
An fully working IMU-Filter and Sensor drivers for the 10DOF-Board over I2C. All in one simple class. Include, calibrate sensors, call read, get angles. (3D Visualisation code for Python also included) Sensors: L3G4200D, ADXL345, HMC5883, BMP085
BMP085.h
00001 // based on http://mbed.org/users/okini3939/code/BMP085/ 00002 00003 #ifndef BMP085_H 00004 #define BMP085_H 00005 00006 #include "mbed.h" 00007 #include "I2C_Sensor.h" 00008 00009 #define BMP085_I2C_ADDRESS 0xEE 00010 00011 // register addresses 00012 #define BMP085_CAL_AC1 0xAA // R Calibration data (16 bits) 00013 #define BMP085_CAL_AC2 0xAC // R Calibration data (16 bits) 00014 #define BMP085_CAL_AC3 0xAE // R Calibration data (16 bits) 00015 #define BMP085_CAL_AC4 0xB0 // R Calibration data (16 bits) 00016 #define BMP085_CAL_AC5 0xB2 // R Calibration data (16 bits) 00017 #define BMP085_CAL_AC6 0xB4 // R Calibration data (16 bits) 00018 #define BMP085_CAL_B1 0xB6 // R Calibration data (16 bits) 00019 #define BMP085_CAL_B2 0xB8 // R Calibration data (16 bits) 00020 #define BMP085_CAL_MB 0xBA // R Calibration data (16 bits) 00021 #define BMP085_CAL_MC 0xBC // R Calibration data (16 bits) 00022 #define BMP085_CAL_MD 0xBE // R Calibration data (16 bits) 00023 #define BMP085_CONTROL 0xF4 // W Control register 00024 #define BMP085_CONTROL_OUTPUT 0xF6 // R Output registers 0xF6=MSB, 0xF7=LSB, 0xF8=XLSB 00025 00026 #define BMP085_SOFTRESET 0xE0 // -- unused registers 00027 #define BMP085_VERSION 0xD1 // ML_VERSION pos=0 len=4 msk=0F AL_VERSION pos=4 len=4 msk=f0 00028 #define BMP085_CHIPID 0xD0 // pos=0 mask=FF len=8 BMP085_CHIP_ID=0x55 00029 00030 // BMP085 Modes 00031 #define MODE_ULTRA_LOW_POWER 0 //oversampling=0, internalsamples=1, maxconvtimepressure=4.5ms, avgcurrent=3uA, RMSnoise_hPA=0.06, RMSnoise_m=0.5 00032 #define MODE_STANDARD 1 //oversampling=1, internalsamples=2, maxconvtimepressure=7.5ms, avgcurrent=5uA, RMSnoise_hPA=0.05, RMSnoise_m=0.4 00033 #define MODE_HIGHRES 2 //oversampling=2, internalsamples=4, maxconvtimepressure=13.5ms, avgcurrent=7uA, RMSnoise_hPA=0.04, RMSnoise_m=0.3 00034 #define MODE_ULTRA_HIGHRES 3 //oversampling=3, internalsamples=8, maxconvtimepressure=25.5ms, avgcurrent=12uA, RMSnoise_hPA=0.03, RMSnoise_m=0.25 00035 // "Sampling rate can be increased to 128 samples per second (standard mode) for 00036 // dynamic measurement.In this case it is sufficient to measure temperature only 00037 // once per second and to use this value for all pressure measurements during period." 00038 // (from BMP085 datasheet Rev1.2 page 10). 00039 // Control register 00040 #define READ_TEMPERATURE 0x2E 00041 #define READ_PRESSURE 0x34 00042 //Other 00043 #define MSLP 101325 // Mean Sea Level Pressure = 1013.25 hPA (1hPa = 100Pa = 1mbar) 00044 00045 class BMP085 : public I2C_Sensor { 00046 public: 00047 BMP085(PinName sda, PinName scl); 00048 00049 virtual void read(); 00050 float Temperature, Pressure, Altitude; 00051 00052 private: 00053 short AC1, AC2, AC3, B1, B2, MB, MC, MD; // calibration data 00054 unsigned short AC4, AC5, AC6; 00055 short oss; 00056 00057 virtual void readraw(); 00058 }; 00059 00060 #endif
Generated on Wed Jul 13 2022 22:03:48 by
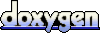