GSwifiInterface library (interface for GainSpan Wi-Fi GS1011 modules) Please see https://mbed.org/users/gsfan/notebook/GSwifiInterface/
Dependents: GSwifiInterface_HelloWorld GSwifiInterface_HelloServo GSwifiInterface_UDPEchoServer GSwifiInterface_UDPEchoClient ... more
Fork of WiflyInterface by
GSwifiInterface Class Reference
Interface using GSwifi to connect to an IP-based network. More...
#include <GSwifiInterface.h>
Inherits GSwifi.
Public Types | |
enum | WiFiMode |
Wi-Fi mode. More... | |
enum | Security |
Wi-Fi security. More... | |
enum | Protocol |
TCP/IP protocol. More... | |
enum | Type |
Client/Server. More... | |
Public Member Functions | |
GSwifiInterface (PinName tx, PinName rx, PinName cts, PinName rts, PinName reset, PinName mosi, PinName miso, PinName sclk, PinName cs, PinName wake, PinName alarm=NC, int baud=9600, int freq=2000000) | |
Constructor. | |
int | init (const char *name=NULL) |
Initialize the interface with DHCP. | |
int | init (const char *ip, const char *mask, const char *gateway, const char *dns=NULL, const char *name=NULL) |
Initialize the interface with a static IP address. | |
int | connect (Security sec, const char *ssid, const char *phrase, WiFiMode mode=WM_INFRASTRUCTURE) |
Connect Bring the interface up, start DHCP if needed. | |
int | disconnect () |
Disconnect Bring the interface down. | |
char * | getMACAddress () |
Get the MAC address of your Ethernet interface. | |
char * | getIPAddress () |
Get the IP address of your Ethernet interface. | |
char * | getGateway () |
Get the Gateway address of your Ethernet interface. | |
char * | getNetworkMask () |
Get the Network mask of your Ethernet interface. | |
int | join () |
Connect the wifi module to the ssid contained in the constructor. | |
int | adhock () |
Connect the wifi module to the adhock in the constructor. | |
int | limitedap () |
Connect the wifi module to the limited AP in the constructor. | |
int | dissociate () |
Disconnect the wifi module from the access point. | |
bool | isAssociated () |
Check if a Wi-Fi link is active. | |
void | poll () |
polling if not use rtos | |
Status | getStatus () |
get Wi-Fi modue status | |
int | setMacAddress (const char *mac) |
set MAC address | |
int | getMacAddress (char *mac) |
get MAC address | |
int | setAddress (const char *name=NULL) |
use DHCP | |
int | setAddress (const char *ip, const char *netmask, const char *gateway, const char *dns=NULL, const char *name=NULL) |
use static IP address | |
int | getAddress (char *ip, char *netmask, char *gateway) |
get IP address | |
int | setSsid (Security sec, const char *ssid, const char *phrase) |
set Wi-Fi security parameter | |
int | getRssi () |
get RSSI | |
int | powerSave (int active, int save) |
power save mode | |
int | setRfPower (int power) |
RF power. | |
int | setTime (time_t time) |
set system time | |
time_t | getTime () |
get system time | |
int | ntpdate (char *host, int sec=0) |
set NTP server | |
int | setGpio (int port, int out) |
GPIO output. | |
int | provisioning (char *user, char *pass) |
Web server. | |
int | standby (int msec) |
standby mode | |
int | deepSleep () |
deep sleep mode | |
int | wakeup () |
restore standby or deep sleep | |
int | getHostByName (const char *host, char *ip) |
Resolv hostname. | |
int | open (Protocol proto, const char *ip, int port, int src=0, void(*func)(int)=NULL) |
TCP/UDP client. | |
int | listen (Protocol proto, int port, void(*func)(int)=NULL) |
TCP/UDP server. | |
int | close (int cid) |
close client/server | |
int | send (int cid, const char *buf, int len) |
send data tcp(s/c), udp(c) | |
int | sendto (int cid, const char *buf, int len, const char *ip, int port) |
send data udp(s) | |
int | recv (int cid, char *buf, int len) |
recv data tcp(s/c), udp(c) | |
int | recvfrom (int cid, char *buf, int len, char *ip, int *port) |
recv data udp(s) | |
int | readable (int cid) |
readable recv data | |
bool | isConnected (int cid) |
tcp/udp connected | |
int | httpGet (const char *host, int port, const char *uri, bool ssl=false, const char *user=NULL, const char *pwd=NULL, void(*func)(int)=NULL) |
http request (GET method) | |
int | httpPost (const char *host, int port, const char *uri, const char *body, bool ssl=false, const char *user=NULL, const char *pwd=NULL, void(*func)(int)=NULL) |
http request (POST method) | |
int | httpd (int port=80) |
start http server | |
void | httpdError (int cid, int err) |
attach uri to dirctory handler | |
int | httpdAttach (const char *uri, const char *dir) |
attach uri to dirctory handler | |
int | httpdAttach (const char *uri, void(*funcCgi)(int), int type=0) |
attach uri to cgi handler | |
int | wsOpen (const char *host, int port, const char *uri, const char *user=NULL, const char *pwd=NULL) |
websocket request (Upgrade method) | |
int | wsSend (int cid, const char *buf, int len, const char *mask=NULL) |
send websocket data | |
int | mail (const char *host, int port, const char *to, const char *from, const char *subject, const char *mesg, const char *user=NULL, const char *pwd=NULL) |
send mail (smtp) | |
int | sendCommand (const char *cmd, Response res=RES_NULL, int timeout=DEFAULT_WAIT_RESP_TIMEOUT) |
Send a command to the wifi module. | |
int | sendData (const char *data, int len, int timeout=CFG_TIMEOUT, const char *cmd=NULL) |
Send a command to the wifi module. |
Detailed Description
Interface using GSwifi to connect to an IP-based network.
