
X-CUBE-SPN1-20150128 example source code for one motor compiled under mbed. Tested OK on Nucleo F401. l6474.cpp is modified from original with defines in l6474_target_config.h to select the original behaviour (motor de-energised when halted), or new mode to continue powering with a (reduced) current in the coils (braking/position hold capability). On F401 avoid using mbed's InterruptIn on pins 10-15 (any port). Beware of other conflicts! L0 & F0 are included but untested.
motorcontrol.cpp
00001 /** 00002 ****************************************************************************** 00003 * @file motorcontrol.c 00004 * @author IPC Rennes 00005 * @version V1.0.0 00006 * @date 12-November-2014 00007 * @brief This file provides common functions for motor control 00008 ****************************************************************************** 00009 * @attention 00010 * 00011 * <h2><center>© COPYRIGHT(c) 2014 STMicroelectronics</center></h2> 00012 * 00013 * Redistribution and use in source and binary forms, with or without modification, 00014 * are permitted provided that the following conditions are met: 00015 * 1. Redistributions of source code must retain the above copyright notice, 00016 * this list of conditions and the following disclaimer. 00017 * 2. Redistributions in binary form must reproduce the above copyright notice, 00018 * this list of conditions and the following disclaimer in the documentation 00019 * and/or other materials provided with the distribution. 00020 * 3. Neither the name of STMicroelectronics nor the names of its contributors 00021 * may be used to endorse or promote products derived from this software 00022 * without specific prior written permission. 00023 * 00024 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" 00025 * AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE 00026 * IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE 00027 * DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE 00028 * FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL 00029 * DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR 00030 * SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER 00031 * CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, 00032 * OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE 00033 * OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00034 * 00035 ****************************************************************************** 00036 */ 00037 /* Includes ------------------------------------------------------------------*/ 00038 #include "motorcontrol.h" 00039 00040 /** @addtogroup BSP 00041 * @{ 00042 */ 00043 00044 /** @defgroup MOTOR_CONTROL 00045 * @{ 00046 */ 00047 00048 /** @defgroup MOTOR_CONTROL_Private_Types_Definitions 00049 * @{ 00050 */ 00051 00052 /** 00053 * @} 00054 */ 00055 00056 00057 /** @defgroup MOTOR_CONTROL_Private_Defines 00058 * @{ 00059 */ 00060 /** 00061 * @} 00062 */ 00063 00064 /** @defgroup MOTOR_CONTROL_Private_Constants 00065 * @{ 00066 */ 00067 /// Error when trying to call undefined functions via motorDrvHandle 00068 #define MOTOR_CONTROL_ERROR_0 (0x0800) 00069 00070 /** 00071 * @} 00072 */ 00073 00074 /** @defgroup MOTOR_CONTROL_Private_Macros 00075 * @{ 00076 */ 00077 /// Error when trying to call undefined functions via motorDrvHandle 00078 #define MOTOR_CONTROL_ERROR_UNDEFINED_FUNCTION(errorNb) (BSP_MotorControl_ErrorHandler(MOTOR_CONTROL_ERROR_0|(errorNb))) 00079 00080 /** 00081 * @} 00082 */ 00083 00084 /** @defgroup MOTOR_CONTROL_Private_Variables 00085 * @{ 00086 */ 00087 00088 static motorDrv_t *motorDrvHandle = 0; 00089 static uint16_t MotorControlBoardId; 00090 /** 00091 * @} 00092 */ 00093 00094 /** @defgroup MOTOR_CONTROL_Weak_Private_Functions 00095 * @{ 00096 */ 00097 /// Get motor handle for L6474 00098 __weak motorDrv_t* L6474_GetMotorHandle(void){return ((motorDrv_t* )0);} 00099 /// Get motor handle for Powerstep 00100 __weak motorDrv_t* Powerstep01_GetMotorHandle(void){return ((motorDrv_t* )0);} 00101 00102 00103 /** 00104 * @} 00105 */ 00106 00107 /** @defgroup MOTOR_CONTROL_Private_Functions 00108 * @{ 00109 */ 00110 00111 /******************************************************//** 00112 * @brief Attaches a user callback to the error Handler. 00113 * The call back will be then called each time the library 00114 * detects an error 00115 * @param[in] callback Name of the callback to attach 00116 * to the error Hanlder 00117 * @retval None 00118 **********************************************************/ 00119 void BSP_MotorControl_AttachErrorHandler(void (*callback)(uint16_t)) 00120 { 00121 if ((motorDrvHandle != 0)&&(motorDrvHandle->AttachErrorHandler != 0)) 00122 { 00123 motorDrvHandle->AttachErrorHandler(callback); 00124 } 00125 else 00126 { 00127 MOTOR_CONTROL_ERROR_UNDEFINED_FUNCTION(1); 00128 } 00129 } 00130 00131 /******************************************************//** 00132 * @brief Attaches a user callback to the Flag interrupt Handler. 00133 * The call back will be then called each time the library 00134 * detects a FLAG signal falling edge. 00135 * @param[in] callback Name of the callback to attach 00136 * to the Flag interrupt Hanlder 00137 * @retval None 00138 **********************************************************/ 00139 void BSP_MotorControl_AttachFlagInterrupt(void (*callback)(void)) 00140 { 00141 if ((motorDrvHandle != 0)&&(motorDrvHandle->AttachFlagInterrupt != 0)) 00142 { 00143 motorDrvHandle->AttachFlagInterrupt(callback); 00144 } 00145 else 00146 { 00147 MOTOR_CONTROL_ERROR_UNDEFINED_FUNCTION(2); 00148 } 00149 } 00150 00151 /******************************************************//** 00152 * @brief Attaches a user callback to the Busy interrupt Handler. 