pins change for LPC1114FN28
Fork of MCP4922 by
MCP4922 Class Reference
The MCP4922 is a dual package 12 bit DAC. More...
#include <MCP4922.h>
Public Member Functions | |
MCP4922 (int SPIchannelNum, PinName CS, PinName LDAC) | |
Initializes the MCP 4922 DAC. | |
virtual void | write (int DACvalue) |
Writes a value between 0-4095 to the currently selected DAC output. | |
virtual void | write_mV (int millivolts) |
Writes a value in mV to the DAC outputs. | |
void | update () |
If automatic updating is disabled, this will update the DAC output on command. | |
virtual void | select (char DACnum) |
select whether to use DAC A or DAC B. | |
void | setGain (int gain_value) |
Manually set the gain of the DAC. | |
Data Fields | |
bool | autoUpdate |
If true, the DAC output will update as soon as the output value is modified. | |
char | DACselect |
The currently selected DAC channel. | |
int | VrefA |
The output scaling factor in mV for channel A. | |
int | VrefB |
The output scaling factor in mV for channel B. | |
char | buffered |
Turn on or off the output buffer. |
Detailed Description
The MCP4922 is a dual package 12 bit DAC.
We should be able to produce two analog output voltages up to twice the externally wired voltage references (VrefA, VrefB). The maximum voltage output is limited by the input voltage at V_DD which can go up to about 5.5V.
Datasheet: http://ww1.microchip.com/downloads/en/DeviceDoc/22250A.pdf
All serial commands are 16 bit words. The highest 4 bits are control bits, while the last 12 are the data bits for the 12-bit DAC MCP4822. + bit 15: select which DAC to use. + bit 14: 0=buffered , 1= unbuffered + bit 13: 0= gain x2, 1= gain x1 + bit 12: 0= DAC active, 1= shut down DAC + bit 11-0: Data bits for the DAC.
Definition at line 19 of file MCP4922.h.
Constructor & Destructor Documentation
MCP4922 | ( | int | SPIchannelNum, |
PinName | CS, | ||
PinName | LDAC | ||
) |
Initializes the MCP 4922 DAC.
- Parameters:
-
SPIchannelNum An int representing the SPI channel used by this DAC channel 0 for p5 and p7 channel 1 for p11 and p13 CS The chip select pin used to identify the device LDAC The LDAC pin used to synchronize updates of multiple devices
Definition at line 3 of file MCP4922.cpp.
Member Function Documentation
void select | ( | char | DACnum ) | [virtual] |
select whether to use DAC A or DAC B.
- Parameters:
-
DACnum 0 to modify DAC A, 1 to modify DAC B
Definition at line 13 of file MCP4922.cpp.
void setGain | ( | int | gain_value ) |
Manually set the gain of the DAC.
The only valid values are 1 and 2. The default gain is x1 to match the wired reference voltage
Definition at line 64 of file MCP4922.cpp.
void update | ( | ) |
If automatic updating is disabled, this will update the DAC output on command.
Definition at line 58 of file MCP4922.cpp.
void write | ( | int | DACvalue ) | [virtual] |
Writes a value between 0-4095 to the currently selected DAC output.
- Parameters:
-
DACvalue a value from 0-4095 to write to the DAC register
Definition at line 29 of file MCP4922.cpp.
void write_mV | ( | int | millivolts ) | [virtual] |
Writes a value in mV to the DAC outputs.
The output will only be accurate if Vref is set to the appropriate voltage reference scaling factor.
- Parameters:
-
millivolte The desired voltage output in millivolts
Definition at line 17 of file MCP4922.cpp.
Field Documentation
bool autoUpdate |
char buffered |
char DACselect |
int VrefA |
The output scaling factor in mV for channel A.
The voltage wired to Vref will be used as the scaling factor which the DAC will use in producing the output voltage. If using the write_mV function, it is important that Vref is properly set. This library uses 5000mV by default.
int VrefB |
The output scaling factor in mV for channel B.
The voltage wired to Vref will be used as the scaling factor which the DAC will use in producing the output voltage. If using the write_mV function, it is important that Vref is properly set. This library uses 5000mV by default.
Generated on Fri Jul 15 2022 14:59:56 by
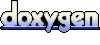