
Rtos API example
socket_api.h File Reference
6LoWPAN Library Socket API More...
Go to the source code of this file.
Data Structures | |
struct | socket_callback_t |
ICMP socket instruction. More... | |
struct | ns_ipv6_mreq |
Multicast group request used for setsockopt() More... | |
Functions | |
ns_cmsghdr_t * | NS_CMSG_NXTHDR (const ns_msghdr_t *msgh, const ns_cmsghdr_t *cmsg) |
Parse next control message from message by current control message header. | |
int8_t | socket_open (uint8_t protocol, uint16_t identifier, void(*passed_fptr)(void *)) |
Create and initialize a socket for communication. | |
int8_t | socket_close (int8_t socket) |
A function to close a socket. | |
int8_t | socket_listen (int8_t socket, uint8_t backlog) |
A function to set a socket to listening mode. | |
int8_t | socket_accept (int8_t socket_id, ns_address_t *addr, void(*passed_fptr)(void *)) |
A function to accept a new connection on an socket. | |
int8_t | socket_connect (int8_t socket, ns_address_t *address, uint8_t randomly_take_src_number) |
A function to connect to remote peer (TCP). | |
int8_t | socket_bind (int8_t socket, const ns_address_t *address) |
Bind socket to address. | |
int8_t | socket_bind2addrsel (int8_t socket, const ns_address_t *dst_address) |
Bind a local address to a socket based on the destination address and the address selection preferences. | |
int8_t | socket_shutdown (int8_t socket, uint8_t how) |
A function to shut down a connection. | |
int16_t | socket_send (int8_t socket, const void *buffer, uint16_t length) |
Send data via a connected socket by client. | |
int16_t | socket_read (int8_t socket, ns_address_t *src_addr, uint8_t *buffer, uint16_t length) |
A function to read received data buffer from a socket. | |
int16_t | socket_recv (int8_t socket, void *buffer, uint16_t length, int flags) |
A function to read received data buffer from a socket,. | |
int16_t | socket_recvfrom (int8_t socket, void *buffer, uint16_t length, int flags, ns_address_t *src_addr) |
A function to read received data buffer from a socket. | |
int16_t | socket_recvmsg (int8_t socket, ns_msghdr_t *msg, int flags) |
A function to read received message with ancillary data from a socket. | |
int16_t | socket_sendto (int8_t socket, const ns_address_t *address, const void *buffer, uint16_t length) |
A function to send UDP, TCP or raw ICMP data via the socket. | |
int16_t | socket_sendmsg (int8_t socket, const ns_msghdr_t *msg, int flags) |
A function to send UDP, TCP or raw ICMP data via the socket with or without ancillary data or destination address. | |
int8_t | socket_getsockname (int8_t socket, ns_address_t *address) |
A function to read local address and port for a bound socket. | |
int8_t | socket_getpeername (int8_t socket, ns_address_t *address) |
A function to read remote address and port for a connected socket. | |
int8_t | socket_getsockopt (int8_t socket, uint8_t level, uint8_t opt_name, void *opt_value, uint16_t *opt_len) |
Get an option for a socket. | |
Variables | |
const uint8_t | ns_in6addr_any [16] |
IPv6 wildcard address IN_ANY. | |
IPv6 socket options | |
IPv6 socket options summary | opt_name / cmsg_type | Data type | set/getsockopt | sendmsg | recvmsg | | :--------------------------: | :--------------: | :-------------: | :-----: | :-------------------------------: | | SOCKET_IPV6_TCLASS | int16_t | Yes | Yes | If enabled with RECVTCLASS | | SOCKET_IPV6_UNICAST_HOPS | int16_t | Yes | No | No | | SOCKET_IPV6_MULTICAST_HOPS | int16_t | Yes | No | No | | SOCKET_IPV6_ADDR_PREFERENCES | int | Yes | No | No | | SOCKET_IPV6_USE_MIN_MTU | int8_t | Yes | Yes | No | | SOCKET_IPV6_DONTFRAG | int8_t | Yes | Yes | No | | SOCKET_IPV6_FLOW_LABEL | int32_t | Yes | No | No | | SOCKET_IPV6_HOPLIMIT | int16_t | No | Yes | If enabled with RECVHOPLIMIT | | SOCKET_IPV6_PKTINFO | ns_in6_pktinfo_t | No | Yes | If enabled with RECVPKTINFO | | SOCKET_IPV6_RECVPKTINFO | bool | Yes | No | No | | SOCKET_IPV6_RECVHOPLIMIT | bool | Yes | No | No | | SOCKET_IPV6_RECVTCLASS | bool | Yes | No | No | | SOCKET_IPV6_MULTICAST_IF | int8_t | Yes | No | No | | SOCKET_IPV6_MULTICAST_LOOP | bool | Yes | Yes | No | | SOCKET_IPV6_JOIN_GROUP | ns_ipv6_mreq_t | Set only | No | No | | SOCKET_IPV6_LEAVE_GROUP | ns_ipv6_mreq_t | Set only | No | No | | SOCKET_BROADCAST_PAN | int8_t | Yes | No | No | | SOCKET_LINK_LAYER_SECURITY | int8_t | Yes | No | No | | SOCKET_INTERFACE_SELECT | int8_t | Yes | No | No | | |
