
Rtos API example
Embed:
(wiki syntax)
Show/hide line numbers
GattServer.h
00001 /* mbed Microcontroller Library 00002 * Copyright (c) 2006-2013 ARM Limited 00003 * 00004 * Licensed under the Apache License, Version 2.0 (the "License"); 00005 * you may not use this file except in compliance with the License. 00006 * You may obtain a copy of the License at 00007 * 00008 * http://www.apache.org/licenses/LICENSE-2.0 00009 * 00010 * Unless required by applicable law or agreed to in writing, software 00011 * distributed under the License is distributed on an "AS IS" BASIS, 00012 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00013 * See the License for the specific language governing permissions and 00014 * limitations under the License. 00015 */ 00016 00017 #ifndef MBED_GATT_SERVER_H__ 00018 #define MBED_GATT_SERVER_H__ 00019 00020 #include "Gap.h" 00021 #include "GattService.h" 00022 #include "GattAttribute.h" 00023 #include "GattServerEvents.h" 00024 #include "GattCallbackParamTypes.h" 00025 #include "CallChainOfFunctionPointersWithContext.h" 00026 00027 /** 00028 * @addtogroup ble 00029 * @{ 00030 * @addtogroup gatt 00031 * @{ 00032 * @addtogroup server 00033 * @{ 00034 */ 00035 00036 /** 00037 * Construct and operates a GATT server. 00038 * 00039 * A Gatt server is a collection of GattService; these services contain 00040 * characteristics that a peer connected to the device may read or write. 00041 * These characteristics may also emit updates to subscribed clients when their 00042 * values change. 00043 * 00044 * @p Server Layout 00045 * 00046 * Application code can add a GattService object to the server with the help of 00047 * the function addService(). That function registers all the GattCharacteristic 00048 * enclosed in the service, as well as all the characteristics descriptors (see 00049 * GattAttribute) these characteristics contain. Service registration assigns 00050 * a unique handle to the various attributes being part of the service; this 00051 * handle should be used for subsequent read or write of these components. 00052 * 00053 * There are no primitives defined to remove a single service; however, a call to 00054 * the function reset() removes all services previously registered in the 00055 * GattServer. 00056 * 00057 * @p Characteristic and attributes access 00058 * 00059 * Values of the characteristic and the characteristic descriptor present in the 00060 * GattServer must be accessed through the handle assigned to them when the service 00061 * has been registered; the GattServer class offers several flavors of read() 00062 * and write() functions that retrieve or mutate an attribute value. 00063 * 00064 * Application code can query if a client has subscribed to a given 00065 * characteristic's value update by invoking the function areUpdatesEnabled(). 00066 * 00067 * @p Events 00068 * 00069 * The GattServer allows application code to register several event handlers that 00070 * can be used to monitor client and server activities: 00071 * - onDataSent(): Register an event handler that is called when a 00072 * characteristic value update has been sent to a client. 00073 * - onDataWriten(): Register an event handler that is called when a 00074 * client has written an attribute of the server. 00075 * - onDataRead(): Register an event handler that is called when a 00076 * client has read an attribute of the server. 00077 * - onUpdatesEnabled: Register an event handler that is called when a 00078 * client subscribes to updates of a characteristic. 00079 * - onUpdatesDisabled: Register an event handler that is called when a 00080 * client unsubscribes from updates of a characteristic. 00081 * - onConfimationReceived: Register an event handler that is called 00082 * when a client acknowledges a characteristic value notification. 00083 * 00084 * @note The term characteristic value update is used to represent 00085 * Characteristic Value Notification and Characteristic Value Indication when 00086 * the nature of the server initiated is not relevant. 00087 */ 00088 class GattServer { 00089 public: 00090 /** 00091 * Event handler invoked when the server has sent data to a client. 00092 * 00093 * @see onDataSent(). 