M41T62 is a serial real-time clock (RTC) made by STMicroelectronics.
Dependents: LPC1114_data_logger Check_external_RTC LPC1114_barometer_with_data_logging
m41t62_rtc.h
00001 /* 00002 * mbed library program 00003 * Control M41T62 RTC Module 00004 * STMicroelectronics 00005 * 00006 * Copyright (c) 2014,'15,'17,'20 Kenji Arai / JH1PJL 00007 * http://www7b.biglobe.ne.jp/~kenjia/ 00008 * https://os.mbed.com/users/kenjiArai/ 00009 * Created: June 21st, 2014 00010 * Revised: August 7th, 2020 00011 */ 00012 00013 /* 00014 *---------------- REFERENCE --------------------------------------------------- 00015 * http://www.st-japan.co.jp/web/jp/ 00016 * catalog/sense_power/FM151/CL1410/SC403/PF82507 00017 * http://strawberry-linux.com/catalog/items?code=12062 00018 */ 00019 00020 #ifndef M41T62_H 00021 #define M41T62_H 00022 00023 #include "mbed.h" 00024 00025 // RTC STmicro M41T62 00026 // Address b7=1,b6=1,b5=0,b4=1,b3=0,b2=0,b1=0, b0=R/W 00027 #define M41T62ADDR (0x68 << 1) // No other choice 00028 00029 // Register definition 00030 #define M41T62_REG_SSEC 0x0 00031 #define M41T62_REG_SEC 0x1 00032 #define M41T62_REG_MIN 0x2 00033 #define M41T62_REG_HOUR 0x3 00034 #define M41T62_REG_WDAY 0x4 00035 #define M41T62_REG_DAY 0x5 00036 #define M41T62_REG_MON 0x6 00037 #define M41T62_REG_YEAR 0x7 00038 #define M41T62_REG_CALIB 0x8 00039 #define M41T62_REG_WDT 0x9 00040 #define M41T62_REG_ALARM_MON 0xa 00041 #define M41T62_REG_ALARM_DAY 0xb 00042 #define M41T62_REG_ALARM_HOUR 0xc 00043 #define M41T62_REG_ALARM_MIN 0xd 00044 #define M41T62_REG_ALARM_SEC 0xe 00045 #define M41T62_REG_FLAGS 0xf 00046 00047 // Buffer size 00048 #define RTC_BUF_SIZ (M41T62_REG_FLAGS + 5) 00049 00050 typedef enum { 00051 RTC_SQW_NONE = 0, 00052 RTC_SQW_32KHZ, 00053 RTC_SQW_8KHZ, 00054 RTC_SQW_4KHZ, 00055 RTC_SQW_2KHZ, 00056 RTC_SQW_1KHZ, 00057 RTC_SQW_512HZ, 00058 RTC_SQW_256HZ, 00059 RTC_SQW_128HZ, 00060 RTC_SQW_64HZ, 00061 RTC_SQW_32HZ, 00062 RTC_SQW_16HZ, 00063 RTC_SQW_8HZ, 00064 RTC_SQW_4HZ, 00065 RTC_SQW_2HZ, 00066 RTC_SQW_1HZ 00067 } sq_wave_t; 00068 00069 /** Interface for RTC (I2C Interface) STMicroelectronics M41T62 00070 * 00071 * Standalone type RTC via I2C interface 00072 * 00073 * @code 00074 * #include "mbed.h" 00075 * #include "m41t62_rtc.h" 00076 * 00077 * // I2C Communication 00078 * M41T62 m41t62(dp5,dp27); // STmicro RTC(M41T62) SDA, SCL 00079 * // If you connected I2C line not only this device but also other devices, 00080 * // you need to declare following method. 00081 * I2C i2c(dp5,dp27); // SDA, SCL 00082 * M41T62 m41t62(i2c); // STmicro RTC(M41T62) 00083 * 00084 * int main() { 00085 * tm t; 00086 * time_t seconds; 00087 * char buf[40]; 00088 * 00089 * m41t62.set_sq_wave(RTC_SQW_NONE); // Stop output for more low current 00090 * while(1){ 00091 * m41t62.read_rtc_std(&t); // read RTC data 00092 * seconds = mktime(&t); 00093 * strftime(buf, 40, "%I:%M:%S %p (%Y/%m/%d)", localtime(&seconds)); 00094 * printf("Date: %s\r\n", buf); 00095 * } 00096 * } 00097 * @endcode 00098 */ 00099 00100 class M41T62 00101 { 00102 public: 00103 00104 typedef struct { // BCD format 00105 uint8_t rtc_seconds; 00106 uint8_t rtc_minutes; 00107 uint8_t rtc_hours; 00108 uint8_t rtc_weekday; 00109 uint8_t rtc_date; 00110 uint8_t rtc_month; 00111 uint8_t rtc_year_raw; 00112 uint16_t rtc_year; 00113 } rtc_time; 00114 00115 /** Configure data pin (with other devices on I2C line) 00116 * @param data SDA and SCL pins 00117 */ 00118 M41T62(PinName p_sda, PinName p_scl); 00119 00120 /** Configure data pin (with other devices on I2C line) 00121 * @param I2C previous definition 00122 */ 00123 M41T62(I2C& p_i2c); 00124 00125 /** Read RTC data with Standard C "struct tm" format 00126 * @param tm (data save area) 00127 * @return none but all data in tm 00128 */ 00129 void read_rtc_std(tm *); 00130 void get_time_rtc(tm *); 00131 00132 /** Write data to RTC data with Standard C "struct tm" format 00133 * @param tm (save writing data) 00134 * @return none but all data in tm 00135 */ 00136 void write_rtc_std(tm *); 00137 void set_time_rtc(tm *); 00138 00139 /** Set Alarm / IRQ time 00140 * @param next time (unit: minutes) from now on minimum = 2 minutes!! 00141 * @return none 00142 */ 00143 void set_next_IRQ(uint16_t time); 00144 00145 /** Clear Alarm / IRQ pin interrupt 00146 * @param none 00147 * @return none 00148 */ 00149 void clear_IRQ(void); 00150 00151 /** Read RTC data with own format 00152 * @param tm (data save area) 00153 * @return none but all data in tm 00154 */ 00155 void read_rtc_direct(rtc_time *); 00156 00157 /** Read RTC data with own format 00158 * @param tm (save writing data) 00159 * @return none but all data in tm 00160 */ 00161 void write_rtc_direct(rtc_time *); 00162 00163 /** Set I2C clock frequency 00164 * @param freq. 00165 * @return none 00166 */ 00167 void frequency(int); 00168 00169 /** Control Square wave output port 00170 * @param output_mode 00171 * @return none 00172 */ 00173 void set_sq_wave(sq_wave_t); 00174 00175 protected: 00176 I2C *_i2c_p; 00177 I2C &_i2c; 00178 00179 uint8_t bin2bcd(uint8_t); 00180 uint8_t bcd2bin(uint8_t); 00181 void set_alarm_reg (uint16_t time); 00182 00183 private: 00184 uint8_t M41T62_addr; 00185 uint8_t rtc_buf[RTC_BUF_SIZ]; // buffer for RTC 00186 00187 }; 00188 00189 #endif // M41T62_H
Generated on Wed Jul 13 2022 00:47:59 by
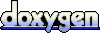