Class library for a Topway LM6029ACW graphics LCD.
Embed:
(wiki syntax)
Show/hide line numbers
LM6029ACW.cpp
00001 #include "LM6029ACW.h" 00002 #include "mbed.h" 00003 00004 00005 /* ***************************************** Public Functions ***************************************** */ 00006 00007 LM6029ACW::LM6029ACW(PinName CS1, PinName RES, PinName RS, PinName WR, PinName RD, PinName DB7, PinName DB6, PinName DB5, PinName DB4, PinName DB3, PinName DB2, PinName DB1, PinName DB0) 00008 : CS1pin(CS1), RESpin(RES), RSpin(RS), WRpin(WR), RDpin(RD), DATApins(DB0, DB1, DB2, DB3, DB4, DB5, DB6, DB7) { 00009 character_x = 0; 00010 character_y = 0; 00011 Init(); 00012 } 00013 00014 00015 void LM6029ACW::ClrScr(unsigned char color) 00016 { 00017 unsigned char i, j, x=0; 00018 00019 CS1pin = 0; 00020 for(i=0; i<8; i++) 00021 { 00022 x=0; 00023 SetColumn(0); 00024 SetPage(i); 00025 for(j=0; j<128; j++) 00026 if(color==0) 00027 { 00028 WriteData(0x00); 00029 DisplayRAM[x][i] = 0x00; 00030 x++; 00031 } 00032 else 00033 { 00034 WriteData(0xff); 00035 DisplayRAM[x][i] = 0xff; 00036 x++; 00037 } 00038 } 00039 CS1pin = 1; 00040 } 00041 00042 00043 void LM6029ACW::PowerSave(void) 00044 { 00045 CS1pin = 0; 00046 WriteCommand(0xae); 00047 WriteCommand(0xa5); 00048 CS1pin = 1; 00049 } 00050 00051 00052 void LM6029ACW::PowerActive(void) 00053 { 00054 CS1pin = 0; 00055 WriteCommand(0xa4); 00056 WriteCommand(0xaf); 00057 CS1pin = 1; 00058 } 00059 00060 00061 void LM6029ACW::DrawPixel(unsigned char x, unsigned char y, unsigned char color) 00062 { 00063 unsigned char page, temp1, temp2; 00064 00065 page = y >> 3; 00066 y = y & 0x07; 00067 temp1 = DisplayRAM[x][page]; 00068 temp2 = 1 << y; 00069 switch(color) 00070 { 00071 case 0: temp1 = temp1 & ~temp2; 00072 break; 00073 case 1: temp1 = temp1 | temp2; 00074 break; 00075 case 2: temp1 = temp1 ^ temp2; 00076 break; 00077 } 00078 DisplayRAM[x][page] = temp1; 00079 CS1pin = 0; 00080 SetColumn(x); 00081 SetPage(page); 00082 WriteData((unsigned char)(temp1)); 00083 CS1pin = 1; 00084 } 00085 00086 00087 void LM6029ACW::DrawLine(int x1, int y1, int x2, int y2, unsigned int color) 00088 { 00089 int dy, dx; 00090 signed char addx=1, addy=1; 00091 signed int P, diff; 00092 00093 int i=0; 00094 dx = abs((signed int)(x2 - x1)); 00095 dy = abs((signed int)(y2 - y1)); 00096 00097 if(x1 > x2) 00098 addx = -1; 00099 if(y1 > y2) 00100 addy = -1; 00101 00102 if(dx >= dy) 00103 { 00104 dy *= 2; 00105 P = dy - dx; 00106 diff = P - dx; 00107 00108 for(; i<=dx; ++i) 00109 { 00110 DrawPixel(x1, y1, color); 00111 00112 if(P < 0) 00113 { 00114 P += dy; 00115 x1 += addx; 00116 } 00117 else 00118 { 00119 P += diff; 00120 x1 += addx; 00121 y1 += addy; 00122 } 00123 } 00124 } 00125 else 00126 { 00127 dx *= 2; 00128 P = dx - dy; 00129 diff = P - dy; 00130 00131 for(; i<=dy; ++i) 00132 { 00133 DrawPixel(x1, y1, color); 00134 00135 if(P < 0) 00136 { 00137 P += dx; 00138 y1 += addy; 00139 } 00140 else 00141 { 00142 P += diff; 00143 x1 += addx; 00144 y1 += addy; 00145 } 00146 } 00147 } 00148 } 00149 00150 00151 void LM6029ACW::DrawCircle(unsigned char x, unsigned char y, unsigned char radius, unsigned char fill, unsigned char color) 00152 { 00153 signed char a, b, P; 00154 00155 a = 0; 00156 b = radius; 00157 P = 1 - radius; 00158 00159 do 00160 { 00161 if(fill) 00162 { 00163 DrawLine(x-a, y+b, x+a, y+b, color); 00164 DrawLine(x-a, y-b, x+a, y-b, color); 00165 DrawLine(x-b, y+a, x+b, y+a, color); 00166 DrawLine(x-b, y-a, x+b, y-a, color); 00167 } 00168 else 00169 { 00170 DrawPixel(a+x, b+y, color); 00171 DrawPixel(b+x, a+y, color); 00172 DrawPixel(x-a, b+y, color); 00173 DrawPixel(x-b, a+y, color); 00174 DrawPixel(b+x, y-a, color); 00175 DrawPixel(a+x, y-b, color); 00176 DrawPixel(x-a, y-b, color); 00177 DrawPixel(x-b, y-a, color); 00178 } 00179 00180 if(P < 0) 00181 P += 3 + 2 * a++; 00182 else 00183 P += 5 + 2 * (a++ - b--); 00184 } while(a <= b); 00185 } 00186 00187 00188 void LM6029ACW::GotoXY(unsigned char x, unsigned char y) 00189 { 00190 if(x<=20) 00191 character_x = x; 00192 else 00193 character_x = 0; 00194 00195 if(y<=7) 00196 character_y = y; 00197 else 00198 character_y = 0; 00199 } 00200 00201 00202 void LM6029ACW::PutStr(char *str, unsigned char color) 00203 { 00204 unsigned int i=0; 00205 00206 do 00207 { 00208 PutChar(str[i], color); 00209 i++; 00210 }while(str[i]!='\0'); 00211 } 00212 00213 00214 void LM6029ACW::PutChar(unsigned char c, unsigned char color) 00215 { 00216 unsigned char x, page; 00217 00218 x = character_x * 6 + 1; 00219 page = character_y; 00220 00221 DrawChar(c, x, page, color); 00222 if(character_x == 20) 00223 { 00224 character_x=0; 00225 if(character_y==7) 00226 character_y=0; 00227 else 00228 character_y++; 00229 } 00230 else 00231 character_x++; 00232 } 00233 00234 00235 00236 00237 00238 00239 00240 00241 00242 00243 00244 00245 /* ***************************************** Private Functions ***************************************** */ 00246 00247 void LM6029ACW::Delay(unsigned int del) 00248 { 00249 unsigned int i=0; 00250 00251 while(i<del) 00252 i++; 00253 } 00254 00255 00256 void LM6029ACW::WriteCommand(unsigned char cmd) 00257 { 00258 RSpin = 0; 00259 00260 DATApins = cmd; 00261 wait(lcd_delayx); 00262 WRpin = 0; 00263 wait(lcd_delayx); 00264 WRpin = 1; 00265 } 00266 00267 00268 void LM6029ACW::WriteData(unsigned char dat) 00269 { 00270 RSpin = 1; 00271 00272 DATApins = dat; 00273 wait(lcd_delayx); 00274 WRpin = 0; 00275 wait(lcd_delayx); 00276 WRpin = 1; 00277 } 00278 00279 00280 void LM6029ACW::SetPage(unsigned char Page) 00281 { 00282 Page = Page & 0x0f; 00283 Page = Page | 0xb0; 00284 WriteCommand(Page); 00285 } 00286 00287 00288 void LM6029ACW::SetColumn(unsigned char Col) 00289 { 00290 unsigned char temp; 00291 00292 temp = Col; 00293 Col = Col & 0x0f; 00294 