This library supports the internal QEI hardware of the LPC1768. WARNING: requires modification of the mbed module.
Dependents: Bracky-MPU6050-DMP mbed__motor_QEIHWv2_interupt_timer_sy_2017_RD_ver020 realtimeMM_V3 realtimeMM_V3 ... more
QEIHW Class Reference
QEI hardware interface class Requires mbed hardware modification: connect encoder PhA to p1.20, and PhB to p1.23. More...
#include <qeihw.h>
Public Member Functions | |
QEIHW (uint32_t _dirinv, uint32_t _sigmode, uint32_t _capmode, uint32_t _invinx) | |
Create a QEI object and configure it. | |
void | Reset (uint32_t ulResetType) |
Resets value for each type of QEI value, such as velocity, position, etc. | |
void | DeInit () |
Powers down the QEI block, returns pins to GPIO mode. | |
FlagStatus | Direction () |
Report direction (QEISTAT bit DIR) | |
uint32_t | GetPosition () |
Get current position value in QEI peripheral. | |
void | SetMaxPosition (uint32_t ulMaxPos) |
Set max position value for QEI peripheral. | |
void | SetPositionComp (uint8_t bPosCompCh, uint32_t ulPosComp) |
Set position compare value for QEI peripheral. | |
uint32_t | GetIndex () |
Get current index counter of QEI peripheral. | |
void | SetIndexComp (uint32_t ulIndexComp) |
Set value for index compare in QEI peripheral. | |
void | SetVelocityTimerReload (uint32_t ulReloadValue) |
Set Velocity timer reload value. | |
void | SetVelocityTimerReload_us (uint32_t ulReloadValue) |
Set Velocity timer reload value in microseconds. | |
uint32_t | GetTimer () |
Get current timer counter in QEI peripheral. | |
uint32_t | GetVelocity () |
Get current velocity pulse counter in current time period. | |
uint32_t | GetVelocityCap () |
Get the most recently measured velocity of the QEI. | |
void | SetVelocityComp (uint32_t ulVelComp) |
Set Velocity Compare value for QEI peripheral. | |
void | SetDigiFilter (uint32_t ulSamplingPulse) |
Set value of sampling count for the digital filter in QEI peripheral. | |
FlagStatus | GetIntStatus (uint32_t ulIntType) |
Check whether if specified interrupt flag status in QEI peripheral is set or not. | |
void | IntCmd (uint32_t ulIntType, FunctionalState NewState) |
Enable/Disable specified interrupt in QEI peripheral. | |
void | IntSet (uint32_t ulIntType) |
Asserts specified interrupt in QEI peripheral. | |
void | IntClear (uint32_t ulIntType) |
De-asserts specified interrupt (pending) in QEI peripheral. | |
void | AppendISR (uint32_t ulISRType, void(*fptr)(void)) |
Append interrupt handler for specific QEI interrupt source. | |
void | UnAppendISR (uint32_t ulISRType) |
Unappend interrupt handler for specific QEI interrupt source. | |
uint32_t | CalculateRPM (uint32_t ulVelCapValue, uint32_t ulPPR) |
Calculates the actual velocity in RPM passed via velocity capture value and Pulse Per Revolution (of the encoder) value parameter input. |
Detailed Description
QEI hardware interface class Requires mbed hardware modification: connect encoder PhA to p1.20, and PhB to p1.23.
Example:
// Display changes in encoder position and direction #include "mbed.h" #include "qeihw.h" DigitalOut led1(LED1); DigitalOut led3(LED3); QEIHW qei(QEI_DIRINV_NONE, QEI_SIGNALMODE_QUAD, QEI_CAPMODE_2X, QEI_INVINX_NONE ); int main() { int32_t temp, position = 0; qei.SetDigiFilter(480UL); qei.SetMaxPosition(0xFFFFFFFF); while(1) { while(position == (temp = qei.GetPosition()) ); position = temp; printf("New position = %d.\r\n", temp); led1 = qei.Direction() == SET ? 1 : 0; led3 = !led1; wait(0.1); } }
Definition at line 70 of file qeihw.h.
Constructor & Destructor Documentation
QEIHW | ( | uint32_t | _dirinv, |
uint32_t | _sigmode, | ||
uint32_t | _capmode, | ||
uint32_t | _invinx | ||
) |
Create a QEI object and configure it.
