USBHost library. NOTE: This library is only officially supported on the LPC1768 platform. For more information, please see the handbook page.
Dependencies: FATFileSystem mbed-rtos
Dependents: BTstack WallbotWii SD to Flash Data Transfer USBHost-MSD_HelloWorld ... more
USBHostMIDI.h
00001 /* Copyright (c) 2014 mbed.org, MIT License 00002 * 00003 * Permission is hereby granted, free of charge, to any person obtaining a copy of this software 00004 * and associated documentation files (the "Software"), to deal in the Software without 00005 * restriction, including without limitation the rights to use, copy, modify, merge, publish, 00006 * distribute, sublicense, and/or sell copies of the Software, and to permit persons to whom the 00007 * Software is furnished to do so, subject to the following conditions: 00008 * 00009 * The above copyright notice and this permission notice shall be included in all copies or 00010 * substantial portions of the Software. 00011 * 00012 * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED, INCLUDING 00013 * BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND 00014 * NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, 00015 * DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00016 * OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE. 00017 */ 00018 00019 #ifndef USBHOSTMIDI_H 00020 #define USBHOSTMIDI_H 00021 00022 #include "USBHostConf.h" 00023 00024 #if USBHOST_MIDI 00025 00026 #include "USBHost.h" 00027 00028 /** 00029 * A class to communicate a USB MIDI device 00030 */ 00031 class USBHostMIDI : public IUSBEnumerator { 00032 public: 00033 /** 00034 * Constructor 00035 */ 00036 USBHostMIDI(); 00037 00038 /** 00039 * Check if a USB MIDI device is connected 00040 * 00041 * @returns true if a midi device is connected 00042 */ 00043 bool connected(); 00044 00045 /** 00046 * Try to connect a midi device 00047 * 00048 * @return true if connection was successful 00049 */ 00050 bool connect(); 00051 00052 /** 00053 * Attach a callback called when miscellaneous function code is received 00054 * 00055 * @param ptr function pointer 00056 * prototype: void onMiscellaneousFunctionCode(uint8_t data1, uint8_t data2, uint8_t data3); 00057 */ 00058 inline void attachMiscellaneousFunctionCode(void (*fn)(uint8_t, uint8_t, uint8_t)) { 00059 miscellaneousFunctionCode = fn; 00060 } 00061 00062 /** 00063 * Attach a callback called when cable event is received 00064 * 00065 * @param ptr function pointer 00066 * prototype: void onCableEvent(uint8_t data1, uint8_t data2, uint8_t data3); 00067 */ 00068 inline void attachCableEvent(void (*fn)(uint8_t, uint8_t, uint8_t)) { 00069 cableEvent = fn; 00070 } 00071 00072 /** 00073 * Attach a callback called when system exclusive is received 00074 * 00075 * @param ptr function pointer 00076 * prototype: void onSystemCommonTwoBytes(uint8_t data1, uint8_t data2); 00077 */ 00078 inline void attachSystemCommonTwoBytes(void (*fn)(uint8_t, uint8_t)) { 00079 systemCommonTwoBytes = fn; 00080 } 00081 00082 /** 00083 * Attach a callback called when system exclusive is received 00084 * 00085 * @param ptr function pointer 00086 * prototype: void onSystemCommonThreeBytes(uint8_t data1, uint8_t data2, uint8_t data3); 00087 */ 00088 inline void attachSystemCommonThreeBytes(void (*fn)(uint8_t, uint8_t, uint8_t)) { 00089 systemCommonThreeBytes = fn; 00090 } 00091 00092 /** 00093 * Attach a callback called when system exclusive is received 00094 * 00095 * @param ptr function pointer 00096 * prototype: void onSystemExclusive(uint8_t *data, uint16_t length, bool hasNextData); 00097 */ 00098 inline void attachSystemExclusive(void (*fn)(uint8_t *, uint16_t, bool)) { 00099 systemExclusive = fn; 00100 } 00101 00102 /** 00103 * Attach a callback called when note on is received 00104 * 00105 * @param ptr function