00153 * The call back will be then called each time the library 00154 * detects a BUSY signal falling edge. 00155 * @param[in] callback Name of the callback to attach 00156 * to the Busy interrupt Hanlder 00157 * @retval None 00158 **********************************************************/ 00159 void BSP_MotorControl_AttachBusyInterrupt(void (*callback)(void)) 00160 { 00161 if ((motorDrvHandle != 0)&&(motorDrvHandle->AttachBusyInterrupt != 0)) 00162 { 00163 motorDrvHandle->AttachBusyInterrupt(callback); 00164 } 00165 else 00166 { 00167 MOTOR_CONTROL_ERROR_UNDEFINED_FUNCTION(3); 00168 } 00169 } 00170 00171 /******************************************************//** 00172 * @brief Motor control error handler 00173 * @param[in] error number of the error 00174 * @retval None 00175 **********************************************************/ 00176 void BSP_MotorControl_ErrorHandler(uint16_t error) 00177 { 00178 if ((motorDrvHandle != 0)&&(motorDrvHandle->ErrorHandler != 0)) 00179 { 00180 motorDrvHandle->ErrorHandler(error); 00181 } 00182 else 00183 { 00184 while(1) 00185 { 00186 /* Infinite loop as Error handler must be defined*/ 00187 } 00188 } 00189 } 00190 /******************************************************//** 00191 * @brief Initialises the motor driver 00192 * @param[in] id Component Id (L6474, Powerstep01,...) 00193 * @param[in] nbDevices Number of motor devices to use (from 1 to 3) 00194 * @retval None 00195 **********************************************************/ 00196 void BSP_MotorControl_Init(uint16_t id, uint8_t nbDevices) 00197 { 00198 MotorControlBoardId = id; 00199 00200 if (id == BSP_MOTOR_CONTROL_BOARD_ID_L6474) 00201 { 00202 motorDrvHandle = L6474_GetMotorHandle(); 00203 } 00204 else if (id == BSP_MOTOR_CONTROL_BOARD_ID_POWERSTEP01) 00205 { 00206 motorDrvHandle = Powerstep01_GetMotorHandle(); 00207 } 00208 else 00209 { 00210 motorDrvHandle = 0; 00211 } 00212 00213 if ((motorDrvHandle != 0)&&(motorDrvHandle->Init != 0)) 00214 { 00215 motorDrvHandle->Init(nbDevices); 00216 } 00217 else 00218 { 00219 MOTOR_CONTROL_ERROR_UNDEFINED_FUNCTION(4); 00220 } 00221 } 00222 00223 /******************************************************//** 00224 * @brief Handlers of the flag interrupt which calls the user callback (if defined) 00225 * @param None 00226 * @retval None 00227 **********************************************************/ 00228 void BSP_MotorControl_FlagInterruptHandler(void) 00229 { 00230 if ((motorDrvHandle != 0)&&(motorDrvHandle->FlagInterruptHandler != 0)) 00231 { 00232 motorDrvHandle->FlagInterruptHandler(); 00233 } 00234 else 00235 { 00236 MOTOR_CONTROL_ERROR_UNDEFINED_FUNCTION(5); 00237 } 00238 } 00239 /******************************************************//** 00240 * @brief Returns the acceleration of the specified device 00241 * @param[in] deviceId (from 0 to 2) 00242 * @retval Acceleration in pps^2 00243 **********************************************************/ 00244 uint16_t BSP_MotorControl_GetAcceleration(uint8_t deviceId) 00245 { 00246 uint16_t acceleration = 0; 00247 00248 if ((motorDrvHandle != 0)&&(motorDrvHandle->GetAcceleration != 0)) 00249 { 00250 acceleration = motorDrvHandle->GetAcceleration(deviceId); 00251 } 00252 else 00253 { 00254 MOTOR_CONTROL_ERROR_UNDEFINED_FUNCTION(6); 00255 } 00256 return(acceleration); 00257 } 00258 00259 /******************************************************//** 00260 * @brief Get board Id the motor driver 00261 * @param None 00262 * @retval Motor control board Id 00263 **********************************************************/ 00264 uint16_t BSP_MotorControl_GetBoardId(void) 00265 { 00266 return (MotorControlBoardId); 00267 } 00268 /******************************************************//** 00269 * @brief Returns the current speed of the specified device 00270 * @param[in] deviceId (from 0 to 2) 00271 * @retval Speed in pps 00272 **********************************************************/ 00273 uint16_t BSP_MotorControl_GetCurrentSpeed(uint8_t deviceId) 00274 { 00275 uint16_t currentSpeed = 0; 00276 if ((motorDrvHandle != 0)&&(motorDrvHandle->GetCurrentSpeed != 0)) 00277 { 00278 currentSpeed = motorDrvHandle->GetCurrentSpeed(deviceId); 00279 } 00280 else 00281 { 00282 MOTOR_CONTROL_ERROR_UNDEFINED_FUNCTION(7); 00283 } 00284 return(currentSpeed); 00285 } 00286 00287 /******************************************************//** 00288 * @brief Returns the deceleration of the specified device 00289 * @param[in] deviceId (from 0 to 2) 00290 * @retval Deceleration in pps^2 00291 **********************************************************/ 00292 uint16_t BSP_MotorControl_GetDeceleration(uint8_t deviceId) 00293 { 00294 uint16_t deceleration = 0; 00295 00296 if ((motorDrvHandle != 0)&&(motorDrvHandle->GetDeceleration != 0)) 00297 { 00298 deceleration = motorDrvHandle->GetDeceleration(deviceId); 00299 } 00300 else 00301 { 00302 MOTOR_CONTROL_ERROR_UNDEFINED_FUNCTION(8); 00303 } 00304 return(deceleration); 00305 } 00306 00307 /******************************************************//** 00308 * @brief Returns the device state 00309 * @param[in] deviceId (from 0 to 2) 00310 * @retval State (ACCELERATING, DECELERATING, STEADY or INACTIVE) 00311 **********************************************************/ 00312 motorState_t BSP_MotorControl_GetDeviceState(uint8_t deviceId) 00313 { 00314 motorState_t state = INACTIVE; 00315 00316 if ((motorDrvHandle != 0)&&(motorDrvHandle->GetDeviceState != 0)) 00317 { 00318 state = motorDrvHandle->GetDeviceState(deviceId); 00319 } 00320 else 00321 { 00322 MOTOR_CONTROL_ERROR_UNDEFINED_FUNCTION(9); 00323 } 00324 return(state); 00325 } 00326 00327 /******************************************************//** 00328 * @brief Returns the FW version of the library 00329 * @param None 00330 * @retval BSP_MotorControl_FW_VERSION 00331 **********************************************************/ 00332 uint8_t BSP_MotorControl_GetFwVersion(void) 00333 { 00334 uint8_t version = 0; 00335 00336 if ((motorDrvHandle != 0)&&(motorDrvHandle->GetFwVersion != 0)) 00337 { 00338 version = motorDrvHandle->GetFwVersion(); 00339 } 00340 else 00341 { 00342 MOTOR_CONTROL_ERROR_UNDEFINED_FUNCTION(10); 00343 } 00344 return(version); 00345 } 00346 00347 /******************************************************//** 00348 * @brief Returns the mark position of the specified device 00349 * @param[in] deviceId (from 0 to 2) 00350 * @retval Mark register value converted in a 32b signed integer 00351 **********************************************************/ 00352 int32_t BSP_MotorControl_GetMark(uint8_t deviceId) 00353 { 00354 int32_t mark = 0; 00355 00356 if ((motorDrvHandle != 0)&&(motorDrvHandle->GetMark != 0)) 00357 { 00358 mark = motorDrvHandle->GetMark(deviceId); 00359 } 00360 else 00361 { 00362 MOTOR_CONTROL_ERROR_UNDEFINED_FUNCTION(11); 00363 } 00364 return(mark); 00365 } 00366 00367 /******************************************************//** 00368 * @brief Returns the max speed of the specified device 00369 * @param[in] deviceId (from 0 to 2) 00370 * @retval maxSpeed in pps 00371 **********************************************************/ 00372 uint16_t BSP_MotorControl_GetMaxSpeed(uint8_t deviceId) 00373 { 00374 uint16_t maxSpeed = 0; 00375 if ((motorDrvHandle != 0)&&(motorDrvHandle->GetMaxSpeed != 0)) 00376 { 00377 maxSpeed = motorDrvHandle->GetMaxSpeed(deviceId); 00378 } 00379 else 00380 { 00381 MOTOR_CONTROL_ERROR_UNDEFINED_FUNCTION(12); 00382 } 00383 return(maxSpeed); 00384 } 00385 00386 /******************************************************//** 00387 * @brief Returns the min speed of the specified device 00388 * @param[in] deviceId (from 0 to 2) 00389 * @retval minSpeed in pps 00390 **********************************************************/ 00391 uint16_t BSP_MotorControl_GetMinSpeed(uint8_t deviceId) 00392 { 00393 uint16_t minSpeed = 0; 00394 00395 if ((motorDrvHandle != 0)&&(motorDrvHandle->GetMinSpeed != 0)) 00396 { 00397 minSpeed = motorDrvHandle->GetMinSpeed(deviceId); 00398 } 00399 else 00400 { 00401 MOTOR_CONTROL_ERROR_UNDEFINED_FUNCTION(13); 00402 } 00403 return(minSpeed); 00404 } 00405 00406 /******************************************************//** 00407 * @brief Returns the ABS_POSITION of the specified device 00408 * @param[in] deviceId (from 0 to 2) 00409 * @retval ABS_POSITION register value converted in a 32b signed integer 00410 **********************************************************/ 00411 int32_t BSP_MotorControl_GetPosition(uint8_t deviceId) 00412 { 00413 int32_t pos = 0; 00414 00415 if ((motorDrvHandle != 0)&&(motorDrvHandle->GetPosition != 0)) 00416 { 00417 pos = motorDrvHandle->GetPosition(deviceId); 00418 } 00419 else 00420 { 00421 MOTOR_CONTROL_ERROR_UNDEFINED_FUNCTION(14); 00422 } 00423 return(pos); 00424 } 00425 00426 /******************************************************//** 00427 * @brief Requests the motor to move to the home position (ABS_POSITION = 0) 00428 * @param[in] deviceId (from 0 to 2) 00429 * @retval None 00430 **********************************************************/ 00431 void BSP_MotorControl_GoHome(uint8_t deviceId) 00432 { 00433 if ((motorDrvHandle != 0)&&(motorDrvHandle->GoHome != 0)) 00434 { 00435 motorDrvHandle->GoHome(deviceId); 00436 } 00437 else 00438 { 00439 MOTOR_CONTROL_ERROR_UNDEFINED_FUNCTION(15); 00440 } 00441 } 00442 00443 /******************************************************//** 00444 * @brief Requests the motor to move to the mark position 00445 * @param[in] deviceId (from 0 to 2) 00446 * @retval None 00447 **********************************************************/ 00448 void BSP_MotorControl_GoMark(uint8_t deviceId) 00449 { 00450 if ((motorDrvHandle != 0)&&(motorDrvHandle->GoMark != 0)) 00451 { 00452 motorDrvHandle->GoMark(deviceId); 00453 } 00454 else 00455 { 00456 MOTOR_CONTROL_ERROR_UNDEFINED_FUNCTION(16); 00457 } 00458 } 00459 00460 /******************************************************//** 00461 * @brief Requests the motor to move to the specified position 00462 * @param[in] deviceId (from 0 to 2) 00463 * @param[in] targetPosition absolute position in steps 00464 * @retval None 00465 **********************************************************/ 00466 void BSP_MotorControl_GoTo(uint8_t deviceId, int32_t targetPosition) 00467 { 00468 if ((motorDrvHandle != 0)&&(motorDrvHandle->GoTo != 0)) 00469 { 00470 motorDrvHandle->GoTo(deviceId, targetPosition); 00471 } 00472 else 00473 { 00474 MOTOR_CONTROL_ERROR_UNDEFINED_FUNCTION(17); 00475 } 00476 } 00477 00478 /******************************************************//** 00479 * @brief Immediatly stops the motor and disable the power bridge 00480 * @param[in] deviceId (from 0 to 2) 00481 * @retval None 00482 **********************************************************/ 00483 void BSP_MotorControl_HardStop(uint8_t deviceId) 00484 { 00485 if ((motorDrvHandle != 0)&&(motorDrvHandle->HardStop != 0)) 00486 { 00487 motorDrvHandle->HardStop(deviceId); 00488 } 00489 else 00490 { 00491 MOTOR_CONTROL_ERROR_UNDEFINED_FUNCTION(18); 00492 } 00493 } 00494 00495 /******************************************************//** 00496 * @brief Moves the motor of the specified number of steps 00497 * @param[in] deviceId (from 0 to 2) 00498 * @param[in] direction FORWARD or BACKWARD 00499 * @param[in] stepCount Number of steps to perform 00500 * @retval None 00501 **********************************************************/ 00502 void BSP_MotorControl_Move(uint8_t deviceId, motorDir_t direction, uint32_t stepCount) 00503 { 00504 if ((motorDrvHandle != 0)&&(motorDrvHandle->Move != 0)) 00505 { 00506 motorDrvHandle->Move(deviceId, direction, stepCount); 00507 } 00508 else 00509 { 00510 MOTOR_CONTROL_ERROR_UNDEFINED_FUNCTION(19); 00511 } 00512 } 00513 00514 /******************************************************//** 00515 * @brief Resets all motor driver devices 00516 * @param None 00517 * @retval None 00518 **********************************************************/ 00519 void BSP_MotorControl_ResetAllDevices(void) 00520 { 00521 if ((motorDrvHandle != 0)&&(motorDrvHandle->ResetAllDevices != 0)) 00522 { 00523 motorDrvHandle->ResetAllDevices(); 00524 } 00525 else 00526 { 00527 MOTOR_CONTROL_ERROR_UNDEFINED_FUNCTION(20); 00528 } 00529 } 00530 00531 /******************************************************//** 00532 * @brief Runs the motor. It will accelerate from the min 00533 * speed up to the max speed by using the device acceleration. 