Col = Col | 0x00; 00295 WriteCommand(Col); 00296 temp = temp >> 4; 00297 Col = temp & 0x0f; 00298 Col = Col | 0x10; 00299 WriteCommand(Col); 00300 } 00301 00302 00303 void LM6029ACW::Init(void) 00304 { 00305 unsigned char i,j; 00306 00307 RDpin = 1; 00308 CS1pin = 0; 00309 RESpin = 0; 00310 wait(lcd_init_delay); 00311 RESpin = 1; 00312 00313 WriteCommand(0xa0); //ADC=0 Normal 00314 WriteCommand(0xc8); //SHL=1 flipped in y-direction 00315 WriteCommand(0xa2); //Bias=0; 1/9 Bias 00316 WriteCommand(0x2f); //Power Control VF, VR, VC 00317 WriteCommand(0xae); //Display OFF 00318 WriteCommand(0x81); //Set voltage reference mode 00319 WriteCommand(0x29); //Power Control VF 00320 WriteCommand(0x40); //Set display start line 00321 WriteCommand(0xaf); //Display ON 00322 00323 for(i=0;i<128;i++) 00324 for(j=0;j<8;j++) 00325 DisplayRAM[i][j]=0x00; 00326 00327 CS1pin = 1; 00328 00329 ClrScr(0); 00330 } 00331 00332 00333 void LM6029ACW::WriteCharCol(unsigned char v, unsigned char x, unsigned char page, unsigned char color) 00334 { 00335 if(color==0) 00336 { 00337 WriteData(~v); 00338 DisplayRAM[x][page] = ~v; 00339 } 00340 else 00341 { 00342 WriteData(v); 00343 DisplayRAM[x][page] = v; 00344 } 00345 } 00346 00347 00348 00349 void LM6029ACW::DrawChar(unsigned char c, unsigned char x, unsigned char page, unsigned char color) 00350 { 00351 CS1pin = 0; 00352 SetColumn(x); 00353 SetPage(page); 00354 00355 switch(c) 00356 { 00357 case 32: // 'SPACE' 00358 { 00359 WriteCharCol(0x00, x, page, color); 00360 WriteCharCol(0x00, x+1, page, color); 00361 WriteCharCol(0x00, x+2, page, color); 00362 WriteCharCol(0x00, x+3, page, color); 00363 WriteCharCol(0x00, x+4, page, color); 00364 }break; 00365 00366 case 33: // '!' 00367 { 00368 WriteCharCol(0x00, x, page, color); 00369 WriteCharCol(0x00, x+1, page, color); 00370 WriteCharCol(0x9e, x+2, page, color); 00371 WriteCharCol(0x00, x+3, page, color); 00372 WriteCharCol(0x00, x+4, page, color); 00373 }break; 00374 00375 case 34: // '"' 00376 { 00377 WriteCharCol(0x00, x, page, color); 00378 WriteCharCol(0x0e, x+1, page, color); 00379 WriteCharCol(0x00, x+2, page, color); 00380 WriteCharCol(0x0e, x+3, page, color); 00381 WriteCharCol(0x00, x+4, page, color); 00382 }break; 00383 00384 case 35: // '#' 00385 { 00386 WriteCharCol(0x28, x, page, color); 00387 WriteCharCol(0xfe, x+1, page, color); 00388 WriteCharCol(0x28, x+2, page, color); 00389 WriteCharCol(0xfe, x+3, page, color); 00390 WriteCharCol(0x28, x+4, page, color); 00391 }break; 00392 00393 case 36: // '$' 00394 { 00395 WriteCharCol(0x48, x, page, color); 00396 WriteCharCol(0x54, x+1, page, color); 00397 WriteCharCol(0xfe, x+2, page, color); 00398 WriteCharCol(0x54, x+3, page, color); 00399 WriteCharCol(0x24, x+4, page, color); 00400 }break; 00401 00402 case 37: // '%' 00403 { 00404 WriteCharCol(0x46, x, page, color); 00405 WriteCharCol(0x26, x+1, page, color); 00406 