIDI.cpp.
bool sendNoteOn | ( | uint8_t | channel, |
uint8_t | note, | ||
uint8_t | velocity | ||
) |
Send a note on event.
- Parameters:
-
channel 0-15 note 0-127 velocity 0-127 (0 means note off)
- Returns:
- true if message sent successfully
Definition at line 297 of file USBHostMIDI.cpp.
bool sendPitchBend | ( | uint8_t | channel, |
uint16_t | value | ||
) |
Send a control change event.
- Parameters:
-
channel 0-15 key 0(lower)-8191(center)-16383(higher)
- Returns:
- true if message sent successfully
Definition at line 317 of file USBHostMIDI.cpp.
bool sendPolyKeyPress | ( | uint8_t | channel, |
uint8_t | note, | ||
uint8_t | pressure | ||
) |
Send a poly keypress event.
- Parameters:
-
channel 0-15 note 0-127 pressure 0-127
- Returns:
- true if message sent successfully
Definition at line 301 of file USBHostMIDI.cpp.
bool sendProgramChange | ( | uint8_t | channel, |
uint8_t | program | ||
) |
Send a program change event.
- Parameters:
-
channel 0-15 program 0-127
- Returns:
- true if message sent successfully
Definition at line 309 of file USBHostMIDI.cpp.
bool sendSingleByte | ( | uint8_t | data ) |
Send a single byte event.
- Parameters:
-
data 0-255
- Returns:
- true if message sent successfully
Definition at line 321 of file USBHostMIDI.cpp.
bool sendSystemCommmonThreeBytes | ( | uint8_t | data1, |
uint8_t | data2, | ||
uint8_t | data3 | ||
) |
Send a system common message with 3 bytes event.
- Parameters:
-
data1 0-255 data2 0-255 data3 0-255
- Returns:
- true if message sent successfully
Definition at line 227 of file USBHostMIDI.cpp.
bool sendSystemCommmonTwoBytes | ( | uint8_t | data1, |
uint8_t | data2 | ||
) |
Send a system common message with 2 bytes event.
- Parameters:
-
data1 0-255 data2 0-255
- Returns:
- true if message sent successfully
Definition at line 223 of file USBHostMIDI.cpp.
bool sendSystemExclusive | ( | uint8_t * | buffer, |
int | length | ||
) |
Send a system exclusive event.
- Parameters:
-
buffer,starts with 0xF0, and end with 0xf7 length
- Returns:
- true if message sent successfully
Definition at line 231 of file USBHostMIDI.cpp.
Generated on Tue Jul 12 2022 13:32:26 by
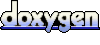