00534 * @param[in] deviceId (from 0 to 2) 00535 * @param[in] direction FORWARD or BACKWARD 00536 * @retval None 00537 **********************************************************/ 00538 void BSP_MotorControl_Run(uint8_t deviceId, motorDir_t direction) 00539 { 00540 if ((motorDrvHandle != 0)&&(motorDrvHandle->Run != 0)) 00541 { 00542 motorDrvHandle->Run(deviceId, direction); 00543 } 00544 else 00545 { 00546 MOTOR_CONTROL_ERROR_UNDEFINED_FUNCTION(21); 00547 } 00548 } 00549 /******************************************************//** 00550 * @brief Changes the acceleration of the specified device 00551 * @param[in] deviceId (from 0 to 2) 00552 * @param[in] newAcc New acceleration to apply in pps^2 00553 * @retval true if the command is successfully executed, else false 00554 * @note The command is not performed is the device is executing 00555 * a MOVE or GOTO command (but it can be used during a RUN command) 00556 **********************************************************/ 00557 bool BSP_MotorControl_SetAcceleration(uint8_t deviceId,uint16_t newAcc) 00558 { 00559 bool status = FALSE; 00560 if ((motorDrvHandle != 0)&&(motorDrvHandle->SetAcceleration != 0)) 00561 { 00562 status = motorDrvHandle->SetAcceleration(deviceId, newAcc); 00563 } 00564 else 00565 { 00566 MOTOR_CONTROL_ERROR_UNDEFINED_FUNCTION(22); 00567 } 00568 return (status); 00569 } 00570 00571 /******************************************************//** 00572 * @brief Changes the deceleration of the specified device 00573 * @param[in] deviceId (from 0 to 2) 00574 * @param[in] newDec New deceleration to apply in pps^2 00575 * @retval true if the command is successfully executed, else false 00576 * @note The command is not performed is the device is executing 00577 * a MOVE or GOTO command (but it can be used during a RUN command) 00578 **********************************************************/ 00579 bool BSP_MotorControl_SetDeceleration(uint8_t deviceId, uint16_t newDec) 00580 { 00581 bool status = FALSE; 00582 if ((motorDrvHandle != 0)&&(motorDrvHandle->SetDeceleration != 0)) 00583 { 00584 status = motorDrvHandle->SetDeceleration(deviceId, newDec); 00585 } 00586 else 00587 { 00588 MOTOR_CONTROL_ERROR_UNDEFINED_FUNCTION(23); 00589 } 00590 return (status); 00591 } 00592 00593 /******************************************************//** 00594 * @brief Set current position to be the Home position (ABS pos set to 0) 00595 * @param[in] deviceId (from 0 to 2) 00596 * @retval None 00597 **********************************************************/ 00598 void BSP_MotorControl_SetHome(uint8_t deviceId) 00599 { 00600 if ((motorDrvHandle != 0)&&(motorDrvHandle->SetHome != 0)) 00601 { 00602 motorDrvHandle->SetHome(deviceId); 00603 } 00604 else 00605 { 00606 MOTOR_CONTROL_ERROR_UNDEFINED_FUNCTION(24); 00607 } 00608 } 00609 00610 /******************************************************//** 00611 * @brief Sets current position to be the Mark position 00612 * @param[in] deviceId (from 0 to 2) 00613 * @retval None 00614 **********************************************************/ 00615 void BSP_MotorControl_SetMark(uint8_t deviceId) 00616 { 00617 if ((motorDrvHandle != 0)&&(motorDrvHandle->SetMark != 0)) 00618 { 00619 motorDrvHandle->SetMark(deviceId); 00620 } 00621 else 00622 { 00623 MOTOR_CONTROL_ERROR_UNDEFINED_FUNCTION(25); 00624 } 00625 } 00626 00627 /******************************************************//** 00628 * @brief Changes the max speed of the specified device 00629 * @param[in] deviceId (from 0 to 2) 00630 * @param[in] newMaxSpeed New max speed to apply in pps 00631 * @retval true if the command is successfully executed, else false 00632 * @note The command is not performed is the device is executing 00633 * a MOVE or GOTO command (but it can be used during a RUN command). 00634 **********************************************************/ 00635 bool BSP_MotorControl_SetMaxSpeed(uint8_t deviceId, uint16_t newMaxSpeed) 00636 { 00637 bool status = FALSE; 00638 if ((motorDrvHandle != 0)&&(motorDrvHandle->SetMaxSpeed != 0)) 00639 { 00640 status = motorDrvHandle->SetMaxSpeed(deviceId, newMaxSpeed); 00641 } 00642 else 00643 { 00644 MOTOR_CONTROL_ERROR_UNDEFINED_FUNCTION(26); 00645 } 00646 return (status); 00647 } 00648 00649 /******************************************************//** 00650 * @brief Changes the min speed of the specified device 00651 * @param[in] deviceId (from 0 to 2) 00652 * @param[in] newMinSpeed New min speed to apply in pps 00653 * @retval true if the command is successfully executed, else false 00654 * @note The command is not performed is the device is executing 00655 * a MOVE or GOTO command (but it can be used during a RUN command). 