pointer 00106 * prototype: void onNoteOn(uint8_t channel, uint8_t note, uint8_t velocity); 00107 */ 00108 inline void attachNoteOn(void (*fn)(uint8_t, uint8_t, uint8_t)) { 00109 noteOn = fn; 00110 } 00111 00112 /** 00113 * Attach a callback called when note off is received 00114 * 00115 * @param ptr function pointer 00116 * prototype: void onNoteOff(uint8_t channel, uint8_t note, uint8_t velocity); 00117 */ 00118 inline void attachNoteOff(void (*fn)(uint8_t, uint8_t, uint8_t)) { 00119 noteOff = fn; 00120 } 00121 00122 /** 00123 * Attach a callback called when poly keypress is received 00124 * 00125 * @param ptr function pointer 00126 * prototype: void onPolyKeyPress(uint8_t channel, uint8_t note, uint8_t pressure); 00127 */ 00128 inline void attachPolyKeyPress(void (*fn)(uint8_t, uint8_t, uint8_t)) { 00129 polyKeyPress = fn; 00130 } 00131 00132 /** 00133 * Attach a callback called when control change is received 00134 * 00135 * @param ptr function pointer 00136 * prototype: void onControlChange(uint8_t channel, uint8_t key, uint8_t value); 00137 */ 00138 inline void attachControlChange(void (*fn)(uint8_t, uint8_t, uint8_t)) { 00139 controlChange = fn; 00140 } 00141 00142 /** 00143 * Attach a callback called when program change is received 00144 * 00145 * @param ptr function pointer 00146 * prototype: void onProgramChange(uint8_t channel, uint8_t program); 00147 */ 00148 inline void attachProgramChange(void (*fn)(uint8_t, uint8_t)) { 00149 programChange = fn; 00150 } 00151 00152 /** 00153 * Attach a callback called when channel pressure is received 00154 * 00155 * @param ptr function pointer 00156 * prototype: void onChannelPressure(uint8_t channel, uint8_t pressure); 00157 */ 00158 inline void attachChannelPressure(void (*fn)(uint8_t, uint8_t)) { 00159 channelPressure = fn; 00160 } 00161 00162 /** 00163 * Attach a callback called when pitch bend is received 00164 * 00165 * @param ptr function pointer 00166 * prototype: void onPitchBend(uint8_t channel, uint16_t value); 00167 */ 00168 inline void attachPitchBend(void (*fn)(uint8_t, uint16_t)) { 00169 pitchBend = fn; 00170 } 00171 00172 /** 00173 * Attach a callback called when single byte is received 00174 * 00175 * @param ptr function pointer 00176 * prototype: void onSingleByte(uint8_t value); 00177 */ 00178 inline void attachSingleByte(void (*fn)(uint8_t)) { 00179 singleByte = fn; 00180 } 00181 00182 /** 00183 * Send a cable event with 3 bytes event 00184 * 00185 * @param data1 0-255 00186 * @param data2 0-255 00187 * @param data3 0-255 00188 * @return true if message sent successfully 00189 */ 00190 bool sendMiscellaneousFunctionCode(uint8_t data1, uint8_t data2, uint8_t data3); 00191 00192 /** 00193 * Send a cable event with 3 bytes event 00194 * 00195 * @param data1 0-255 00196 * @param data2 0-255 00197 * @param data3 0-255 00198 * @return true if message sent successfully 00199 */ 00200 bool sendCableEvent(uint8_t data1, uint8_t data2, uint8_t data3); 00201 00202 /** 00203 * Send a system common message with 2 bytes event 00204 * 00205 * @param data1 0-255 00206 * @param data2 0-255 00207 * @return true if message sent successfully 00208 */ 00209 bool sendSystemCommmonTwoBytes(uint8_t data1, uint8_t data2); 00210 00211 /** 00212 * Send a system common message with 3 bytes event 00213 * 00214 * @param data1 0-255 00215 * @param data2 0-255 00216 * @param data3 0-255 00217 * @return true if message sent successfully 00218 */ 00219 bool sendSystemCommmonThreeBytes(uint8_t data1, uint8_t data2, uint8_t data3); 00220 00221 /** 00222 * Send a system exclusive event 00223 * 00224 * @param buffer, starts with 0xF0, and end with 0xf7 00225 * @param length 00226 * @return true if message sent successfully 00227 */ 00228 bool sendSystemExclusive(uint8_t *buffer, int length); 00229 00230 /** 00231 * Send