
Rtos API example
Embed:
(wiki syntax)
Show/hide line numbers
socket_api.h
Go to the documentation of this file.
00001 /* 00002 * Copyright (c) 2010-2017 ARM Limited. All rights reserved. 00003 * 00004 * SPDX-License-Identifier: LicenseRef-PBL 00005 * 00006 * Licensed under the Permissive Binary License, Version 1.0 (the "License"); you may not use this file except in compliance with the License. 00007 * You may obtain a copy of the License at 00008 * 00009 * https://www.mbed.com/licenses/PBL-1.0 00010 * 00011 * See the License for the specific language governing permissions and limitations under the License. 00012 * 00013 */ 00014 #ifndef _NS_SOCKET_API_H 00015 #define _NS_SOCKET_API_H 00016 00017 #ifdef __cplusplus 00018 extern "C" { 00019 #endif 00020 /** 00021 * \file socket_api.h 00022 * \brief 6LoWPAN Library Socket API 00023 * 00024 * \section socket-com Common socket API 00025 * - socket_open(), A function to open a socket. 00026 * - socket_close(), A function to close a socket. 00027 * - socket_connect(), A function to connect to a remote peer. 00028 * - socket_bind(), A function to bind a local address or port or both. 00029 * - socket_getpeername(), A function to get remote address and port of a connected socket. 00030 * - socket_getsockname(), A function to get local address and port of a bound socket. 00031 * 00032 * \section socket-read Socket read API at callback 00033 * - socket_read(), A function to read received data buffer from a socket. 00034 * - socket_recvmsg(), A function to read received data buffer from a socket to Posix defined message structure 00035 * 00036 * \section socket-tx Socket TX API 00037 * - socket_send(), A function to write data buffer to a socket. 00038 * - socket_sendto(), A function to write data to a specific destination in the socket. 00039 * - socket_sendmsg(), A function which support socket_send and socket_sendto functionality which supports ancillary data 00040 * 00041 * \section sock-connect TCP socket connection handle 00042 * - socket_listen(), A function to set the socket to listening mode. 00043 * - socket_accept(), A function to accept an incoming connection. 00044 * - socket_shutdown(), A function to shut down a connection. 00045 * 00046 * Sockets are a common abstraction model for network communication and are used in most operating systems. 00047 * 6LoWPAN Library API follows BSD socket API conventions closely with some extensions necessitated 00048 * by the event-based scheduling model. 00049 * 00050 * Library supports the following socket types: 00051 * | Socket name | Socket description | 00052 * | :------------: | :----------------------------: | 00053 * | SOCKET_UDP | UDP socket type | 00054 * | SOCKET_TCP | TCP socket type | 00055 * | SOCKET_ICMP | ICMP raw socket type | 00056 * 00057 * \section socket-raw ICMP RAW socket instruction 00058 * An ICMP raw socket can be created with the function socket_open() and the 00059 * identifier 0xffff. When using ICMP sockets, the minimum packet length is 4 00060 * bytes which is the 4 bytes of ICMP header. The first byte is for type, second 00061 * for code and last two are for the checksum that must always be set to zero. 00062 * The stack will calculate the checksum automatically before transmitting the packet. 00063 * THe header is followed by the payload if there is any. 00064 * NOTE: While it is perfectly legal to send an ICMP packet without payload, 00065 * some packet sniffing softwares might regard such packets as malformed. 00066 * In such a case, a dummy payload can be attached by providing a socket_sendto() 00067 * function with a pointer to your desired data buffer. 00068 * Moreover, the current implementation of the stack allows only certain ICMP types, for example 00069 * ICMP echo, reply, destination unreachable, router advertisement, router solicitation. 00070 * It will drop any other unimplemented types. For example, Private experimentation type (200) will 00071 * be dropped by default. 00072 00073 * | ICMP Type | ICMP Code | Checksum | Payload | Notes | 00074 * | :-------: | :----------: | :-------: | :--------: | :--------------:| 00075 * | 1 | 1 | 2 | n bytes | Length in bytes | 00076 * | 0xXX | 0xXX | 0x00 0x00 | n bytes | Transmit | 00077 * | 0xXX | 0xXX | 0xXX 0xXX | n bytes | Receive | 00078 * 00079 * ICMP echo request with 4 bytes of payload (ping6). 00080 * 00081 * | ICMP Type | ICMP Code | Checksum | Payload | 00082 * | :-------: | :----------: | :-------: | :-----------------: | 00083 * | 0x80 | 0x00 | 0x00 0x00 | 0x00 0x01 0x02 0x03 | 00084 * 00085 * ICMP echo response for the message above. 00086 * 00087 * | ICMP Type | ICMP Code | Checksum | Payload | 00088 * | :-------: | :----------: | :-------: | :-----------------: | 00089 * | 0x81 | 0x00 | 0xXX 0xXX | 0x00 0x01 0x02 0x03 | 00090 * 00091 * \section socket-receive Socket receiving 00092 * When there is data to read from the socket, a receive callback function is called with the event type parameter set to SOCKET_DATA. 00093 * The application must read the received data with socket_read() or socket_recvmsg() during the callback because the stack will release the data 00094 * when returning from the receive callback. 