
Rtos API example
Embed:
(wiki syntax)
Show/hide line numbers
GattCallbackParamTypes.h
00001 /* mbed Microcontroller Library 00002 * Copyright (c) 2006-2013 ARM Limited 00003 * 00004 * Licensed under the Apache License, Version 2.0 (the "License"); 00005 * you may not use this file except in compliance with the License. 00006 * You may obtain a copy of the License at 00007 * 00008 * http://www.apache.org/licenses/LICENSE-2.0 00009 * 00010 * Unless required by applicable law or agreed to in writing, software 00011 * distributed under the License is distributed on an "AS IS" BASIS, 00012 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00013 * See the License for the specific language governing permissions and 00014 * limitations under the License. 00015 */ 00016 00017 #ifndef MBED_BLE_GATT_CALLBACK_PARAM_TYPES_H__ 00018 #define MBED_BLE_GATT_CALLBACK_PARAM_TYPES_H__ 00019 00020 /** 00021 * @addtogroup ble 00022 * @{ 00023 * @addtogroup gatt 00024 * @{ 00025 */ 00026 00027 /** 00028 * GATT Write event definition. 00029 * 00030 * Instances of this type are created and passed to user registered callbacks 00031 * whether the GattServer has received a write request or a GattClient has 00032 * received a write response. 00033 * 00034 * @important The GattServer only populates the fields offset, len and data 00035 * when it has received a write request. Callbacks attached to the GattClient 00036 * do not use those fields. 00037 * 00038 * @important The GattClient only populates the fields status and error_code 00039 * when it has received a write response. Callbacks attached to the GattServer 00040 * do not use those fields. 00041 */ 00042 struct GattWriteCallbackParams { 00043 /** 00044 * Enumeration of allowed write operations. 00045 */ 00046 enum WriteOp_t { 00047 /** 00048 * Invalid operation. 00049 */ 00050 OP_INVALID = 0x00, 00051 00052 /** 00053 * Write request. 00054 */ 00055 OP_WRITE_REQ = 0x01, 00056 00057 /** 00058 * Write command. 00059 */ 00060 OP_WRITE_CMD = 0x02, 00061 00062 /** 00063 * Signed write command. 00064 */ 00065 OP_SIGN_WRITE_CMD = 0x03, 00066 00067 /** 00068 * Prepare write request. 00069 */ 00070 OP_PREP_WRITE_REQ = 0x04, 00071 00072 /** 00073 * Execute write request: cancel all prepared writes. 00074 */ 00075 OP_EXEC_WRITE_REQ_CANCEL = 0x05, 00076 00077 /** 00078 * Execute write request: immediately execute all prepared writes. 00079 */ 00080 OP_EXEC_WRITE_REQ_NOW = 0x06, 00081 }; 00082 00083 /** 00084 * Handle of the connection that triggered the event. 00085 */ 00086 Gap::Handle_t connHandle; 00087 00088 /** 00089 * Handle of the attribute to which the write operation applies. 00090 */ 00091 GattAttribute::Handle_t handle; 00092 00093 /** 00094 * Type of the write operation. 00095 */ 00096 WriteOp_t writeOp; 00097 00098 union { 00099 /** 00100 * Offset within the attribute value to be written. 00101 * 00102 * @important Reserved for GattServer registered callbacks. 00103 */ 00104 uint16_t offset; 00105 00106 /** 00107 * Status of the GattClient Write operation. 00108 * 00109 * @important Reserved for GattClient registered callbacks. 00110 */ 00111 ble_error_t status; 00112 }; 00113 00114 union { 00115 /** 00116 * Length (in bytes) of the data to write. 00117 * 00118 * @important Reserved for GattServer registered callbacks. 00119 */ 00120 uint16_t len; 00121 00122 /** 00123 * Error code of the GattClient Write operation. 