HD44780 compatible 16x2 character LCD driver with HC595 shiftregister
Embed:
(wiki syntax)
Show/hide line numbers
TriPinLcd.h
00001 /** this is for HD44780 compatible 16x2 charachter LCD with HC595 by bitbanging 00002 * by Kazuki Yamamoto, or _K4ZUKI_ 00003 */ 00004 00005 #ifndef __TRIPINLCD_H__ 00006 #define __TRIPINLCD_H__ 00007 00008 #include <inttypes.h> 00009 #include "mbed.h" 00010 #include "ShiftOut.h" 00011 00012 // commands 00013 #define LCD_CLEARDISPLAY 0x01 00014 #define LCD_RETURNHOME 0x02 00015 #define LCD_ENTRYMODESET 0x04 00016 #define LCD_DISPLAYCONTROL 0x08 00017 #define LCD_CURSORSHIFT 0x10 00018 #define LCD_FUNCTIONSET 0x20 00019 #define LCD_SETCGRAMADDR 0x40 00020 #define LCD_SETDDRAMADDR 0x80 00021 00022 // flags for display entry mode 00023 #define LCD_ENTRYRIGHT 0x00 00024 #define LCD_ENTRYLEFT 0x02 00025 #define LCD_ENTRYSHIFTINCREMENT 0x01 00026 #define LCD_ENTRYSHIFTDECREMENT 0x00 00027 00028 // flags for display on/off control 00029 #define LCD_DISPLAYON 0x04 00030 #define LCD_DISPLAYOFF 0x00 00031 #define LCD_CURSORON 0x02 00032 #define LCD_CURSOROFF 0x00 00033 #define LCD_BLINKON 0x01 00034 #define LCD_BLINKOFF 0x00 00035 00036 // flags for display/cursor shift 00037 #define LCD_DISPLAYMOVE 0x08 00038 #define LCD_CURSORMOVE 0x00 00039 #define LCD_MOVERIGHT 0x04 00040 #define LCD_MOVELEFT 0x00 00041 00042 // flags for function set 00043 #define LCD_3WIERMODE 0x20 00044 #define LCD_8BITMODE 0x10 00045 #define LCD_4BITMODE 0x00 00046 #define LCD_2LINE 0x08 00047 #define LCD_1LINE 0x00 00048 #define LCD_5x10DOTS 0x04 00049 #define LCD_5x8DOTS 0x00 00050 00051 //#define SHIFT_RS (0x02) //B00000010 00052 #define SHIFT_RS (0x01) //B00000010 00053 #define SHIFT_EN (0x08) //B00001000 00054 00055 #define DATA_BYTE (1) 00056 #define CMND_BYTE (0) 00057 00058 /** tripinLcd 00059 * mbed library for HD44780 compatible charachter LCD with HC595 by bitbanging 00060 * 00061 * Example: 00062 * @code 00063 #include "mbed.h" 00064 #include "TriPinLcd.h" 00065 00066 Timer t; 00067 TriPinLcd lcd(p5,p7,p6);//clk = p7, data = p5, latch = p6 00068 00069 int main() 00070 { 00071 00072 lcd.begin(16, 2); 00073 lcd.noBlink(); 00074 00075 lcd.printf("LCD3WIRE mbed"); 00076 t.start(); 00077 while(1) { 00078 lcd.setCursor(0, 1); 00079 // lcd.printf("%.0f", t.read()); 00080 lcd.printf("%d", t.read_us()); 00081 } 00082 } 00083 00084 * @endcode 00085 */ 00086 00087 class TriPinLcd : public Stream 00088 { 00089 00090 public: 00091 /** Constructor 00092 * @param data data input 00093 * @param clock clock input 00094 * @param latch latches HC595 and sends data to LCD 00095 */ 00096 TriPinLcd(PinName data, PinName clock, PinName latch); 00097 00098 void begin(uint8_t cols, uint8_t rows, uint8_t dotsize = LCD_5x8DOTS); 00099 00100 /** 00101 clears display, set cursor position to zero 00102 */ 00103 void clear(); 00104 00105 /** 00106 set cursor position to zero 00107 */ 00108 void home(); 00109 /** 00110 Turn the display off 00111 */ 00112 void noDisplay(); 00113 /** 00114 Turn the display on 00115 */ 00116 void display(); 00117 /** 00118 Turns off the blinking cursor 00119 */ 00120 void noBlink(); 00121 /** 00122 Turns on the blinking cursor 00123 */ 00124 void blink(); 00125 /** 00126 Turns the underline cursor off 00127 */ 00128 void noCursor(); 00129 /** 00130 Turns the underline cursor on 00131 */ 00132 void cursor(); 00133 /** 00134 scroll the display to left without changing the RAM 00135 */ 00136 void scrollDisplayLeft(); 00137 /** 00138 scroll the display to right without changing the RAM 00139 */ 00140 void scrollDisplayRight(); 00141 /** 00142 This is for text that flows Left to Right 00143 */ 00144 void leftToRight(); 00145 /** 00146 This is for text that flows Right to Left 00147 */ 00148 void rightToLeft(); 00149 /** 00150 This will 'right justify' text from the cursor 00151 */ 00152 void autoscroll(); 00153 /** 00154 This will 'left justify' text from the cursor 00155 */ 00156 void noAutoscroll(); 00157 00158 /** 00159 Allows us to fill the first 8 CGRAM locations 00160 with custom characters 00161 */ 00162 void createChar(uint8_t, uint8_t[]); 00163 /** 00164 set cursor position to (x=col,y=row); (0,0) is left-top 00165 @param col X position 00166 @param row Y position 00167 */ 00168 void setCursor(uint8_t, uint8_t); 00169 00170 00171 private: 00172 ShiftOut::ShiftOut _lcd; 00173 void _write(uint8_t); 00174 void _command(uint8_t); 00175 00176 virtual int _putc(int value); 00177 virtual int _getc(); 00178 00179 void _send(uint8_t, uint8_t); 00180 void _write4bits(uint8_t); 00181 00182 uint8_t _displayfunction; 00183 uint8_t _displaycontrol; 00184 uint8_t _displaymode; 00185 00186 uint8_t _numlines,_currline; 00187 00188 }; 00189 00190 #endif
Generated on Thu Jul 14 2022 03:30:42 by
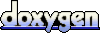