00095 * 00096 * Socket event has event_type and socket_id fields. The receive callback function must be defined when socket is opened using socket_open() API. 00097 * 00098 * All supported socket event types are listed here: 00099 * 00100 * | Event Type | Value | Description | 00101 * | :------------------------: | :---: | :-----------------------------------------------------------------: | 00102 * | SOCKET_EVENT_MASK | 0xF0 | NC Socket event mask. | 00103 * | SOCKET_DATA | 0x00 | Data received, read data length available in d_len field. | 00104 * | SOCKET_CONNECT_DONE | 0x10 | TCP connection ready. | 00105 * | SOCKET_CONNECT_FAIL | 0x20 | TCP connection failed. | 00106 * | SOCKET_INCOMING_CONNECTION | 0x40 | TCP incoming connection on listening socket. | 00107 * | SOCKET_TX_FAIL | 0x50 | Socket data send failed. | 00108 * | SOCKET_CONNECT_CLOSED | 0x60 | TCP connection closed. | 00109 * | SOCKET_CONNECTION_RESET | 0x70 | TCP connection reset. | 00110 * | SOCKET_NO_ROUTE | 0x80 | No route available to destination. | 00111 * | SOCKET_TX_DONE | 0x90 | UDP: link layer TX ready (d_len = length of datagram). | 00112 * | | | TCP: some data acknowledged (d_len = data remaining in send queue) | 00113 * | SOCKET_NO_RAM | 0xA0 | No RAM available. | 00114 * | SOCKET_CONNECTION_PROBLEM | 0xB0 | TCP connection is retrying. | 00115 * 00116 * 00117 * \section socket-tcp How to use TCP sockets: 00118 * 00119 * | API | Socket Type | Description | 00120 * | :---------------------------: | :-----------: | :------------------------------------------------------------: | 00121 * | socket_open() | Server/Client | Open socket to specific or dynamic port number. | 00122 * | socket_shutdown() | Server/Client | Shut down opened TCP connection. | 00123 * | socket_listen() | Server | Set server port to listen state. | 00124 * | socket_accept() | Server | Accept a connection to a listening socket as a new socket. | 00125 * | socket_connect() | Client | Connect client socket to specific destination. | 00126 * | socket_close() | Server/Client | Closes the TCP Socket. | 00127 * | socket_send() | Server/Client | Send data to peer. | 00128 * | socket_recv() | Server/Client | Receive data from peer. | 00129 * 00130 * When the TCP socket is opened it is in closed state. It must be set either to listen or to connect state before it can be used to receive or transmit data. 00131 * 00132 * A socket can be set to listen mode with the socket_listen() function. After the call, the socket can accept an incoming connection from a remote host. 00133 * 00134 * A TCP socket can be connected to a remote host with socket_connect() with correct arguments. After the function call, a (non-blocking) application must wait for the socket event to confirm the successful state change of the socket. 00135 * After the successful state change, data can be sent using socket_send(). 00136 * The connection can be shut down in either direction with socket_shutdown() function - shutting down write signals end-of-data to the peer. 00137 * 00138 * \section socket-udpicmp How to use UDP and RAW socket: 00139 * 00140 * A UDP socket is ready to receive and send data immediately after a successful call of socket_open() and a NET_READY event is received. 00141 * Data can be transmitted with the socket_sendto() function. An ICMP socket works with same function call. 00142 */ 00143 00144 #include "ns_address.h" 00145 00146 /** \name Protocol IDs used with sockets. */ 00147 ///@{ 00148 /** UDP socket type. */ 00149 #define SOCKET_UDP 17 00150 /** Normal TCP socket type. */ 00151 #define SOCKET_TCP 6 00152 /** ICMPv6 raw socket type */ 00153 #define SOCKET_ICMP 32 00154 /** Raw IPv6 socket type (socket_open identifier is IPv6 protocol number) */ 00155 #define SOCKET_RAW 2 00156 /** REMOVED Feature in this release socket open return error Local Sockets for Tun interface functionality to APP-APP trough any BUS */ 00157 #define SOCKET_LOCAL 1 00158 ///@} 00159 00160 /** ICMP socket instruction 00161 * 00162 * ICMP header is comprised of 4 bytes. The first byte is for type, second for code and 00163 * the last two are for checksum that must always be zero. 00164 * The stack will calculate the checksum automatically when sending the packet. 00165 * The payload comes after the header and it can be of any length. It can also be set to 0. 00166 * 00167 * This applies for reading and sending. 00168 * 00169 * ICMP TYPE ICMP Code ICMP Checksum (2 bytes) Payload n bytes 00170 * -------- -------- -------- -------- -------- -------- ....... ------- 00171 * 0xXX 0xXX 0x00 0x00 0xXX 0xXX ...... 0xXX 00172 * 00173 * Example data flow for ping: 00174 * 00175 * ICMP echo request with 4-bytes payload (Ping6). 