00124 * 00125 * @important Reserved for GattClient registered callbacks. 00126 */ 00127 uint8_t error_code; 00128 }; 00129 00130 /** 00131 * Pointer to the data to write. 00132 * 00133 * @important Data may not persist beyond the callback scope. 00134 * 00135 * @important Reserved for GattServer registered callbacks. 00136 */ 00137 const uint8_t *data; 00138 }; 00139 00140 /** 00141 * GATT Read event definition. 00142 * 00143 * Instances of this type are created and passed to user registered callbacks 00144 * whether the GattServer has received a read request or a GattClient has 00145 * received a read response. 00146 * 00147 * @important The GattClient only populates the fields status and error_code 00148 * when it has received a read response. Callbacks attached to the GattServer 00149 * do not use those fields. 00150 */ 00151 struct GattReadCallbackParams { 00152 /** 00153 * Handle of the connection that triggered the event. 00154 */ 00155 Gap::Handle_t connHandle; 00156 00157 /** 00158 * Attribute Handle to which the read operation applies. 00159 */ 00160 GattAttribute::Handle_t handle; 00161 00162 /** 00163 * Offset within the attribute value read. 00164 */ 00165 uint16_t offset; 00166 00167 union { 00168 /** 00169 * Length in bytes of the data read. 00170 */ 00171 uint16_t len; 00172 00173 /** 00174 * Error code of the GattClient read operation. 00175 * 00176 * @important Reserved for GattClient registered callbacks. 00177 * 00178 * @important set if status is not equal to BLE_ERROR_NONE; otherwise, 00179 * this field is interpreted as len. 00180 */ 00181 uint8_t error_code; 00182 }; 00183 00184 /** 00185 * Pointer to the data read. 00186 * 00187 * @important Data may not persist beyond the callback scope. 00188 */ 00189 const uint8_t *data; 00190 00191 /** 00192 * Status of the GattClient Read operation. 00193 * 00194 * @important Reserved for GattClient registered callbacks. 00195 */ 00196 ble_error_t status; 00197 }; 00198 00199 /** 00200 * @addtogroup server 00201 * @{ 00202 */ 00203 00204 /** 00205 * Enumeration of allowed values returned by read or write authorization process. 00206 */ 00207 enum GattAuthCallbackReply_t { 00208 /** 00209 * Success. 00210 */ 00211 AUTH_CALLBACK_REPLY_SUCCESS = 0x00, 00212 00213 /** 00214 * ATT Error: Invalid attribute handle. 00215 */ 00216 AUTH_CALLBACK_REPLY_ATTERR_INVALID_HANDLE = 0x0101, 00217 00218 /** 00219 * ATT Error: Read not permitted. 00220 */ 00221 AUTH_CALLBACK_REPLY_ATTERR_READ_NOT_PERMITTED = 0x0102, 00222 00223 /** 00224 * ATT Error: Write not permitted. 00225 */ 00226 AUTH_CALLBACK_REPLY_ATTERR_WRITE_NOT_PERMITTED = 0x0103, 00227 00228 /** 00229 * ATT Error: Authenticated link required. 00230 */ 00231 AUTH_CALLBACK_REPLY_ATTERR_INSUF_AUTHENTICATION = 0x0105, 00232 00233 /** 00234 * ATT Error: The specified offset was past the end of the attribute. 00235 */ 00236 AUTH_CALLBACK_REPLY_ATTERR_INVALID_OFFSET = 0x0107, 00237 00238 /** 00239 * ATT Error: Used in ATT as "insufficient authorization". 00240 */ 00241 AUTH_CALLBACK_REPLY_ATTERR_INSUF_AUTHORIZATION = 0x0108, 00242 00243 /** 00244 * ATT Error: Used in ATT as "prepare queue full". 00245 */ 00246 AUTH_CALLBACK_REPLY_ATTERR_PREPARE_QUEUE_FULL = 0x0109, 00247 00248 /** 00249 * ATT Error: Used in ATT as "attribute not found". 