HD44780 compatible 16x2 character LCD driver with HC595 shiftregister
Embed:
(wiki syntax)
Show/hide line numbers
TriPinLcd.cpp
00001 00002 #include "mbed.h" 00003 #include "TriPinLcd.h" 00004 00005 TriPinLcd::TriPinLcd(PinName data, PinName clock, PinName latch): _lcd(data,clock,latch,8) 00006 { 00007 00008 _displayfunction = LCD_3WIERMODE | LCD_1LINE | LCD_5x8DOTS; 00009 00010 begin(16, 1); 00011 setCursor(0,0); 00012 00013 } 00014 /* 00015 TriPinLcd(PinName data, PinName clock, PinName latch); 00016 00017 void init(); 00018 00019 void begin(uint8_t cols, uint8_t rows, uint8_t charsize = LCD_5x8DOTS); 00020 void clear(); 00021 void home(); 00022 00023 void noDisplay(); 00024 void display(); 00025 void noBlink(); 00026 void blink(); 00027 void noCursor(); 00028 void cursor(); 00029 void scrollDisplayLeft(); 00030 void scrollDisplayRight(); 00031 void leftToRight(); 00032 void rightToLeft(); 00033 void autoscroll(); 00034 void noAutoscroll(); 00035 00036 void createChar(uint8_t, uint8_t[]); 00037 void setCursor(uint8_t, uint8_t); 00038 00039 virtual int _putc(int value); 00040 virtual int _getc(); 00041 00042 */ 00043 00044 void TriPinLcd::begin(uint8_t cols, uint8_t lines, uint8_t dotsize) 00045 { 00046 if (lines > 1) { 00047 _displayfunction |= LCD_2LINE; 00048 } 00049 _numlines = lines; 00050 _currline = 0; 00051 00052 // for some 1 line displays you can select a 10 pixel high font 00053 if ((dotsize != 0) && (lines == 1)) { 00054 _displayfunction |= LCD_5x10DOTS; 00055 } 00056 00057 // SEE PAGE 45/46 FOR INITIALIZATION SPECIFICATION! 00058 // according to datasheet, we need at least 40ms after power rises above 2.7V 00059 // before sending commands. Arduino can turn on way befer 4.5V so we'll wait 50 00060 wait_us(40000); 00061 00062 //put the LCD into 4 bit or 8 bit mode 00063 if (! (_displayfunction & LCD_8BITMODE)) { 00064 // this is according to the hitachi HD44780 datasheet 00065 // figure 24, pg 46 00066 00067 // we start in 8bit mode, try to set 4 bit mode 00068 _write4bits(0x03); 00069 wait_us(4500); // wait min 4.1ms 00070 00071 // second try 00072 _write4bits(0x03); 00073 wait_us(4500); // wait min 4.1ms 00074 00075 // third go! 00076 _write4bits(0x03); 00077 wait_us(150); 00078 00079 // finally, set to 4-bit interface 00080 _write4bits(0x02); 00081 } 00082 _command(LCD_FUNCTIONSET | _displayfunction); 00083 00084 // turn the display on with no cursor or blinking default 00085 _displaycontrol = LCD_DISPLAYON | LCD_CURSOROFF | LCD_BLINKOFF; 00086 display(); 00087 00088 // clear it off 00089 clear(); 00090 00091 // Initialize to default text direction (for romance languages) 00092 _displaymode = LCD_ENTRYLEFT | LCD_ENTRYSHIFTDECREMENT; 00093 // set the entry mode 00094 _command(LCD_ENTRYMODESET | _displaymode); 00095 wait_us(150); 00096 } 00097 00098 // ********** high level commands, for the user! 00099 void TriPinLcd::clear() 00100 { 00101 _command(LCD_CLEARDISPLAY); // clear display, set cursor position to zero 00102 wait_ms(30); // this command takes a long time! 00103 } 00104 00105 void TriPinLcd::home() 00106 { 00107 _command(LCD_RETURNHOME); // set cursor position to zero 00108 wait_ms(30); // this command takes a long time! 00109 } 00110 00111 void TriPinLcd::setCursor(uint8_t col, uint8_t row) 00112 { 00113 int row_offsets[] = { 0x00, 0x40, 0x14, 0x54 }; 00114 if ( row >= _numlines ) { 00115 row = _numlines-1; // we count rows starting w/0 00116 } 00117 00118 _command(LCD_SETDDRAMADDR | (col + row_offsets[row])); 00119 } 00120 00121 // Turn the display on/off (quickly) 00122 void TriPinLcd::noDisplay() 00123 { 00124 _displaycontrol &= ~LCD_DISPLAYON; 00125 _command(LCD_DISPLAYCONTROL | _displaycontrol); 00126 } 00127 void TriPinLcd::display() 00128 { 00129 _displaycontrol |= LCD_DISPLAYON; 00130 _command(LCD_DISPLAYCONTROL | _displaycontrol); 00131 } 00132 