00176 * 0x80, 0x00, 0x00, 0x00, 0x00, 0x01, 0x02, 0x03 00177 * 00178 * ICMP echo response for the message above. 00179 * 0x81, 0x00, 0xXX, 0xXX, 0x00, 0x01, 0x02, 0x03 00180 */ 00181 00182 /*! 00183 * \struct socket_callback_t 00184 * \brief Socket Callback function structure type. 00185 */ 00186 00187 typedef struct socket_callback_t { 00188 uint8_t event_type; /**< Socket Callback Event check list below */ 00189 int8_t socket_id; /**< Socket id queue which socket cause call back */ 00190 int8_t interface_id; /**< Network Interface ID where Packet came */ 00191 uint16_t d_len; /**< Data length if event type is SOCKET_DATA */ 00192 uint8_t LINK_LQI; /**< LINK LQI info if interface cuold give */ 00193 } socket_callback_t; 00194 00195 /*! 00196 * \struct ns_msghdr_t 00197 * \brief Normal IP socket message structure for socket_recvmsg() and socket_sendmsg(). 00198 */ 00199 00200 typedef struct ns_msghdr { 00201 void *msg_name; /**< Optional address send use for destination and receive it will be source. MUST be ns_address_t */ 00202 uint_fast16_t msg_namelen; /**< Length of optional address use sizeof(ns_address_t) when msg_name is defined */ 00203 ns_iovec_t *msg_iov; /**< Message data vector list */ 00204 uint_fast16_t msg_iovlen; /**< Data vector count in msg_iov */ 00205 void *msg_control; /**< Ancillary data list of ns_cmsghdr_t pointer */ 00206 uint_fast16_t msg_controllen; /**< Ancillary data length */ 00207 int msg_flags; /**< Flags for received messages */ 00208 } ns_msghdr_t; 00209 00210 /*! 00211 * \struct ns_cmsghdr_t 00212 * \brief Control messages. 00213 */ 00214 typedef struct ns_cmsghdr { 00215 uint16_t cmsg_len; /**< Ancillary data control messages length including cmsghdr */ 00216 uint8_t cmsg_level; /**< Originating protocol level should be SOCKET_IPPROTO_IPV6 */ 00217 uint8_t cmsg_type; /**< Protocol Specific types for example SOCKET_IPV6_PKTINFO, */ 00218 } ns_cmsghdr_t; 00219 00220 /** \name Error values 00221 * \anchor ERROR_CODES 00222 */ 00223 ///@{ 00224 /** No data currently available to read, or insufficient queue space for write */ 00225 #define NS_EWOULDBLOCK (-100) 00226 ///@} 00227 00228 /** \name socket_recvfrom() or socket_recvmsg() flags. 00229 * \anchor MSG_HEADER_FLAGS 00230 */ 00231 ///@{ 00232 /** In msg_flags, indicates that given data buffer was smaller than received datagram. Can also be passed as an flag parameter to recvmsg. */ 00233 #define NS_MSG_TRUNC 1 00234 /** Indicates that given ancillary data buffer was smaller than enabled at socket msg->msg_controllen define proper writed data lengths. */ 00235 #define NS_MSG_CTRUNC 2 00236 /** Can be passed as an input flag to socket_recvfrom() to not consume data */ 00237 #define NS_MSG_PEEK 4 00238 /** \deprecated Can be passed as an input flag to get legacy returns of zero - used by socket_read() and socket_sendto() */ 00239 #define NS_MSG_LEGACY0 0x4000 00240 ///@} 00241 /*! 00242 * \struct ns_in6_pktinfo_t 00243 * \brief IPv6 packet info which is used for socket_recvmsg() socket_sendmsg(). 00244 */ 00245 typedef struct ns_in6_pktinfo { 00246 uint8_t ipi6_addr[16]; /**< src/dst IPv6 address */ 00247 int8_t ipi6_ifindex; /**< send/recv interface index */ 00248 } ns_in6_pktinfo_t; 00249 00250 00251 /** \name Alignment macros for control message headers 00252 * \anchor CMSG_ALIGN_FLAGS 00253 */ 00254 ///@{ 00255 /** Base header alignment size */ 00256 #define CMSG_HEADER_ALIGN sizeof(long) 00257 /** Base data alignment size */ 00258 #define CMSG_DATA_ALIGN CMSG_HEADER_ALIGN 00259 /** Returns control message alignment size for data or header based upon alignment base */ 00260 #ifndef NS_ALIGN_SIZE 00261 #define NS_ALIGN_SIZE(length, aligment_base) \ 00262 ((length + (aligment_base -1 )) & ~(aligment_base -1)) 00263 #endif 00264 ///@} 00265 00266 /** 00267 * \brief Parse first control message header from message ancillary data. 00268 * 00269 * \param msgh Pointer for socket message. 00270 * 00271 * \return Pointer to first control message header , Could be NULL when control_message is undefined. 00272 */ 00273 #define NS_CMSG_FIRSTHDR(msgh) \ 00274 ((msgh)->msg_controllen >= sizeof(struct ns_cmsghdr) ? \ 00275 (struct ns_cmsghdr *)(msgh)->msg_control : \ 00276 (struct ns_cmsghdr *)NULL ) 00277 /** 00278 * \brief Parse next control message from message by current control message header. 00279 * 00280 * \param msgh Pointer for socket message. 00281 * \param cmsg Pointer for last parsed control message 00282 * 00283 * \return Pointer to Next control message header , Could be NULL when no more control messages data. 00284 */ 00285 00286 ns_cmsghdr_t *NS_CMSG_NXTHDR(const ns_msghdr_t *msgh, const ns_cmsghdr_t *cmsg); 00287 00288 /** 00289 * \brief Get Data pointer from control message header. 00290 * 00291 * \param cmsg Pointer (ns_cmsghdr_t) for last parsed control message 00292 * 00293 * \return Data pointer. 