HD44780 compatible 16x2 character LCD driver with HC595 shiftregister
TriPinLcd Class Reference
tripinLcd mbed library for HD44780 compatible charachter LCD with HC595 by bitbanging More...
#include <TriPinLcd.h>
Public Member Functions | |
TriPinLcd (PinName data, PinName clock, PinName latch) | |
Constructor. | |
void | clear () |
clears display, set cursor position to zero | |
void | home () |
set cursor position to zero | |
void | noDisplay () |
Turn the display off. | |
void | display () |
Turn the display on. | |
void | noBlink () |
Turns off the blinking cursor. | |
void | blink () |
Turns on the blinking cursor. | |
void | noCursor () |
Turns the underline cursor off. | |
void | cursor () |
Turns the underline cursor on. | |
void | scrollDisplayLeft () |
scroll the display to left without changing the RAM | |
void | scrollDisplayRight () |
scroll the display to right without changing the RAM | |
void | leftToRight () |
This is for text that flows Left to Right. | |
void | rightToLeft () |
This is for text that flows Right to Left. | |
void | autoscroll () |
This will 'right justify' text from the cursor. | |
void | noAutoscroll () |
This will 'left justify' text from the cursor. | |
void | createChar (uint8_t, uint8_t[]) |
Allows us to fill the first 8 CGRAM locations with custom characters. | |
void | setCursor (uint8_t, uint8_t) |
set cursor position to (x=col,y=row); (0,0) is left-top |
Detailed Description
tripinLcd mbed library for HD44780 compatible charachter LCD with HC595 by bitbanging
Example:
#include "mbed.h" #include "TriPinLcd.h" Timer t; TriPinLcd lcd(p5,p7,p6);//clk = p7, data = p5, latch = p6 int main() { lcd.begin(16, 2); lcd.noBlink(); lcd.printf("LCD3WIRE mbed"); t.start(); while(1) { lcd.setCursor(0, 1); // lcd.printf("%.0f", t.read()); lcd.printf("%d", t.read_us()); } }
Definition at line 87 of file TriPinLcd.h.
Constructor & Destructor Documentation
TriPinLcd | ( | PinName | data, |
PinName | clock, | ||
PinName | latch | ||
) |
Constructor.
- Parameters:
-
data data input clock clock input latch latches HC595 and sends data to LCD
Definition at line 5 of file TriPinLcd.cpp.
Member Function Documentation
void autoscroll | ( | void | ) |
This will 'right justify' text from the cursor.
Definition at line 182 of file TriPinLcd.cpp.
void blink | ( | ) |
Turns on the blinking cursor.
Definition at line 151 of file TriPinLcd.cpp.
void clear | ( | ) |
clears display, set cursor position to zero
Definition at line 99 of file TriPinLcd.cpp.
void createChar | ( | uint8_t | location, |
uint8_t | charmap[] | ||
) |
Allows us to fill the first 8 CGRAM locations with custom characters.
Definition at line 197 of file TriPinLcd.cpp.
void cursor | ( | ) |
Turns the underline cursor on.
Definition at line 139 of file TriPinLcd.cpp.
void display | ( | ) |
Turn the display on.
Definition at line 127 of file TriPinLcd.cpp.
void home | ( | ) |
set cursor position to zero
Definition at line 105 of file TriPinLcd.cpp.
void leftToRight | ( | void | ) |
This is for text that flows Left to Right.
Definition at line 168 of file TriPinLcd.cpp.
void noAutoscroll | ( | void | ) |
This will 'left justify' text from the cursor.
Definition at line 189 of file TriPinLcd.cpp.
void noBlink | ( | ) |
Turns off the blinking cursor.
Definition at line 146 of file TriPinLcd.cpp.
void noCursor | ( | ) |
Turns the underline cursor off.
Definition at line 134 of file TriPinLcd.cpp.
void noDisplay | ( | ) |
Turn the display off.
Definition at line 122 of file TriPinLcd.cpp.
void rightToLeft | ( | void | ) |
This is for text that flows Right to Left.
Definition at line 175 of file TriPinLcd.cpp.
void scrollDisplayLeft | ( | void | ) |
scroll the display to left without changing the RAM
Definition at line 158 of file TriPinLcd.cpp.
void scrollDisplayRight | ( | void | ) |
scroll the display to right without changing the RAM
Definition at line 162 of file TriPinLcd.cpp.
void setCursor | ( | uint8_t | col, |
uint8_t | row | ||
) |
set cursor position to (x=col,y=row); (0,0) is left-top
- Parameters:
-
col X position row Y position
Definition at line 111 of file TriPinLcd.cpp.
Generated on Thu Jul 14 2022 03:30:42 by
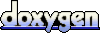