Definition at line 32 of file GSwifiInterface.h.
Member Enumeration Documentation
Constructor & Destructor Documentation
GSwifiInterface | ( | PinName | tx, |
PinName | rx, | ||
PinName | cts, | ||
PinName | rts, | ||
PinName | reset, | ||
PinName | mosi, | ||
PinName | miso, | ||
PinName | sclk, | ||
PinName | cs, | ||
PinName | wake, | ||
PinName | alarm = NC , |
||
int | baud = 9600 , |
||
int | freq = 2000000 |
||
) |
Constructor.
- Parameters:
-
tx mbed pin to use for tx line of Serial interface rx mbed pin to use for rx line of Serial interface cts mbed pin to use for cts line of Serial interface rts mbed pin to use for rts line of Serial interface reset reset pin of the wifi module alarm alarm pin of the wifi module (default: NC) baud baud rate of Serial interface (default: 9600)
Definition at line 8 of file GSwifiInterface.cpp.
Member Function Documentation
int adhock | ( | ) | [inherited] |
Connect the wifi module to the adhock in the constructor.
- Parameters:
-
sec Security type (NONE, WEP_128 or WPA) ssid ssid of the network phrase WEP or WPA key
- Returns:
- 0 if connected, -1 otherwise
Definition at line 204 of file GSwifi.cpp.
int close | ( | int | cid ) | [inherited] |
close client/server
Definition at line 85 of file GSwifi_sock.cpp.
int connect | ( | Security | sec, |
const char * | ssid, | ||
const char * | phrase, | ||
WiFiMode | mode = WM_INFRASTRUCTURE |
||
) |
Connect Bring the interface up, start DHCP if needed.
- Parameters:
-
sec the Wi-Fi security type ssid the Wi-Fi SSID phrase the Wi-Fi passphrase or security key mode the Wi-Fi mode
- Returns:
- 0 on success, a negative number on failure
Definition at line 29 of file GSwifiInterface.cpp.
int deepSleep | ( | ) | [inherited] |
deep sleep mode
Definition at line 132 of file GSwifi_util.cpp.
int disconnect | ( | ) |
Disconnect Bring the interface down.
- Returns:
- 0 on success, a negative number on failure
Reimplemented from GSwifi.
Definition at line 43 of file GSwifiInterface.cpp.
int dissociate | ( | ) | [inherited] |
Disconnect the wifi module from the access point.
- Returns:
- 0 if successful
Definition at line 306 of file GSwifi.cpp.
int getAddress | ( | char * | ip, |
char * | netmask, | ||
char * | gateway | ||
) | [inherited] |
get IP address
Definition at line 453 of file GSwifi.cpp.
char * getGateway | ( | ) |
Get the Gateway address of your Ethernet interface.
- Returns:
- a pointer to a string containing the Gateway address
Definition at line 58 of file GSwifiInterface.cpp.
int getHostByName | ( | const char * | host, |
char * | ip | ||
) | [inherited] |
Resolv hostname.
- Parameters:
-
name hostname ip resolved ip address
Definition at line 21 of file GSwifi_sock.cpp.
char * getIPAddress | ( | ) |
Get the IP address of your Ethernet interface.
- Returns:
- a pointer to a string containing the IP address
Definition at line 53 of file GSwifiInterface.cpp.
int getMacAddress | ( | char * | mac ) | [inherited] |
get MAC address
Definition at line 427 of file GSwifi.cpp.
char * getMACAddress | ( | ) |
Get the MAC address of your Ethernet interface.