typedef struct ns_ipv6_mreq | ns_ipv6_mreq_t |
Multicast group request used for setsockopt() | |
int8_t | socket_setsockopt (int8_t socket, uint8_t level, uint8_t opt_name, const void *opt_value, uint16_t opt_len) |
Set an option for a socket. |
Detailed Description
6LoWPAN Library Socket API
Common socket API
- socket_open(), A function to open a socket.
- socket_close(), A function to close a socket.
- socket_connect(), A function to connect to a remote peer.
- socket_bind(), A function to bind a local address or port or both.
- socket_getpeername(), A function to get remote address and port of a connected socket.
- socket_getsockname(), A function to get local address and port of a bound socket.
Socket read API at callback
- socket_read(), A function to read received data buffer from a socket.
- socket_recvmsg(), A function to read received data buffer from a socket to Posix defined message structure
Socket TX API
- socket_send(), A function to write data buffer to a socket.
- socket_sendto(), A function to write data to a specific destination in the socket.
- socket_sendmsg(), A function which support socket_send and socket_sendto functionality which supports ancillary data
TCP socket connection handle
- socket_listen(), A function to set the socket to listening mode.
- socket_accept(), A function to accept an incoming connection.
- socket_shutdown(), A function to shut down a connection.
Sockets are a common abstraction model for network communication and are used in most operating systems. 6LoWPAN Library API follows BSD socket API conventions closely with some extensions necessitated by the event-based scheduling model.
Library supports the following socket types: | Socket name | Socket description | | :------------: | :----------------------------: | | SOCKET_UDP | UDP socket type | | SOCKET_TCP | TCP socket type | | SOCKET_ICMP | ICMP raw socket type |
ICMP RAW socket instruction
An ICMP raw socket can be created with the function socket_open() and the identifier 0xffff. When using ICMP sockets, the minimum packet length is 4 bytes which is the 4 bytes of ICMP header. The first byte is for type, second for code and last two are for the checksum that must always be set to zero. The stack will calculate the checksum automatically before transmitting the packet. THe header is followed by the payload if there is any. NOTE: While it is perfectly legal to send an ICMP packet without payload, some packet sniffing softwares might regard such packets as malformed. In such a case, a dummy payload can be attached by providing a socket_sendto() function with a pointer to your desired data buffer. Moreover, the current implementation of the stack allows only certain ICMP types, for example ICMP echo, reply, destination unreachable, router advertisement, router solicitation. It will drop any other unimplemented types. For example, Private experimentation type (200) will be dropped by default.
| ICMP Type | ICMP Code | Checksum | Payload | Notes | | :-------: | :----------: | :-------: | :--------: | :--------------:| | 1 | 1 | 2 | n bytes | Length in bytes | | 0xXX | 0xXX | 0x00 0x00 | n bytes | Transmit | | 0xXX | 0xXX | 0xXX 0xXX | n bytes | Receive |
ICMP echo request with 4 bytes of payload (ping6).
| ICMP Type | ICMP Code | Checksum | Payload | | :-------: | :----------: | :-------: | :-----------------: | | 0x80 | 0x00 | 0x00 0x00 | 0x00 0x01 0x02 0x03 |
ICMP echo response for the message above.
| ICMP Type | ICMP Code | Checksum | Payload | | :-------: | :----------: | :-------: | :-----------------: | | 0x81 | 0x00 | 0xXX 0xXX | 0x00 0x01 0x02 0x03 |
Socket receiving
When there is data to read from the socket, a receive callback function is called with the event type parameter set to SOCKET_DATA. The application must read the received data with socket_read() or socket_recvmsg() during the callback because the stack will release the data when returning from the receive callback.
