USBHost library. NOTE: This library is only officially supported on the LPC1768 platform. For more information, please see the handbook page.
Dependencies: FATFileSystem mbed-rtos
Dependents: BTstack WallbotWii SD to Flash Data Transfer USBHost-MSD_HelloWorld ... more
USBHost Class Reference
USBHost class This class is a singleton. More...
#include <USBHost.h>
Inherits USBHALHost.
Data Structures | |
class | Lock |
Instantiate to protect USB thread from accessing shared objects (USBConnectedDevices and Interfaces) More... | |
Public Member Functions | |
USB_TYPE | controlRead (USBDeviceConnected *dev, uint8_t requestType, uint8_t request, uint32_t value, uint32_t index, uint8_t *buf, uint32_t len) |
Control read: setup stage, data stage and status stage. | |
USB_TYPE | controlWrite (USBDeviceConnected *dev, uint8_t requestType, uint8_t request, uint32_t value, uint32_t index, uint8_t *buf, uint32_t len) |
Control write: setup stage, data stage and status stage. | |
USB_TYPE | bulkRead (USBDeviceConnected *dev, USBEndpoint *ep, uint8_t *buf, uint32_t len, bool blocking=true) |
Bulk read. | |
USB_TYPE | bulkWrite (USBDeviceConnected *dev, USBEndpoint *ep, uint8_t *buf, uint32_t len, bool blocking=true) |
Bulk write. | |
USB_TYPE | interruptRead (USBDeviceConnected *dev, USBEndpoint *ep, uint8_t *buf, uint32_t len, bool blocking=true) |
Interrupt read. | |
USB_TYPE | interruptWrite (USBDeviceConnected *dev, USBEndpoint *ep, uint8_t *buf, uint32_t len, bool blocking=true) |
Interrupt write. | |
USB_TYPE | enumerate (USBDeviceConnected *dev, IUSBEnumerator *pEnumerator) |
Enumerate a device. | |
USB_TYPE | resetDevice (USBDeviceConnected *dev) |
reset a specific device | |
USBDeviceConnected * | getDevice (uint8_t index) |
Get a device. | |
template<typename T > | |
void | registerDriver (USBDeviceConnected *dev, uint8_t intf, T *tptr, void(T::*mptr)(void)) |
register a driver into the host associated with a callback function called when the device is disconnected | |
void | registerDriver (USBDeviceConnected *dev, uint8_t intf, void(*fn)(void)) |
register a driver into the host associated with a callback function called when the device is disconnected | |
Static Public Member Functions | |
static USBHost * | getHostInst () |
Static method to create or retrieve the single USBHost instance. | |
Protected Member Functions | |
virtual void | transferCompleted (volatile uint32_t addr) |
Virtual method called when a transfer has been completed. | |
virtual void | deviceConnected (int hub, int port, bool lowSpeed, USBHostHub *hub_parent=NULL) |
Virtual method called when a device has been connected. | |
virtual void | deviceDisconnected (int hub, int port, USBHostHub *hub_parent, volatile uint32_t addr) |
Virtuel method called when a device has been disconnected. | |
void | init () |
Initialize host controller. | |
void | resetRootHub () |
reset the root hub | |
uint32_t | controlHeadED () |
return the value contained in the control HEAD ED register | |
uint32_t | bulkHeadED () |
return the value contained in the bulk HEAD ED register | |
uint32_t | interruptHeadED () |
return the value of the head interrupt ED contained in the HCCA | |
void | updateControlHeadED (uint32_t addr) |
Update the head ED for control transfers. | |
void | updateBulkHeadED (uint32_t addr) |
Update the head ED for bulk transfers. | |
void | updateInterruptHeadED (uint32_t addr) |
Update the head ED for interrupt transfers. | |
void | enableList (ENDPOINT_TYPE type) |
Enable List for the specified endpoint type. | |
bool | disableList (ENDPOINT_TYPE type) |
Disable List for the specified endpoint type. | |
volatile uint8_t * | getED () |
Find a memory section for a new ED. | |
volatile uint8_t * | getTD () |
Find a memory section for a new TD. | |
void | freeED (volatile uint8_t *ed) |
Release a previous memory section reserved for an ED. | |
void | freeTD (volatile uint8_t *td) |
Release a previous memory section reserved for an TD. | |
Friends | |
class | USBHostHub |
Detailed Description
USBHost class This class is a singleton.