00094 */ 00095 typedef FunctionPointerWithContext<unsigned> DataSentCallback_t; 00096 00097 /** 00098 * Callchain of DataSentCallback_t objects. 00099 * 00100 * @see onDataSent(). 00101 */ 00102 typedef CallChainOfFunctionPointersWithContext<unsigned> 00103 DataSentCallbackChain_t; 00104 00105 /** 00106 * Event handler invoked when the client has written an attribute of the 00107 * server. 00108 * 00109 * @see onDataWritten(). 00110 */ 00111 typedef FunctionPointerWithContext<const GattWriteCallbackParams*> 00112 DataWrittenCallback_t; 00113 00114 /** 00115 * Callchain of DataWrittenCallback_t objects. 00116 * 00117 * @see onDataWritten(). 00118 */ 00119 typedef CallChainOfFunctionPointersWithContext<const GattWriteCallbackParams*> 00120 DataWrittenCallbackChain_t; 00121 00122 /** 00123 * Event handler invoked when the client has read an attribute of the server. 00124 * 00125 * @see onDataRead(). 00126 */ 00127 typedef FunctionPointerWithContext<const GattReadCallbackParams*> 00128 DataReadCallback_t; 00129 00130 /** 00131 * Callchain of DataReadCallback_t. 00132 * 00133 * @see onDataRead(). 00134 */ 00135 typedef CallChainOfFunctionPointersWithContext<const GattReadCallbackParams*> 00136 DataReadCallbackChain_t; 00137 00138 /** 00139 * Event handler invoked when the GattServer is reset. 00140 * 00141 * @see onShutdown() reset() 00142 */ 00143 typedef FunctionPointerWithContext<const GattServer *> 00144 GattServerShutdownCallback_t; 00145 00146 /** 00147 * Callchain of GattServerShutdownCallback_t. 00148 * 00149 * @see onShutdown() reset() 00150 */ 00151 typedef CallChainOfFunctionPointersWithContext<const GattServer*> 00152 GattServerShutdownCallbackChain_t; 00153 00154 /** 00155 * Event handler that handles subscription to characteristic updates, 00156 * unsubscription from characteristic updates and notification confirmation. 00157 * 00158 * @see onUpdatesEnabled() onUpdateDisabled() onConfirmationReceived() 00159 */ 00160 typedef FunctionPointerWithContext<GattAttribute::Handle_t> EventCallback_t; 00161 00162 protected: 00163 /** 00164 * Construct a GattServer instance. 00165 */ 00166 GattServer() : 00167 serviceCount(0), 00168 characteristicCount(0), 00169 dataSentCallChain(), 00170 dataWrittenCallChain(), 00171 dataReadCallChain(), 00172 updatesEnabledCallback(NULL), 00173 updatesDisabledCallback(NULL), 00174 confirmationReceivedCallback(NULL) { 00175 } 00176 00177 /* 00178 * The following functions are meant to be overridden in the platform 00179 * specific subclass. 00180 */ 00181 public: 00182 00183 /** 00184 * Add a service declaration to the local attribute server table. 00185 * 00186 * This functions inserts a service declaration in the attribute table 00187 * followed by the characteristic declarations (including characteristic 00188 * descriptors) present in @p service. 00189 * 00190 * The process assigns a unique attribute handle to all the elements added 00191 * into the attribute table. This handle is an ID that must be used for 00192 * subsequent interractions with the elements. 00193 * 00194 * @note There is no mirror function that removes a single service. 00195 * Application code can remove all the registered services by calling 00196 * reset(). 00197 * 00198 * @important Service, characteristics and descriptors objects registered 00199 * within the GattServer must remain reachable until reset() is called. 00200 * 00201 * @param[in] service The service to be added; attribute handle of services, 00202 * characteristic and characteristic descriptors are updated by the 00203 * process. 00204 * 00205 * @return BLE_ERROR_NONE if the service was successfully added. 00206 */ 00207 virtual ble_error_t addService(GattService &service) 00208 { 00209 /* Avoid compiler warnings about unused variables. */ 00210 (void)service; 00211 00212 /* Requesting action from porters: override this API if this capability 00213 is supported. */ 00214 return BLE_ERROR_NOT_IMPLEMENTED; 00215 } 00216 00217 /** 00218 * Read the value of an attribute present in the local GATT server. 