pins change for LPC1114FN28
Fork of MCP4922 by
Embed:
(wiki syntax)
Show/hide line numbers
MCP4922.h
00001 #ifndef MCP4922_H 00002 #define MCP4922_H 00003 00004 #include "DAC_SPI.h" 00005 00006 /** 00007 * The MCP4922 is a dual package 12 bit DAC. We should be able to produce two analog output voltages up to twice the externally wired voltage references (VrefA, VrefB). 00008 The maximum voltage output is limited by the input voltage at V_DD which can go up to about 5.5V. 00009 00010 * Datasheet: http://ww1.microchip.com/downloads/en/DeviceDoc/22250A.pdf 00011 00012 * All serial commands are 16 bit words. The highest 4 bits are control bits, while the last 12 are the data bits for the 12-bit DAC MCP4822. 00013 * + bit 15: select which DAC to use. 00014 * + bit 14: 0=buffered , 1= unbuffered 00015 * + bit 13: 0= gain x2, 1= gain x1 00016 * + bit 12: 0= DAC active, 1= shut down DAC 00017 * + bit 11-0: Data bits for the DAC. 00018 */ 00019 class MCP4922: public DAC_SPI 00020 { 00021 public: 00022 00023 /** Initializes the MCP 4922 DAC 00024 * 00025 * @param SPIchannelNum An int representing the SPI channel used by this DAC 00026 * channel 0 for p5 and p7 00027 * channel 1 for p11 and p13 00028 * @param CS The chip select pin used to identify the device 00029 * @param LDAC The LDAC pin used to synchronize updates of multiple devices 00030 */ 00031 MCP4922(int SPIchannelNum, PinName CS, PinName LDAC); 00032 00033 /** Writes a value between 0-4095 to the currently selected DAC output 00034 * @param DACvalue a value from 0-4095 to write to the DAC register 00035 */ 00036 virtual void write(int DACvalue); 00037 00038 /** Writes a value in mV to the DAC outputs. 00039 * The output will only be accurate if Vref is set to the appropriate voltage reference scaling factor. 00040 * @param millivolte The desired voltage output in millivolts 00041 */ 00042 virtual void write_mV(int millivolts); 00043 00044 /** If automatic updating is disabled, this will update the DAC output on command */ 00045 void update(); 00046 00047 /** select whether to use DAC A or DAC B. 00048 * @param DACnum 0 to modify DAC A, 1 to modify DAC B 00049 */ 00050 virtual void select(char DACnum); 00051 00052 /** If true, the DAC output will update as soon as the output value is modified. If false, it will wait for an update command*/ 00053 bool autoUpdate; 00054 00055 /** The currently selected DAC channel. 0 for DAC A, 1 for DAC B*/ 00056 char DACselect; 00057 00058 /** The output scaling factor in mV for channel A. 00059 * The voltage wired to Vref will be used as the scaling factor which the DAC will use in producing the output voltage. 00060 * If using the write_mV function, it is important that Vref is properly set. This library uses 5000mV by default. 00061 */ 00062 int VrefA; 00063 /** The output scaling factor in mV for channel B. 00064 * The voltage wired to Vref will be used as the scaling factor which the DAC will use in producing the output voltage. 00065 * If using the write_mV function, it is important that Vref is properly set. This library uses 5000mV by default. 00066 */ 00067 int VrefB; 00068 00069 /** Manually set the gain of the DAC. The only valid values are 1 and 2. The default gain is x1 to match the wired reference voltage*/ 00070 void setGain(int gain_value); 00071 00072 /** Turn on or off the output buffer. The default value for the buffer is off. See section 4.1.2 of the datasheet for more information. */ 00073 char buffered; 00074 00075 private: 00076 /** The output gain bit of the DAC. If set to 0, gain is x2. If set to 1 gain is x1. The default gain is 1.*/ 00077 bool gain; 00078 }; 00079 00080 #endif //MCP4922_H
Generated on Fri Jul 15 2022 14:59:56 by
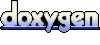