00656 **********************************************************/ 00657 bool BSP_MotorControl_SetMinSpeed(uint8_t deviceId, uint16_t newMinSpeed) 00658 { 00659 bool status = FALSE; 00660 if ((motorDrvHandle != 0)&&(motorDrvHandle->SetMinSpeed != 0)) 00661 { 00662 status = motorDrvHandle->SetMinSpeed(deviceId, newMinSpeed); 00663 } 00664 else 00665 { 00666 MOTOR_CONTROL_ERROR_UNDEFINED_FUNCTION(27); 00667 } 00668 00669 return (status); 00670 } 00671 00672 /******************************************************//** 00673 * @brief Stops the motor by using the device deceleration 00674 * @param[in] deviceId (from 0 to 2) 00675 * @retval true if the command is successfully executed, else false 00676 * @note The command is not performed is the device is in INACTIVE state. 00677 **********************************************************/ 00678 bool BSP_MotorControl_SoftStop(uint8_t deviceId) 00679 { 00680 bool status = FALSE; 00681 if ((motorDrvHandle != 0)&&(motorDrvHandle->SoftStop != 0)) 00682 { 00683 status = motorDrvHandle->SoftStop(deviceId); 00684 } 00685 else 00686 { 00687 MOTOR_CONTROL_ERROR_UNDEFINED_FUNCTION(28); 00688 } 00689 return (status); 00690 } 00691 00692 /******************************************************//** 00693 * @brief Handles the device state machine at each ste 00694 * @param[in] deviceId (from 0 to 2) 00695 * @retval None 00696 * @note Must only be called by the timer ISR 00697 **********************************************************/ 00698 void BSP_MotorControl_StepClockHandler(uint8_t deviceId) 00699 { 00700 if ((motorDrvHandle != 0)&&(motorDrvHandle->StepClockHandler != 0)) 00701 { 00702 motorDrvHandle->StepClockHandler(deviceId); 00703 } 00704 else 00705 { 00706 MOTOR_CONTROL_ERROR_UNDEFINED_FUNCTION(29); 00707 } 00708 } 00709 /******************************************************//** 00710 * @brief Locks until the device state becomes Inactive 00711 * @param[in] deviceId (from 0 to 2) 00712 * @retval None 00713 **********************************************************/ 00714 void BSP_MotorControl_WaitWhileActive(uint8_t deviceId) 00715 { 00716 if ((motorDrvHandle != 0)&&(motorDrvHandle->WaitWhileActive != 0)) 00717 { 00718 motorDrvHandle->WaitWhileActive(deviceId); 00719 } 00720 else 00721 { 00722 MOTOR_CONTROL_ERROR_UNDEFINED_FUNCTION(30); 00723 } 00724 } 00725 00726 /** 00727 * @} 00728 */ 00729 00730 /** @defgroup BSP_MotorControl_Control_Functions 00731 * @{ 00732 */ 00733 00734 /******************************************************//** 00735 * @brief Issue the Disable command to the motor driver of the specified device 00736 * @param[in] deviceId (from 0 to 2) 00737 * @retval None 00738 **********************************************************/ 00739 void BSP_MotorControl_CmdDisable(uint8_t deviceId) 00740 { 00741 if ((motorDrvHandle != 0)&&(motorDrvHandle->CmdDisable != 0)) 00742 { 00743 motorDrvHandle->CmdDisable(deviceId); 00744 } 00745 else 00746 { 00747 MOTOR_CONTROL_ERROR_UNDEFINED_FUNCTION(31); 00748 } 00749 } 00750 00751 /******************************************************//** 00752 * @brief Issues the Enable command to the motor driver of the specified device 00753 * @param[in] deviceId (from 0 to 2) 00754 * @retval None 00755 **********************************************************/ 00756 void BSP_MotorControl_CmdEnable(uint8_t deviceId) 00757 { 00758 if ((motorDrvHandle != 0)&&(motorDrvHandle->CmdEnable != 0)) 00759 { 00760 motorDrvHandle->CmdEnable(deviceId); 00761 } 00762 else 00763 { 00764 MOTOR_CONTROL_ERROR_UNDEFINED_FUNCTION(32); 00765 } 00766 } 00767 00768 /******************************************************//** 00769 * @brief Issues the GetParam command to the motor driver of the specified device 00770 * @param[in] deviceId (from 0 to 2) 00771 * @param[in] param Register adress (BSP_MotorControl_ABS_POS, BSP_MotorControl_MARK,...) 00772 * @retval Register value 00773 **********************************************************/ 00774 uint32_t BSP_MotorControl_CmdGetParam(uint8_t deviceId, 00775 uint32_t param) 00776 { 00777 uint32_t value = 0; 00778 if ((motorDrvHandle != 0)&&(motorDrvHandle->CmdGetParam != 0)) 00779 { 00780 value = motorDrvHandle->CmdGetParam(deviceId, param); 00781 } 00782 else 00783 { 00784 MOTOR_CONTROL_ERROR_UNDEFINED_FUNCTION(33); 00785 } 00786 return (value); 00787 } 00788 00789 /******************************************************//** 00790 * @brief Issues the GetStatus command to the motor driver of the specified device 00791 * @param[in] deviceId (from 0 to 2) 00792 * @retval Status Register value 00793 * @note Once the GetStatus command is performed, the flags of the status register 00794 * are reset. This is not the case when the status register is read with the 00795 * GetParam command (via the functions ReadStatusRegister or CmdGetParam). 00796 **********************************************************/ 00797 uint16_t BSP_MotorControl_CmdGetStatus(uint8_t deviceId) 00798 { 00799 uint16_t status = 0; 00800 if ((motorDrvHandle != 0)&&(motorDrvHandle->CmdGetStatus != 0)) 00801 { 00802 status = motorDrvHandle->CmdGetStatus(deviceId); 00803 } 00804 else 00805 { 00806 MOTOR_CONTROL_ERROR_UNDEFINED_FUNCTION(34); 00807 } 00808 return (status); 00809 } 00810 00811 /******************************************************//** 00812 * @brief Issues the Nop command to the motor driver of the specified device 00813 * @param[in] deviceId (from 0 to 2) 00814 * @retval None 00815 **********************************************************/ 00816 void BSP_MotorControl_CmdNop(uint8_t deviceId) 00817 { 00818 if ((motorDrvHandle != 0)&&(motorDrvHandle->CmdNop != 0)) 00819 { 00820 motorDrvHandle->CmdNop(deviceId); 00821 } 00822 else 00823 { 00824 MOTOR_CONTROL_ERROR_UNDEFINED_FUNCTION(35); 00825 } 00826 } 00827 00828 /******************************************************//** 00829 * @brief Issues the SetParam command to the motor driver of the specified device 00830 * @param[in] deviceId (from 0 to 2) 00831 * @param[in] param Register adress (BSP_MotorControl_ABS_POS, BSP_MotorControl_MARK,...) 