- Returns:
- a pointer to a string containing the MAC address
Definition at line 48 of file GSwifiInterface.cpp.
char * getNetworkMask | ( | ) |
Get the Network mask of your Ethernet interface.
- Returns:
- a pointer to a string containing the Network mask
Definition at line 63 of file GSwifiInterface.cpp.
int getRssi | ( | ) | [inherited] |
GSwifi::Status getStatus | ( | ) | [inherited] |
time_t getTime | ( | ) | [inherited] |
int httpd | ( | int | port = 80 ) |
[inherited] |
int httpdAttach | ( | const char * | uri, |
const char * | dir | ||
) | [inherited] |
attach uri to dirctory handler
Definition at line 126 of file GSwifi_httpd_util.cpp.
int httpdAttach | ( | const char * | uri, |
void(*)(int) | funcCgi, | ||
int | type = 0 |
||
) | [inherited] |
attach uri to cgi handler
Definition at line 142 of file GSwifi_httpd_util.cpp.
void httpdError | ( | int | cid, |
int | err | ||
) | [inherited] |
attach uri to dirctory handler
Definition at line 79 of file GSwifi_httpd_util.cpp.
int httpGet | ( | const char * | host, |
int | port, | ||
const char * | uri, | ||
bool | ssl = false , |
||
const char * | user = NULL , |
||
const char * | pwd = NULL , |
||
void(*)(int) | func = NULL |
||
) | [inherited] |
http request (GET method)
Definition at line 21 of file GSwifi_http.cpp.
int httpPost | ( | const char * | host, |
int | port, | ||
const char * | uri, | ||
const char * | body, | ||
bool | ssl = false , |
||
const char * | user = NULL , |
||
const char * | pwd = NULL , |
||
void(*)(int) | func = NULL |
||
) | [inherited] |
http request (POST method)
Definition at line 62 of file GSwifi_http.cpp.
int init | ( | const char * | ip, |
const char * | mask, | ||
const char * | gateway, | ||
const char * | dns = NULL , |
||
const char * | name = NULL |
||
) |
Initialize the interface with a static IP address.
Initialize the interface and configure it with the following static configuration (no connection at this point).
- Parameters:
-
ip the IP address to use mask the IP address mask gateway the gateway to use
- Returns:
- 0 on success, a negative number on failure
Definition at line 24 of file GSwifiInterface.cpp.
int init | ( | const char * | name = NULL ) |
Initialize the interface with DHCP.
Initialize the interface and configure it to use DHCP (no connection at this point).
- Returns:
- 0 on success, a negative number on failure
Definition at line 19 of file GSwifiInterface.cpp.
bool isAssociated | ( | ) | [inherited] |
Check if a Wi-Fi link is active.
- Returns:
- true if successful
Definition at line 335 of file GSwifi.cpp.
bool isConnected | ( | int | cid ) | [inherited] |
tcp/udp connected
Definition at line 162 of file GSwifi_sock.cpp.
int join | ( | ) | [inherited] |
Connect the wifi module to the ssid contained in the constructor.
- Parameters:
-
sec Security type (NONE, WEP_128 or WPA) ssid ssid of the network phrase WEP or WPA key
- Returns:
- 0 if connected, -1 otherwise
Definition at line 79 of file GSwifi.cpp.
int limitedap | ( | ) | [inherited] |
Connect the wifi module to the limited AP in the constructor.
- Parameters:
-
sec Security type (NONE, WEP_128 or WPA) ssid ssid of the network phrase WEP or WPA key
- Returns:
- 0 if connected, -1 otherwise
Definition at line 252 of file GSwifi.cpp.
int listen | ( | Protocol | proto, |
int | port, | ||
void(*)(int) | func = NULL |
||
) | [inherited] |
int mail | ( | const char * | host, |
int | port, | ||
const char * | to, | ||
const char * | from, | ||
const char * | subject, | ||
const char * | mesg, | ||
const char * | user = NULL , |
||
const char * | pwd = NULL |
||
) | [inherited] |
send mail (smtp)
- Parameters:
-
host SMTP server port SMTP port (25 or 587 or etc.) to To address from From address subject Subject mesg Message user username (SMTP Auth) pwd password (SMTP Auth)
- Return values:
-
0 success -1 failure
Definition at line 23 of file GSwifi_smtp.cpp.
int ntpdate | ( | char * | host, |
int | sec = 0 |
||
) | [inherited] |
set NTP server
- Parameters:
-
host SNTP server sec time sync interval, 0:one time
Definition at line 87 of file GSwifi_util.cpp.
int open | ( | Protocol | proto, |
const char * | ip, | ||
int | port, | ||
int | src = 0 , |
||
void(*)(int) | func = NULL |
||
) | [inherited] |
void poll | ( | ) | [inherited] |
polling if not use rtos
Definition at line 340 of file GSwifi.cpp.
int powerSave | ( | int | active, |
int | save | ||
) | [inherited] |
power save mode
- Parameters:
-
active rx radio 0:switched off, 1:always on save power save 0:disable, 1:enable
Definition at line 63 of file GSwifi_util.cpp.
int provisioning | ( | char * | user, |
char * | pass | ||
) | [inherited] |
Web server.