Socket event has event_type and socket_id fields. The receive callback function must be defined when socket is opened using socket_open() API.
All supported socket event types are listed here:
| Event Type | Value | Description | | :------------------------: | :---: | :-----------------------------------------------------------------: | | SOCKET_EVENT_MASK | 0xF0 | NC Socket event mask. | | SOCKET_DATA | 0x00 | Data received, read data length available in d_len field. | | SOCKET_CONNECT_DONE | 0x10 | TCP connection ready. | | SOCKET_CONNECT_FAIL | 0x20 | TCP connection failed. | | SOCKET_INCOMING_CONNECTION | 0x40 | TCP incoming connection on listening socket. | | SOCKET_TX_FAIL | 0x50 | Socket data send failed. | | SOCKET_CONNECT_CLOSED | 0x60 | TCP connection closed. | | SOCKET_CONNECTION_RESET | 0x70 | TCP connection reset. | | SOCKET_NO_ROUTE | 0x80 | No route available to destination. | | SOCKET_TX_DONE | 0x90 | UDP: link layer TX ready (d_len = length of datagram). | | | | TCP: some data acknowledged (d_len = data remaining in send queue) | | SOCKET_NO_RAM | 0xA0 | No RAM available. | | SOCKET_CONNECTION_PROBLEM | 0xB0 | TCP connection is retrying. |
How to use TCP sockets:
| API | Socket Type | Description | | :---------------------------: | :-----------: | :------------------------------------------------------------: | | socket_open() | Server/Client | Open socket to specific or dynamic port number. | | socket_shutdown() | Server/Client | Shut down opened TCP connection. | | socket_listen() | Server | Set server port to listen state. | | socket_accept() | Server | Accept a connection to a listening socket as a new socket. | | socket_connect() | Client | Connect client socket to specific destination. | | socket_close() | Server/Client | Closes the TCP Socket. | | socket_send() | Server/Client | Send data to peer. | | socket_recv() | Server/Client | Receive data from peer. |
When the TCP socket is opened it is in closed state. It must be set either to listen or to connect state before it can be used to receive or transmit data.
A socket can be set to listen mode with the socket_listen() function. After the call, the socket can accept an incoming connection from a remote host.
A TCP socket can be connected to a remote host with socket_connect() with correct arguments. After the function call, a (non-blocking) application must wait for the socket event to confirm the successful state change of the socket. After the successful state change, data can be sent using socket_send(). The connection can be shut down in either direction with socket_shutdown() function - shutting down write signals end-of-data to the peer.
How to use UDP and RAW socket:
A UDP socket is ready to receive and send data immediately after a successful call of socket_open() and a NET_READY event is received. Data can be transmitted with the socket_sendto() function. An ICMP socket works with same function call.
Definition in file socket_api.h.
Typedef Documentation
typedef struct ns_ipv6_mreq ns_ipv6_mreq_t |
Multicast group request used for setsockopt()
Function Documentation
ns_cmsghdr_t* NS_CMSG_NXTHDR | ( | const ns_msghdr_t * | msgh, |
const ns_cmsghdr_t * | cmsg | ||
) |
Parse next control message from message by current control message header.
- Parameters:
-
msgh Pointer for socket message. cmsg Pointer for last parsed control message
- Returns:
- Pointer to Next control message header , Could be NULL when no more control messages data.
Definition at line 111 of file socket_api_stub.c.
int8_t socket_accept | ( | int8_t | socket_id, |
ns_address_t * | addr, | ||
void(*)(void *) | passed_fptr | ||
) |
A function to accept a new connection on an socket.
- Parameters:
-
socket_id The socket ID of the listening socket. addr Either NULL pointer or pointer to structure where the remote address of the connecting host is copied. passed_fptr A function pointer to a function that is called whenever a data frame is received to the new socket.
