
opencv on mbed
SimpleBlobDetector Class Reference
[Feature Detection and Description]
Class for extracting blobs from an image. More...
#include <features2d.hpp>
Inherits cv::Feature2D.
Public Member Functions | |
virtual CV_WRAP void | detect (InputArray image, CV_OUT std::vector< KeyPoint > &keypoints, InputArray mask=noArray()) |
Detects keypoints in an image (first variant) or image set (second variant). | |
virtual void | detect (InputArrayOfArrays images, std::vector< std::vector< KeyPoint > > &keypoints, InputArrayOfArrays masks=noArray()) |
This is an overloaded member function, provided for convenience. It differs from the above function only in what argument(s) it accepts. | |
virtual CV_WRAP void | compute (InputArray image, CV_OUT CV_IN_OUT std::vector< KeyPoint > &keypoints, OutputArray descriptors) |
Computes the descriptors for a set of keypoints detected in an image (first variant) or image set (second variant). | |
virtual void | compute (InputArrayOfArrays images, std::vector< std::vector< KeyPoint > > &keypoints, OutputArrayOfArrays descriptors) |
This is an overloaded member function, provided for convenience. It differs from the above function only in what argument(s) it accepts. | |
virtual CV_WRAP void | detectAndCompute (InputArray image, InputArray mask, CV_OUT std::vector< KeyPoint > &keypoints, OutputArray descriptors, bool useProvidedKeypoints=false) |
Detects keypoints and computes the descriptors. | |
virtual CV_WRAP bool | empty () const |
Return true if detector object is empty. | |
virtual CV_WRAP void | clear () |
Clears the algorithm state. | |
virtual void | write (FileStorage &fs) const |
Stores algorithm parameters in a file storage. | |
virtual void | read (const FileNode &fn) |
Reads algorithm parameters from a file storage. | |
virtual CV_WRAP void | save (const String &filename) const |
Saves the algorithm to a file. | |
virtual CV_WRAP String | getDefaultName () const |
Returns the algorithm string identifier. | |
Static Public Member Functions | |
template<typename _Tp > | |
static Ptr< _Tp > | read (const FileNode &fn) |
Reads algorithm from the file node. | |
template<typename _Tp > | |
static Ptr< _Tp > | load (const String &filename, const String &objname=String()) |
Loads algorithm from the file. | |
template<typename _Tp > | |
static Ptr< _Tp > | loadFromString (const String &strModel, const String &objname=String()) |
Loads algorithm from a String. |
Detailed Description
Class for extracting blobs from an image.
:
The class implements a simple algorithm for extracting blobs from an image:
1. Convert the source image to binary images by applying thresholding with several thresholds from minThreshold (inclusive) to maxThreshold (exclusive) with distance thresholdStep between neighboring thresholds. 2. Extract connected components from every binary image by findContours and calculate their centers. 3. Group centers from several binary images by their coordinates. Close centers form one group that corresponds to one blob, which is controlled by the minDistBetweenBlobs parameter. 4. From the groups, estimate final centers of blobs and their radiuses and return as locations and sizes of keypoints.
This class performs several filtrations of returned blobs. You should set filterBy\* to true/false to turn on/off corresponding filtration. Available filtrations:
- **By color**. This filter compares the intensity of a binary image at the center of a blob to blobColor. If they differ, the blob is filtered out. Use blobColor = 0 to extract dark blobs and blobColor = 255 to extract light blobs.
- **By area**. Extracted blobs have an area between minArea (inclusive) and maxArea (exclusive).
- **By circularity**. Extracted blobs have circularity (
) between minCircularity (inclusive) and maxCircularity (exclusive).
- **By ratio of the minimum inertia to maximum inertia**. Extracted blobs have this ratio between minInertiaRatio (inclusive) and maxInertiaRatio (exclusive).
- **By convexity**. Extracted blobs have convexity (area / area of blob convex hull) between minConvexity (inclusive) and maxConvexity (exclusive).
Default values of parameters are tuned to extract dark circular blobs.
Definition at line 545 of file features2d.hpp.
Member Function Documentation
virtual CV_WRAP void clear | ( | ) | [virtual, inherited] |
Clears the algorithm state.
Reimplemented in DescriptorMatcher, and FlannBasedMatcher.
virtual CV_WRAP void compute | ( | InputArray | image, |
CV_OUT CV_IN_OUT std::vector< KeyPoint > & | keypoints, | ||
OutputArray | descriptors | ||
) | [virtual, inherited] |
Computes the descriptors for a set of keypoints detected in an image (first variant) or image set (second variant).