Definition at line 100 of file GSwifi_util.cpp.
int readable | ( | int | cid ) | [inherited] |
readable recv data
Definition at line 155 of file GSwifi_sock.cpp.
int recv | ( | int | cid, |
char * | buf, | ||
int | len | ||
) | [inherited] |
int recvfrom | ( | int | cid, |
char * | buf, | ||
int | len, | ||
char * | ip, | ||
int * | port | ||
) | [inherited] |
int send | ( | int | cid, |
const char * | buf, | ||
int | len | ||
) | [inherited] |
send data tcp(s/c), udp(c)
Definition at line 93 of file GSwifi_sock.cpp.
int sendCommand | ( | const char * | cmd, |
Response | res = RES_NULL , |
||
int | timeout = DEFAULT_WAIT_RESP_TIMEOUT |
||
) | [inherited] |
Send a command to the wifi module.
Check if the module is in command mode. If not enter in command mode
- Parameters:
-
cmd string to be sent res need response timeout
- Returns:
- 0 if successful
Definition at line 28 of file GSwifi_at.cpp.
int sendData | ( | const char * | data, |
int | len, | ||
int | timeout = CFG_TIMEOUT , |
||
const char * | cmd = NULL |
||
) | [inherited] |
Send a command to the wifi module.
Check if the module is in command mode. If not enter in command mode
- Parameters:
-
data string to be sent res need response timeout cmd
- Returns:
- 0 if successful
Definition at line 67 of file GSwifi_at.cpp.
int sendto | ( | int | cid, |
const char * | buf, | ||
int | len, | ||
const char * | ip, | ||
int | port | ||
) | [inherited] |
send data udp(s)
Definition at line 109 of file GSwifi_sock.cpp.
int setAddress | ( | const char * | name = NULL ) |
[inherited] |
use DHCP
Definition at line 433 of file GSwifi.cpp.
int setAddress | ( | const char * | ip, |
const char * | netmask, | ||
const char * | gateway, | ||
const char * | dns = NULL , |
||
const char * | name = NULL |
||
) | [inherited] |
use static IP address
- Parameters:
-
ip my ip address (dhcp start address) netmask subnet mask gateway default gateway dns name server (default NULL) name my host name (default NULL)
Definition at line 439 of file GSwifi.cpp.
int setGpio | ( | int | port, |
int | out | ||
) | [inherited] |
GPIO output.
- Parameters:
-
port 10,11,30,31 out 0:set(high), 1:reset(low)
Definition at line 96 of file GSwifi_util.cpp.
int setMacAddress | ( | const char * | mac ) | [inherited] |
set MAC address
Definition at line 421 of file GSwifi.cpp.
int setRfPower | ( | int | power ) | [inherited] |
int setSsid | ( | Security | sec, |
const char * | ssid, | ||
const char * | phrase | ||
) | [inherited] |
set Wi-Fi security parameter
- Parameters:
-
sec Security ssid SSID pass pass phrase
Definition at line 460 of file GSwifi.cpp.
int setTime | ( | time_t | time ) | [inherited] |
int standby | ( | int | msec ) | [inherited] |
standby mode
- Parameters:
-
msec wakeup after wakeup after msec or alarm1/2 core off, save to RTC ram
Definition at line 107 of file GSwifi_util.cpp.
int wakeup | ( | ) | [inherited] |
restore standby or deep sleep
Definition at line 139 of file GSwifi_util.cpp.
int wsOpen | ( | const char * | host, |
int | port, | ||
const char * | uri, | ||
const char * | user = NULL , |
||
const char * | pwd = NULL |
||
) | [inherited] |
websocket request (Upgrade method)
- Returns:
- CID, -1:failure
Definition at line 25 of file GSwifi_httpd_ws.cpp.
int wsSend | ( | int | cid, |
const char * | buf, | ||
int | len, | ||
const char * | mask = NULL |
||
) | [inherited] |
send websocket data
Definition at line 124 of file GSwifi_httpd_ws.cpp.
Generated on Thu Jul 14 2022 07:53:37 by
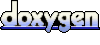