WriteCharCol(0x10, x+2, page, color); 00407 WriteCharCol(0xc8, x+3, page, color); 00408 WriteCharCol(0xc4, x+4, page, color); 00409 }break; 00410 00411 case 38: // '&' 00412 { 00413 WriteCharCol(0x6c, x, page, color); 00414 WriteCharCol(0x92, x+1, page, color); 00415 WriteCharCol(0xaa, x+2, page, color); 00416 WriteCharCol(0x44, x+3, page, color); 00417 WriteCharCol(0xa0, x+4, page, color); 00418 }break; 00419 00420 case 39: // ''' 00421 { 00422 WriteCharCol(0x00, x, page, color); 00423 WriteCharCol(0x0a, x+1, page, color); 00424 WriteCharCol(0x06, x+2, page, color); 00425 WriteCharCol(0x00, x+3, page, color); 00426 WriteCharCol(0x00, x+4, page, color); 00427 }break; 00428 00429 case 40: // '(' 00430 { 00431 WriteCharCol(0x00, x, page, color); 00432 WriteCharCol(0x38, x+1, page, color); 00433 WriteCharCol(0x44, x+2, page, color); 00434 WriteCharCol(0x82, x+3, page, color); 00435 WriteCharCol(0x00, x+4, page, color); 00436 }break; 00437 00438 case 41: // ')' 00439 { 00440 WriteCharCol(0x00, x, page, color); 00441 WriteCharCol(0x82, x+1, page, color); 00442 WriteCharCol(0x44, x+2, page, color); 00443 WriteCharCol(0x38, x+3, page, color); 00444 WriteCharCol(0x00, x+4, page, color); 00445 }break; 00446 00447 case 42: // '*' 00448 { 00449 WriteCharCol(0x28, x, page, color); 00450 WriteCharCol(0x10, x+1, page, color); 00451 WriteCharCol(0x7c, x+2, page, color); 00452 WriteCharCol(0x10, x+3, page, color); 00453 WriteCharCol(0x28, x+4, page, color); 00454 }break; 00455 00456 case 43: // '+' 00457 { 00458 WriteCharCol(0x10, x, page, color); 00459 WriteCharCol(0x10, x+1, page, color); 00460 WriteCharCol(0x7c, x+2, page, color); 00461 WriteCharCol(0x10, x+3, page, color); 00462 WriteCharCol(0x10, x+4, page, color); 00463 }break; 00464 00465 case 44: // ',' 00466 { 00467 WriteCharCol(0x00, x, page, color); 00468 WriteCharCol(0xa0, x+1, page, color); 00469 WriteCharCol(0x60, x+2, page, color); 00470 WriteCharCol(0x00, x+3, page, color); 00471 WriteCharCol(0x00, x+4, page, color); 00472 }break; 00473 00474 case 45: // '-' 00475 { 00476 WriteCharCol(0x10, x, page, color); 00477 WriteCharCol(0x10, x+1, page, color); 00478 WriteCharCol(0x10, x+2, page, color); 00479 WriteCharCol(0x10, x+3, page, color); 00480 WriteCharCol(0x10, x+4, page, color); 00481 }break; 00482 00483 case 46: // '.' 00484 { 00485 WriteCharCol(0x00, x, page, color); 00486 WriteCharCol(0xc0, x+1, page, color); 00487 WriteCharCol(0xc0, x+2, page, color); 00488 WriteCharCol(0x00, x+3, page, color); 00489 WriteCharCol(0x00, x+4, page, color); 00490 }break; 00491 00492 case 47: // '/' 00493 { 00494 WriteCharCol(0x40, x, page, color); 00495 WriteCharCol(0x20, x+1, page, color); 00496 WriteCharCol(0x10, x+2, page, color); 00497 WriteCharCol(0x08, x+3, page, color); 00498 WriteCharCol(0x04, x+4, page, color); 00499 }break; 00500 00501 case 48: // '0' 00502 { 00503 WriteCharCol(0x7c, x, page, color); 00504 