Device driver for the BMP180 Digital pressure and temperature sensor.
Dependents: BMP180_example MAXWSNENV_sensors MAXWSNENV_sensors nRF51822_BMP180 ... more
BMP180.h
00001 /******************************************************************************* 00002 * Copyright (C) 2015 Maxim Integrated Products, Inc., All Rights Reserved. 00003 * 00004 * Permission is hereby granted, free of charge, to any person obtaining a 00005 * copy of this software and associated documentation files (the "Software"), 00006 * to deal in the Software without restriction, including without limitation 00007 * the rights to use, copy, modify, merge, publish, distribute, sublicense, 00008 * and/or sell copies of the Software, and to permit persons to whom the 00009 * Software is furnished to do so, subject to the following conditions: 00010 * 00011 * The above copyright notice and this permission notice shall be included 00012 * in all copies or substantial portions of the Software. 00013 * 00014 * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS 00015 * OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF 00016 * MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. 00017 * IN NO EVENT SHALL MAXIM INTEGRATED BE LIABLE FOR ANY CLAIM, DAMAGES 00018 * OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, 00019 * ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR 00020 * OTHER DEALINGS IN THE SOFTWARE. 00021 * 00022 * Except as contained in this notice, the name of Maxim Integrated 00023 * Products, Inc. shall not be used except as stated in the Maxim Integrated 00024 * Products, Inc. Branding Policy. 00025 * 00026 * The mere transfer of this software does not imply any licenses 00027 * of trade secrets, proprietary technology, copyrights, patents, 00028 * trademarks, maskwork rights, or any other form of intellectual 00029 * property whatsoever. Maxim Integrated Products, Inc. retains all 00030 * ownership rights. 00031 ******************************************************************************* 00032 */ 00033 00034 #ifndef _BMP180_H_ 00035 #define _BMP180_H_ 00036 00037 #include "mbed.h" 00038 00039 /** 00040 * Bosch BMP180 Digital Pressure Sensor 00041 * 00042 * @code 00043 * #include <stdio.h> 00044 * #include "mbed.h" 00045 * #include "BMP180.h" 00046 * 00047 * I2C i2c(I2C_SDA, I2C_SCL); 00048 * BMP180 bmp180(&i2c); 00049 * 00050 * int main(void) { 00051 * 00052 * while(1) { 00053 * if (bmp180.init() != 0) { 00054 * printf("Error communicating with BMP180\n"); 00055 * } else { 00056 * printf("Initialized BMP180\n"); 00057 * break; 00058 * } 00059 * wait(1); 00060 * } 00061 * 00062 * while(1) { 00063 * bmp180.startTemperature(); 00064 * wait_ms(5); // Wait for conversion to complete 00065 * float temp; 00066 * if(bmp180.getTemperature(&temp) != 0) { 00067 * printf("Error getting temperature\n"); 00068 * continue; 00069 * } 00070 * 00071 * bmp180.startPressure(BMP180::ULTRA_LOW_POWER); 00072 * wait_ms(10); // Wait for conversion to complete 00073 * int pressure; 00074 * if(bmp180.getPressure(&pressure) != 0) { 00075 * printf("Error getting pressure\n"); 00076 * continue; 00077 * } 00078 * 00079 * printf("Pressure = %d Pa Temperature = %f C\n", pressure, temp); 00080 * wait(1); 00081 * } 00082 * } 00083 * @endcode 00084 */ 00085 class BMP180 00086 { 00087 00088 public: 00089 00090 /** 00091 * @brief Oversampling ratio. 00092 * @details Dictates how many pressure samples to take. Conversion time varies 00093 * depending on the number of samples taken. Refer to data sheet 00094 * for timing specifications. 00095 */ 00096 typedef enum { 00097 ULTRA_LOW_POWER = 0, ///< 1 pressure sample 00098 STANDARD = 1, ///< 2 pressure samples 00099 HIGH_RESOLUTION = 2, ///< 4 pressure samples 00100 ULTRA_HIGH_RESOLUTION = 3, ///< 8 pressure samples 00101 } oversampling_t; 00102 00103 /** 00104 * BMP180 constructor. 