M41T62 is a serial real-time clock (RTC) made by STMicroelectronics.
Dependents: LPC1114_data_logger Check_external_RTC LPC1114_barometer_with_data_logging
M41T62 Class Reference
Interface for RTC (I2C Interface) STMicroelectronics M41T62. More...
#include <m41t62_rtc.h>
Public Member Functions | |
M41T62 (PinName p_sda, PinName p_scl) | |
Configure data pin (with other devices on I2C line) | |
M41T62 (I2C &p_i2c) | |
Configure data pin (with other devices on I2C line) | |
void | read_rtc_std (tm *) |
Read RTC data with Standard C "struct tm" format. | |
void | write_rtc_std (tm *) |
Write data to RTC data with Standard C "struct tm" format. | |
void | set_next_IRQ (uint16_t time) |
Set Alarm / IRQ time. | |
void | clear_IRQ (void) |
Clear Alarm / IRQ pin interrupt. | |
void | read_rtc_direct (rtc_time *) |
Read RTC data with own format. | |
void | write_rtc_direct (rtc_time *) |
Read RTC data with own format. | |
void | frequency (int) |
Set I2C clock frequency. | |
void | set_sq_wave (sq_wave_t) |
Control Square wave output port. |
Detailed Description
Interface for RTC (I2C Interface) STMicroelectronics M41T62.
Standalone type RTC via I2C interface
#include "mbed.h" #include "m41t62_rtc.h" // I2C Communication M41T62 m41t62(dp5,dp27); // STmicro RTC(M41T62) SDA, SCL // If you connected I2C line not only this device but also other devices, // you need to declare following method. I2C i2c(dp5,dp27); // SDA, SCL M41T62 m41t62(i2c); // STmicro RTC(M41T62) int main() { tm t; time_t seconds; char buf[40]; m41t62.set_sq_wave(RTC_SQW_NONE); // Stop output for more low current while(1){ m41t62.read_rtc_std(&t); // read RTC data seconds = mktime(&t); strftime(buf, 40, "%I:%M:%S %p (%Y/%m/%d)", localtime(&seconds)); printf("Date: %s\r\n", buf); } }
Definition at line 100 of file m41t62_rtc.h.
Constructor & Destructor Documentation
M41T62 | ( | PinName | p_sda, |
PinName | p_scl | ||
) |
Configure data pin (with other devices on I2C line)
- Parameters:
-
data SDA and SCL pins
Definition at line 18 of file m41t62_rtc.cpp.
M41T62 | ( | I2C & | p_i2c ) |
Configure data pin (with other devices on I2C line)
- Parameters:
-
I2C previous definition
Definition at line 25 of file m41t62_rtc.cpp.
Member Function Documentation
void clear_IRQ | ( | void | ) |
Clear Alarm / IRQ pin interrupt.
- Parameters:
-
none
- Returns:
- none
Definition at line 171 of file m41t62_rtc.cpp.
void frequency | ( | int | hz ) |
Set I2C clock frequency.
- Parameters:
-
freq.
- Returns:
- none
Definition at line 198 of file m41t62_rtc.cpp.
void read_rtc_direct | ( | rtc_time * | tm ) |
Read RTC data with own format.
- Parameters:
-
tm (data save area)
- Returns:
- none but all data in tm
Definition at line 82 of file m41t62_rtc.cpp.
void read_rtc_std | ( | tm * | t ) |
Read RTC data with Standard C "struct tm" format.
- Parameters:
-
tm (data save area)
- Returns:
- none but all data in tm
Definition at line 38 of file m41t62_rtc.cpp.
void set_next_IRQ | ( | uint16_t | time ) |
Set Alarm / IRQ time.
- Parameters:
-
next time (unit: minutes) from now on minimum = 2 minutes!!
- Returns:
- none
Definition at line 153 of file m41t62_rtc.cpp.
void set_sq_wave | ( | sq_wave_t | sqw_dt ) |
Control Square wave output port.
- Parameters:
-
output_mode
- Returns:
- none
Definition at line 204 of file m41t62_rtc.cpp.
void write_rtc_direct | ( | rtc_time * | tm ) |
Read RTC data with own format.
- Parameters:
-
tm (save writing data)
- Returns:
- none but all data in tm
Definition at line 97 of file m41t62_rtc.cpp.
void write_rtc_std | ( | tm * | t ) |
Write data to RTC data with Standard C "struct tm" format.
- Parameters:
-
tm (save writing data)
- Returns:
- none but all data in tm
Definition at line 63 of file m41t62_rtc.cpp.
Generated on Wed Jul 13 2022 00:47:59 by
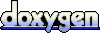