
opencv on mbed
ANN_MLP Class Reference
[Machine Learning]
Artificial Neural Networks - Multi-Layer Perceptrons. More...
#include <ml.hpp>
Inherits cv::ml::StatModel.
Public Types | |
enum | TrainingMethods { BACKPROP = 0, RPROP = 1 } |
Available training methods. More... | |
enum | ActivationFunctions { IDENTITY = 0, SIGMOID_SYM = 1, GAUSSIAN = 2 } |
possible activation functions More... | |
enum | TrainFlags { UPDATE_WEIGHTS = 1, NO_INPUT_SCALE = 2, NO_OUTPUT_SCALE = 4 } |
Train options. More... | |
enum | Flags { , RAW_OUTPUT = 1 } |
Predict options. More... | |
Public Member Functions | |
virtual CV_WRAP void | setTrainMethod (int method, double param1=0, double param2=0)=0 |
Sets training method and common parameters. | |
virtual CV_WRAP int | getTrainMethod () const =0 |
Returns current training method. | |
virtual CV_WRAP void | setActivationFunction (int type, double param1=0, double param2=0)=0 |
Initialize the activation function for each neuron. | |
virtual CV_WRAP void | setLayerSizes (InputArray _layer_sizes)=0 |
Integer vector specifying the number of neurons in each layer including the input and output layers. | |
virtual CV_WRAP cv::Mat | getLayerSizes () const =0 |
Integer vector specifying the number of neurons in each layer including the input and output layers. | |
virtual CV_WRAP TermCriteria | getTermCriteria () const =0 |
Termination criteria of the training algorithm. | |
virtual CV_WRAP void | setTermCriteria (TermCriteria val)=0 |
Termination criteria of the training algorithm. | |
virtual CV_WRAP double | getBackpropWeightScale () const =0 |
BPROP: Strength of the weight gradient term. | |
virtual CV_WRAP void | setBackpropWeightScale (double val)=0 |
BPROP: Strength of the weight gradient term. | |
virtual CV_WRAP double | getBackpropMomentumScale () const =0 |
BPROP: Strength of the momentum term (the difference between weights on the 2 previous iterations). | |
virtual CV_WRAP void | setBackpropMomentumScale (double val)=0 |
BPROP: Strength of the momentum term (the difference between weights on the 2 previous iterations). | |
virtual CV_WRAP double | getRpropDW0 () const =0 |
RPROP: Initial value ![]() ![]() | |
virtual CV_WRAP void | setRpropDW0 (double val)=0 |
RPROP: Initial value | |
virtual CV_WRAP double | getRpropDWPlus () const =0 |
RPROP: Increase factor ![]() | |
virtual CV_WRAP void | setRpropDWPlus (double val)=0 |
RPROP: Increase factor | |
virtual CV_WRAP double | getRpropDWMinus () const =0 |
RPROP: Decrease factor ![]() | |
virtual CV_WRAP void | setRpropDWMinus (double val)=0 |
RPROP: Decrease factor | |
virtual CV_WRAP double | getRpropDWMin () const =0 |
RPROP: Update-values lower limit ![]() | |
virtual CV_WRAP void | setRpropDWMin (double val)=0 |
RPROP: Update-values lower limit | |
virtual CV_WRAP double | getRpropDWMax () const =0 |
RPROP: Update-values upper limit ![]() | |
virtual CV_WRAP void | setRpropDWMax (double val)=0 |
RPROP: Update-values upper limit | |
virtual CV_WRAP int | getVarCount () const =0 |
Returns the number of variables in training samples. | |
virtual CV_WRAP bool | empty () const |
Returns true if the Algorithm is empty (e.g. | |
virtual CV_WRAP bool | isTrained () const =0 |
Returns true if the model is trained. | |
virtual CV_WRAP bool | isClassifier () const =0 |
Returns true if the model is classifier. | |
virtual CV_WRAP bool | train (const Ptr< TrainData > &trainData, int flags=0) |
Trains the statistical model. | |
virtual CV_WRAP bool | train (InputArray samples, int layout, InputArray responses) |
Trains the statistical model. | |
virtual CV_WRAP float | calcError (const Ptr< TrainData > &data, bool test, OutputArray resp) const |
Computes error on the training or test dataset. | |
virtual CV_WRAP float | predict (InputArray samples, OutputArray results=noArray(), int flags=0) const =0 |
Predicts response(s) for the provided sample(s) | |