- Returns:
- 0 or greater on success; return value is the new socket ID.
- -1 on failure.
- NS_EWOULDBLOCK if no pending connections.
int8_t socket_bind | ( | int8_t | socket, |
const ns_address_t * | address | ||
) |
Bind socket to address.
Used by the application to bind a socket to a port and/or an address. Binding of each of address and port can only be done once.
If address is ns_in6addr_any, the address binding is not changed. If port is 0, the port binding is not changed.
- Parameters:
-
socket Socket ID of the socket to bind. address Address structure containing the port and/or address to bind.
- Returns:
- 0 on success.
- -1 if the given address is NULL.
- -2 if the port is already bound to another socket.
- -3 if address is not us.
- -4 if the socket is already bound.
- -5 bind is not supported on this type of socket.
Definition at line 52 of file socket_api_stub.c.
int8_t socket_bind2addrsel | ( | int8_t | socket, |
const ns_address_t * | dst_address | ||
) |
Bind a local address to a socket based on the destination address and the address selection preferences.
Binding happens to the same address that socket_connect() would bind to. Reference: RFC5014 IPv6 Socket API for Source Address Selection.
- Parameters:
-
socket The socket ID. dst_address The destination address to which the socket wants to communicate.
- Returns:
- 0 on success.
- -1 if the given address is NULL or socket ID is invalid.
- -2 if the memory allocation failed.
- -3 if the socket is already bound to address.
- -4 if the interface cannot be found.
- -5 if the source address selection fails.
- -6 bind2addrsel is not supported on this type of socket.
int8_t socket_close | ( | int8_t | socket ) |
A function to close a socket.
- Parameters:
-
socket ID of the socket to be closed.
- Returns:
- 0 socket closed.
- -1 socket not closed.
Definition at line 28 of file socket_api_stub.c.
int8_t socket_connect | ( | int8_t | socket, |
ns_address_t * | address, | ||
uint8_t | randomly_take_src_number | ||
) |
A function to connect to remote peer (TCP).
- Parameters:
-
socket The socket ID. address The address of a remote peer. randomly_take_src_number Ignored - random local port is always chosen if not yet bound
- Returns:
- 0 on success.
- -1 in case of an invalid socket ID or parameter.
- -2 if memory allocation fails.
- -3 if the socket is in listening state.
- -4 if the socket is already connected.
- -5 connect is not supported on this type of socket.
- -6 if the TCP session state is wrong.
- -7 if the source address selection fails.
Definition at line 44 of file socket_api_stub.c.
int8_t socket_getpeername | ( | int8_t | socket, |
ns_address_t * | address | ||
) |
A function to read remote address and port for a connected socket.
- Parameters:
-
socket The socket ID. address A pointer to the address structure where the remote address information is written to.
- Returns:
- 0 on success.
- -1 if no socket is found.
- -2 if no socket is not connected.
Definition at line 73 of file socket_api_stub.c.
int8_t socket_getsockname | ( | int8_t | socket, |
ns_address_t * | address | ||
) |
A function to read local address and port for a bound socket.
This call writes ns_in6addr_any if address is not bound and 0 if the port is not bound.
- Parameters:
-
socket The socket ID. address A pointer to the address structure where the local address information is written to.
- Returns:
- 0 on success.
- -1 if no socket is found.
int8_t socket_getsockopt | ( | int8_t | socket, |
uint8_t | level, | ||
uint8_t | opt_name, | ||
void * | opt_value, | ||
uint16_t * | opt_len | ||
) |
Get an option for a socket.
Used to read miscellaneous options for a socket. Supported levels and names defined above. If the buffer is smaller than the option, the output is silently truncated; otherwise opt_len is modified to indicate the actual length.
- Parameters:
-
socket The socket ID. level The protocol level. opt_name The option name (interpretation depends on level). See OPTNAMES_IPV6. opt_value A pointer to output buffer. opt_len A pointer to length of output buffer; updated on exit.
- Returns:
- 0 on success.
- -1 invalid socket ID.
- -2 invalid/unsupported option.
Definition at line 89 of file socket_api_stub.c.
int8_t socket_listen | ( | int8_t | socket, |
uint8_t | backlog | ||
) |
A function to set a socket to listening mode.
