TextLCD library for controlling various LCD panels based on the HD44780 4-bit interface khdvdhjhd
Dependents: tarea2 PID tarea2 teclados_incrementales_PID
Fork of TextLCD by
TextLCD.h
00001 /* mbed TextLCD Library, for a 4-bit LCD based on HD44780 00002 * Copyright (c) 2007-2010, sford, http://mbed.org 00003 * 00004 * Permission is hereby granted, free of charge, to any person obtaining a copy 00005 * of this software and associated documentation files (the "Software"), to deal 00006 * in the Software without restriction, including without limitation the rights 00007 * to use, copy, modify, merge, publish, distribute, sublicense, and/or sell 00008 * copies of the Software, and to permit persons to whom the Software is 00009 * furnished to do so, subject to the following conditions: 00010 * 00011 * The above copyright notice and this permission notice shall be included in 00012 * all copies or substantial portions of the Software. 00013 * 00014 * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR 00015 * IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, 00016 * FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE 00017 * AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER 00018 * LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00019 * OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN 00020 * THE SOFTWARE. 00021 */ 00022 00023 #ifndef MBED_TEXTLCD_H 00024 #define MBED_TEXTLCD_H 00025 00026 #include "mbed.h" 00027 00028 /** A TextLCD interface for driving 4-bit HD44780-based LCDs 00029 * 00030 * Currently supports 16x2, 20x2 and 20x4 panels 00031 * 00032 * @code 00033 * #include "mbed.h" 00034 * #include "TextLCD.h" 00035 * 00036 * TextLCD lcd(p10, p12, p15, p16, p29, p30); // rs, e, d4-d7 00037 * 00038 * int main() { 00039 * lcd.printf("Hello World!\n"); 00040 * } 00041 * @endcode 00042 */ 00043 class TextLCD : public Stream { 00044 public: 00045 void writeCommand(int command); //quedo publica ya la podemos usar en el codigo C 00046 /** LCD panel format */ 00047 enum LCDType { 00048 LCD16x2 /**< 16x2 LCD panel (default) */ 00049 , LCD16x2B /**< 16x2 LCD panel alternate addressing */ 00050 , LCD20x2 /**< 20x2 LCD panel */ 00051 , LCD20x4 /**< 20x4 LCD panel */ 00052 }; 00053 00054 /** Create a TextLCD interface 00055 * 00056 * @param rs Instruction/data control line 00057 * @param e Enable line (clock) 00058 * @param d4-d7 Data lines for using as a 4-bit interface 00059 * @param type Sets the panel size/addressing mode (default = LCD16x2) 00060 */ 00061 TextLCD(PinName rs, PinName e, PinName d4, PinName d5, PinName d6, PinName d7, LCDType type = LCD16x2); 00062 00063 #if DOXYGEN_ONLY 00064 /** Write a character to the LCD 00065 * 00066 * @param c The character to write to the display 00067 */ 00068 int putc(int c); 00069 00070 /** Write a formated string to the LCD 00071 * 00072 * @param format A printf-style format string, followed by the 00073 * variables to use in formating the string. 00074 */ 00075 int printf(const char* format, ...); 00076 #endif 00077 00078 /** Locate to a screen column and row 00079 * 00080 * @param column The horizontal position from the left, indexed from 0 00081 * @param row The vertical position from the top, indexed from 0 00082 */ 00083 void locate(int column, int row); 00084 00085 /** Clear the screen and locate to 0,0 */ 00086 void cls(); 00087 00088 int rows(); 00089 int columns(); 00090 00091 00092 protected: 00093 00094 // Stream implementation functions 00095 virtual int _putc(int value); 00096 virtual int _getc(); 00097 00098 int address(int column, int row); 00099 void character(int column, int row, int c); 00100 void writeByte(int value); 00101 //void writeCommand(int command); esta se documenta para poder poner comandos quedo publica 00102 void writeData(int data); 00103 00104 DigitalOut _rs, _e; 00105 BusOut _d; 00106 LCDType _type; 00107 00108 int _column; 00109 int _row; 00110 }; 00111 00112 #endif 00113
Generated on Thu Jul 21 2022 00:13:24 by
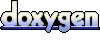