00832 * @param[in] value Value to set in the register 00833 * @retval None 00834 **********************************************************/ 00835 void BSP_MotorControl_CmdSetParam(uint8_t deviceId, 00836 uint32_t param, 00837 uint32_t value) 00838 { 00839 if ((motorDrvHandle != 0)&&(motorDrvHandle->CmdSetParam != 0)) 00840 { 00841 motorDrvHandle->CmdSetParam(deviceId, param, value); 00842 } 00843 else 00844 { 00845 MOTOR_CONTROL_ERROR_UNDEFINED_FUNCTION(36); 00846 } 00847 } 00848 00849 /******************************************************//** 00850 * @brief Reads the Status Register value 00851 * @param[in] deviceId (from 0 to 2) 00852 * @retval Status register valued 00853 * @note The status register flags are not cleared 00854 * at the difference with CmdGetStatus() 00855 **********************************************************/ 00856 uint16_t BSP_MotorControl_ReadStatusRegister(uint8_t deviceId) 00857 { 00858 uint16_t status = 0; 00859 if ((motorDrvHandle != 0)&&(motorDrvHandle->ReadStatusRegister != 0)) 00860 { 00861 status = motorDrvHandle->ReadStatusRegister(deviceId); 00862 } 00863 else 00864 { 00865 MOTOR_CONTROL_ERROR_UNDEFINED_FUNCTION(37); 00866 } 00867 return (status); 00868 } 00869 00870 /******************************************************//** 00871 * @brief Releases the motor driver (pin set to High) of all devices 00872 * @param None 00873 * @retval None 00874 **********************************************************/ 00875 void BSP_MotorControl_ReleaseReset(void) 00876 { 00877 if ((motorDrvHandle != 0)&&(motorDrvHandle->ReleaseReset != 0)) 00878 { 00879 motorDrvHandle->ReleaseReset(); 00880 } 00881 else 00882 { 00883 MOTOR_CONTROL_ERROR_UNDEFINED_FUNCTION(38); 00884 } 00885 } 00886 00887 /******************************************************//** 00888 * @brief Resets the motor driver (reset pin set to low) of all devices 00889 * @param None 00890 * @retval None 00891 **********************************************************/ 00892 void BSP_MotorControl_Reset(void) 00893 { 00894 if ((motorDrvHandle != 0)&&(motorDrvHandle->Reset != 0)) 00895 { 00896 motorDrvHandle->Reset(); 00897 } 00898 else 00899 { 00900 MOTOR_CONTROL_ERROR_UNDEFINED_FUNCTION(39); 00901 } 00902 } 00903 00904 /******************************************************//** 00905 * @brief Set the stepping mode 00906 * @param[in] deviceId (from 0 to 2) 00907 * @param[in] stepMod from full step to 1/16 microstep as specified in enum BSP_MotorControl_STEP_SEL_t 00908 * @retval None 00909 **********************************************************/ 00910 void BSP_MotorControl_SelectStepMode(uint8_t deviceId, motorStepMode_t stepMod) 00911 { 00912 if ((motorDrvHandle != 0)&&(motorDrvHandle->SelectStepMode != 0)) 00913 { 00914 motorDrvHandle->SelectStepMode(deviceId, stepMod); 00915 } 00916 else 00917 { 00918 MOTOR_CONTROL_ERROR_UNDEFINED_FUNCTION(40); 00919 } 00920 } 00921 00922 /******************************************************//** 00923 * @brief Specifies the direction 00924 * @param[in] deviceId (from 0 to 2) 00925 * @param[in] dir FORWARD or BACKWARD 00926 * @note The direction change is only applied if the device 00927 * is in INACTIVE state 00928 * @retval None 00929 **********************************************************/ 00930 void BSP_MotorControl_SetDirection(uint8_t deviceId, motorDir_t dir) 00931 { 00932 if ((motorDrvHandle != 0)&&(motorDrvHandle->SetDirection != 0)) 00933 { 00934 motorDrvHandle->SetDirection(deviceId, dir); 00935 } 00936 else 00937 { 00938 MOTOR_CONTROL_ERROR_UNDEFINED_FUNCTION(41); 00939 } 00940 } 00941 00942 /******************************************************//** 00943 * @brief Issues Go To Dir command 00944 * @param[in] deviceId (from 0 to MAX_NUMBER_OF_DEVICES-1 ) 00945 * @param[in] dir movement direction 00946 * @param[in] abs_pos absolute position where requested to move 00947 * @retval None 00948 **********************************************************/ 00949 void BSP_MotorControl_CmdGoToDir(uint8_t deviceId, motorDir_t dir, int32_t abs_pos) 00950 { 00951 if ((motorDrvHandle != 0)&&(motorDrvHandle->CmdGoToDir != 0)) 00952 { 00953 motorDrvHandle->CmdGoToDir(deviceId, dir, abs_pos); 00954 } 00955 else 00956 { 00957 MOTOR_CONTROL_ERROR_UNDEFINED_FUNCTION(42); 00958 } 00959 } 00960 00961 /******************************************************//** 00962 * @brief Checks if at least one device is busy by checking 00963 * busy pin position. 00964 * The busy pin is shared between all devices. 00965 * @param None 00966 * @retval One if at least one device is busy, otherwise zero 00967 **********************************************************/ 00968 uint8_t BSP_MotorControl_CheckBusyHw(void) 00969 { 00970 uint8_t value = 0; 00971 if ((motorDrvHandle != 0)&&(motorDrvHandle->CheckBusyHw != 0)) 00972 { 00973 value = motorDrvHandle->CheckBusyHw(); 00974 } 00975 else 00976 { 00977 MOTOR_CONTROL_ERROR_UNDEFINED_FUNCTION(43); 00978 } 00979 return (value); 00980 } 00981 00982 /******************************************************//** 00983 * @brief Checks if at least one device has an alarm flag set 00984 * by reading flag pin position. 00985 * The flag pin is shared between all devices. 