WriteCharCol(0xa2, x+1, page, color); 00505 WriteCharCol(0x92, x+2, page, color); 00506 WriteCharCol(0x8a, x+3, page, color); 00507 WriteCharCol(0x7c, x+4, page, color); 00508 }break; 00509 00510 case 49: // '1' 00511 { 00512 WriteCharCol(0x00, x, page, color); 00513 WriteCharCol(0x84, x+1, page, color); 00514 WriteCharCol(0xfe, x+2, page, color); 00515 WriteCharCol(0x80, x+3, page, color); 00516 WriteCharCol(0x00, x+4, page, color); 00517 }break; 00518 00519 case 50: // '2' 00520 { 00521 WriteCharCol(0x84, x, page, color); 00522 WriteCharCol(0xc2, x+1, page, color); 00523 WriteCharCol(0xa2, x+2, page, color); 00524 WriteCharCol(0x92, x+3, page, color); 00525 WriteCharCol(0x8c, x+4, page, color); 00526 }break; 00527 00528 case 51: // '3' 00529 { 00530 WriteCharCol(0x42, x, page, color); 00531 WriteCharCol(0x82, x+1, page, color); 00532 WriteCharCol(0x8a, x+2, page, color); 00533 WriteCharCol(0x96, x+3, page, color); 00534 WriteCharCol(0x62, x+4, page, color); 00535 }break; 00536 00537 case 52: // '4' 00538 { 00539 WriteCharCol(0x30, x, page, color); 00540 WriteCharCol(0x28, x+1, page, color); 00541 WriteCharCol(0x24, x+2, page, color); 00542 WriteCharCol(0xfe, x+3, page, color); 00543 WriteCharCol(0x20, x+4, page, color); 00544 }break; 00545 00546 case 53: // '5' 00547 { 00548 WriteCharCol(0x4e, x, page, color); 00549 WriteCharCol(0x8a, x+1, page, color); 00550 WriteCharCol(0x8a, x+2, page, color); 00551 WriteCharCol(0x8a, x+3, page, color); 00552 WriteCharCol(0x72, x+4, page, color); 00553 }break; 00554 00555 case 54: // '6' 00556 { 00557 WriteCharCol(0x78, x, page, color); 00558 WriteCharCol(0x94, x+1, page, color); 00559 WriteCharCol(0x92, x+2, page, color); 00560 WriteCharCol(0x92, x+3, page, color); 00561 WriteCharCol(0x60, x+4, page, color); 00562 }break; 00563 00564 case 55: // '7' 00565 { 00566 WriteCharCol(0x02, x, page, color); 00567 WriteCharCol(0xe2, x+1, page, color); 00568 WriteCharCol(0x12, x+2, page, color); 00569 WriteCharCol(0x0a, x+3, page, color); 00570 WriteCharCol(0x06, x+4, page, color); 00571 }break; 00572 00573 case 56: // '8' 00574 { 00575 WriteCharCol(0x6c, x, page, color); 00576 WriteCharCol(0x92, x+1, page, color); 00577 WriteCharCol(0x92, x+2, page, color); 00578 WriteCharCol(0x92, x+3, page, color); 00579 WriteCharCol(0x6c, x+4, page, color); 00580 }break; 00581 00582 case 57: // '9' 00583 { 00584 WriteCharCol(0x0c, x, page, color); 00585 WriteCharCol(0x92, x+1, page, color); 00586 WriteCharCol(0x92, x+2, page, color); 00587 WriteCharCol(0x52, x+3, page, color); 00588 WriteCharCol(0x3c, x+4, page, color); 00589 }break; 00590 00591 case 58: // ':' 00592 { 00593 WriteCharCol(0x00, x, page, color); 00594 WriteCharCol(0x6c, x+1, page, color); 00595 WriteCharCol(0x6c, x+2, page, color); 00596 WriteCharCol(0x00, x+3, page, color); 00597 WriteCharCol(0x00, x+4, page, color); 00598 }break; 00599 00600 case 59: // ';' 00601 { 00602 