00219 * 00220 * @param[in] attributeHandle Handle of the attribute to read. 00221 * @param[out] buffer A buffer to hold the value being read. 00222 * @param[in,out] lengthP Length of the buffer being supplied. If the 00223 * attribute value is longer than the size of the supplied buffer, this 00224 * variable holds upon return the total attribute value length (excluding 00225 * offset). The application may use this information to allocate a suitable 00226 * buffer size. 00227 * 00228 * @return BLE_ERROR_NONE if a value was read successfully into the buffer. 00229 * 00230 * @important read(Gap::Handle_t, GattAttribute::Handle_t, uint8_t *, uint16_t *) 00231 * must be used to read Client Characteristic Configuration Descriptor (CCCD) 00232 * because the value of this type of attribute depends on the connection. 00233 */ 00234 virtual ble_error_t read( 00235 GattAttribute::Handle_t attributeHandle, 00236 uint8_t buffer[], 00237 uint16_t *lengthP 00238 ) { 00239 /* Avoid compiler warnings about unused variables. */ 00240 (void)attributeHandle; 00241 (void)buffer; 00242 (void)lengthP; 00243 00244 /* Requesting action from porters: override this API if this capability 00245 is supported. */ 00246 return BLE_ERROR_NOT_IMPLEMENTED; 00247 } 00248 00249 /** 00250 * Read the value of an attribute present in the local GATT server. 00251 * 00252 * The connection handle allows application code to read the value of a 00253 * Client Characteristic Configuration Descriptor for a given connection. 00254 * 00255 * @param[in] connectionHandle Connection handle. 00256 * @param[in] attributeHandle Attribute handle for the value attribute of 00257 * the characteristic. 00258 * @param[out] buffer A buffer to hold the value being read. 00259 * @param[in,out] lengthP Length of the buffer being supplied. If the 00260 * attribute value is longer than the size of the supplied buffer, this 00261 * variable holds upon return the total attribute value length (excluding 00262 * offset). The application may use this information to allocate a suitable 00263 * buffer size. 00264 * 00265 * @return BLE_ERROR_NONE if a value was read successfully into the buffer. 00266 */ 00267 virtual ble_error_t read( 00268 Gap::Handle_t connectionHandle, 00269 GattAttribute::Handle_t attributeHandle, 00270 uint8_t *buffer, 00271 uint16_t *lengthP 00272 ) { 00273 /* Avoid compiler warnings about unused variables. */ 00274 (void)connectionHandle; 00275 (void)attributeHandle; 00276 (void)buffer; 00277 (void)lengthP; 00278 00279 /* Requesting action from porters: override this API if this capability 00280 is supported. */ 00281 return BLE_ERROR_NOT_IMPLEMENTED; 00282 } 00283 00284 /** 00285 * Update the value of an attribute present in the local GATT server. 00286 * 00287 * @param[in] attributeHandle Handle of the attribute to write. 00288 * @param[in] value A pointer to a buffer holding the new value. 00289 * @param[in] size Size in bytes of the new value (in bytes). 00290 * @param[in] localOnly If this flag is false and the attribute handle 00291 * written is a characteristic value, then the server sends an update 00292 * containing the new value to all clients that have subscribed to the 00293 * characteristic's notifications or indications. Otherwise, the update does 00294 * not generate a single server initiated event. 00295 * 00296 * @return BLE_ERROR_NONE if the attribute value has been successfully 00297 * updated. 00298 */ 00299 virtual ble_error_t write( 00300 GattAttribute::Handle_t attributeHandle, 00301 const uint8_t *value, 00302 uint16_t size, 00303 bool localOnly = false 00304 ) { 00305 /* Avoid compiler warnings about unused variables. */ 00306 (void)attributeHandle; 00307 (void)value; 00308 (void)size; 00309 (void)localOnly; 00310 00311 /* Requesting action from porters: override this API if this capability 00312 is supported. */ 00313 return BLE_ERROR_NOT_IMPLEMENTED; 00314 } 00315 00316 /** 00317 * Update the value of an attribute present in the local GATT server. 00318 * 00319 * The connection handle parameter allows application code to direct 00320 * notification or indication resulting from the update to a specific client. 00321 * 00322 * @param[in] connectionHandle Connection handle. 00323 * @param[in] attributeHandle Handle for the value attribute of the 00324 * characteristic. 