
Rtos API example
Embed:
(wiki syntax)
Show/hide line numbers
socket_api_stub.c
00001 /* 00002 * Copyright (c) 2015-2017 ARM Limited. All Rights Reserved. 00003 */ 00004 #include "socket_api.h" 00005 #include "socket_api_stub.h" 00006 00007 socket_api_stub_data_t socket_api_stub; 00008 const uint8_t ns_in6addr_any[16] = {0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0}; 00009 00010 int8_t socket_raw_open(void (*passed_fptr)(void *)) 00011 { 00012 if( socket_api_stub.counter >= 0){ 00013 return socket_api_stub.values[socket_api_stub.counter--]; 00014 } 00015 00016 return socket_api_stub.int8_value; 00017 } 00018 int8_t socket_open(uint8_t protocol, uint16_t identifier, void (*passed_fptr)(void *)) 00019 { 00020 socket_api_stub.recv_cb = passed_fptr; 00021 if( socket_api_stub.counter >= 0){ 00022 return socket_api_stub.values[socket_api_stub.counter--]; 00023 } 00024 00025 return socket_api_stub.int8_value; 00026 } 00027 00028 int8_t socket_close(int8_t socket) 00029 { 00030 if( socket_api_stub.counter >= 0){ 00031 return socket_api_stub.values[socket_api_stub.counter--]; 00032 } 00033 00034 return socket_api_stub.int8_value; 00035 } 00036 int8_t socket_listen(int8_t socket, uint8_t backlog) 00037 { 00038 if( socket_api_stub.counter >= 0){ 00039 return socket_api_stub.values[socket_api_stub.counter--]; 00040 } 00041 00042 return socket_api_stub.int8_value; 00043 } 00044 int8_t socket_connect(int8_t socket, ns_address_t *address, uint8_t randomly_take_src_number) 00045 { 00046 if( socket_api_stub.counter >= 0){ 00047 return socket_api_stub.values[socket_api_stub.counter--]; 00048 } 00049 00050 return socket_api_stub.int8_value; 00051 } 00052 int8_t socket_bind(int8_t socket, const ns_address_t *address) 00053 { 00054 if( socket_api_stub.counter >= 0){ 00055 return socket_api_stub.values[socket_api_stub.counter--]; 00056 } 00057 00058 return socket_api_stub.int8_value; 00059 } 00060 00061 int16_t socket_read(int8_t socket, ns_address_t *address, uint8_t *buffer, uint16_t length) 00062 { 00063 if( address ){ 00064 memset(&address->address, 0, 16); 00065 address->identifier = 0; 00066 } 00067 if( socket_api_stub.counter >= 0){ 00068 return socket_api_stub.values[socket_api_stub.counter--]; 00069 } 00070 00071 return socket_api_stub.int8_value; 00072 } 00073 int8_t socket_getpeername(int8_t socket, ns_address_t *address) 00074 { 00075 if( socket_api_stub.counter >= 0){ 00076 return socket_api_stub.values[socket_api_stub.counter--]; 00077 } 00078 00079 return socket_api_stub.int8_value; 00080 } 00081 int8_t socket_setsockopt(int8_t socket, uint8_t level, uint8_t opt_name, const void *opt_value, uint16_t opt_len) 00082 { 00083 if( socket_api_stub.counter >= 0){ 00084 return socket_api_stub.values[socket_api_stub.counter--]; 00085 } 00086 00087 return socket_api_stub.int8_value; 00088 } 00089 int8_t socket_getsockopt(int8_t socket, uint8_t level, uint8_t opt_name, void *opt_value, uint16_t *opt_len) 00090 { 00091 if( socket_api_stub.counter >= 0){ 00092 return socket_api_stub.values[socket_api_stub.counter--]; 00093 } 00094 00095 return socket_api_stub.int8_value; 00096 } 00097 int16_t socket_sendmsg(int8_t socket, const ns_msghdr_t *msg, int flags) 00098 { 00099 if( socket_api_stub.counter >= 0){ 00100 return socket_api_stub.values[socket_api_stub.counter--]; 00101 } 00102 00103 return socket_api_stub.int8_value; 00104 } 00105 00106 int16_t socket_recvmsg(int8_t socket, ns_msghdr_t *msg, int flags) 00107 { 00108 return -1; 00109 } 00110 00111 ns_cmsghdr_t *NS_CMSG_NXTHDR(const ns_msghdr_t *msgh, const ns_cmsghdr_t *cmsg) 00112 { 00113 return NULL; 00114 }
Generated on Sun Jul 17 2022 08:25:30 by
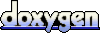