GSwifiInterface library (interface for GainSpan Wi-Fi GS1011 modules) Please see https://mbed.org/users/gsfan/notebook/GSwifiInterface/
Dependents: GSwifiInterface_HelloWorld GSwifiInterface_HelloServo GSwifiInterface_UDPEchoServer GSwifiInterface_UDPEchoClient ... more
Fork of WiflyInterface by
GSwifi_http.cpp
00001 /* Copyright (C) 2013 gsfan, MIT License 00002 * 00003 * Permission is hereby granted, free of charge, to any person obtaining a copy of this software 00004 * and associated documentation files (the "Software"), to deal in the Software without restriction, 00005 * including without limitation the rights to use, copy, modify, merge, publish, distribute, 00006 * sublicense, and/or sell copies of the Software, and to permit persons to whom the Software is 00007 * furnished to do so, subject to the following conditions: 00008 * 00009 * The above copyright notice and this permission notice shall be included in all copies or 00010 * substantial portions of the Software. 00011 * 00012 * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED, INCLUDING 00013 * BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND 00014 * NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, 00015 * DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00016 * OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE. 00017 */ 00018 00019 #include "GSwifi.h" 00020 00021 int GSwifi::httpGet (const char *host, int port, const char *uri, bool ssl, const char *user, const char *pwd, void(*func)(int)) { 00022 char ip[17]; 00023 int cid; 00024 00025 if (!isAssociated() || _state.status != STAT_READY) return -1; 00026 00027 if (getHostByName(host, ip)) return -1; 00028 if (! port) { 00029 if (ssl) { 00030 port = 443; 00031 } else { 00032 port = 80; 00033 } 00034 } 00035 00036 if (cmdHTTPCONF(3, "close")) return -1; // Connection 00037 cmdHTTPCONFDEL(5); 00038 cmdHTTPCONFDEL(7); 00039 cmdHTTPCONF(11, host); // Host 00040 if (user && pwd) { 00041 char tmp[CFG_CMD_SIZE], tmp2[CFG_CMD_SIZE]; 00042 snprintf(tmp, sizeof(tmp), "%s:%s", user, pwd); 00043 base64encode(tmp, strlen(tmp), tmp2, sizeof(tmp2)); 00044 sprintf(tmp, "Basic %s", tmp2); 00045 cmdHTTPCONF(2, tmp); // Authorization 00046 } else { 00047 cmdHTTPCONFDEL(2); 00048 } 00049 00050 _state.cid = -1; 00051 if (cmdHTTPOPEN(ip, port, ssl)) return -1; 00052 if (_state.cid < 0) return -1; 00053 cid = _state.cid; 00054 _con[cid].protocol = PROTO_HTTPGET; 00055 _con[cid].type = TYPE_CLIENT; 00056 _con[cid].func = func; 00057 00058 cmdHTTPSEND(cid, false, uri); // GET 00059 return cid; 00060 } 00061 00062 int GSwifi::httpPost (const char *host, int port, const char *uri, const char *body, bool ssl, const char *user, const char *pwd, void(*func)(int)) { 00063 char cmd[CFG_CMD_SIZE]; 00064 char ip[17]; 00065 int cid, len; 00066 00067 if (!isAssociated() || _state.status != STAT_READY) return -1; 00068 00069 if (getHostByName(host, ip)) return -1; 00070 if (! port) { 00071 if (ssl) { 00072 port = 443; 00073 } else { 00074 port = 80; 00075 } 00076 } 00077 len = strlen(body); 00078 00079 if (cmdHTTPCONF(3, "close")) return -1; // Connection 00080 sprintf(cmd, "%d", len); 00081 cmdHTTPCONF(5, cmd); // Content-Length 00082 cmdHTTPCONF(7, "application/x-www-form-urlencoded"); // Content-type 00083 cmdHTTPCONF(11, host); // Host 00084 if (user && pwd) { 