- Parameters:
-
_dirinv Direction invert. When = 1, complements the QEICONF register DIR bit _sigmode Signal mode. When = 0, PhA and PhB are quadrature inputs. When = 1, PhA is direction and PhB is clock _capmode Capture mode. When = 0, count PhA edges only (2X mode). Whe = 1, count PhB edges also (4X mode). _invinx Invert index. When = 1, inverts the sense of the index signal _dirinv Direction invert. When = 1, complements the QEICONF register DIR bit _sigmode Signal mode. When = 0, PhA and PhB are quadrature inputs. When = 1, PhA is direction and PhB is clock _capmode Capture mode. When = 0, count PhA edges only (2X mode). Whe = 1, count PhB edges also (4X mode). _invinx Invert index. When = 1, inverts the sense of the index signal
- Returns:
- None
Member Function Documentation
void AppendISR | ( | uint32_t | ulISRType, |
void(*)(void) | fptr | ||
) |
Append interrupt handler for specific QEI interrupt source.
- Parameters:
-
[in] ulISRType Interrupt Flag Status type, should be: - QEI_INTFLAG_INX_Int: index pulse was detected interrupt
- QEI_INTFLAG_TIM_Int: Velocity timer over flow interrupt
- QEI_INTFLAG_VELC_Int: Capture velocity is less than compare interrupt
- QEI_INTFLAG_DIR_Int: Change of direction interrupt
- QEI_INTFLAG_ERR_Int: An encoder phase error interrupt
- QEI_INTFLAG_ENCLK_Int: An encoder clock pulse was detected interrupt
- QEI_INTFLAG_POS0_Int: position 0 compare value is equal to the current position interrupt
- QEI_INTFLAG_POS1_Int: position 1 compare value is equal to the current position interrupt
- QEI_INTFLAG_POS2_Int: position 2 compare value is equal to the current position interrupt
- QEI_INTFLAG_REV_Int: Index compare value is equal to the current index count interrupt
- QEI_INTFLAG_POS0REV_Int: Combined position 0 and revolution count interrupt
- QEI_INTFLAG_POS1REV_Int: Combined position 1 and revolution count interrupt
- QEI_INTFLAG_POS2REV_Int: Combined position 2 and revolution count interrupt
- Returns:
- none
uint32_t CalculateRPM | ( | uint32_t | ulVelCapValue, |
uint32_t | ulPPR | ||
) |
Calculates the actual velocity in RPM passed via velocity capture value and Pulse Per Revolution (of the encoder) value parameter input.
- Parameters:
-
[in] ulVelCapValue Velocity capture input value that can be got from QEI_GetVelocityCap() function [in] ulPPR Pulse per round of encoder
- Returns:
- The actual value of velocity in RPM (Revolutions per minute)
void DeInit | ( | ) |
FlagStatus Direction | ( | ) |
uint32_t GetIndex | ( | ) |
FlagStatus GetIntStatus | ( | uint32_t | ulIntType ) |
Check whether if specified interrupt flag status in QEI peripheral is set or not.
- Parameters:
-
[in] ulIntType Interrupt Flag Status type, should be: - QEI_INTFLAG_INX_Int: index pulse was detected interrupt
- QEI_INTFLAG_TIM_Int: Velocity timer over flow interrupt
- QEI_INTFLAG_VELC_Int: Capture velocity is less than compare interrupt
- QEI_INTFLAG_DIR_Int: Change of direction interrupt
- QEI_INTFLAG_ERR_Int: An encoder phase error interrupt
- QEI_INTFLAG_ENCLK_Int: An encoder clock pulse was detected interrupt
- QEI_INTFLAG_POS0_Int: position 0 compare value is equal to the current position interrupt
- QEI_INTFLAG_POS1_Int: position 1 compare value is equal to the current position interrupt
- QEI_INTFLAG_POS2_Int: position 2 compare value is equal to the current position interrupt
- QEI_INTFLAG_REV_Int: Index compare value is equal to the current index count interrupt
- QEI_INTFLAG_POS0REV_Int: Combined position 0 and revolution count interrupt
- QEI_INTFLAG_POS1REV_Int: Combined position 1 and revolution count interrupt
- QEI_INTFLAG_POS2REV_Int: Combined position 2 and revolution count interrupt
- Returns:
- New State of specified interrupt flag status (SET or RESET)
uint32_t GetPosition | ( | ) |
uint32_t GetTimer | ( | ) |
uint32_t GetVelocity | ( | ) |
uint32_t GetVelocityCap | ( | ) |
Get the most recently measured velocity of the QEI.