00325 * @param[in] value A pointer to a buffer holding the new value. 00326 * @param[in] size Size of the new value (in bytes). 00327 * @param[in] localOnly If this flag is false and the attribute handle 00328 * written is a characteristic value, then the server sends an update 00329 * containing the new value to the client identified by the parameter 00330 * @p connectionHandle if it is subscribed to the characteristic's 00331 * notifications or indications. Otherwise, the update does not generate a 00332 * single server initiated event. 00333 * 00334 * @return BLE_ERROR_NONE if the attribute value has been successfully 00335 * updated. 00336 */ 00337 virtual ble_error_t write( 00338 Gap::Handle_t connectionHandle, 00339 GattAttribute::Handle_t attributeHandle, 00340 const uint8_t *value, 00341 uint16_t size, 00342 bool localOnly = false 00343 ) { 00344 /* Avoid compiler warnings about unused variables. */ 00345 (void)connectionHandle; 00346 (void)attributeHandle; 00347 (void)value; 00348 (void)size; 00349 (void)localOnly; 00350 00351 /* Requesting action from porters: override this API if this capability 00352 is supported. */ 00353 return BLE_ERROR_NOT_IMPLEMENTED; 00354 } 00355 00356 /** 00357 * Determine if one of the connected clients has subscribed to notifications 00358 * or indications of the characteristic in input. 00359 * 00360 * @param[in] characteristic The characteristic. 00361 * @param[out] enabledP Upon return, *enabledP is true if updates are 00362 * enabled for a connected client; otherwise, *enabledP is false. 00363 * 00364 * @return BLE_ERROR_NONE if the connection and handle are found. False 00365 * otherwise. 00366 */ 00367 virtual ble_error_t areUpdatesEnabled( 00368 const GattCharacteristic &characteristic, 00369 bool *enabledP 00370 ) { 00371 /* Avoid compiler warnings about unused variables. */ 00372 (void)characteristic; 00373 (void)enabledP; 00374 00375 /* Requesting action from porters: override this API if this capability 00376 is supported. */ 00377 return BLE_ERROR_NOT_IMPLEMENTED; 00378 } 00379 00380 /** 00381 * Determine if an identified client has subscribed to notifications or 00382 * indications of a given characteristic. 00383 * 00384 * @param[in] connectionHandle The connection handle. 00385 * @param[in] characteristic The characteristic. 00386 * @param[out] enabledP Upon return, *enabledP is true if the client 00387 * identified by @p connectionHandle has subscribed to notifications or 00388 * indications of @p characteristic; otherwise, *enabledP is false. 00389 * 00390 * @return BLE_ERROR_NONE if the connection and handle are found. False 00391 * otherwise. 00392 */ 00393 virtual ble_error_t areUpdatesEnabled( 00394 Gap::Handle_t connectionHandle, 00395 const GattCharacteristic &characteristic, 00396 bool *enabledP 00397 ) { 00398 /* Avoid compiler warnings about unused variables. */ 00399 (void)connectionHandle; 00400 (void)characteristic; 00401 (void)enabledP; 00402 00403 /* Requesting action from porters: override this API if this capability 00404 is supported. */ 00405 return BLE_ERROR_NOT_IMPLEMENTED; 00406 } 00407 00408 /** 00409 * Indicate if the underlying stack emit events when an attribute is read by 00410 * a client. 00411 * 00412 * @important This function should be overridden to return true if 00413 * applicable. 00414 * 00415 * @return true if onDataRead is supported; false otherwise. 00416 */ 00417 virtual bool isOnDataReadAvailable() const 00418 { 00419 /* Requesting action from porters: override this API if this capability 00420 is supported. */ 00421 return false; 00422 } 00423 00424 /* 00425 * APIs with nonvirtual implementations. 00426 */ 00427 public: 00428 /** 00429 * Add an event handler that monitors emission of characteristic value 00430 * updates. 00431 * 00432 * @param[in] callback Event handler being registered. 00433 * 00434 * @note It is possible to chain together multiple onDataSent callbacks 00435 * (potentially from different modules of an application). 00436 */ 00437 void onDataSent(const DataSentCallback_t &callback) 00438 { 00439 dataSentCallChain.add(callback); 00440 } 00441 00442 /** 00443 * Add an event handler that monitors emission of characteristic value 00444 * updates. 