00986 * @param None 00987 * @retval One if at least one device has an alarm flag set , 00988 * otherwise zero 00989 **********************************************************/ 00990 uint8_t BSP_MotorControl_CheckStatusHw(void) 00991 { 00992 uint8_t value = 0; 00993 if ((motorDrvHandle != 0)&&(motorDrvHandle->CheckStatusHw != 0)) 00994 { 00995 value = motorDrvHandle->CheckStatusHw(); 00996 } 00997 else 00998 { 00999 MOTOR_CONTROL_ERROR_UNDEFINED_FUNCTION(44); 01000 } 01001 return (value); 01002 } 01003 01004 /******************************************************//** 01005 * @brief Issues Go Until command 01006 * @param[in] deviceId (from 0 to MAX_NUMBER_OF_DEVICES-1 ) 01007 * @param[in] action ACTION_RESET or ACTION_COPY 01008 * @param[in] dir movement direction 01009 * @param[in] speed 01010 * @retval None 01011 **********************************************************/ 01012 void BSP_MotorControl_CmdGoUntil(uint8_t deviceId, motorAction_t action, motorDir_t dir, uint32_t speed) 01013 { 01014 if ((motorDrvHandle != 0)&&(motorDrvHandle->CmdGoUntil != 0)) 01015 { 01016 motorDrvHandle->CmdGoUntil(deviceId, action, dir, speed); 01017 } 01018 else 01019 { 01020 MOTOR_CONTROL_ERROR_UNDEFINED_FUNCTION(45); 01021 } 01022 } 01023 01024 /******************************************************//** 01025 * @brief Issues Hard HiZ command 01026 * @param[in] deviceId (from 0 to MAX_NUMBER_OF_DEVICES-1 ) 01027 * @retval None 01028 **********************************************************/ 01029 void BSP_MotorControl_CmdHardHiZ(uint8_t deviceId) 01030 { 01031 if ((motorDrvHandle != 0)&&(motorDrvHandle->CmdHardHiZ != 0)) 01032 { 01033 motorDrvHandle->CmdHardHiZ(deviceId); 01034 } 01035 else 01036 { 01037 MOTOR_CONTROL_ERROR_UNDEFINED_FUNCTION(46); 01038 } 01039 } 01040 01041 /******************************************************//** 01042 * @brief Issues Release SW command 01043 * @param[in] deviceId (from 0 to MAX_NUMBER_OF_DEVICES-1 ) 01044 * @param[in] action 01045 * @param[in] dir movement direction 01046 * @retval None 01047 **********************************************************/ 01048 void BSP_MotorControl_CmdReleaseSw(uint8_t deviceId, motorAction_t action, motorDir_t dir) 01049 { 01050 if ((motorDrvHandle != 0)&&(motorDrvHandle->CmdReleaseSw != 0)) 01051 { 01052 motorDrvHandle->CmdReleaseSw(deviceId, action, dir); 01053 } 01054 else 01055 { 01056 MOTOR_CONTROL_ERROR_UNDEFINED_FUNCTION(47); 01057 } 01058 } 01059 01060 /******************************************************//** 01061 * @brief Issues Reset Device command 01062 * @param[in] deviceId (from 0 to MAX_NUMBER_OF_DEVICES-1 ) 01063 * @retval None 01064 **********************************************************/ 01065 void BSP_MotorControl_CmdResetDevice(uint8_t deviceId) 01066 { 01067 if ((motorDrvHandle != 0)&&(motorDrvHandle->CmdResetDevice != 0)) 01068 { 01069 motorDrvHandle->CmdResetDevice(deviceId); 01070 } 01071 else 01072 { 01073 MOTOR_CONTROL_ERROR_UNDEFINED_FUNCTION(48); 01074 } 01075 } 01076 01077 /******************************************************//** 01078 * @brief Issues Reset Pos command 01079 * @param[in] deviceId (from 0 to MAX_NUMBER_OF_DEVICES-1 ) 01080 * @retval None 01081 **********************************************************/ 01082 void BSP_MotorControl_CmdResetPos(uint8_t deviceId) 01083 { 01084 if ((motorDrvHandle != 0)&&(motorDrvHandle->CmdResetPos != 0)) 01085 { 01086 motorDrvHandle->CmdResetPos(deviceId); 01087 } 01088 else 01089 { 01090 MOTOR_CONTROL_ERROR_UNDEFINED_FUNCTION(49); 01091 } 01092 } 01093 01094 /******************************************************//** 01095 * @brief Issues Run command 01096 * @param[in] deviceId (from 0 to MAX_NUMBER_OF_DEVICES-1 ) 01097 * @param[in] dir Movement direction (FORWARD, BACKWARD) 01098 * @param[in] speed in steps/s 01099 * @retval None 01100 **********************************************************/ 01101 void BSP_MotorControl_CmdRun(uint8_t deviceId, motorDir_t dir, uint32_t speed) 01102 { 01103 if ((motorDrvHandle != 0)&&(motorDrvHandle->CmdRun != 0)) 01104 { 01105 motorDrvHandle->CmdRun(deviceId, dir, speed); 01106 } 01107 else 01108 { 01109 MOTOR_CONTROL_ERROR_UNDEFINED_FUNCTION(50); 01110 } 01111 } 01112 01113 /******************************************************//** 01114 * @brief Issues Soft HiZ command 01115 * @param[in] deviceId (from 0 to MAX_NUMBER_OF_DEVICES-1 ) 01116 * @retval None 01117 **********************************************************/ 01118 void BSP_MotorControl_CmdSoftHiZ(uint8_t deviceId) 01119 { 01120 if ((motorDrvHandle != 0)&&(motorDrvHandle->CmdSoftHiZ != 0)) 01121 { 01122 motorDrvHandle->CmdSoftHiZ(deviceId); 01123 } 01124 else 01125 { 01126 MOTOR_CONTROL_ERROR_UNDEFINED_FUNCTION(51); 01127 } 01128 } 01129 01130 /******************************************************//** 01131 * @brief Issues Step Clock command 01132 * @param[in] deviceId (from 0 to MAX_NUMBER_OF_DEVICES-1 ) 01133 * @param[in] dir Movement direction (FORWARD, BACKWARD) 01134 * @retval None 01135 **********************************************************/ 01136 void BSP_MotorControl_CmdStepClock(uint8_t deviceId, motorDir_t dir) 01137 { 01138 if ((motorDrvHandle != 0)&&(motorDrvHandle->CmdStepClock != 0)) 01139 { 01140 motorDrvHandle->CmdStepClock(deviceId, dir); 01141 } 01142 else 01143 { 01144 MOTOR_CONTROL_ERROR_UNDEFINED_FUNCTION(52); 01145 } 01146 } 01147 01148 /******************************************************//** 01149 * @brief Fetch and clear status flags of all devices 01150 * by issuing a GET_STATUS command simultaneously 01151 * to all devices. 01152 * Then, the fetched status of each device can be retrieved 01153 * by using the BSP_MotorControl_GetFetchedStatus function 01154 * provided there is no other calls to functions which 01155 * use the SPI in between. 01156 * @param None 01157 * @retval None 01158 **********************************************************/ 01159 void BSP_MotorControl_FetchAndClearAllStatus(void) 01160 { 01161 if ((motorDrvHandle != 0)&&(motorDrvHandle->FetchAndClearAllStatus != 0)) 01162 { 01163 motorDrvHandle->FetchAndClearAllStatus(); 01164 } 01165 else 01166 { 01167 MOTOR_CONTROL_ERROR_UNDEFINED_FUNCTION(53); 01168 } 01169 } 01170 01171 /******************************************************//** 01172 * @brief Get the value of the STATUS register which was 01173 * fetched by using BSP_MotorControl_FetchAndClearAllStatus. 