virtual CV_WRAP void | clear () |
Clears the algorithm state. | |
virtual void | write (FileStorage &fs) const |
Stores algorithm parameters in a file storage. | |
virtual void | read (const FileNode &fn) |
Reads algorithm parameters from a file storage. | |
virtual CV_WRAP void | save (const String &filename) const |
Saves the algorithm to a file. | |
virtual CV_WRAP String | getDefaultName () const |
Returns the algorithm string identifier. | |
Static Public Member Functions | |
static CV_WRAP Ptr< ANN_MLP > | create () |
Creates empty model. | |
template<typename _Tp > | |
static Ptr< _Tp > | train (const Ptr< TrainData > &data, int flags=0) |
Create and train model with default parameters. | |
template<typename _Tp > | |
static Ptr< _Tp > | read (const FileNode &fn) |
Reads algorithm from the file node. | |
template<typename _Tp > | |
static Ptr< _Tp > | load (const String &filename, const String &objname=String()) |
Loads algorithm from the file. | |
template<typename _Tp > | |
static Ptr< _Tp > | loadFromString (const String &strModel, const String &objname=String()) |
Loads algorithm from a String. |
Detailed Description
Artificial Neural Networks - Multi-Layer Perceptrons.
Unlike many other models in ML that are constructed and trained at once, in the MLP model these steps are separated. First, a network with the specified topology is created using the non-default constructor or the method ANN_MLP::create. All the weights are set to zeros. Then, the network is trained using a set of input and output vectors. The training procedure can be repeated more than once, that is, the weights can be adjusted based on the new training data.
Additional flags for StatModel::train are available: ANN_MLP::TrainFlags.
- See also:
- ml_intro_ann
Definition at line 1254 of file ml.hpp.
Member Enumeration Documentation
enum ActivationFunctions |
possible activation functions
- Enumerator:
enum Flags [inherited] |
enum TrainFlags |
Train options.
- Enumerator:
enum TrainingMethods |
Member Function Documentation
virtual CV_WRAP float calcError | ( | const Ptr< TrainData > & | data, |
bool | test, | ||
OutputArray | resp | ||
) | const [virtual, inherited] |
Computes error on the training or test dataset.
- Parameters:
-
data the training data test if true, the error is computed over the test subset of the data, otherwise it's computed over the training subset of the data. Please note that if you loaded a completely different dataset to evaluate already trained classifier, you will probably want not to set the test subset at all with TrainData::setTrainTestSplitRatio and specify test=false, so that the error is computed for the whole new set. Yes, this sounds a bit confusing. resp the optional output responses.
The method uses StatModel::predict to compute the error. For regression models the error is computed as RMS, for classifiers - as a percent of missclassified samples (0-100%).
virtual CV_WRAP void clear | ( | ) | [virtual, inherited] |
Clears the algorithm state.
Reimplemented in DescriptorMatcher, and FlannBasedMatcher.
Creates empty model.
Use StatModel::train to train the model, Algorithm::load<ANN_MLP>(filename) to load the pre-trained model. Note that the train method has optional flags: ANN_MLP::TrainFlags.
virtual CV_WRAP bool empty | ( | ) | const [virtual, inherited] |
virtual CV_WRAP double getBackpropMomentumScale | ( | ) | const [pure virtual] |
BPROP: Strength of the momentum term (the difference between weights on the 2 previous iterations).
This parameter provides some inertia to smooth the random fluctuations of the weights. It can vary from 0 (the feature is disabled) to 1 and beyond. The value 0.1 or so is good enough. Default value is 0.1.
- See also:
- setBackpropMomentumScale
virtual CV_WRAP double getBackpropWeightScale | ( | ) | const [pure virtual] |
BPROP: Strength of the weight gradient term.