- Parameters:
-
image Image. keypoints Input collection of keypoints. Keypoints for which a descriptor cannot be computed are removed. Sometimes new keypoints can be added, for example: SIFT duplicates keypoint with several dominant orientations (for each orientation). descriptors Computed descriptors. In the second variant of the method descriptors[i] are descriptors computed for a keypoints[i]. Row j is the keypoints (or keypoints[i]) is the descriptor for keypoint j-th keypoint.
virtual void compute | ( | InputArrayOfArrays | images, |
std::vector< std::vector< KeyPoint > > & | keypoints, | ||
OutputArrayOfArrays | descriptors | ||
) | [virtual, inherited] |
This is an overloaded member function, provided for convenience. It differs from the above function only in what argument(s) it accepts.
- Parameters:
-
images Image set. keypoints Input collection of keypoints. Keypoints for which a descriptor cannot be computed are removed. Sometimes new keypoints can be added, for example: SIFT duplicates keypoint with several dominant orientations (for each orientation). descriptors Computed descriptors. In the second variant of the method descriptors[i] are descriptors computed for a keypoints[i]. Row j is the keypoints (or keypoints[i]) is the descriptor for keypoint j-th keypoint.
virtual CV_WRAP void detect | ( | InputArray | image, |
CV_OUT std::vector< KeyPoint > & | keypoints, | ||
InputArray | mask = noArray() |
||
) | [virtual, inherited] |
Detects keypoints in an image (first variant) or image set (second variant).
- Parameters:
-
image Image. keypoints The detected keypoints. In the second variant of the method keypoints[i] is a set of keypoints detected in images[i] . mask Mask specifying where to look for keypoints (optional). It must be a 8-bit integer matrix with non-zero values in the region of interest.
virtual void detect | ( | InputArrayOfArrays | images, |
std::vector< std::vector< KeyPoint > > & | keypoints, | ||
InputArrayOfArrays | masks = noArray() |
||
) | [virtual, inherited] |
This is an overloaded member function, provided for convenience. It differs from the above function only in what argument(s) it accepts.
- Parameters:
-
images Image set. keypoints The detected keypoints. In the second variant of the method keypoints[i] is a set of keypoints detected in images[i] . masks Masks for each input image specifying where to look for keypoints (optional). masks[i] is a mask for images[i].
virtual CV_WRAP void detectAndCompute | ( | InputArray | image, |
InputArray | mask, | ||
CV_OUT std::vector< KeyPoint > & | keypoints, | ||
OutputArray | descriptors, | ||
bool | useProvidedKeypoints = false |
||
) | [virtual, inherited] |
Detects keypoints and computes the descriptors.
virtual CV_WRAP bool empty | ( | ) | const [virtual, inherited] |
Return true if detector object is empty.
Reimplemented from Algorithm.
virtual CV_WRAP String getDefaultName | ( | ) | const [virtual, inherited] |
Returns the algorithm string identifier.
This string is used as top level xml/yml node tag when the object is saved to a file or string.
static Ptr<_Tp> load | ( | const String & | filename, |
const String & | objname = String() |
||
) | [static, inherited] |
Loads algorithm from the file.
- Parameters:
-
filename Name of the file to read. objname The optional name of the node to read (if empty, the first top-level node will be used)
This is static template method of Algorithm. It's usage is following (in the case of SVM):
Ptr<SVM> svm = Algorithm::load<SVM>("my_svm_model.xml");
In order to make this method work, the derived class must overwrite Algorithm::read(const FileNode& fn).
static Ptr<_Tp> loadFromString | ( | const String & | strModel, |
const String & | objname = String() |
||
) | [static, inherited] |
Loads algorithm from a String.
- Parameters:
-
strModel The string variable containing the model you want to load. objname The optional name of the node to read (if empty, the first top-level node will be used)
This is static template method of Algorithm. It's usage is following (in the case of SVM):
Ptr<SVM> svm = Algorithm::loadFromString<SVM>(myStringModel);
virtual void read | ( | const FileNode & | fn ) | [virtual, inherited] |
Reads algorithm parameters from a file storage.
Reimplemented in DescriptorMatcher, and FlannBasedMatcher.
Reads algorithm from the file node.
This is static template method of Algorithm. It's usage is following (in the case of SVM):
Ptr<SVM> svm = Algorithm::read<SVM>(fn);
In order to make this method work, the derived class must overwrite Algorithm::read(const FileNode& fn) and also have static create() method without parameters (or with all the optional parameters)
Reimplemented in DescriptorMatcher, and FlannBasedMatcher.
virtual CV_WRAP void save | ( | const String & | filename ) | const [virtual, inherited] |
Saves the algorithm to a file.
In order to make this method work, the derived class must implement Algorithm::write(FileStorage& fs).
virtual void write | ( | FileStorage & | fs ) | const [virtual, inherited] |
Stores algorithm parameters in a file storage.
Reimplemented in DescriptorMatcher, and FlannBasedMatcher.
Generated on Tue Jul 12 2022 16:42:43 by
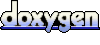