01174 * The fetched values are available as long as there 01175 * no other calls to functions which use the SPI. 01176 * @param[in] deviceId (from 0 to MAX_NUMBER_OF_DEVICES-1 ) 01177 * @retval Last fetched value of the STATUS register 01178 **********************************************************/ 01179 uint16_t BSP_MotorControl_GetFetchedStatus(uint8_t deviceId) 01180 { 01181 uint16_t value = 0; 01182 if ((motorDrvHandle != 0)&&(motorDrvHandle->GetFetchedStatus != 0)) 01183 { 01184 value = motorDrvHandle->GetFetchedStatus(deviceId); 01185 } 01186 else 01187 { 01188 MOTOR_CONTROL_ERROR_UNDEFINED_FUNCTION(54); 01189 } 01190 return (value); 01191 } 01192 01193 /******************************************************//** 01194 * @brief Return the number of devices in the daisy chain 01195 * @param None 01196 * @retval number of devices from 1 to MAX_NUMBER_OF_DEVICES 01197 **********************************************************/ 01198 uint8_t BSP_MotorControl_GetNbDevices(void) 01199 { 01200 uint8_t value = 0; 01201 if ((motorDrvHandle != 0)&&(motorDrvHandle->GetNbDevices != 0)) 01202 { 01203 value = motorDrvHandle->GetNbDevices(); 01204 } 01205 else 01206 { 01207 MOTOR_CONTROL_ERROR_UNDEFINED_FUNCTION(55); 01208 } 01209 return (value); 01210 } 01211 01212 /******************************************************//** 01213 * @brief Checks if the specified device is busy 01214 * by reading the Busy flag bit ot its status Register 01215 * @param[in] deviceId (from 0 to MAX_NUMBER_OF_DEVICES-1 ) 01216 * @retval true if device is busy, false zero 01217 **********************************************************/ 01218 bool BSP_MotorControl_IsDeviceBusy(uint8_t deviceId) 01219 { 01220 bool value = 0; 01221 if ((motorDrvHandle != 0)&&(motorDrvHandle->IsDeviceBusy != 0)) 01222 { 01223 value = motorDrvHandle->IsDeviceBusy(deviceId); 01224 } 01225 else 01226 { 01227 MOTOR_CONTROL_ERROR_UNDEFINED_FUNCTION(56); 01228 } 01229 return (value); 01230 } 01231 01232 /******************************************************//** 01233 * @brief Sends commands stored in the queue by previously 01234 * Powerstep01_QueueCommands 01235 * @param None 01236 * @retval None 01237 *********************************************************/ 01238 void BSP_MotorControl_SendQueuedCommands(void) 01239 { 01240 if ((motorDrvHandle != 0)&&(motorDrvHandle->SendQueuedCommands != 0)) 01241 { 01242 motorDrvHandle->SendQueuedCommands(); 01243 } 01244 else 01245 { 01246 MOTOR_CONTROL_ERROR_UNDEFINED_FUNCTION(57); 01247 } 01248 } 01249 01250 /******************************************************//** 01251 * @brief Put commands in queue before synchronous sending 01252 * done by calling BSP_MotorControl_SendQueuedCommands. 01253 * Any call to functions that use the SPI between the calls of 01254 * BSP_MotorControl_QueueCommands and BSP_MotorControl_SendQueuedCommands 01255 * will corrupt the queue. 01256 * A command for each device of the daisy chain must be 01257 * specified before calling BSP_MotorControl_SendQueuedCommands. 01258 * @param[in] deviceId deviceId (from 0 to MAX_NUMBER_OF_DEVICES-1 ) 01259 * @param[in] param Command to queue (all BSP_MotorControl commmands 01260 * except SET_PARAM, GET_PARAM, GET_STATUS) 01261 * @param[in] value argument of the command to queue 01262 * @retval None 01263 *********************************************************/ 01264 void BSP_MotorControl_QueueCommands(uint8_t deviceId, uint8_t param, uint32_t value) 01265 { 01266 if ((motorDrvHandle != 0)&&(motorDrvHandle->QueueCommands != 0)) 01267 { 01268 motorDrvHandle->QueueCommands(deviceId, param, value); 01269 } 01270 else 01271 { 01272 MOTOR_CONTROL_ERROR_UNDEFINED_FUNCTION(58); 01273 } 01274 } 01275 01276 /******************************************************//** 01277 * @brief Locks until all devices become not busy 01278 * @param None 01279 * @retval None 01280 **********************************************************/ 01281 void BSP_MotorControl_WaitForAllDevicesNotBusy(void) 01282 { 01283 if ((motorDrvHandle != 0)&&(motorDrvHandle->WaitForAllDevicesNotBusy != 0)) 01284 { 01285 motorDrvHandle->WaitForAllDevicesNotBusy(); 01286 } 01287 else 01288 { 01289 MOTOR_CONTROL_ERROR_UNDEFINED_FUNCTION(59); 01290 } 01291 } 01292 01293 /******************************************************//** 01294 * @brief Handler of the busy interrupt which calls the user callback (if defined) 01295 * @param None 01296 * @retval None 01297 **********************************************************/ 01298 void BSP_MotorControl_BusyInterruptHandler(void) 01299 { 01300 if ((motorDrvHandle != 0)&&(motorDrvHandle->BusyInterruptHandler != 0)) 01301 { 01302 motorDrvHandle->BusyInterruptHandler(); 01303 } 01304 else 01305 { 01306 MOTOR_CONTROL_ERROR_UNDEFINED_FUNCTION(60); 01307 } 01308 } 01309 01310 /******************************************************//** 01311 * @brief Issues PowerStep01 Soft Stop command 01312 * @param[in] deviceId (from 0 to MAX_NUMBER_OF_DEVICES-1 ) 01313 * @retval None 01314 **********************************************************/ 01315 void BSP_MotorControl_CmdSoftStop(uint8_t deviceId) 01316 { 01317 if ((motorDrvHandle != 0)&&(motorDrvHandle->CmdSoftStop != 0)) 01318 { 01319 motorDrvHandle->CmdSoftStop(deviceId); 01320 } 01321 else 01322 { 01323 MOTOR_CONTROL_ERROR_UNDEFINED_FUNCTION(61); 01324 } 01325 } 01326 01327 /** 01328 * @} 01329 */ 01330 01331 /** 01332 * @} 01333 */ 01334 01335 /** 01336 * @} 01337 */ 01338 01339 /************************ (C) COPYRIGHT STMicroelectronics *****END OF FILE****/
Generated on Tue Jul 12 2022 22:53:31 by
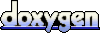