00294 */ 00295 #define NS_CMSG_DATA(cmsg) \ 00296 ((uint8_t *)(cmsg) + NS_ALIGN_SIZE(sizeof(ns_cmsghdr_t), CMSG_DATA_ALIGN)) 00297 00298 /** 00299 * \brief Returns the total space required for a cmsg header plus the specified data, allowing for all padding 00300 * 00301 * \param length size of the ancillary data 00302 * 00303 * For example, the space required for a SOCKET_IPV6_PKTINFO is NS_CMSG_SPACE(sizeof(ns_in6_pktinfo)) 00304 * 00305 * \return Total size of CMSG header, data and trailing padding. 00306 */ 00307 #define NS_CMSG_SPACE(length) \ 00308 (NS_ALIGN_SIZE(sizeof(ns_cmsghdr_t), CMSG_DATA_ALIGN) + NS_ALIGN_SIZE(length, CMSG_HEADER_ALIGN)) 00309 00310 /** 00311 * \brief Calculate length to store in cmsg_len with taking into account any necessary alignment. 00312 * 00313 * \param length size of the ancillary data 00314 * 00315 * For example, cmsg_len for a SOCKET_IPV6_PKTINFO is NS_CMSG_LEN(sizeof(ns_in6_pktinfo)) 00316 * 00317 * \return Size of CMSG header plus data, without trailing padding. 00318 */ 00319 #define NS_CMSG_LEN(length) \ 00320 (NS_ALIGN_SIZE(sizeof(ns_cmsghdr_t), CMSG_DATA_ALIGN) + length) 00321 00322 /** IPv6 wildcard address IN_ANY */ 00323 extern const uint8_t ns_in6addr_any[16]; 00324 00325 /** 00326 * \brief Create and initialize a socket for communication. 00327 * 00328 * \param protocol Defines the protocol to use. 00329 * \param identifier The socket port. 0 indicates that port is not specified and it will be selected automatically when using the socket. 00330 * \param passed_fptr A function pointer to a function that is called whenever a data frame is received to this socket. 00331 * 00332 * \return 0 or greater on success; Return value is the socket ID. 00333 * \return -1 on failure. 00334 * \return -2 on port reserved. 00335 */ 00336 int8_t socket_open(uint8_t protocol, uint16_t identifier, void (*passed_fptr)(void *)); 00337 00338 /** 00339 * \brief A function to close a socket. 00340 * 00341 * \param socket ID of the socket to be closed. 00342 * 00343 * \return 0 socket closed. 00344 * \return -1 socket not closed. 00345 */ 00346 int8_t socket_close(int8_t socket); 00347 00348 /** 00349 * \brief A function to set a socket to listening mode. 00350 * 00351 * \param socket The socket ID. 00352 * \param backlog The pending connections queue size. 00353 * \return 0 on success. 00354 * \return -1 on failure. 00355 */ 00356 int8_t socket_listen(int8_t socket, uint8_t backlog); 00357 00358 /** 00359 * \brief A function to accept a new connection on an socket. 00360 * 00361 * \param socket_id The socket ID of the listening socket. 00362 * \param addr Either NULL pointer or pointer to structure where the remote address of the connecting host is copied. 00363 * \param passed_fptr A function pointer to a function that is called whenever a data frame is received to the new socket. 00364 * \return 0 or greater on success; return value is the new socket ID. 00365 * \return -1 on failure. 00366 * \return NS_EWOULDBLOCK if no pending connections. 00367 */ 00368 int8_t socket_accept(int8_t socket_id, ns_address_t *addr, void (*passed_fptr)(void *)); 00369 00370 /** 00371 * \brief A function to connect to remote peer (TCP). 00372 * 00373 * \param socket The socket ID. 00374 * \param address The address of a remote peer. 00375 * \deprecated \param randomly_take_src_number Ignored - random local port is always chosen if not yet bound 00376 * 00377 * \return 0 on success. 00378 * \return -1 in case of an invalid socket ID or parameter. 00379 * \return -2 if memory allocation fails. 00380 * \return -3 if the socket is in listening state. 00381 * \return -4 if the socket is already connected. 00382 * \return -5 connect is not supported on this type of socket. 00383 * \return -6 if the TCP session state is wrong. 00384 * \return -7 if the source address selection fails. 00385 */ 00386 int8_t socket_connect(int8_t socket, ns_address_t *address, uint8_t randomly_take_src_number); 00387 00388 /** 00389 * \brief Bind socket to address. 00390 * 00391 * Used by the application to bind a socket to a port and/or an address. Binding of each 00392 * of address and port can only be done once. 00393 * 00394 * If address is ns_in6addr_any, the address binding is not changed. If port is 0, 00395 * the port binding is not changed. 00396 * 00397 * \param socket Socket ID of the socket to bind. 00398 * \param address Address structure containing the port and/or address to bind. 00399 * 00400 * \return 0 on success. 00401 * \return -1 if the given address is NULL. 00402 * \return -2 if the port is already bound to another socket. 00403 * \return -3 if address is not us. 00404 * \return -4 if the socket is already bound. 00405 * \return -5 bind is not supported on this type of socket. 00406 * 00407 */ 00408 int8_t socket_bind(int8_t socket, const ns_address_t *address); 00409 00410 /** 00411 * \brief Bind a local address to a socket based on the destination address and 00412 * the address selection preferences. 00413 * 00414 * Binding happens to the same address that socket_connect() would bind to. 00415 * Reference: RFC5014 IPv6 Socket API for Source Address Selection. 