
opencv on mbed
OpenGL support
[High-level GUI]
Functions | |
CV_EXPORTS void | imshow (const String &winname, const ogl::Texture2D &tex) |
Displays OpenGL 2D texture in the specified window. | |
CV_EXPORTS void | setOpenGlDrawCallback (const String &winname, OpenGlDrawCallback onOpenGlDraw, void *userdata=0) |
Sets a callback function to be called to draw on top of displayed image. | |
CV_EXPORTS void | setOpenGlContext (const String &winname) |
Sets the specified window as current OpenGL context. | |
CV_EXPORTS void | updateWindow (const String &winname) |
Force window to redraw its context and call draw callback ( See cv::setOpenGlDrawCallback ). |
Function Documentation
CV_EXPORTS void cv::imshow | ( | const String & | winname, |
const ogl::Texture2D & | tex | ||
) |
Displays OpenGL 2D texture in the specified window.
- Parameters:
-
winname Name of the window. tex OpenGL 2D texture data.
CV_EXPORTS void cv::setOpenGlContext | ( | const String & | winname ) |
Sets the specified window as current OpenGL context.
- Parameters:
-
winname Name of the window.
CV_EXPORTS void cv::setOpenGlDrawCallback | ( | const String & | winname, |
OpenGlDrawCallback | onOpenGlDraw, | ||
void * | userdata = 0 |
||
) |
Sets a callback function to be called to draw on top of displayed image.
The function setOpenGlDrawCallback can be used to draw 3D data on the window. See the example of callback function below:
void on_opengl(void* param) { glLoadIdentity(); glTranslated(0.0, 0.0, -1.0); glRotatef( 55, 1, 0, 0 ); glRotatef( 45, 0, 1, 0 ); glRotatef( 0, 0, 0, 1 ); static const int coords[6][4][3] = { { { +1, -1, -1 }, { -1, -1, -1 }, { -1, +1, -1 }, { +1, +1, -1 } }, { { +1, +1, -1 }, { -1, +1, -1 }, { -1, +1, +1 }, { +1, +1, +1 } }, { { +1, -1, +1 }, { +1, -1, -1 }, { +1, +1, -1 }, { +1, +1, +1 } }, { { -1, -1, -1 }, { -1, -1, +1 }, { -1, +1, +1 }, { -1, +1, -1 } }, { { +1, -1, +1 }, { -1, -1, +1 }, { -1, -1, -1 }, { +1, -1, -1 } }, { { -1, -1, +1 }, { +1, -1, +1 }, { +1, +1, +1 }, { -1, +1, +1 } } }; for (int i = 0; i < 6; ++i) { glColor3ub( i*20, 100+i*10, i*42 ); glBegin(GL_QUADS); for (int j = 0; j < 4; ++j) { glVertex3d(0.2 * coords[i][j][0], 0.2 * coords[i][j][1], 0.2 * coords[i][j][2]); } glEnd(); } }
- Parameters:
-
winname Name of the window. onOpenGlDraw Pointer to the function to be called every frame. This function should be prototyped as void Foo(void\*) . userdata Pointer passed to the callback function.(__Optional__)
CV_EXPORTS void cv::updateWindow | ( | const String & | winname ) |
Force window to redraw its context and call draw callback ( See cv::setOpenGlDrawCallback ).
- Parameters:
-
winname Name of the window.
Generated on Tue Jul 12 2022 16:42:41 by
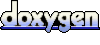