00133 // Turns the underline cursor on/off 00134 void TriPinLcd::noCursor() 00135 { 00136 _displaycontrol &= ~LCD_CURSORON; 00137 _command(LCD_DISPLAYCONTROL | _displaycontrol); 00138 } 00139 void TriPinLcd::cursor() 00140 { 00141 _displaycontrol |= LCD_CURSORON; 00142 _command(LCD_DISPLAYCONTROL | _displaycontrol); 00143 } 00144 00145 // Turn on and off the blinking cursor 00146 void TriPinLcd::noBlink() 00147 { 00148 _displaycontrol &= ~LCD_BLINKON; 00149 _command(LCD_DISPLAYCONTROL | _displaycontrol); 00150 } 00151 void TriPinLcd::blink() 00152 { 00153 _displaycontrol |= LCD_BLINKON; 00154 _command(LCD_DISPLAYCONTROL | _displaycontrol); 00155 } 00156 00157 // These commands scroll the display without changing the RAM 00158 void TriPinLcd::scrollDisplayLeft(void) 00159 { 00160 _command(LCD_CURSORSHIFT | LCD_DISPLAYMOVE | LCD_MOVELEFT); 00161 } 00162 void TriPinLcd::scrollDisplayRight(void) 00163 { 00164 _command(LCD_CURSORSHIFT | LCD_DISPLAYMOVE | LCD_MOVERIGHT); 00165 } 00166 00167 // This is for text that flows Left to Right 00168 void TriPinLcd::leftToRight(void) 00169 { 00170 _displaymode |= LCD_ENTRYLEFT; 00171 _command(LCD_ENTRYMODESET | _displaymode); 00172 } 00173 00174 // This is for text that flows Right to Left 00175 void TriPinLcd::rightToLeft(void) 00176 { 00177 _displaymode &= ~LCD_ENTRYLEFT; 00178 _command(LCD_ENTRYMODESET | _displaymode); 00179 } 00180 00181 // This will 'right justify' text from the cursor 00182 void TriPinLcd::autoscroll(void) 00183 { 00184 _displaymode |= LCD_ENTRYSHIFTINCREMENT; 00185 _command(LCD_ENTRYMODESET | _displaymode); 00186 } 00187 00188 // This will 'left justify' text from the cursor 00189 void TriPinLcd::noAutoscroll(void) 00190 { 00191 _displaymode &= ~LCD_ENTRYSHIFTINCREMENT; 00192 _command(LCD_ENTRYMODESET | _displaymode); 00193 } 00194 00195 // Allows us to fill the first 8 CGRAM locations 00196 // with custom characters 00197 void TriPinLcd::createChar(uint8_t location, uint8_t charmap[]) 00198 { 00199 location &= 0x07; // we only have 8 locations 0-7 00200 _command(LCD_SETCGRAMADDR | (location << 3)); 00201 for (int i=0; i<8; i++) { 00202 _write(charmap[i]); 00203 } 00204 } 00205 00206 // *********** mid level commands, for sending data/cmds 00207 00208 inline void TriPinLcd::_command(uint8_t value) 00209 { 00210 _send(value, CMND_BYTE); 00211 } 00212 00213 inline void TriPinLcd::_write(uint8_t value) 00214 { 00215 _send(value, DATA_BYTE); 00216 } 00217 00218 int TriPinLcd::_putc(int value) 00219 { 00220 _send(value, DATA_BYTE); 00221 wait_us(1000); 00222 return value; 00223 } 00224 00225 int TriPinLcd::_getc() 00226 { 00227 return -1; 00228 } 00229 00230 00231 void TriPinLcd::_send(uint8_t value, uint8_t mode) 00232 { 00233 00234 int data = value & 0xf0; //send the 4 MSbits (from 8) 00235 00236 data |= (mode << SHIFT_RS); // set DI mode 00237 data &= ~SHIFT_EN; // set Enable LOW 00238 _lcd.write(data); 00239 data |= SHIFT_EN; // Set Enable HIGH 00240 _lcd.write(data); 00241 data &= ~SHIFT_EN; // set Enable LOW 00242 _lcd.write(data); 00243 00244 data = value << 4; // send the 4 LSbits (from 8) 00245 00246 data |= (mode << SHIFT_RS); // set DI mode 00247 data &= ~SHIFT_EN; // set Enable LOW 00248 _lcd.write(data); 00249 data |= SHIFT_EN; // Set Enable HIGH 00250 _lcd.write(data); 00251 data &= ~SHIFT_EN; // set Enable LOW 00252 _lcd.write(data); 00253 00254 } 00255 00256 void TriPinLcd::_write4bits(uint8_t value) 00257 { 00258 int data=0; //= value & 0x0f; 00259 00260 data = value << 4; //send the first 4 databits (from 8) 00261 00262 data &= ~SHIFT_EN; // set Enable LOW 00263 _lcd.write(data); 00264 data |= SHIFT_EN; // Set Enable HIGH 00265 _lcd.write(data); 00266 data &= ~SHIFT_EN; // set Enable LOW 00267 _lcd.write(data); 00268 00269 }
Generated on Thu Jul 14 2022 03:30:42 by
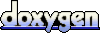