Class library for a Topway LM6029ACW graphics LCD.
Embed:
(wiki syntax)
Show/hide line numbers
LM6029ACW.h
00001 /* LM6029ACW Library v1.0 00002 * Copyright (c) 2016 Grant Phillips 00003 * grant.phillips@nmmu.ac.za 00004 * 00005 * 00006 * Permission is hereby granted, free of charge, to any person obtaining a copy 00007 * of this software and associated documentation files (the "Software"), to deal 00008 * in the Software without restriction, including without limitation the rights 00009 * to use, copy, modify, merge, publish, distribute, sublicense, and/or sell 00010 * copies of the Software, and to permit persons to whom the Software is 00011 * furnished to do so, subject to the following conditions: 00012 * 00013 * The above copyright notice and this permission notice shall be included in 00014 * all copies or substantial portions of the Software. 00015 * 00016 * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR 00017 * IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, 00018 * FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE 00019 * AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER 00020 * LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00021 * OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN 00022 * THE SOFTWARE. 00023 */ 00024 00025 #ifndef LM6029ACW_H 00026 #define LM6029ACW_H 00027 00028 #include "mbed.h" 00029 00030 #define lcd_delayx 0.000001 //delay for writing and reading from lcd - 1us 00031 #define lcd_init_delay 0.001 //delay when initializing the lcd - 1ms 00032 00033 /** Class library for a Topway LM6029ACW graphics LCD. 00034 * 00035 * Example: 00036 * @code 00037 * #include "mbed.h" 00038 * #include "LM6029ACW.h" 00039 * 00040 * LM6029ACW lcd(PD_7, PE_2, PD_6, PD_3, PD_2, PC_15, PC_14, PC_13, PC_11, PC_9, PC_8, PC_6, PC_2); 00041 * // CS1 RES RS WR RD DB7 DB6 DB5 DB4 DB3 DB2 DB1 DB0 00042 * 00043 * DigitalOut backlight(PB_7); 00044 * 00045 * int main() { 00046 * backlight = 0; //turn on the lcd's backlight 00047 * while(1) { 00048 * lcd.GotoXY(4, 3); //move cursor to row, col 00049 * lcd.ClrScr(0); //clear all the pixels on the display 00050 * lcd.PutStr("Hello World!", 1); //print text in black pixels 00051 * wait(2.0); 00052 * 00053 * lcd.GotoXY(4, 3); //move cursor to row, col 00054 * lcd.ClrScr(1); //fill all the pixels on the display 00055 * lcd.PutStr("Hello World!", 0); //print text in clear pixels 00056 * wait(2.0); 00057 * } 00058 * } 00059 * @endcode 00060 */ 00061 00062 class LM6029ACW { 00063 public: 00064 /** Create a LM6029ACW object for a graphics LCD connected to the specified pins. 00065 * @param CS1 Chip Select pin used to connect to LM6029ACW's CS1 pin 00066 * @param RES Reset pin used to connect to LM6029ACW's RES pin 00067 * @param RS Register Select pin used to connect to LM6029ACW's RS pin 00068 * @param WR Write pin used to connect to LM6029ACW's WR pin 00069 * @param RD Read pin used to connect to LM6029ACW's RD pin 00070 * @param DB7-DB0 Data Bus pin useds to connect to LM6029ACW's DB7 - DB0 pins 00071 */ 00072 LM6029ACW(PinName CS1, PinName RES, PinName RS, PinName WR, PinName RD, PinName DB7, PinName DB6, PinName DB5, PinName DB4, PinName DB3, PinName DB2, PinName DB1, PinName DB0); 00073 00074 /** Clears the lcd by clearing or filling all the pixels. 00075 * @param color Bit value; clear pixels(0) or fill pixels(1) 00076 */ 00077 void ClrScr(unsigned char color); 00078 00079 /** Puts the lcd in power save mode - display off. 00080 */ 00081 void PowerSave(void); 00082 00083 /** Puts the lcd in normal power mode - display on. 00084 */ 00085 void PowerActive(void); 00086 00087 /** Draws a pixel on the lcd at the specified xy position. 