GSwifiInterface library (interface for GainSpan Wi-Fi GS1011 modules) Please see https://mbed.org/users/gsfan/notebook/GSwifiInterface/
Dependents: GSwifiInterface_HelloWorld GSwifiInterface_HelloServo GSwifiInterface_UDPEchoServer GSwifiInterface_UDPEchoClient ... more
Fork of WiflyInterface by
GSwifi_util.cpp
00001 /* Copyright (C) 2013 gsfan, MIT License 00002 * 00003 * Permission is hereby granted, free of charge, to any person obtaining a copy of this software 00004 * and associated documentation files (the "Software"), to deal in the Software without restriction, 00005 * including without limitation the rights to use, copy, modify, merge, publish, distribute, 00006 * sublicense, and/or sell copies of the Software, and to permit persons to whom the Software is 00007 * furnished to do so, subject to the following conditions: 00008 * 00009 * The above copyright notice and this permission notice shall be included in all copies or 00010 * substantial portions of the Software. 00011 * 00012 * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED, INCLUDING 00013 * BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND 00014 * NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, 00015 * DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00016 * OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE. 00017 */ 00018 00019 #include "GSwifi.h" 00020 00021 int GSwifi::x2i (char c) { 00022 if (c >= '0' && c <= '9') { 00023 return c - '0'; 00024 } else 00025 if (c >= 'A' && c <= 'F') { 00026 return c - 'A' + 10; 00027 } else 00028 if (c >= 'a' && c <= 'f') { 00029 return c - 'a' + 10; 00030 } 00031 return 0; 00032 } 00033 00034 char GSwifi::i2x (int i) { 00035 if (i >= 0 && i <= 9) { 00036 return i + '0'; 00037 } else 00038 if (i >= 10 && i <= 15) { 00039 return i - 10 + 'A'; 00040 } 00041 return 0; 00042 } 00043 00044 int GSwifi::from_hex (int ch) { 00045 return isdigit(ch) ? ch - '0' : tolower(ch) - 'a' + 10; 00046 } 00047 00048 int GSwifi::to_hex (int code) { 00049 static char hex[] = "0123456789abcdef"; 00050 return hex[code & 15]; 00051 } 00052 00053 00054 int GSwifi::setRegion (int reg) { 00055 return cmdWREGDOMAIN(reg); 00056 } 00057 00058 int GSwifi::getRssi () { 00059 cmdWRSSI(); 00060 return _state.rssi; 00061 } 00062 00063 int GSwifi::powerSave (int active, int save) { 00064 cmdWRXACTIVE(active); 00065 return cmdWRXPS(save); 00066 } 00067 00068 int GSwifi::setRfPower (int power) { 00069 if (power < 0 || power > 7) return false; 00070 return cmdWP(power); 00071 } 00072 00073 int GSwifi::setTime (time_t time) { 00074 char dmy[16], hms[16]; 00075 struct tm *t = localtime(&time); 00076 00077 sprintf(dmy, "%d/%d/%d", t->tm_mday, t->tm_mon + 1, t->tm_year + 1900); 00078 sprintf(hms, "%d:%d:%d", t->tm_hour, t->tm_min, t->tm_sec); 00079 return cmdSETTIME(dmy, hms); 00080 } 00081 00082 time_t GSwifi::getTime () { 00083 cmdGETTIME(); 00084 return _state.time; 00085 } 00086 00087 int GSwifi::ntpdate (char *host, int sec) { 00088 char ip[17]; 00089 00090 if (!isAssociated() || _state.status != STAT_READY) return -1; 00091 00092 if (getHostByName(host, ip)) return -1; 00093 return cmdNTIMESYNC(true, ip, sec); 00094 } 00095 00096 int GSwifi::setGpio (int port, int out) { 00097 return cmdDGPIO(port, out); 00098 } 00099 00100 int GSwifi::provisioning (char *user, char *pass) { 00101 00102 if (!isAssociated() || _state.status != STAT_READY) return -1; 00103 00104 return cmdWEBPROV(user, pass); 00105 } 00106 00107 int GSwifi::standby (int msec) { 00108 switch (_state.status) { 00109 case STAT_READY: 00110 cmdNCLOSEALL(); 00111 for (int i = 0; i < 16; i ++) { 00112 _con[i].connected = false; 00113 } 00114 cmdSTORENWCONN(); 00115 // cmdWRXACTIVE(false); 00116 break; 00117 case STAT_WAKEUP: 00118 if (cmdE(false)) return -1; 00119 if (_flow) { 00120 cmdR(true); 00121 } 00122 break; 00123 default: 00124 return -1; 00125 } 00126 00127 _state.status = STAT_STANDBY; 00128 return cmdPSSTBY(msec, 0); 00129 } 00130 00131 00132 int GSwifi::deepSleep () { 00133 if (_state.status != STAT_READY) return -1; 00134 00135 _state.status = STAT_DEEPSLEEP; 00136 return cmdPSDPSLEEP(); // go deep sleep 00137 } 00138 00139 int GSwifi::wakeup () { 00140 int r; 00141 00142 if (_state.status == STAT_STANDBY) { 00143 Timer timeout; 00144 setAlarm(true); 00145 timeout.start(); 00146 while (timeout.read() < CFG_TIMEOUT) { 00147 if (_state.status == STAT_WAKEUP) break; 00148 } 00149 timeout.stop(); 00150 setAlarm(false); 00151 } 00152 00153 switch (_state.status) { 00154 case STAT_WAKEUP: 00155 if (cmdE(false)) return -1; 00156 if (_flow) { 00157 cmdR(true); 00158 } 00159 cmdBDATA(true); 00160 r = cmdRESTORENWCONN(); 00161 if (!r) { 00162 wait_ms(100); 00163 // cmdWRXACTIVE(true); 00164 _state.status = STAT_READY; 00165 } 00166 return r; 00167 case STAT_DEEPSLEEP: 00168 r = cmdAT(); 00169 if (!r) { 00170 _state.status = STAT_READY; 00171 } 00172 return r; 00173 default: 00174 break; 00175 } 00176 return -1; 00177 }
Generated on Thu Jul 14 2022 07:53:37 by
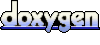