Class library for a Topway LM6029ACW graphics LCD.
LM6029ACW Class Reference
Class library for a Topway LM6029ACW graphics LCD. More...
#include <LM6029ACW.h>
Public Member Functions | |
LM6029ACW (PinName CS1, PinName RES, PinName RS, PinName WR, PinName RD, PinName DB7, PinName DB6, PinName DB5, PinName DB4, PinName DB3, PinName DB2, PinName DB1, PinName DB0) | |
Create a LM6029ACW object for a graphics LCD connected to the specified pins. | |
void | ClrScr (unsigned char color) |
Clears the lcd by clearing or filling all the pixels. | |
void | PowerSave (void) |
Puts the lcd in power save mode - display off. | |
void | PowerActive (void) |
Puts the lcd in normal power mode - display on. | |
void | DrawPixel (unsigned char x, unsigned char y, unsigned char color) |
Draws a pixel on the lcd at the specified xy position. | |
void | DrawLine (int x1, int y1, int x2, int y2, unsigned int color) |
Draws a line on the lcd from x1,y1 to x2,y2. | |
void | DrawCircle (unsigned char x, unsigned char y, unsigned char radius, unsigned char fill, unsigned char color) |
Draws a circle on the lcd at x,y with the option to fill it. | |
void | GotoXY (unsigned char x, unsigned char y) |
Changes the position on the lcd of the current character cursor from where the next characters will be printed. | |
void | PutStr (char *str, unsigned char color) |
Prints a string on the lcd at the current character cursor position. | |
void | PutChar (unsigned char c, unsigned char color) |
Prints a character on the lcd at the current character cursor position. |
Detailed Description
Class library for a Topway LM6029ACW graphics LCD.
Example:
#include "mbed.h" #include "LM6029ACW.h" LM6029ACW lcd(PD_7, PE_2, PD_6, PD_3, PD_2, PC_15, PC_14, PC_13, PC_11, PC_9, PC_8, PC_6, PC_2); // CS1 RES RS WR RD DB7 DB6 DB5 DB4 DB3 DB2 DB1 DB0 DigitalOut backlight(PB_7); int main() { backlight = 0; //turn on the lcd's backlight while(1) { lcd.GotoXY(4, 3); //move cursor to row, col lcd.ClrScr(0); //clear all the pixels on the display lcd.PutStr("Hello World!", 1); //print text in black pixels wait(2.0); lcd.GotoXY(4, 3); //move cursor to row, col lcd.ClrScr(1); //fill all the pixels on the display lcd.PutStr("Hello World!", 0); //print text in clear pixels wait(2.0); } }
Definition at line 62 of file LM6029ACW.h.
Constructor & Destructor Documentation
LM6029ACW | ( | PinName | CS1, |
PinName | RES, | ||
PinName | RS, | ||
PinName | WR, | ||
PinName | RD, | ||
PinName | DB7, | ||
PinName | DB6, | ||
PinName | DB5, | ||
PinName | DB4, | ||
PinName | DB3, | ||
PinName | DB2, | ||
PinName | DB1, | ||
PinName | DB0 | ||
) |
Create a LM6029ACW object for a graphics LCD connected to the specified pins.
- Parameters:
-
CS1 Chip Select pin used to connect to LM6029ACW's CS1 pin RES Reset pin used to connect to LM6029ACW's RES pin RS Register Select pin used to connect to LM6029ACW's RS pin WR Write pin used to connect to LM6029ACW's WR pin RD Read pin used to connect to LM6029ACW's RD pin DB7-DB0 Data Bus pin useds to connect to LM6029ACW's DB7 - DB0 pins
Definition at line 7 of file LM6029ACW.cpp.
Member Function Documentation
void ClrScr | ( | unsigned char | color ) |
Clears the lcd by clearing or filling all the pixels.
- Parameters:
-
color Bit value; clear pixels(0) or fill pixels(1)
Definition at line 15 of file LM6029ACW.cpp.
void DrawCircle | ( | unsigned char | x, |
unsigned char | y, | ||
unsigned char | radius, | ||
unsigned char | fill, | ||
unsigned char | color | ||
) |
Draws a circle on the lcd at x,y with the option to fill it.
- Parameters:
-
x x position (0 - 127) y y position (0 - 63) radius radius of the circle (0 - 127) fill circle must be filled (=1) or not filled (=0) color mode of the pixel; cleared(0), filled(1)
Definition at line 151 of file LM6029ACW.cpp.
void DrawLine | ( | int | x1, |
int | y1, | ||
int | x2, | ||
int | y2, | ||
unsigned int | color | ||
) |
Draws a line on the lcd from x1,y1 to x2,y2.
- Parameters:
-
x1 x1 position (0 - 127) y1 y1 position (0 - 63) x2 x2 position (0 - 127) y2 y2 position (0 - 63) color mode of the pixel; cleared(0), filled(1), or toggle(2)
Definition at line 87 of file LM6029ACW.cpp.
void DrawPixel | ( | unsigned char | x, |
unsigned char | y, | ||
unsigned char | color | ||
) |
Draws a pixel on the lcd at the specified xy position.
- Parameters:
-
x x position (0 - 127) y y position (0 - 63) color mode of the pixel; cleared(0), filled(1), or toggle(2)
Definition at line 61 of file LM6029ACW.cpp.
void GotoXY | ( | unsigned char | x, |
unsigned char | y | ||
) |
Changes the position on the lcd of the current character cursor from where the next characters will be printed.
- Parameters:
-
x column position (0 - 20) y row position (0 - 7)
Definition at line 188 of file LM6029ACW.cpp.
void PowerActive | ( | void | ) |
Puts the lcd in normal power mode - display on.
Definition at line 52 of file LM6029ACW.cpp.
void PowerSave | ( | void | ) |
Puts the lcd in power save mode - display off.
Definition at line 43 of file LM6029ACW.cpp.
void PutChar | ( | unsigned char | c, |
unsigned char | color | ||
) |
Prints a character on the lcd at the current character cursor position.
- Parameters:
-
c character to print color mode of the character; normal(1), inverted(0)
Definition at line 214 of file LM6029ACW.cpp.
void PutStr | ( | char * | str, |
unsigned char | color | ||
) |
Prints a string on the lcd at the current character cursor position.
- Parameters:
-
str string (char array) to print color mode of the characters in the string; normal(1), inverted(0)
Definition at line 202 of file LM6029ACW.cpp.
Generated on Thu Jul 14 2022 21:57:33 by
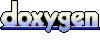