00105 * 00106 * @param sda mbed pin to use for SDA line of I2C interface. 00107 * @param scl mbed pin to use for SCL line of I2C interface. 00108 */ 00109 BMP180(PinName sda, PinName scl); 00110 00111 /** 00112 * BMP180 constructor. 00113 * 00114 * @param i2c I2C object to use. 00115 */ 00116 BMP180(I2C *i2c); 00117 00118 /** 00119 * BMP180 destructor. 00120 */ 00121 ~BMP180(); 00122 00123 /** 00124 * @brief Initialize BMP180. 00125 * @details Gets the device ID and saves the calibration values. 00126 * @returns 0 if no errors, -1 if error. 00127 */ 00128 int init(void); 00129 00130 /** 00131 * @brief Reset BMP180. 00132 * @details Performs a soft reset of the device. Same sequence as power on reset. 00133 * @returns 0 if no errors, -1 if error. 00134 */ 00135 int reset(void); 00136 00137 /** 00138 * @brief Check ID. 00139 * @details Checks the device ID, should be 0x55 on reset. 00140 * @returns 0 if no errors, -1 if error. 00141 */ 00142 int checkId(void); 00143 00144 /** 00145 * @brief Start pressure conversion. 00146 * @details Initiates the pressure conversion sequence. Refer to data sheet 00147 * for timing specifications. 00148 * 00149 * @param oss Number of samples to take. 00150 * @returns 0 if no errors, -1 if error. 00151 */ 00152 int startPressure(BMP180::oversampling_t oss); 00153 00154 /** 00155 * @brief Get pressure reading. 00156 * @details Calculates the pressure using the data calibration data and formula. 00157 * Pressure is reported in Pascals. 00158 * @note This function should be called after calling startPressure(). 00159 * Refer to the data sheet for the timing requirements. Calling this 00160 * function too soon can result in oversampling. 00161 * 00162 * @param pressure Pointer to store pressure reading. 00163 * @returns 0 if no errors, -1 if error. 00164 */ 00165 int getPressure(int *pressure); 00166 00167 /** 00168 * @brief Start temperature conversion. 00169 * @details Initiates the temperature conversion sequence. Refer to data 00170 * sheet for timing specifications. 00171 * @returns 0 if no errors, -1 if error. 00172 */ 00173 int startTemperature(void); 00174 00175 /** 00176 * @brief Get temperature reading. 00177 * @details Calculates the temperature using the data calibration data and formula. 00178 * Temperature is reported in degrees Celcius. 00179 * 00180 * @note This function should be called after calling startTemperature(). 00181 * Refer to the data sheet for the timing requirements. Calling this 00182 * function too soon can result in oversampling. 00183 * 00184 * @param tempC Pointer to store temperature reading. 00185 * @returns 0 if no errors, -1 if error. 00186 */ 00187 int getTemperature(float *tempC); 00188 00189 /** 00190 * @brief Get temperature reading. 00191 * @details Calculates the temperature using the data calibration data and formula. 00192 * Temperature is reported in 1/10ths degrees Celcius. 00193 * 00194 * @note This function should be called after calling startTemperature(). 00195 * Refer to the data sheet for the timing requirements. Calling this 00196 * function too soon can result in oversampling. 00197 * 00198 * @param tempCx10 Pointer to store temperature reading. 00199 * @returns 0 if no errors, -1 if error. 00200 */ 00201 int getTemperature(int16_t *tempCx10); 00202 00203 private: 00204 00205 typedef union { 00206 uint16_t value[11]; 00207 struct { 00208 int16_t ac1; 00209 int16_t ac2; 00210 int16_t ac3; 00211 uint16_t ac4; 00212 uint16_t ac5; 00213 uint16_t ac6; 00214 int16_t b1; 00215 int16_t b2; 00216 int16_t mb; 00217 int16_t mc; 00218 int16_t md; 00219 }; 00220 } calibration_t; 00221 00222 I2C *i2c_; 00223 bool i2c_owner; 00224 00225 BMP180::calibration_t calib; 00226 int32_t b5; 00227 BMP180::oversampling_t oss_; 00228 }; 00229 00230 #endif /* _BMP180_H_ */
Generated on Tue Jul 12 2022 16:46:11 by
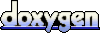