00416 * 00417 * \param socket The socket ID. 00418 * \param dst_address The destination address to which the socket wants to communicate. 00419 * 00420 * \return 0 on success. 00421 * \return -1 if the given address is NULL or socket ID is invalid. 00422 * \return -2 if the memory allocation failed. 00423 * \return -3 if the socket is already bound to address. 00424 * \return -4 if the interface cannot be found. 00425 * \return -5 if the source address selection fails. 00426 * \return -6 bind2addrsel is not supported on this type of socket. 00427 */ 00428 int8_t socket_bind2addrsel(int8_t socket, const ns_address_t *dst_address); 00429 00430 /** 00431 * Options for the socket_shutdown() parameter 'how'. 00432 */ 00433 #define SOCKET_SHUT_RD 0 ///< Disables further receive operations. 00434 #define SOCKET_SHUT_WR 1 ///< Disables further send operations. 00435 #define SOCKET_SHUT_RDWR 2 ///< Disables further send and receive operations. 00436 00437 /** 00438 * \brief A function to shut down a connection. 00439 * 00440 * \param socket The ID of the socket to be shut down. 00441 * \param how How socket is to be shut down, one of SOCKET_SHUT_XX. 00442 * 00443 * \return 0 on success. 00444 * \return -1 if the given socket ID is not found, if the socket type is wrong or TCP layer returns a failure. 00445 * \return -2 if socket is not connected. 00446 */ 00447 int8_t socket_shutdown(int8_t socket, uint8_t how); 00448 00449 /** 00450 * \brief Send data via a connected socket by client. 00451 * 00452 * Note: The socket connection must be ready before using this function. 00453 * The stack uses automatically the address of the remote connected host as the destination address for the packet. 00454 * 00455 * This call is equivalent to socket_sendto() with address set to NULL - see 00456 * that call for more details. 00457 * 00458 * \param socket The socket ID. 00459 * \param buffer A pointer to data. 00460 * \param length Data length. 00461 */ 00462 int16_t socket_send(int8_t socket, const void *buffer, uint16_t length); 00463 00464 /** 00465 * \brief A function to read received data buffer from a socket. 00466 * \deprecated 00467 * 00468 * Used by the application to get data from a socket. See socket_recvfrom() 00469 * for more details. 00470 * 00471 * This is equivalent to socket_recvfrom, except that it passes the 00472 * flag NS_MSG_LEGACY0, which modifies the return behaviour for zero data. 00473 * 00474 * \param socket The socket ID. 00475 * \param src_addr A pointer to a structure where the sender's address is stored. 00476 * May be NULL if not required. 00477 * \param buffer A pointer to an array where the read data is written to. 00478 * \param length The maximum length of the allocated buffer. 00479 * 00480 * \return >0 indicates the length of the data copied to buffer. 00481 * \return 0 if no data was read (includes zero-length datagram, 00482 * end of stream and no data currently available) 00483 * \return -1 invalid input parameters. 00484 */ 00485 int16_t socket_read(int8_t socket, ns_address_t *src_addr, uint8_t *buffer, uint16_t length); 00486 00487 /** 00488 * \brief A function to read received data buffer from a socket, 00489 * 00490 * Equivalent to socket_recvfrom with src_address set to NULL. 00491 * 00492 * \param socket The socket ID. 00493 * \param buffer A pointer to an array where the read data is written to. 00494 * \param length The maximum length of the allocated buffer. 00495 * \param flags Flags for read call 00496 * 00497 * \return as for socket_recvfrom 00498 */ 00499 int16_t socket_recv(int8_t socket, void *buffer, uint16_t length, int flags); 00500 00501 /** 00502 * \brief A function to read received data buffer from a socket 00503 * 00504 * Used by the application to get data from a socket. 00505 * 00506 * This has two modes of operation. 00507 * 00508 * 1) For non-stream sockets, if the receive queue is disabled (set to 0 via 00509 * SOCKET_SO_RCVBUF), which is the non-stream default and original Nanostack 00510 * behaviour, then applications receive exactly one SOCKET_DATA callback per 00511 * datagram, indicating that datagram's length. They must make 1 read call 00512 * in that callback, and they will be given the data. If not read, the 00513 * datagram is discarded on return from the callback. 00514 * 00515 * 2) Otherwise - stream sockets or SOCKET_SO_RCVBUF non-zero - behaviour is 00516 * akin to traditional BSD. SOCKET_DATA callbacks occur when new data arrives, 00517 * and read calls can be made any time. Data will be queued to an extent 00518 * determined by the receive buffer size. The length in the data callback 00519 * is the total amount of data in the receive queue - possibly multiple 00520 * datagrams. 00521 * 00522 * \param socket The socket ID. 00523 * \param buffer A pointer to an array where the read data is written to. 00524 * \param length The maximum length of the allocated buffer. 