The recommended value is about 0.1. Default value is 0.1.
- See also:
- setBackpropWeightScale
virtual CV_WRAP String getDefaultName | ( | ) | const [virtual, inherited] |
Returns the algorithm string identifier.
This string is used as top level xml/yml node tag when the object is saved to a file or string.
virtual CV_WRAP cv::Mat getLayerSizes | ( | ) | const [pure virtual] |
Integer vector specifying the number of neurons in each layer including the input and output layers.
The very first element specifies the number of elements in the input layer. The last element - number of elements in the output layer.
- See also:
- setLayerSizes
virtual CV_WRAP double getRpropDW0 | ( | ) | const [pure virtual] |
virtual CV_WRAP double getRpropDWMax | ( | ) | const [pure virtual] |
virtual CV_WRAP double getRpropDWMin | ( | ) | const [pure virtual] |
RPROP: Update-values lower limit .
It must be positive. Default value is FLT_EPSILON.
- See also:
- setRpropDWMin
virtual CV_WRAP double getRpropDWMinus | ( | ) | const [pure virtual] |
virtual CV_WRAP double getRpropDWPlus | ( | ) | const [pure virtual] |
virtual CV_WRAP TermCriteria getTermCriteria | ( | ) | const [pure virtual] |
Termination criteria of the training algorithm.
You can specify the maximum number of iterations (maxCount) and/or how much the error could change between the iterations to make the algorithm continue (epsilon). Default value is TermCriteria(TermCriteria::MAX_ITER + TermCriteria::EPS, 1000, 0.01).
- See also:
- setTermCriteria
virtual CV_WRAP int getTrainMethod | ( | ) | const [pure virtual] |
Returns current training method.
virtual CV_WRAP int getVarCount | ( | ) | const [pure virtual, inherited] |
Returns the number of variables in training samples.
virtual CV_WRAP bool isClassifier | ( | ) | const [pure virtual, inherited] |
Returns true if the model is classifier.
virtual CV_WRAP bool isTrained | ( | ) | const [pure virtual, inherited] |
Returns true if the model is trained.
static Ptr<_Tp> load | ( | const String & | filename, |
const String & | objname = String() |
||
) | [static, inherited] |
Loads algorithm from the file.
- Parameters:
-
filename Name of the file to read. objname The optional name of the node to read (if empty, the first top-level node will be used)
This is static template method of Algorithm. It's usage is following (in the case of SVM):
Ptr<SVM> svm = Algorithm::load<SVM>("my_svm_model.xml");
In order to make this method work, the derived class must overwrite Algorithm::read(const FileNode& fn).
static Ptr<_Tp> loadFromString | ( | const String & | strModel, |
const String & | objname = String() |
||
) | [static, inherited] |
Loads algorithm from a String.
- Parameters:
-
strModel The string variable containing the model you want to load. objname The optional name of the node to read (if empty, the first top-level node will be used)
This is static template method of Algorithm. It's usage is following (in the case of SVM):
Ptr<SVM> svm = Algorithm::loadFromString<SVM>(myStringModel);
virtual CV_WRAP float predict | ( | InputArray | samples, |
OutputArray | results = noArray() , |
||
int | flags = 0 |
||
) | const [pure virtual, inherited] |
Predicts response(s) for the provided sample(s)
- Parameters:
-
samples The input samples, floating-point matrix results The optional output matrix of results. flags The optional flags, model-dependent. See cv::ml::StatModel::Flags.
Implemented in LogisticRegression.
virtual void read | ( | const FileNode & | fn ) | [virtual, inherited] |
Reads algorithm parameters from a file storage.
Reimplemented in DescriptorMatcher, and FlannBasedMatcher.
Reads algorithm from the file node.
This is static template method of Algorithm. It's usage is following (in the case of SVM):
Ptr<SVM> svm = Algorithm::read<SVM>(fn);
In order to make this method work, the derived class must overwrite Algorithm::read(const FileNode& fn) and also have static create() method without parameters (or with all the optional parameters)
Reimplemented in DescriptorMatcher, and FlannBasedMatcher.
virtual CV_WRAP void save | ( | const String & | filename ) | const [virtual, inherited] |
Saves the algorithm to a file.