WriteCharCol(0x00, x, page, color); 00603 WriteCharCol(0xac, x+1, page, color); 00604 WriteCharCol(0x6c, x+2, page, color); 00605 WriteCharCol(0x00, x+3, page, color); 00606 WriteCharCol(0x00, x+4, page, color); 00607 }break; 00608 00609 case 60: // '<' 00610 { 00611 WriteCharCol(0x10, x, page, color); 00612 WriteCharCol(0x28, x+1, page, color); 00613 WriteCharCol(0x44, x+2, page, color); 00614 WriteCharCol(0x82, x+3, page, color); 00615 WriteCharCol(0x00, x+4, page, color); 00616 }break; 00617 00618 case 61: // '=' 00619 { 00620 WriteCharCol(0x28, x, page, color); 00621 WriteCharCol(0x28, x+1, page, color); 00622 WriteCharCol(0x28, x+2, page, color); 00623 WriteCharCol(0x28, x+3, page, color); 00624 WriteCharCol(0x28, x+4, page, color); 00625 }break; 00626 00627 case 62: // '>' 00628 { 00629 WriteCharCol(0x00, x, page, color); 00630 WriteCharCol(0x82, x+1, page, color); 00631 WriteCharCol(0x44, x+2, page, color); 00632 WriteCharCol(0x28, x+3, page, color); 00633 WriteCharCol(0x10, x+4, page, color); 00634 }break; 00635 00636 case 63: // '?' 00637 { 00638 WriteCharCol(0x04, x, page, color); 00639 WriteCharCol(0x02, x+1, page, color); 00640 WriteCharCol(0xa2, x+2, page, color); 00641 WriteCharCol(0x12, x+3, page, color); 00642 WriteCharCol(0x0c, x+4, page, color); 00643 }break; 00644 00645 case 64: // '@' 00646 { 00647 WriteCharCol(0x64, x, page, color); 00648 WriteCharCol(0x92, x+1, page, color); 00649 WriteCharCol(0xf2, x+2, page, color); 00650 WriteCharCol(0x82, x+3, page, color); 00651 WriteCharCol(0x7c, x+4, page, color); 00652 }break; 00653 00654 case 65: // 'A' 00655 { 00656 WriteCharCol(0xfc, x, page, color); 00657 WriteCharCol(0x22, x+1, page, color); 00658 WriteCharCol(0x22, x+2, page, color); 00659 WriteCharCol(0x22, x+3, page, color); 00660 WriteCharCol(0xfc, x+4, page, color); 00661 }break; 00662 00663 case 66: // 'B' 00664 { 00665 WriteCharCol(0xfe, x, page, color); 00666 WriteCharCol(0x92, x+1, page, color); 00667 WriteCharCol(0x92, x+2, page, color); 00668 WriteCharCol(0x92, x+3, page, color); 00669 WriteCharCol(0x6c, x+4, page, color); 00670 }break; 00671 00672 case 67: // 'C' 00673 { 00674 WriteCharCol(0x7c, x, page, color); 00675 WriteCharCol(0x82, x+1, page, color); 00676 WriteCharCol(0x82, x+2, page, color); 00677 WriteCharCol(0x82, x+3, page, color); 00678 WriteCharCol(0x44, x+4, page, color); 00679 }break; 00680 00681 case 68: // 'D' 00682 { 00683 WriteCharCol(0xfe, x, page, color); 00684 WriteCharCol(0x82, x+1, page, color); 00685 WriteCharCol(0x82, x+2, page, color); 00686 WriteCharCol(0x44, x+3, page, color); 00687 WriteCharCol(0x38, x+4, page, color); 00688 }break; 00689 00690 case 69: // 'E' 00691 { 00692 WriteCharCol(0xfe, x, page, color); 00693 WriteCharCol(0x92, x+1, page, color); 00694 WriteCharCol(0x92, x+2, page, color); 00695 WriteCharCol(0x92, x+3, page, color); 00696 WriteCharCol(0x82, x+4, page, color); 00697 }break; 