00525 * \param flags Flags for read call 00526 * \param src_addr A pointer to a structure where the sender's address is stored. 00527 * May be NULL if not required. 00528 * 00529 * The returned length is normally the length of data actually written to the buffer; if 00530 * NS_MSG_TRUNC is set in flags, then for non-stream sockets, the actual datagram length is 00531 * returned instead, which may be larger than the buffer size. 00532 * 00533 * Return values assume flag NS_MSG_LEGACY0 is not set - if it is set, they are 00534 * as per socket_read(). 00535 * 00536 * \return >0 indicates the length of the data copied to buffer (or original datagram size) 00537 * \return 0 if end of stream or zero-length datagram 00538 * \return -1 invalid input parameters. 00539 * \return NS_EWOULDBLOCK if no data is currently available 00540 */ 00541 int16_t socket_recvfrom(int8_t socket, void *buffer, uint16_t length, int flags, ns_address_t *src_addr); 00542 00543 /** 00544 * \brief A function to read received message with ancillary data from a socket. 00545 * 00546 * Used by the application to get data from a socket. See socket_recvfrom for 00547 * details of the two delivery mechanisms. 00548 * 00549 * Ancillary data must request by socket_setsockopt(). 00550 * 00551 * msg->msg_controllen is updated to indicate actual length of ancillary data output 00552 * 00553 * \param socket The socket ID. 00554 * \param msg A pointer to a structure where messages is stored with or without ancillary data 00555 * \param flags A flags for message read. 00556 * 00557 * \return as for socket_recvfrom 00558 */ 00559 int16_t socket_recvmsg(int8_t socket, ns_msghdr_t *msg, int flags); 00560 00561 /** 00562 * \brief A function to send UDP, TCP or raw ICMP data via the socket. 00563 * 00564 * Used by the application to send data. 00565 * 00566 * The return of 0 on success is unconventional, and obtained by passing 00567 * NS_MSG_LEGACY0 to socket_sendmsg internally - to get conventional 00568 * return values, you can use socket_sendmsg() instead. 00569 * 00570 * \param socket The socket ID. 00571 * \param address A pointer to the destination address information. 00572 * \param buffer A pointer to data to be sent. 00573 * \param length Length of the data to be sent. 00574 * 00575 * \return 0 On success (whole packet queued) 00576 * \return NS_EWOULDBLOCK if nothing written due to lack of queue space. 00577 * 00578 * Error returns: 00579 * 00580 * \return -1 Invalid socket ID. 00581 * \return -2 Socket memory allocation fail. 00582 * \return -3 TCP state not established or address scope not defined . 00583 * \return -4 Unknown interface. 00584 * \return -5 Socket not connected 00585 * \return -6 Packet too short (ICMP raw socket error). 00586 */ 00587 int16_t socket_sendto(int8_t socket, const ns_address_t *address, const void *buffer, uint16_t length); 00588 00589 /** 00590 * \brief A function to send UDP, TCP or raw ICMP data via the socket with or without ancillary data or destination address. 00591 * 00592 * Used by the application to send data message header support also vector list socket_send() and socket_sendto() use this functionality internally. 00593 * 00594 * \param socket The socket ID. 00595 * \param msg A pointer to the Message header which include address, payload and ancillary data. 00596 * \param flags A flags for message send (eg NS_MSG_LEGACY0) 00597 * 00598 * Messages destination address is defined by msg->msg_name which must be ns_address_t. If msg->msg_nme is NULL socket select connected address 00599 * 00600 * Messages payload and length is defined msg->msg_iov and msg->msg_iovlen. API support to send multiple data vector. 00601 * 00602 * Supported ancillary data for send defined by msg->msg_control and msg->msg_controllen. 00603 * 00604 * msg->msg_flags is unused, and need not be initialised. 00605 * 00606 * The following main return values assume flag NS_MSG_LEGACY0 is not set - 00607 * if it is set, they are as per socket_sendto(). 00608 * 00609 * \return length if entire amount written (which could be 0) 00610 * \return value >0 and <length if partial amount written (stream only) 00611 * \return NS_EWOULDBLOCK if nothing written due to lack of queue space. 00612 00613 * Error returns: 00614 * 00615 * \return -1 Invalid socket ID. 00616 * \return -2 Socket memory allocation fail. 00617 * \return -3 TCP state not established or address scope not defined . 00618 * \return -4 Unknown interface. 00619 * \return -5 Socket not connected 00620 * \return -6 Packet too short (ICMP raw socket error). 00621 */ 00622 int16_t socket_sendmsg(int8_t socket, const ns_msghdr_t *msg, int flags); 00623 00624 /** 00625 * \brief A function to read local address and port for a bound socket. 00626 * 00627 * This call writes ns_in6addr_any if address is not bound and 0 if the port is not bound. 00628 * 00629 * \param socket The socket ID. 00630 * \param address A pointer to the address structure where the local address information is written to. 00631 * 00632 * \return 0 on success. 