In order to make this method work, the derived class must implement Algorithm::write(FileStorage& fs).
virtual CV_WRAP void setActivationFunction | ( | int | type, |
double | param1 = 0 , |
||
double | param2 = 0 |
||
) | [pure virtual] |
Initialize the activation function for each neuron.
Currently the default and the only fully supported activation function is ANN_MLP::SIGMOID_SYM.
- Parameters:
-
type The type of activation function. See ANN_MLP::ActivationFunctions. param1 The first parameter of the activation function, . Default value is 0.
param2 The second parameter of the activation function, . Default value is 0.
virtual CV_WRAP void setBackpropMomentumScale | ( | double | val ) | [pure virtual] |
BPROP: Strength of the momentum term (the difference between weights on the 2 previous iterations).
- See also:
- getBackpropMomentumScale
virtual CV_WRAP void setBackpropWeightScale | ( | double | val ) | [pure virtual] |
BPROP: Strength of the weight gradient term.
- See also:
- getBackpropWeightScale
virtual CV_WRAP void setLayerSizes | ( | InputArray | _layer_sizes ) | [pure virtual] |
Integer vector specifying the number of neurons in each layer including the input and output layers.
The very first element specifies the number of elements in the input layer. The last element - number of elements in the output layer. Default value is empty Mat.
- See also:
- getLayerSizes
virtual CV_WRAP void setRpropDW0 | ( | double | val ) | [pure virtual] |
RPROP: Initial value of update-values
.
- See also:
- getRpropDW0
virtual CV_WRAP void setRpropDWMax | ( | double | val ) | [pure virtual] |
RPROP: Update-values upper limit .
- See also:
- getRpropDWMax
virtual CV_WRAP void setRpropDWMin | ( | double | val ) | [pure virtual] |
RPROP: Update-values lower limit .
- See also:
- getRpropDWMin
virtual CV_WRAP void setRpropDWMinus | ( | double | val ) | [pure virtual] |
RPROP: Decrease factor .
- See also:
- getRpropDWMinus
virtual CV_WRAP void setRpropDWPlus | ( | double | val ) | [pure virtual] |
RPROP: Increase factor .
- See also:
- getRpropDWPlus
virtual CV_WRAP void setTermCriteria | ( | TermCriteria | val ) | [pure virtual] |
Termination criteria of the training algorithm.
- See also:
- getTermCriteria
virtual CV_WRAP void setTrainMethod | ( | int | method, |
double | param1 = 0 , |
||
double | param2 = 0 |
||
) | [pure virtual] |
Sets training method and common parameters.
- Parameters:
-
method Default value is ANN_MLP::RPROP. See ANN_MLP::TrainingMethods. param1 passed to setRpropDW0 for ANN_MLP::RPROP and to setBackpropWeightScale for ANN_MLP::BACKPROP param2 passed to setRpropDWMin for ANN_MLP::RPROP and to setBackpropMomentumScale for ANN_MLP::BACKPROP.
virtual CV_WRAP bool train | ( | const Ptr< TrainData > & | trainData, |
int | flags = 0 |
||
) | [virtual, inherited] |
Trains the statistical model.
- Parameters:
-
trainData training data that can be loaded from file using TrainData::loadFromCSV or created with TrainData::create. flags optional flags, depending on the model. Some of the models can be updated with the new training samples, not completely overwritten (such as NormalBayesClassifier or ANN_MLP).
virtual CV_WRAP bool train | ( | InputArray | samples, |
int | layout, | ||
InputArray | responses | ||
) | [virtual, inherited] |
Trains the statistical model.
- Parameters:
-
samples training samples layout See ml::SampleTypes. responses vector of responses associated with the training samples.
virtual void write | ( | FileStorage & | fs ) | const [virtual, inherited] |
Stores algorithm parameters in a file storage.
Reimplemented in DescriptorMatcher, and FlannBasedMatcher.
Generated on Tue Jul 12 2022 16:42:45 by
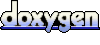