00698 00699 case 70: // 'F' 00700 { 00701 WriteCharCol(0xfe, x, page, color); 00702 WriteCharCol(0x12, x+1, page, color); 00703 WriteCharCol(0x12, x+2, page, color); 00704 WriteCharCol(0x12, x+3, page, color); 00705 WriteCharCol(0x02, x+4, page, color); 00706 }break; 00707 00708 case 71: // 'G' 00709 { 00710 WriteCharCol(0x7c, x, page, color); 00711 WriteCharCol(0x82, x+1, page, color); 00712 WriteCharCol(0x92, x+2, page, color); 00713 WriteCharCol(0x92, x+3, page, color); 00714 WriteCharCol(0xf4, x+4, page, color); 00715 }break; 00716 00717 case 72: // 'H' 00718 { 00719 WriteCharCol(0xfe, x, page, color); 00720 WriteCharCol(0x10, x+1, page, color); 00721 WriteCharCol(0x10, x+2, page, color); 00722 WriteCharCol(0x10, x+3, page, color); 00723 WriteCharCol(0xfe, x+4, page, color); 00724 }break; 00725 00726 case 73: // 'I' 00727 { 00728 WriteCharCol(0x00, x, page, color); 00729 WriteCharCol(0x82, x+1, page, color); 00730 WriteCharCol(0xfe, x+2, page, color); 00731 WriteCharCol(0x82, x+3, page, color); 00732 WriteCharCol(0x00, x+4, page, color); 00733 }break; 00734 00735 case 74: // 'J' 00736 { 00737 WriteCharCol(0x40, x, page, color); 00738 WriteCharCol(0x80, x+1, page, color); 00739 WriteCharCol(0x82, x+2, page, color); 00740 WriteCharCol(0x7e, x+3, page, color); 00741 WriteCharCol(0x02, x+4, page, color); 00742 }break; 00743 00744 case 75: // 'K' 00745 { 00746 WriteCharCol(0xfe, x, page, color); 00747 WriteCharCol(0x10, x+1, page, color); 00748 WriteCharCol(0x28, x+2, page, color); 00749 WriteCharCol(0x44, x+3, page, color); 00750 WriteCharCol(0x82, x+4, page, color); 00751 }break; 00752 00753 case 76: // 'L' 00754 { 00755 WriteCharCol(0xfe, x, page, color); 00756 WriteCharCol(0x80, x+1, page, color); 00757 WriteCharCol(0x80, x+2, page, color); 00758 WriteCharCol(0x80, x+3, page, color); 00759 WriteCharCol(0x80, x+4, page, color); 00760 }break; 00761 00762 case 77: // 'M' 00763 { 00764 WriteCharCol(0xfe, x, page, color); 00765 WriteCharCol(0x04, x+1, page, color); 00766 WriteCharCol(0x18, x+2, page, color); 00767 WriteCharCol(0x04, x+3, page, color); 00768 WriteCharCol(0xfe, x+4, page, color); 00769 }break; 00770 00771 case 78: // 'N' 00772 { 00773 WriteCharCol(0xfe, x, page, color); 00774 WriteCharCol(0x08, x+1, page, color); 00775 WriteCharCol(0x10, x+2, page, color); 00776 WriteCharCol(0x20, x+3, page, color); 00777 WriteCharCol(0xfe, x+4, page, color); 00778 }break; 00779 00780 case 79: // 'O' 00781 { 00782 WriteCharCol(0x7c, x, page, color); 00783 WriteCharCol(0x82, x+1, page, color); 00784 WriteCharCol(0x82, x+2, page, color); 00785 WriteCharCol(0x82, x+3, page, color); 00786 WriteCharCol(0x7c, x+4, page, color); 00787 }break; 00788 00789 case 80: // 'P' 00790 { 00791 WriteCharCol(0xfe, x, page, color); 00792 WriteCharCol(0x12, x+1, page, color); 00793 WriteCharCol(0x12, x+2, page, color); 00794 WriteCharCol(0x12, x+3, page, color); 00795 