All drivers have a reference on the static USBHost instance
Definition at line 34 of file USBHost.h.
Member Function Documentation
uint32_t bulkHeadED | ( | ) | [protected, inherited] |
return the value contained in the bulk HEAD ED register
- Returns:
- address of the bulk head ED
USB_TYPE bulkRead | ( | USBDeviceConnected * | dev, |
USBEndpoint * | ep, | ||
uint8_t * | buf, | ||
uint32_t | len, | ||
bool | blocking = true |
||
) |
Bulk read.
- Parameters:
-
dev the bulk transfer will be done for this device ep USBEndpoint which will be used to read a packet buf pointer on a buffer where will be store the data received len length of the transfer blocking if true, the read is blocking (wait for completion)
- Returns:
- status of the bulk read
Definition at line 1053 of file USBHost.cpp.
USB_TYPE bulkWrite | ( | USBDeviceConnected * | dev, |
USBEndpoint * | ep, | ||
uint8_t * | buf, | ||
uint32_t | len, | ||
bool | blocking = true |
||
) |
Bulk write.
- Parameters:
-
dev the bulk transfer will be done for this device ep USBEndpoint which will be used to write a packet buf pointer on a buffer which will be written len length of the transfer blocking if true, the write is blocking (wait for completion)
- Returns:
- status of the bulk write
Definition at line 1048 of file USBHost.cpp.
uint32_t controlHeadED | ( | ) | [protected, inherited] |
return the value contained in the control HEAD ED register
- Returns:
- address of the control Head ED
USB_TYPE controlRead | ( | USBDeviceConnected * | dev, |
uint8_t | requestType, | ||
uint8_t | request, | ||
uint32_t | value, | ||
uint32_t | index, | ||
uint8_t * | buf, | ||
uint32_t | len | ||
) |
Control read: setup stage, data stage and status stage.
- Parameters:
-
dev the control read will be done for this device requestType request type request request value value index index buf pointer on a buffer where will be store the data received len length of the transfer
- Returns:
- status of the control read
Definition at line 1147 of file USBHost.cpp.
USB_TYPE controlWrite | ( | USBDeviceConnected * | dev, |
uint8_t | requestType, | ||
uint8_t | request, | ||
uint32_t | value, | ||
uint32_t | index, | ||
uint8_t * | buf, | ||
uint32_t | len | ||
) |
Control write: setup stage, data stage and status stage.
- Parameters:
-
dev the control write will be done for this device requestType request type request request value value index index buf pointer on a buffer which will be written len length of the transfer
- Returns:
- status of the control write
Definition at line 1152 of file USBHost.cpp.
void deviceConnected | ( | int | hub, |
int | port, | ||
bool | lowSpeed, | ||
USBHostHub * | hub_parent = NULL |
||
) | [protected, virtual] |
Virtual method called when a device has been connected.
- Parameters:
-
hub hub number of the device port port number of the device lowSpeed 1 if low speed, 0 otherwise hub_parent reference on the parent hub
Implements USBHALHost.
Definition at line 402 of file USBHost.cpp.
void deviceDisconnected | ( | int | hub, |
int | port, | ||
USBHostHub * | hub_parent, | ||
volatile uint32_t | addr | ||
) | [protected, virtual] |
Virtuel method called when a device has been disconnected.
- Parameters:
-
hub hub number of the device port port number of the device addr list of the TDs which have been completed to dequeue freed TDs
Implements USBHALHost.
Definition at line 425 of file USBHost.cpp.
bool disableList | ( | ENDPOINT_TYPE | type ) | [protected, inherited] |
Disable List for the specified endpoint type.
- Parameters:
-
type disable the list of ENDPOINT_TYPE type
void enableList | ( | ENDPOINT_TYPE | type ) | [protected, inherited] |
Enable List for the specified endpoint type.
