pins change for LPC1114FN28
Fork of MCP4922 by
Embed:
(wiki syntax)
Show/hide line numbers
MCP4922.cpp
00001 #include "MCP4922.h" 00002 00003 MCP4922::MCP4922(int SPIchannelNum, PinName _CS, PinName _LDAC) : DAC_SPI(SPIchannelNum, _CS, _LDAC){ 00004 messageBits(16); //messages use a 16 bit word 00005 frequency(20000000); //set default frequency to 20MHz 00006 autoUpdate=1; 00007 VrefA=5000; //assume a 5V reference voltage for use with write_mv(). This value can be configured; 00008 VrefB=5000; 00009 gain =1; //set gain to x1 to match Vref. 00010 buffered =0; //leave output buffer off 00011 } 00012 00013 void MCP4922::select(char DACnum){ 00014 DACselect=DACnum & 0x01; //choose between DAC A and DAC B 00015 } 00016 00017 void MCP4922::write_mV(int mV){ 00018 int Vref; 00019 if (DACselect==1){ 00020 Vref=VrefB; 00021 } 00022 else{ 00023 Vref=VrefA; 00024 } 00025 int DACvalue= mV* 4096/Vref *(gain+1); //scale voltage to a DAC value. 00026 write(DACvalue); 00027 } 00028 00029 void MCP4922::write(int value){ 00030 //valid input values are 0 - 4095. 4096 should scale to Vref. 00031 //All serial commands are 16 bit words. The highest 4 bits are control bits, while the last 12 are the data bits for the 12-bit DAC MCP4822. 00032 //bit 15: select which DAC to use. 00033 //bit 14: 0=unbuffered , 1= buffered 00034 //bit 13: 0= gain x2, 1= gain x1 00035 //bit 12: 0= DAC active, 1= shut down DAC 00036 //bit 11-0: Data bits for the DAC. 00037 00038 if (value > 0xFFF){ 00039 value = 0xFFF; //any out of range values will be truncated to our max value 00040 } 00041 value=value & 0xFFF; //limit our value to 12 bits. 00042 00043 //SCK=0; //set the clock low. Data is read on the rising edge of the clock. 00044 LDAC=1; 00045 CS=0; //enable the chip to recieve data 00046 //bit 13: 0= gain x2, 1= gain x1 00047 char gain2 = 0; 00048 if( gain == 0 ) 00049 gain2 = 1; 00050 char controlbits=(DACselect<<3) + (buffered<<2) + (gain2<<1) +1; 00051 00052 int message= (controlbits<<12)+value; 00053 (*DACspi).write(message); 00054 CS=1; //signal end of message. The data will be loaded into the internal registers. 00055 if(autoUpdate){ LDAC=0;} //trigger the update of the output voltage. 00056 00057 } 00058 void MCP4922::update(){ 00059 //triggers the DAC to update output on command. Useful if we wish to synchronize the DAC output value with another event. 00060 LDAC=0; 00061 } 00062 00063 //Set the multiplying factor for the output. Valid inputs are gains of 1 and 2.*/ 00064 void MCP4922::setGain(int gain_value){ 00065 if (gain_value>1){ 00066 gain =1; //Set gain to x2 00067 } 00068 else{ 00069 gain=0; //Set gain to x1. Limits range to 2.098mV 00070 } 00071 }
Generated on Fri Jul 15 2022 14:59:56 by
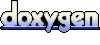