00633 * \return -1 if no socket is found. 00634 */ 00635 int8_t socket_getsockname(int8_t socket, ns_address_t *address); 00636 00637 /** 00638 * \brief A function to read remote address and port for a connected socket. 00639 * 00640 * \param socket The socket ID. 00641 * \param address A pointer to the address structure where the remote address information is written to. 00642 * 00643 * \return 0 on success. 00644 * \return -1 if no socket is found. 00645 * \return -2 if no socket is not connected. 00646 */ 00647 int8_t socket_getpeername(int8_t socket, ns_address_t *address); 00648 00649 /* Backwards compatibility */ 00650 static inline int8_t socket_read_session_address(int8_t socket, ns_address_t *address) 00651 { 00652 return socket_getpeername(socket, address); 00653 } 00654 00655 /** \name Flags for SOCKET_IPV6_ADDR_PREFERENCES - opposites 16 bits apart. */ 00656 ///@{ 00657 #define SOCKET_IPV6_PREFER_SRC_TMP 0x00000001 /**< Prefer temporary address (RFC 4941); default. */ 00658 #define SOCKET_IPV6_PREFER_SRC_PUBLIC 0x00010000 /**< Prefer public address (RFC 4941). */ 00659 #define SOCKET_IPV6_PREFER_SRC_6LOWPAN_SHORT 0x00000100 /**< Prefer 6LoWPAN short address. */ 00660 #define SOCKET_IPV6_PREFER_SRC_6LOWPAN_LONG 0x01000000 /**< Prefer 6LoWPAN long address. */ 00661 ///@} 00662 00663 /** \name Options for SOCKET_IPV6_ADDRESS_SELECT. */ 00664 ///@{ 00665 #define SOCKET_SRC_ADDRESS_MODE_PRIMARY 0 /**< Default value always. */ 00666 #define SOCKET_SRC_ADDRESS_MODE_SECONDARY 1 /**< This mode typically selects the secondary address. */ 00667 ///@} 00668 00669 /** \name Protocol levels used for socket_setsockopt. */ 00670 ///@{ 00671 #define SOCKET_SOL_SOCKET 0 /**< Socket level */ 00672 #define SOCKET_IPPROTO_IPV6 41 /**< IPv6. */ 00673 ///@} 00674 00675 /** \name Option names for protocol level SOCKET_SOL_SOCKET. 00676 * \anchor OPTNAMES_SOCKET 00677 */ 00678 ///@{ 00679 /** Specify receive buffer size in payload bytes, as int32_t. 0 means traditional Nanostack behaviour - unread data dropped unless read in data callback */ 00680 #define SOCKET_SO_RCVBUF 1 00681 /** Specify send buffer size in payload bytes, as int32_t. Only currently used for stream sockets. */ 00682 #define SOCKET_SO_SNDBUF 2 00683 /** Specify receive low water mark in payload bytes, as int32_t. Not yet implemented. */ 00684 #define SOCKET_SO_RCVLOWAT 3 00685 /** Specify send low water mark in payload bytes, as int32_t. Queued sends will only be accepted if this many bytes of send queue space are available, else NS_EWOULDBLOCK is returned. */ 00686 #define SOCKET_SO_SNDLOWAT 4 00687 ///@} 00688 00689 /** \name IPv6 socket options 00690 * \anchor OPTNAMES_IPV6 00691 * 00692 * IPv6 socket options summary 00693 * 00694 * | opt_name / cmsg_type | Data type | set/getsockopt | sendmsg | recvmsg | 00695 * | :--------------------------: | :--------------: | :-------------: | :-----: | :-------------------------------: | 00696 * | SOCKET_IPV6_TCLASS | int16_t | Yes | Yes | If enabled with RECVTCLASS | 00697 * | SOCKET_IPV6_UNICAST_HOPS | int16_t | Yes | No | No | 00698 * | SOCKET_IPV6_MULTICAST_HOPS | int16_t | Yes | No | No | 00699 * | SOCKET_IPV6_ADDR_PREFERENCES | int | Yes | No | No | 00700 * | SOCKET_IPV6_USE_MIN_MTU | int8_t | Yes | Yes | No | 00701 * | SOCKET_IPV6_DONTFRAG | int8_t | Yes | Yes | No | 00702 * | SOCKET_IPV6_FLOW_LABEL | int32_t | Yes | No | No | 00703 * | SOCKET_IPV6_HOPLIMIT | int16_t | No | Yes | If enabled with RECVHOPLIMIT | 00704 * | SOCKET_IPV6_PKTINFO | ns_in6_pktinfo_t | No | Yes | If enabled with RECVPKTINFO | 00705 * | SOCKET_IPV6_RECVPKTINFO | bool | Yes | No | No | 00706 * | SOCKET_IPV6_RECVHOPLIMIT | bool | Yes | No | No | 00707 * | SOCKET_IPV6_RECVTCLASS | bool | Yes | No | No | 00708 * | SOCKET_IPV6_MULTICAST_IF | int8_t | Yes | No | No | 00709 * | SOCKET_IPV6_MULTICAST_LOOP | bool | Yes | Yes | No | 00710 * | SOCKET_IPV6_JOIN_GROUP | ns_ipv6_mreq_t | Set only | No | No | 00711 * | SOCKET_IPV6_LEAVE_GROUP | ns_ipv6_mreq_t | Set only | No | No | 00712 * | SOCKET_BROADCAST_PAN | int8_t | Yes | No | No | 00713 * | SOCKET_LINK_LAYER_SECURITY | int8_t | Yes | No | No | 00714 * | SOCKET_INTERFACE_SELECT | int8_t | Yes | No | No | 00715 * 00716 */ 00717 00718 ///@{ 00719 /** Specify traffic class for outgoing packets, as int16_t (RFC 3542 S6.5 says int); valid values 0-255, or -1 for system default. */ 00720 #define SOCKET_IPV6_TCLASS 1 00721 /** Specify hop limit for outgoing unicast packets, as int16_t (RFC 3493 S5.1 says int); valid values 0-255, or -1 for system default. */ 00722 #define SOCKET_IPV6_UNICAST_HOPS 2 00723 /** Specify hop limit for outgoing multicast packets, as int16_t (RFC 3493 S5.2 says int); valid values 0-255, or -1 for system default. */ 00724 #define SOCKET_IPV6_MULTICAST_HOPS 3 00725 /** Specify source address preferences, as uint32_t - see RFC 5014; valid values combinations of SOCKET_IPV6_PREFER_xxx