00445 * 00446 * @param[in] objPtr Pointer to the instance that is used to invoke the 00447 * event handler. 00448 * @param[in] memberPtr Event handler being registered. It is a member 00449 * function. 00450 */ 00451 template <typename T> 00452 void onDataSent(T *objPtr, void (T::*memberPtr)(unsigned count)) 00453 { 00454 dataSentCallChain.add(objPtr, memberPtr); 00455 } 00456 00457 /** 00458 * Access the callchain of data sent event handlers. 00459 * 00460 * @return A reference to the DATA_SENT event callback chain. 00461 */ 00462 DataSentCallbackChain_t &onDataSent() 00463 { 00464 return dataSentCallChain; 00465 } 00466 00467 /** 00468 * Set an event handler that is called after 00469 * a connected peer has written an attribute. 00470 * 00471 * @param[in] callback The event handler being registered. 00472 * 00473 * @important It is possible to set multiple event handlers. Registered 00474 * handlers may be removed with onDataWritten().detach(callback). 00475 */ 00476 void onDataWritten(const DataWrittenCallback_t &callback) 00477 { 00478 dataWrittenCallChain.add(callback); 00479 } 00480 00481 /** 00482 * Set an event handler that is called after 00483 * a connected peer has written an attribute. 00484 * 00485 * @param[in] objPtr Pointer to the instance that is used to invoke the 00486 * event handler (@p memberPtr). 00487 * @param[in] memberPtr Event handler being registered. It is a member 00488 * function. 00489 */ 00490 template <typename T> 00491 void onDataWritten( 00492 T *objPtr, 00493 void (T::*memberPtr)(const GattWriteCallbackParams *context) 00494 ) { 00495 dataWrittenCallChain.add(objPtr, memberPtr); 00496 } 00497 00498 /** 00499 * Access the callchain of data written event handlers. 00500 * 00501 * @return A reference to the data written event callbacks chain. 00502 * 00503 * @note It is possible to register callbacks using 00504 * onDataWritten().add(callback). 00505 * 00506 * @note It is possible to unregister callbacks using 00507 * onDataWritten().detach(callback). 00508 */ 00509 DataWrittenCallbackChain_t &onDataWritten() 00510 { 00511 return dataWrittenCallChain; 00512 } 00513 00514 /** 00515 * Set an event handler that monitors attribute reads from connected clients. 00516 * 00517 * @param[in] callback Event handler being registered. 00518 * 00519 * @return BLE_ERROR_NOT_IMPLEMENTED if this functionality isn't available; 00520 * else BLE_ERROR_NONE. 00521 * 00522 * @note This functionality may not be available on all underlying stacks. 00523 * Application code may work around that limitation by monitoring read 00524 * requests instead of read events. 00525 * 00526 * @see GattCharacteristic::setReadAuthorizationCallback() 00527 * @see isOnDataReadAvailable(). 00528 * 00529 * @important It is possible to set multiple event handlers. Registered 00530 * handlers may be removed with onDataRead().detach(callback). 00531 */ 00532 ble_error_t onDataRead(const DataReadCallback_t &callback) 00533 { 00534 if (!isOnDataReadAvailable()) { 00535 return BLE_ERROR_NOT_IMPLEMENTED; 00536 } 00537 00538 dataReadCallChain.add(callback); 00539 return BLE_ERROR_NONE; 00540 } 00541 00542 /** 00543 * Set an event handler that monitors attribute reads from connected clients. 00544 * 00545 * @param[in] objPtr Pointer to the instance that is used to invoke the 00546 * event handler (@p memberPtr). 00547 * @param[in] memberPtr Event handler being registered. It is a member 00548 * function. 00549 */ 00550 template <typename T> 00551 ble_error_t onDataRead( 00552 T *objPtr, 00553 void (T::*memberPtr)(const GattReadCallbackParams *context) 00554 ) { 00555 if (!isOnDataReadAvailable()) { 00556 return BLE_ERROR_NOT_IMPLEMENTED; 00557 } 00558 00559 dataReadCallChain.add(objPtr, memberPtr); 00560 return BLE_ERROR_NONE; 00561 } 00562 00563 /** 00564 * Access the callchain of data read event handlers. 00565 * 00566 * @return A reference to the data read event callbacks chain. 00567 * 00568 * @note It is possible to register callbacks using 00569 * onDataRead().add(callback). 00570 * 00571 * @note It is possible to unregister callbacks using 00572 * onDataRead().detach(callback). 