00085 char tmp[CFG_CMD_SIZE], tmp2[CFG_CMD_SIZE]; 00086 snprintf(tmp, sizeof(tmp), "%s:%s", user, pwd); 00087 base64encode(tmp, strlen(tmp), tmp2, sizeof(tmp2)); 00088 sprintf(tmp, "Basic %s", tmp2); 00089 cmdHTTPCONF(2, tmp); // Authorization 00090 } else { 00091 cmdHTTPCONFDEL(2); 00092 } 00093 00094 _state.cid = -1; 00095 if (cmdHTTPOPEN(ip, port, ssl)) return -1; 00096 if (_state.cid < 0) return -1; 00097 cid = _state.cid; 00098 _con[cid].protocol = PROTO_HTTPPOST; 00099 _con[cid].type = TYPE_CLIENT; 00100 _con[cid].func = func; 00101 00102 cmdHTTPSEND(cid, true, uri, len); // POST 00103 sprintf(cmd, "\x1bH%X", cid); 00104 sendData(body, len, DEFAULT_WAIT_RESP_TIMEOUT, cmd); 00105 return cid; 00106 } 00107 00108 00109 /* base64encode code from 00110 * Copyright (c) 2010 Donatien Garnier (donatiengar [at] gmail [dot] com) 00111 */ 00112 int GSwifi::base64encode (const char *input, int length, char *output, int len) { 00113 static const char base64[] = "ABCDEFGHIJKLMNOPQRSTUVWXYZabcdefghijklmnopqrstuvwxyz0123456789+/"; 00114 unsigned int c, c1, c2, c3; 00115 00116 if (len < ((((length-1)/3)+1)<<2)) return -1; 00117 for(unsigned int i = 0, j = 0; i<length; i+=3,j+=4) { 00118 c1 = ((((unsigned char)*((unsigned char *)&input[i])))); 00119 c2 = (length>i+1)?((((unsigned char)*((unsigned char *)&input[i+1])))):0; 00120 c3 = (length>i+2)?((((unsigned char)*((unsigned char *)&input[i+2])))):0; 00121 00122 c = ((c1 & 0xFC) >> 2); 00123 output[j+0] = base64[c]; 00124 c = ((c1 & 0x03) << 4) | ((c2 & 0xF0) >> 4); 00125 output[j+1] = base64[c]; 00126 c = ((c2 & 0x0F) << 2) | ((c3 & 0xC0) >> 6); 00127 output[j+2] = (length>i+1)?base64[c]:'='; 00128 c = (c3 & 0x3F); 00129 output[j+3] = (length>i+2)?base64[c]:'='; 00130 } 00131 output[(((length-1)/3)+1)<<2] = '\0'; 00132 return 0; 00133 } 00134 00135 00136 /* urlencode code from 00137 * Copyright (c) 2010 Donatien Garnier (donatiengar [at] gmail [dot] com) 00138 */ 00139 int GSwifi::urlencode (const char *str, char *buf, int len) { 00140 // char *pstr = str, *buf = (char*)malloc(strlen(str) * 3 + 1), *pbuf = buf; 00141 const char *pstr = str; 00142 char *pbuf = buf; 00143 00144 if (len < (strlen(str) * 3 + 1)) return -1; 00145 while (*pstr) { 00146 if (isalnum(*pstr) || *pstr == '-' || *pstr == '_' || *pstr == '.' || *pstr == '~') 00147 *pbuf++ = *pstr; 00148 else if (*pstr == ' ') 00149 *pbuf++ = '+'; 00150 else 00151 *pbuf++ = '%', *pbuf++ = to_hex(*pstr >> 4), *pbuf++ = to_hex(*pstr & 15); 00152 pstr++; 00153 } 00154 *pbuf = '\0'; 00155 return 0; 00156 } 00157 00158 /* urldecode code from 00159 * Copyright (c) 2010 Donatien Garnier (donatiengar [at] gmail [dot] com) 00160 */ 00161 int GSwifi::urldecode (const char *str, char *buf, int len) { 00162 // char *pstr = str, *buf = (char*)malloc(strlen(str) + 1), *pbuf = buf; 00163 const char *pstr = str; 00164 char *pbuf = buf; 00165 00166 if (len < (strlen(str) / 3 - 1)) return -1; 00167 while (*pstr) { 00168 if (*pstr == '%') { 00169 if (pstr[1] && pstr[2]) { 00170 *pbuf++ = from_hex(pstr[1]) << 4 | from_hex(pstr[2]); 00171 pstr += 2; 00172 } 00173 } else if (*pstr == '+') { 00174 *pbuf++ = ' '; 00175 } else { 00176 *pbuf++ = *pstr; 00177 } 00178 pstr++; 00179 } 00180 *pbuf = '\0'; 00181 return 0; 00182 }
Generated on Thu Jul 14 2022 07:53:37 by
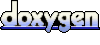