Get the most recently captured velocity of the QEI.
When the Velocity timer in QEI is over-flow, the current velocity value will be loaded into Velocity Capture register.
- Returns:
- The most recently measured velocity value
void IntClear | ( | uint32_t | ulIntType ) |
De-asserts specified interrupt (pending) in QEI peripheral.
De-assert specified interrupt (pending) in QEI peripheral.
- Parameters:
-
[in] ulIntType Interrupt Flag Status type, should be: - QEI_INTFLAG_INX_Int: index pulse was detected interrupt
- QEI_INTFLAG_TIM_Int: Velocity timer over flow interrupt
- QEI_INTFLAG_VELC_Int: Capture velocity is less than compare interrupt
- QEI_INTFLAG_DIR_Int: Change of direction interrupt
- QEI_INTFLAG_ERR_Int: An encoder phase error interrupt
- QEI_INTFLAG_ENCLK_Int: An encoder clock pulse was detected interrupt
- QEI_INTFLAG_POS0_Int: position 0 compare value is equal to the current position interrupt
- QEI_INTFLAG_POS1_Int: position 1 compare value is equal to the current position interrupt
- QEI_INTFLAG_POS2_Int: position 2 compare value is equal to the current position interrupt
- QEI_INTFLAG_REV_Int: Index compare value is equal to the current index count interrupt
- QEI_INTFLAG_POS0REV_Int: Combined position 0 and revolution count interrupt
- QEI_INTFLAG_POS1REV_Int: Combined position 1 and revolution count interrupt
- QEI_INTFLAG_POS2REV_Int: Combined position 2 and revolution count interrupt
- Returns:
- None
void IntCmd | ( | uint32_t | ulIntType, |
FunctionalState | NewState | ||
) |
Enable/Disable specified interrupt in QEI peripheral.
- Parameters:
-
[in] ulIntType Interrupt Flag Status type, should be: - QEI_INTFLAG_INX_Int: index pulse was detected interrupt
- QEI_INTFLAG_TIM_Int: Velocity timer over flow interrupt
- QEI_INTFLAG_VELC_Int: Capture velocity is less than compare interrupt
- QEI_INTFLAG_DIR_Int: Change of direction interrupt
- QEI_INTFLAG_ERR_Int: An encoder phase error interrupt
- QEI_INTFLAG_ENCLK_Int: An encoder clock pulse was detected interrupt
- QEI_INTFLAG_POS0_Int: position 0 compare value is equal to the current position interrupt
- QEI_INTFLAG_POS1_Int: position 1 compare value is equal to the current position interrupt
- QEI_INTFLAG_POS2_Int: position 2 compare value is equal to the current position interrupt
- QEI_INTFLAG_REV_Int: Index compare value is equal to the current index count interrupt
- QEI_INTFLAG_POS0REV_Int: Combined position 0 and revolution count interrupt
- QEI_INTFLAG_POS1REV_Int: Combined position 1 and revolution count interrupt
- QEI_INTFLAG_POS2REV_Int: Combined position 2 and revolution count interrupt
[in] NewState New function state, should be: - DISABLE
- ENABLE
- Returns:
- None
void IntSet | ( | uint32_t | ulIntType ) |
Asserts specified interrupt in QEI peripheral.
Assert specified interrupt in QEI peripheral.