flags. */ 00726 #define SOCKET_IPV6_ADDR_PREFERENCES 4 00727 /** Specify PMTU preference, as int8_t (RFC 3542 S11.1 says int); valid values -1 (PMTUD for unicast, default), 0 (PMTUD always), 1 (PMTUD off). */ 00728 #define SOCKET_IPV6_USE_MIN_MTU 5 00729 /** Specify not to fragment datagrams, as int8_t (RFC 3542 S11.2 says int); valid values 0 (fragment to path MTU, default), 1 (no fragmentation, TX fails if bigger than outgoing interface MTU). */ 00730 #define SOCKET_IPV6_DONTFRAG 6 00731 /** Specify flow label for outgoing packets, as int32_t; valid values 0-0xfffff, or -1 for system default, or -2 to always autogenerate */ 00732 #define SOCKET_IPV6_FLOW_LABEL 7 00733 /** Hop limit control for socket_sendmsg() and indicate for hop limit socket_recmsg(), as int16_t; valid values 0-255, -1 for default for outgoing packet */ 00734 #define SOCKET_IPV6_HOPLIMIT 8 00735 /** Specify control messages packet info for send and receive as ns_in6_pktinfo_t socket_sendmsg() it define source address and outgoing interface. socket_recvmsg() it define messages destination address and arrival interface. Reference at RFC3542*/ 00736 #define SOCKET_IPV6_PKTINFO 9 00737 00738 /** Specify socket_recvmsg() ancillary data request state for Packet info (destination address and interface id), as bool; valid values true write enabled, false write disabled */ 00739 #define SOCKET_IPV6_RECVPKTINFO 10 00740 /** Specify socket_recvmsg() ancillary data request state for receive messages hop-limit, as bool; valid values true write enabled, false information write disabled */ 00741 #define SOCKET_IPV6_RECVHOPLIMIT 11 00742 /** Specify socket_recvmsg() ancillary data request state for receive messages traffic class, as bool; valid values true write enabled, false information write disabled */ 00743 #define SOCKET_IPV6_RECVTCLASS 12 00744 00745 /** Set the interface to use for outgoing multicast packets, as int8_t (RFC3493 S5.2 says unsigned int); 0 means unspecified (use routing table) */ 00746 #define SOCKET_IPV6_MULTICAST_IF 13 00747 /** Specify whether outgoing multicast packets are looped back, as bool (RFC3493 S5.2 says unsigned int) */ 00748 #define SOCKET_IPV6_MULTICAST_LOOP 14 00749 /** Join a multicast group, using ns_ipv6_mreq_t */ 00750 #define SOCKET_IPV6_JOIN_GROUP 15 00751 /** Leave a multicast group, using ns_ipv6_mreq_t */ 00752 #define SOCKET_IPV6_LEAVE_GROUP 16 00753 00754 #define SOCKET_BROADCAST_PAN 0xfc /**< Internal use - transmit with IEEE 802.15.4 broadcast PAN ID */ 00755 #define SOCKET_LINK_LAYER_SECURITY 0xfd /**< Not standard enable or disable socket security at link layer (For 802.15.4). */ 00756 #define SOCKET_INTERFACE_SELECT 0xfe /**< Not standard socket interface ID. */ 00757 #define SOCKET_IPV6_ADDRESS_SELECT 0xff /**< Deprecated - use SOCKET_IPV6_ADDR_PREFERENCES instead. */ 00758 00759 /** Multicast group request used for setsockopt() */ 00760 typedef struct ns_ipv6_mreq { 00761 uint8_t ipv6mr_multiaddr[16]; /**< IPv6 multicast address */ 00762 int8_t ipv6mr_interface; /**< interface id */ 00763 } ns_ipv6_mreq_t; 00764 00765 /** 00766 * \brief Set an option for a socket 00767 * 00768 * Used to specify miscellaneous options for a socket. Supported levels and 00769 * names defined above. 00770 * 00771 * \param socket The socket ID. 00772 * \param level The protocol level. 00773 * \param opt_name The option name (interpretation depends on level). 00774 * See \ref OPTNAMES_SOCKET and \ref OPTNAMES_IPV6. 00775 * \param opt_value A pointer to value for the specified option. 00776 * \param opt_len Size of the data pointed to by the value. 00777 * 00778 * \return 0 on success. 00779 * \return -1 invalid socket ID. 00780 * \return -2 invalid/unsupported option. 00781 * \return -3 invalid option value. 00782 */ 00783 int8_t socket_setsockopt(int8_t socket, uint8_t level, uint8_t opt_name, const void *opt_value, uint16_t opt_len); 00784 00785 ///@} 00786 00787 /** 00788 * \brief Get an option for a socket. 00789 * 00790 * Used to read miscellaneous options for a socket. Supported levels and 00791 * names defined above. If the buffer is smaller than the option, the output 00792 * is silently truncated; otherwise opt_len is modified to indicate the actual 00793 * length. 00794 * 00795 * \param socket The socket ID. 00796 * \param level The protocol level. 00797 * \param opt_name The option name (interpretation depends on level). See \ref OPTNAMES_IPV6. 00798 * \param opt_value A pointer to output buffer. 00799 * \param opt_len A pointer to length of output buffer; updated on exit. 00800 * 00801 * \return 0 on success. 00802 * \return -1 invalid socket ID. 00803 * \return -2 invalid/unsupported option. 00804 */ 00805 int8_t socket_getsockopt(int8_t socket, uint8_t level, uint8_t opt_name, void *opt_value, uint16_t *opt_len); 00806 00807 #ifdef __cplusplus 00808 } 00809 #endif 00810 #endif /*_NS_SOCKET_H*/
Generated on Sun Jul 17 2022 08:25:30 by
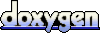