00573 */ 00574 DataReadCallbackChain_t &onDataRead() 00575 { 00576 return dataReadCallChain; 00577 } 00578 00579 /** 00580 * Set an event handler that monitors shutdown or reset of the GattServer. 00581 * 00582 * The event handler is invoked when the GattServer instance is about 00583 * to be shut down. This can result in a call to reset() or BLE::reset(). 00584 * 00585 * @param[in] callback Event handler being registered. 00586 * 00587 * @note It is possible to set up multiple shutdown event handlers. 00588 * 00589 * @note It is possible to unregister a callback using 00590 * onShutdown().detach(callback) 00591 */ 00592 void onShutdown(const GattServerShutdownCallback_t &callback) 00593 { 00594 shutdownCallChain.add(callback); 00595 } 00596 00597 /** 00598 * Set an event handler that monitors shutdown or reset of the GattServer. 00599 * 00600 * The event handler is invoked when the GattServer instance is about 00601 * to be shut down. This can result of a call to reset() or BLE::reset(). 00602 * 00603 * @param[in] objPtr Pointer to the instance that is used to invoke the 00604 * event handler (@p memberPtr). 00605 * @param[in] memberPtr Event handler being registered. It is a member 00606 * function. 00607 */ 00608 template <typename T> 00609 void onShutdown(T *objPtr, void (T::*memberPtr)(const GattServer *)) 00610 { 00611 shutdownCallChain.add(objPtr, memberPtr); 00612 } 00613 00614 /** 00615 * Access the callchain of shutdown event handlers. 00616 * 00617 * @return A reference to the shutdown event callbacks chain. 00618 * 00619 * @note It is possible to register callbacks using 00620 * onShutdown().add(callback). 00621 * 00622 * @note It is possible to unregister callbacks using 00623 * onShutdown().detach(callback). 00624 */ 00625 GattServerShutdownCallbackChain_t & onShutdown() 00626 { 00627 return shutdownCallChain; 00628 } 00629 00630 /** 00631 * Set up an event handler that monitors subscription to characteristic 00632 * updates. 00633 * 00634 * @param[in] callback Event handler being registered. 00635 */ 00636 void onUpdatesEnabled(EventCallback_t callback) 00637 { 00638 updatesEnabledCallback = callback; 00639 } 00640 00641 /** 00642 * Set up an event handler that monitors unsubscription from characteristic 00643 * updates. 00644 * 00645 * @param[in] callback Event handler being registered. 00646 */ 00647 void onUpdatesDisabled(EventCallback_t callback) 00648 { 00649 updatesDisabledCallback = callback; 00650 } 00651 00652 /** 00653 * Set up an event handler that monitors notification acknowledgment. 00654 * 00655 * The event handler is called when a client sends a confirmation that it has 00656 * correctly received a notification from the server. 00657 * 00658 * @param[in] callback Event handler being registered. 00659 */ 00660 void onConfirmationReceived(EventCallback_t callback) 00661 { 00662 confirmationReceivedCallback = callback; 00663 } 00664 00665 /* Entry points for the underlying stack to report events back to the user. */ 00666 protected: 00667 /** 00668 * Helper function that notifies all registered handlers of an occurrence 00669 * of a data written event. 00670 * 00671 * @important Vendor implementation must invoke this function after one of 00672 * the GattServer attributes has been written. 00673 * 00674 * @param[in] params The data written parameters passed to the registered 00675 * handlers. 00676 */ 00677 void handleDataWrittenEvent(const GattWriteCallbackParams *params) 00678 { 00679 dataWrittenCallChain.call(params); 00680 } 00681 00682 /** 00683 * Helper function that notifies all registered handlers of an occurrence 00684 * of a data read event. 00685 * 00686 * @important Vendor implementation must invoke this function after one of 00687 * the GattServer attributes has been read. 00688 * 00689 * @param[in] params The data read parameters passed to the registered 00690 * handlers. 00691 */ 00692 void handleDataReadEvent(const GattReadCallbackParams *params) 00693 { 00694 dataReadCallChain.call(params); 00695 } 00696 00697 /** 00698 * Helper function that notifies the registered handler of an occurrence 00699 * of updates enabled, updates disabled or confirmation received events. 