- Parameters:
-
[in] ulIntType Interrupt Flag Status type, should be: - QEI_INTFLAG_INX_Int: index pulse was detected interrupt
- QEI_INTFLAG_TIM_Int: Velocity timer over flow interrupt
- QEI_INTFLAG_VELC_Int: Capture velocity is less than compare interrupt
- QEI_INTFLAG_DIR_Int: Change of direction interrupt
- QEI_INTFLAG_ERR_Int: An encoder phase error interrupt
- QEI_INTFLAG_ENCLK_Int: An encoder clock pulse was detected interrupt
- QEI_INTFLAG_POS0_Int: position 0 compare value is equal to the current position interrupt
- QEI_INTFLAG_POS1_Int: position 1 compare value is equal to the current position interrupt
- QEI_INTFLAG_POS2_Int: position 2 compare value is equal to the current position interrupt
- QEI_INTFLAG_REV_Int: Index compare value is equal to the current index count interrupt
- QEI_INTFLAG_POS0REV_Int: Combined position 0 and revolution count interrupt
- QEI_INTFLAG_POS1REV_Int: Combined position 1 and revolution count interrupt
- QEI_INTFLAG_POS2REV_Int: Combined position 2 and revolution count interrupt
- Returns:
- None
void Reset | ( | uint32_t | ulResetType ) |
Resets value for each type of QEI value, such as velocity, position, etc.
Resets value for each type of QEI value, such as velocity, counter, position, etc.
- Parameters:
-
[in] ulResetType QEI Reset Type, should be one of the following: - QEI_RESET_POS: Reset Position Counter
- QEI_RESET_POSOnIDX: Reset Position Counter on Index signal
- QEI_RESET_VEL: Reset Velocity
- QEI_RESET_IDX: Reset Index Counter
[in] ulResetType QEI Reset Type, should be one of the following: - QEI_RESET_POS: Reset Position Counter
- QEI_RESET_POSOnIDX: Reset Position Counter on Index signal
- QEI_RESET_VEL: Reset Velocity
- QEI_RESET_IDX: Reset Index Counter
- Returns:
- None
void SetDigiFilter | ( | uint32_t | ulSamplingPulse ) |
void SetIndexComp | ( | uint32_t | ulIndexComp ) |
void SetMaxPosition | ( | uint32_t | ulMaxPos ) |
void SetPositionComp | ( | uint8_t | bPosCompCh, |
uint32_t | ulPosComp | ||
) |
Set position compare value for QEI peripheral.
- Parameters:
-
[in] bPosCompCh Compare Position channel, should be: - QEI_COMPPOS_CH_0: QEI compare position channel 0
- QEI_COMPPOS_CH_1: QEI compare position channel 1
- QEI_COMPPOS_CH_2: QEI compare position channel 2
[in] ulPosComp Compare Position value to set
- Returns:
- None
- Parameters:
-
[in] QEIx QEI peripheral, should be LPC_QEI [in] bPosCompCh Compare Position channel, should be: - QEI_COMPPOS_CH_0: QEI compare position channel 0
- QEI_COMPPOS_CH_1: QEI compare position channel 1
- QEI_COMPPOS_CH_2: QEI compare position channel 2
[in] ulPosComp Compare Position value to set
- Returns:
- None
void SetVelocityComp | ( | uint32_t | ulVelComp ) |
void SetVelocityTimerReload | ( | uint32_t | ulReloadValue ) |
void SetVelocityTimerReload_us | ( | uint32_t | ulReloadValue ) |
void UnAppendISR | ( | uint32_t | ulISRType ) |
Unappend interrupt handler for specific QEI interrupt source.
- Parameters:
-
[in] ulISRType Interrupt Flag Status type, should be: - QEI_INTFLAG_INX_Int: index pulse was detected interrupt
- QEI_INTFLAG_TIM_Int: Velocity timer over flow interrupt
- QEI_INTFLAG_VELC_Int: Capture velocity is less than compare interrupt
- QEI_INTFLAG_DIR_Int: Change of direction interrupt
- QEI_INTFLAG_ERR_Int: An encoder phase error interrupt
- QEI_INTFLAG_ENCLK_Int: An encoder clock pulse was detected interrupt
- QEI_INTFLAG_POS0_Int: position 0 compare value is equal to the current position interrupt
- QEI_INTFLAG_POS1_Int: position 1 compare value is equal to the current position interrupt
- QEI_INTFLAG_POS2_Int: position 2 compare value is equal to the current position interrupt
- QEI_INTFLAG_REV_Int: Index compare value is equal to the current index count interrupt
- QEI_INTFLAG_POS0REV_Int: Combined position 0 and revolution count interrupt
- QEI_INTFLAG_POS1REV_Int: Combined position 1 and revolution count interrupt
- QEI_INTFLAG_POS2REV_Int: Combined position 2 and revolution count interrupt
- Returns:
- none
Generated on Tue Jul 12 2022 18:43:44 by
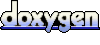