- Parameters:
-
socket The socket ID. backlog The pending connections queue size.
- Returns:
- 0 on success.
- -1 on failure.
Definition at line 36 of file socket_api_stub.c.
int8_t socket_open | ( | uint8_t | protocol, |
uint16_t | identifier, | ||
void(*)(void *) | passed_fptr | ||
) |
Create and initialize a socket for communication.
- Parameters:
-
protocol Defines the protocol to use. identifier The socket port. 0 indicates that port is not specified and it will be selected automatically when using the socket. passed_fptr A function pointer to a function that is called whenever a data frame is received to this socket.
- Returns:
- 0 or greater on success; Return value is the socket ID.
- -1 on failure.
- -2 on port reserved.
Definition at line 18 of file socket_api_stub.c.
int16_t socket_read | ( | int8_t | socket, |
ns_address_t * | src_addr, | ||
uint8_t * | buffer, | ||
uint16_t | length | ||
) |
A function to read received data buffer from a socket.
Used by the application to get data from a socket. See socket_recvfrom() for more details.
This is equivalent to socket_recvfrom, except that it passes the flag NS_MSG_LEGACY0, which modifies the return behaviour for zero data.
- Parameters:
-
socket The socket ID. src_addr A pointer to a structure where the sender's address is stored. May be NULL if not required. buffer A pointer to an array where the read data is written to. length The maximum length of the allocated buffer.
- Returns:
- >0 indicates the length of the data copied to buffer.
- 0 if no data was read (includes zero-length datagram, end of stream and no data currently available)
- -1 invalid input parameters.
Definition at line 61 of file socket_api_stub.c.
int16_t socket_recv | ( | int8_t | socket, |
void * | buffer, | ||
uint16_t | length, | ||
int | flags | ||
) |
A function to read received data buffer from a socket,.
Equivalent to socket_recvfrom with src_address set to NULL.
- Parameters:
-
socket The socket ID. buffer A pointer to an array where the read data is written to. length The maximum length of the allocated buffer. flags Flags for read call
- Returns:
- as for socket_recvfrom
int16_t socket_recvfrom | ( | int8_t | socket, |
void * | buffer, | ||
uint16_t | length, | ||
int | flags, | ||
ns_address_t * | src_addr | ||
) |
A function to read received data buffer from a socket.
Used by the application to get data from a socket.
This has two modes of operation.
1) For non-stream sockets, if the receive queue is disabled (set to 0 via SOCKET_SO_RCVBUF), which is the non-stream default and original Nanostack behaviour, then applications receive exactly one SOCKET_DATA callback per datagram, indicating that datagram's length. They must make 1 read call in that callback, and they will be given the data. If not read, the datagram is discarded on return from the callback.
2) Otherwise - stream sockets or SOCKET_SO_RCVBUF non-zero - behaviour is akin to traditional BSD. SOCKET_DATA callbacks occur when new data arrives, and read calls can be made any time. Data will be queued to an extent determined by the receive buffer size. The length in the data callback is the total amount of data in the receive queue - possibly multiple datagrams.
- Parameters:
-
socket The socket ID. buffer A pointer to an array where the read data is written to. length The maximum length of the allocated buffer. flags Flags for read call src_addr A pointer to a structure where the sender's address is stored. May be NULL if not required.
The returned length is normally the length of data actually written to the buffer; if NS_MSG_TRUNC is set in flags, then for non-stream sockets, the actual datagram length is returned instead, which may be larger than the buffer size.
Return values assume flag NS_MSG_LEGACY0 is not set - if it is set, they are as per socket_read().
- Returns:
- >0 indicates the length of the data copied to buffer (or original datagram size)
- 0 if end of stream or zero-length datagram
- -1 invalid input parameters.
- NS_EWOULDBLOCK if no data is currently available
int16_t socket_recvmsg | ( | int8_t | socket, |
ns_msghdr_t * | msg, | ||
int | flags | ||
) |
A function to read received message with ancillary data from a socket.
Used by the application to get data from a socket. See socket_recvfrom for details of the two delivery mechanisms.
Ancillary data must request by socket_setsockopt().
msg->msg_controllen is updated to indicate actual length of ancillary data output
- Parameters:
-
socket The socket ID. msg A pointer to a structure where messages is stored with or without ancillary data flags A flags for message read.