00088 * @param x x position (0 - 127) 00089 * @param y y position (0 - 63) 00090 * @param color mode of the pixel; cleared(0), filled(1), or toggle(2) 00091 */ 00092 void DrawPixel(unsigned char x, unsigned char y, unsigned char color); 00093 00094 /** Draws a line on the lcd from x1,y1 to x2,y2. 00095 * @param x1 x1 position (0 - 127) 00096 * @param y1 y1 position (0 - 63) 00097 * @param x2 x2 position (0 - 127) 00098 * @param y2 y2 position (0 - 63) 00099 * @param color mode of the pixel; cleared(0), filled(1), or toggle(2) 00100 */ 00101 void DrawLine(int x1, int y1, int x2, int y2, unsigned int color); 00102 00103 /** Draws a circle on the lcd at x,y with the option to fill it. 00104 * @param x x position (0 - 127) 00105 * @param y y position (0 - 63) 00106 * @param radius radius of the circle (0 - 127) 00107 * @param fill circle must be filled (=1) or not filled (=0) 00108 * @param color mode of the pixel; cleared(0), filled(1) 00109 */ 00110 void DrawCircle(unsigned char x, unsigned char y, unsigned char radius, unsigned char fill, unsigned char color); 00111 00112 /** Changes the position on the lcd of the current character cursor from where the next characters will be printed. 00113 * @param x column position (0 - 20) 00114 * @param y row position (0 - 7) 00115 */ 00116 void GotoXY(unsigned char x, unsigned char y); 00117 00118 /** Prints a string on the lcd at the current character cursor position. 00119 * @param str string (char array) to print 00120 * @param color mode of the characters in the string; normal(1), inverted(0) 00121 */ 00122 void PutStr(char *str, unsigned char color); 00123 00124 /** Prints a character on the lcd at the current character cursor position. 00125 * @param c character to print 00126 * @param color mode of the character; normal(1), inverted(0) 00127 */ 00128 void PutChar(unsigned char c, unsigned char color); 00129 00130 00131 private: 00132 DigitalOut CS1pin, RESpin, RSpin, WRpin, RDpin; 00133 BusOut DATApins; 00134 unsigned char DisplayRAM[128][8]; 00135 unsigned char character_x, character_y; 00136 00137 00138 /* Creates a delay which is simply a code loop which is independent from any hardware timers. 00139 * @param del 16-bit value to represent the delay cycles 00140 */ 00141 void Delay(unsigned int del); 00142 00143 /* Writes a COMMAND to the LM6029ACW lcd module. 00144 * @param cmd The byte of command to write to the lcd 00145 */ 00146 void WriteCommand(unsigned char cmd); 00147 00148 /* Writes DATA to the LM6029ACW lcd module. 00149 * @param dat The byte of data to write to the lcd 00150 */ 00151 void WriteData(unsigned char dat); 00152 00153 /* Set the display RAM page address. 00154 * @param Page 4-bit value representing the page address (0 - 7) 00155 */ 00156 void SetPage(unsigned char Page); 00157 00158 /* Set the column address counter. 00159 * @param Page Byte value representing the column (0 - 127) 00160 */ 00161 void SetColumn(unsigned char Col); 00162 00163 /** Initializes the LM6029ACW lcd module. 00164 */ 00165 void Init(void); 00166 00167 /* Used by LM6029ACW_DrawChar only updates the column and page numbers.. 00168 */ 00169 void WriteCharCol(unsigned char v, unsigned char x, unsigned char page, unsigned char color); 00170 00171 /* Used by LM6029ACW_PutChar() only and draws the specified character at byte level using a set font. 00172 */ 00173 void DrawChar(unsigned char c, unsigned char x, unsigned char page, unsigned char color); 00174 }; 00175 00176 #endif
Generated on Thu Jul 14 2022 21:57:33 by
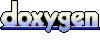