00700 * 00701 * @important Vendor implementation must invoke this function when a client 00702 * subscribes to characteristic updates, unsubscribes from characteristic 00703 * updates or a notification confirmation has been received. 00704 * 00705 * @param[in] type The type of event that occurred. 00706 * @param[in] attributeHandle The handle of the attribute concerned by the 00707 * event. 00708 */ 00709 void handleEvent( 00710 GattServerEvents::gattEvent_e type, 00711 GattAttribute::Handle_t attributeHandle 00712 ) { 00713 switch (type) { 00714 case GattServerEvents::GATT_EVENT_UPDATES_ENABLED: 00715 if (updatesEnabledCallback) { 00716 updatesEnabledCallback(attributeHandle); 00717 } 00718 break; 00719 case GattServerEvents::GATT_EVENT_UPDATES_DISABLED: 00720 if (updatesDisabledCallback) { 00721 updatesDisabledCallback(attributeHandle); 00722 } 00723 break; 00724 case GattServerEvents::GATT_EVENT_CONFIRMATION_RECEIVED: 00725 if (confirmationReceivedCallback) { 00726 confirmationReceivedCallback(attributeHandle); 00727 } 00728 break; 00729 default: 00730 break; 00731 } 00732 } 00733 00734 /** 00735 * Helper function that notifies all registered handlers of an occurrence 00736 * of a data sent event. 00737 * 00738 * @important Vendor implementation must invoke this function after the 00739 * emission of a notification or an indication. 00740 * 00741 * @param[in] count Number of packets sent. 00742 */ 00743 void handleDataSentEvent(unsigned count) 00744 { 00745 dataSentCallChain.call(count); 00746 } 00747 00748 public: 00749 /** 00750 * Shut down the GattServer instance. 00751 * 00752 * This function notifies all event handlers listening for shutdown events 00753 * that the GattServer is about to be shut down; then it clears all 00754 * GattServer state. 00755 * 00756 * @note This function is meant to be overridden in the platform-specific 00757 * subclass. Overides must call the parent function before any cleanup. 00758 * 00759 * @return BLE_ERROR_NONE on success. 00760 */ 00761 virtual ble_error_t reset(void) 00762 { 00763 /* Notify that the instance is about to shutdown */ 00764 shutdownCallChain.call(this); 00765 shutdownCallChain.clear(); 00766 00767 serviceCount = 0; 00768 characteristicCount = 0; 00769 00770 dataSentCallChain.clear(); 00771 dataWrittenCallChain.clear(); 00772 dataReadCallChain.clear(); 00773 updatesEnabledCallback = NULL; 00774 updatesDisabledCallback = NULL; 00775 confirmationReceivedCallback = NULL; 00776 00777 return BLE_ERROR_NONE; 00778 } 00779 00780 protected: 00781 /** 00782 * The total number of services added to the ATT table. 00783 */ 00784 uint8_t serviceCount; 00785 00786 /** 00787 * The total number of characteristics added to the ATT table. 00788 */ 00789 uint8_t characteristicCount; 00790 00791 private: 00792 /** 00793 * Callchain containing all registered callback handlers for data sent 00794 * events. 00795 */ 00796 DataSentCallbackChain_t dataSentCallChain; 00797 00798 /** 00799 * Callchain containing all registered callback handlers for data written 00800 * events. 00801 */ 00802 DataWrittenCallbackChain_t dataWrittenCallChain; 00803 00804 /** 00805 * Callchain containing all registered callback handlers for data read 00806 * events. 00807 */ 00808 DataReadCallbackChain_t dataReadCallChain; 00809 00810 /** 00811 * Callchain containing all registered callback handlers for shutdown 00812 * events. 00813 */ 00814 GattServerShutdownCallbackChain_t shutdownCallChain; 00815 00816 /** 00817 * The registered callback handler for updates enabled events. 00818 */ 00819 EventCallback_t updatesEnabledCallback; 00820 00821 /** 00822 * The registered callback handler for updates disabled events. 00823 */ 00824 EventCallback_t updatesDisabledCallback; 00825 00826 /** 00827 * The registered callback handler for confirmation received events. 00828 */ 00829 EventCallback_t confirmationReceivedCallback; 00830 00831 private: 00832 /* Disallow copy and assignment. */ 00833 GattServer(const GattServer &); 00834 GattServer& operator=(const GattServer &); 00835 }; 00836 00837 /** 00838 * @} 00839 * @} 00840 * @} 00841 */ 00842 00843 #endif /* ifndef MBED_GATT_SERVER_H__ */
Generated on Sun Jul 17 2022 08:25:23 by
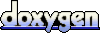