- Returns:
- as for socket_recvfrom
Definition at line 106 of file socket_api_stub.c.
int16_t socket_send | ( | int8_t | socket, |
const void * | buffer, | ||
uint16_t | length | ||
) |
Send data via a connected socket by client.
Note: The socket connection must be ready before using this function. The stack uses automatically the address of the remote connected host as the destination address for the packet.
This call is equivalent to socket_sendto() with address set to NULL - see that call for more details.
- Parameters:
-
socket The socket ID. buffer A pointer to data. length Data length.
int16_t socket_sendmsg | ( | int8_t | socket, |
const ns_msghdr_t * | msg, | ||
int | flags | ||
) |
A function to send UDP, TCP or raw ICMP data via the socket with or without ancillary data or destination address.
Used by the application to send data message header support also vector list socket_send() and socket_sendto() use this functionality internally.
- Parameters:
-
socket The socket ID. msg A pointer to the Message header which include address, payload and ancillary data. flags A flags for message send (eg NS_MSG_LEGACY0)
Messages destination address is defined by msg->msg_name which must be ns_address_t. If msg->msg_nme is NULL socket select connected address
Messages payload and length is defined msg->msg_iov and msg->msg_iovlen. API support to send multiple data vector.
Supported ancillary data for send defined by msg->msg_control and msg->msg_controllen.
msg->msg_flags is unused, and need not be initialised.
The following main return values assume flag NS_MSG_LEGACY0 is not set - if it is set, they are as per socket_sendto().
- Returns:
- length if entire amount written (which could be 0)
- value >0 and <length if partial amount written (stream only)
- NS_EWOULDBLOCK if nothing written due to lack of queue space.
Error returns:
- Returns:
- -1 Invalid socket ID.
- -2 Socket memory allocation fail.
- -3 TCP state not established or address scope not defined .
- -4 Unknown interface.
- -5 Socket not connected
- -6 Packet too short (ICMP raw socket error).
Definition at line 97 of file socket_api_stub.c.
int16_t socket_sendto | ( | int8_t | socket, |
const ns_address_t * | address, | ||
const void * | buffer, | ||
uint16_t | length | ||
) |
A function to send UDP, TCP or raw ICMP data via the socket.
Used by the application to send data.
The return of 0 on success is unconventional, and obtained by passing NS_MSG_LEGACY0 to socket_sendmsg internally - to get conventional return values, you can use socket_sendmsg() instead.
- Parameters:
-
socket The socket ID. address A pointer to the destination address information. buffer A pointer to data to be sent. length Length of the data to be sent.
- Returns:
- 0 On success (whole packet queued)
- NS_EWOULDBLOCK if nothing written due to lack of queue space.
Error returns:
int8_t socket_setsockopt | ( | int8_t | socket, |
uint8_t | level, | ||
uint8_t | opt_name, | ||
const void * | opt_value, | ||
uint16_t | opt_len | ||
) |
Set an option for a socket.
Used to specify miscellaneous options for a socket. Supported levels and names defined above.
- Parameters:
-
socket The socket ID. level The protocol level. opt_name The option name (interpretation depends on level). See OPTNAMES_SOCKET and OPTNAMES_IPV6. opt_value A pointer to value for the specified option. opt_len Size of the data pointed to by the value.
- Returns:
- 0 on success.
- -1 invalid socket ID.
- -2 invalid/unsupported option.
- -3 invalid option value.
Definition at line 81 of file socket_api_stub.c.
int8_t socket_shutdown | ( | int8_t | socket, |
uint8_t | how | ||
) |
A function to shut down a connection.
- Parameters:
-
socket The ID of the socket to be shut down. how How socket is to be shut down, one of SOCKET_SHUT_XX.
- Returns:
- 0 on success.
- -1 if the given socket ID is not found, if the socket type is wrong or TCP layer returns a failure.
- -2 if socket is not connected.
Variable Documentation
const uint8_t ns_in6addr_any[16] |
IPv6 wildcard address IN_ANY.
Definition at line 8 of file socket_api_stub.c.
Generated on Sun Jul 17 2022 08:25:36 by
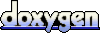