a note off event 00232 * 00233 * @param channel 0-15 00234 * @param note 0-127 00235 * @param velocity 0-127 00236 * @return true if message sent successfully 00237 */ 00238 bool sendNoteOff(uint8_t channel, uint8_t note, uint8_t velocity); 00239 00240 /** 00241 * Send a note on event 00242 * 00243 * @param channel 0-15 00244 * @param note 0-127 00245 * @param velocity 0-127 (0 means note off) 00246 * @return true if message sent successfully 00247 */ 00248 bool sendNoteOn(uint8_t channel, uint8_t note, uint8_t velocity); 00249 00250 /** 00251 * Send a poly keypress event 00252 * 00253 * @param channel 0-15 00254 * @param note 0-127 00255 * @param pressure 0-127 00256 * @return true if message sent successfully 00257 */ 00258 bool sendPolyKeyPress(uint8_t channel, uint8_t note, uint8_t pressure); 00259 00260 /** 00261 * Send a control change event 00262 * 00263 * @param channel 0-15 00264 * @param key 0-127 00265 * @param value 0-127 00266 * @return true if message sent successfully 00267 */ 00268 bool sendControlChange(uint8_t channel, uint8_t key, uint8_t value); 00269 00270 /** 00271 * Send a program change event 00272 * 00273 * @param channel 0-15 00274 * @param program 0-127 00275 * @return true if message sent successfully 00276 */ 00277 bool sendProgramChange(uint8_t channel, uint8_t program); 00278 00279 /** 00280 * Send a channel pressure event 00281 * 00282 * @param channel 0-15 00283 * @param pressure 0-127 00284 * @return true if message sent successfully 00285 */ 00286 bool sendChannelPressure(uint8_t channel, uint8_t pressure); 00287 00288 /** 00289 * Send a control change event 00290 * 00291 * @param channel 0-15 00292 * @param key 0(lower)-8191(center)-16383(higher) 00293 * @return true if message sent successfully 00294 */ 00295 bool sendPitchBend(uint8_t channel, uint16_t value); 00296 00297 /** 00298 * Send a single byte event 00299 * 00300 * @param data 0-255 00301 * @return true if message sent successfully 00302 */ 00303 bool sendSingleByte(uint8_t data); 00304 00305 protected: 00306 //From IUSBEnumerator 00307 virtual void setVidPid(uint16_t vid, uint16_t pid); 00308 virtual bool parseInterface(uint8_t intf_nb, uint8_t intf_class, uint8_t intf_subclass, uint8_t intf_protocol); //Must return true if the interface should be parsed 00309 virtual bool useEndpoint(uint8_t intf_nb, ENDPOINT_TYPE type, ENDPOINT_DIRECTION dir); //Must return true if the endpoint will be used 00310 00311 private: 00312 USBHost * host; 00313 USBDeviceConnected * dev; 00314 USBEndpoint * bulk_in; 00315 USBEndpoint * bulk_out; 00316 uint32_t size_bulk_in; 00317 uint32_t size_bulk_out; 00318 00319 bool dev_connected; 00320 00321 void init(); 00322 00323 uint8_t buf[64]; 00324 00325 void rxHandler(); 00326 00327 uint16_t sysExBufferPos; 00328 uint8_t sysExBuffer[64]; 00329 00330 void (*miscellaneousFunctionCode)(uint8_t, uint8_t, uint8_t); 00331 void (*cableEvent)(uint8_t, uint8_t, uint8_t); 00332 void (*systemCommonTwoBytes)(uint8_t, uint8_t); 00333 void (*systemCommonThreeBytes)(uint8_t, uint8_t, uint8_t); 00334 void (*systemExclusive)(uint8_t *, uint16_t, bool); 00335 void (*noteOff)(uint8_t, uint8_t, uint8_t); 00336 void (*noteOn)(uint8_t, uint8_t, uint8_t); 00337 void (*polyKeyPress)(uint8_t, uint8_t, uint8_t); 00338 void (*controlChange)(uint8_t, uint8_t, uint8_t); 00339 void (*programChange)(uint8_t, uint8_t); 00340 void (*channelPressure)(uint8_t, uint8_t); 00341 void (*pitchBend)(uint8_t, uint16_t); 00342 void (*singleByte)(uint8_t); 00343 00344 bool sendMidiBuffer(uint8_t data0, uint8_t data1, uint8_t data2, uint8_t data3); 00345 00346 int midi_intf; 00347 bool midi_device_found; 00348 00349 }; 00350 00351 #endif /* USBHOST_MIDI */ 00352 00353 #endif /* USBHOSTMIDI_H */
Generated on Tue Jul 12 2022 13:32:26 by
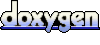