00250 */ 00251 AUTH_CALLBACK_REPLY_ATTERR_ATTRIBUTE_NOT_FOUND = 0x010A, 00252 00253 /** 00254 * ATT Error: Attribute cannot be read or written using read/write blob 00255 * requests. 00256 */ 00257 AUTH_CALLBACK_REPLY_ATTERR_ATTRIBUTE_NOT_LONG = 0x010B, 00258 00259 /** 00260 * ATT Error: Invalid value size. 00261 */ 00262 AUTH_CALLBACK_REPLY_ATTERR_INVALID_ATT_VAL_LENGTH = 0x010D, 00263 00264 /** 00265 * ATT Error: Encrypted link required. 00266 */ 00267 AUTH_CALLBACK_REPLY_ATTERR_INSUF_RESOURCES = 0x0111, 00268 }; 00269 00270 /** 00271 * GATT write authorization request event. 00272 */ 00273 struct GattWriteAuthCallbackParams { 00274 /** 00275 * Handle of the connection that triggered the event. 00276 */ 00277 Gap::Handle_t connHandle; 00278 00279 /** 00280 * Attribute Handle to which the write operation applies. 00281 */ 00282 GattAttribute::Handle_t handle; 00283 00284 /** 00285 * Offset for the write operation. 00286 */ 00287 uint16_t offset; 00288 00289 /** 00290 * Length of the incoming data. 00291 */ 00292 uint16_t len; 00293 00294 /** 00295 * Incoming data. 00296 */ 00297 const uint8_t *data; 00298 00299 /** 00300 * Authorization result. 00301 * 00302 * The callback sets this parameter. If the value is set to 00303 * AUTH_CALLBACK_REPLY_SUCCESS, then the write request is accepted; 00304 * otherwise, an error code is returned to the peer client. 00305 */ 00306 GattAuthCallbackReply_t authorizationReply; 00307 }; 00308 00309 /** 00310 * GATT read authorization request event. 00311 */ 00312 struct GattReadAuthCallbackParams { 00313 /** 00314 * The handle of the connection that triggered the event. 00315 */ 00316 Gap::Handle_t connHandle; 00317 00318 /** 00319 * Attribute Handle to which the read operation applies. 00320 */ 00321 GattAttribute::Handle_t handle; 00322 00323 /** 00324 * Offset for the read operation. 00325 */ 00326 uint16_t offset; 00327 00328 /** 00329 * Optional: new length of the outgoing data. 00330 */ 00331 uint16_t len; 00332 00333 /** 00334 * Optional: new outgoing data. Leave at NULL if data is unchanged. 00335 */ 00336 uint8_t *data; 00337 00338 /** 00339 * Authorization result. 00340 * 00341 * The callback sets this parameter. If the value is set to 00342 * AUTH_CALLBACK_REPLY_SUCCESS, then the read request is accepted; 00343 * otherwise, an error code is returned to the peer client. 00344 */ 00345 GattAuthCallbackReply_t authorizationReply; 00346 }; 00347 00348 /** 00349 * Handle Value Notification/Indication event. 00350 * 00351 * The GattClient generates this type of event upon the reception of a 00352 * Handle Value Notification or Indication. 00353 * 00354 * The event is passed to callbacks registered by GattClient::onHVX(). 00355 */ 00356 struct GattHVXCallbackParams { 00357 /** 00358 * The handle of the connection that triggered the event. 00359 */ 00360 Gap::Handle_t connHandle; 00361 00362 /** 00363 * Attribute Handle to which the HVx operation applies. 00364 */ 00365 GattAttribute::Handle_t handle; 00366 00367 /** 00368 * Indication or Notification, see HVXType_t. 00369 */ 00370 HVXType_t type; 00371 00372 /** 00373 * Attribute value length. 00374 */ 00375 uint16_t len; 00376 00377 /** 00378 * Attribute value. 00379 */ 00380 const uint8_t *data; 00381 00382 }; 00383 00384 /** 00385 * @} 00386 * @} 00387 * @} 00388 */ 00389 00390 #endif /*MBED_BLE_GATT_CALLBACK_PARAM_TYPES_H__*/
Generated on Sun Jul 17 2022 08:25:23 by
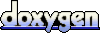