- Parameters:
-
type enable the list of ENDPOINT_TYPE type
USB_TYPE enumerate | ( | USBDeviceConnected * | dev, |
IUSBEnumerator * | pEnumerator | ||
) |
Enumerate a device.
- Parameters:
-
dev device which will be enumerated
- Returns:
- status of the enumeration
Definition at line 895 of file USBHost.cpp.
void freeED | ( | volatile uint8_t * | ed ) | [protected, inherited] |
Release a previous memory section reserved for an ED.
- Parameters:
-
ed address of the ED
void freeTD | ( | volatile uint8_t * | td ) | [protected, inherited] |
Release a previous memory section reserved for an TD.
- Parameters:
-
td address of the TD
USBDeviceConnected * getDevice | ( | uint8_t | index ) |
Get a device.
- Parameters:
-
index index of the device which will be returned
- Returns:
- pointer on the "index" device
Definition at line 563 of file USBHost.cpp.
volatile uint8_t* getED | ( | ) | [protected, inherited] |
Find a memory section for a new ED.
- Returns:
- the address of the new ED
USBHost * getHostInst | ( | ) | [static] |
Static method to create or retrieve the single USBHost instance.
Definition at line 388 of file USBHost.cpp.
volatile uint8_t* getTD | ( | ) | [protected, inherited] |
Find a memory section for a new TD.
- Returns:
- the address of the new TD
void init | ( | ) | [protected, inherited] |
Initialize host controller.
Enable USB interrupts. This part is not in the constructor because, this function calls a virtual method if a device is already connected
uint32_t interruptHeadED | ( | ) | [protected, inherited] |
return the value of the head interrupt ED contained in the HCCA
- Returns:
- address of the head interrupt ED contained in the HCCA
USB_TYPE interruptRead | ( | USBDeviceConnected * | dev, |
USBEndpoint * | ep, | ||
uint8_t * | buf, | ||
uint32_t | len, | ||
bool | blocking = true |
||
) |
Interrupt read.
- Parameters:
-
dev the bulk transfer will be done for this device ep USBEndpoint which will be used to write a packet buf pointer on a buffer which will be written len length of the transfer blocking if true, the read is blocking (wait for completion)
- Returns:
- status of the interrupt read
Definition at line 1063 of file USBHost.cpp.
USB_TYPE interruptWrite | ( | USBDeviceConnected * | dev, |
USBEndpoint * | ep, | ||
uint8_t * | buf, | ||
uint32_t | len, | ||
bool | blocking = true |
||
) |
Interrupt write.
- Parameters:
-
dev the bulk transfer will be done for this device ep USBEndpoint which will be used to write a packet buf pointer on a buffer which will be written len length of the transfer blocking if true, the write is blocking (wait for completion)
- Returns:
- status of the interrupt write
Definition at line 1058 of file USBHost.cpp.
void registerDriver | ( | USBDeviceConnected * | dev, |
uint8_t | intf, | ||
void(*)(void) | fn | ||
) |
void registerDriver | ( | USBDeviceConnected * | dev, |
uint8_t | intf, | ||
T * | tptr, | ||
void(T::*)(void) | mptr | ||
) |
USB_TYPE resetDevice | ( | USBDeviceConnected * | dev ) |
reset a specific device
- Parameters:
-
dev device which will be resetted
Definition at line 594 of file USBHost.cpp.
void resetRootHub | ( | ) | [protected, inherited] |
reset the root hub
void transferCompleted | ( | volatile uint32_t | addr ) | [protected, virtual] |
Virtual method called when a transfer has been completed.
- Parameters:
-
addr list of the TDs which have been completed
Implements USBHALHost.
Definition at line 328 of file USBHost.cpp.
void updateBulkHeadED | ( | uint32_t | addr ) | [protected, inherited] |
Update the head ED for bulk transfers.
void updateControlHeadED | ( | uint32_t | addr ) | [protected, inherited] |
Update the head ED for control transfers.
void updateInterruptHeadED | ( | uint32_t | addr ) | [protected, inherited] |
Update the head ED for interrupt transfers.
Generated on Tue Jul 12 2022 13:32:26 by
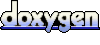