WriteCharCol(0x0c, x+4, page, color); 00796 }break; 00797 00798 case 81: // 'Q' 00799 { 00800 WriteCharCol(0x7c, x, page, color); 00801 WriteCharCol(0x82, x+1, page, color); 00802 WriteCharCol(0xa2, x+2, page, color); 00803 WriteCharCol(0x42, x+3, page, color); 00804 WriteCharCol(0xbc, x+4, page, color); 00805 }break; 00806 00807 case 82: // 'R' 00808 { 00809 WriteCharCol(0xfe, x, page, color); 00810 WriteCharCol(0x12, x+1, page, color); 00811 WriteCharCol(0x32, x+2, page, color); 00812 WriteCharCol(0x52, x+3, page, color); 00813 WriteCharCol(0x8c, x+4, page, color); 00814 }break; 00815 00816 case 83: // 'S' 00817 { 00818 WriteCharCol(0x8c, x, page, color); 00819 WriteCharCol(0x92, x+1, page, color); 00820 WriteCharCol(0x92, x+2, page, color); 00821 WriteCharCol(0x92, x+3, page, color); 00822 WriteCharCol(0x62, x+4, page, color); 00823 }break; 00824 00825 case 84: // 'T' 00826 { 00827 WriteCharCol(0x02, x, page, color); 00828 WriteCharCol(0x02, x+1, page, color); 00829 WriteCharCol(0xfe, x+2, page, color); 00830 WriteCharCol(0x02, x+3, page, color); 00831 WriteCharCol(0x02, x+4, page, color); 00832 }break; 00833 00834 case 85: // 'U' 00835 { 00836 WriteCharCol(0x7e, x, page, color); 00837 WriteCharCol(0x80, x+1, page, color); 00838 WriteCharCol(0x80, x+2, page, color); 00839 WriteCharCol(0x80, x+3, page, color); 00840 WriteCharCol(0x7e, x+4, page, color); 00841 }break; 00842 00843 case 86: // 'V' 00844 { 00845 WriteCharCol(0x3e, x, page, color); 00846 WriteCharCol(0x40, x+1, page, color); 00847 WriteCharCol(0x80, x+2, page, color); 00848 WriteCharCol(0x40, x+3, page, color); 00849 WriteCharCol(0x3e, x+4, page, color); 00850 }break; 00851 00852 case 87: // 'W' 00853 { 00854 WriteCharCol(0x7e, x, page, color); 00855 WriteCharCol(0x80, x+1, page, color); 00856 WriteCharCol(0x70, x+2, page, color); 00857 WriteCharCol(0x80, x+3, page, color); 00858 WriteCharCol(0x7e, x+4, page, color); 00859 }break; 00860 00861 case 88: // 'X' 00862 { 00863 WriteCharCol(0xc6, x, page, color); 00864 WriteCharCol(0x28, x+1, page, color); 00865 WriteCharCol(0x10, x+2, page, color); 00866 WriteCharCol(0x28, x+3, page, color); 00867 WriteCharCol(0xc6, x+4, page, color); 00868 }break; 00869 00870 case 89: // 'Y' 00871 { 00872 WriteCharCol(0x0e, x, page, color); 00873 WriteCharCol(0x10, x+1, page, color); 00874 WriteCharCol(0xe0, x+2, page, color); 00875 WriteCharCol(0x10, x+3, page, color); 00876 WriteCharCol(0x0e, x+4, page, color); 00877 }break; 00878 00879 case 90: // 'Z' 00880 { 00881 WriteCharCol(0xc2, x, page, color); 00882 WriteCharCol(0xa2, x+1, page, color); 00883 WriteCharCol(0x92, x+2, page, color); 00884 WriteCharCol(0x8a, x+3, page, color); 00885 WriteCharCol(0x86, x+4, page, color); 00886 }break; 00887 00888 case 91: // '[' 00889 { 00890 WriteCharCol(0x00, x, page, color); 00891 WriteCharCol(0xfe, x+1, page, color); 00892 WriteCharCol(0x82, x+2, page, color); 00893 WriteCharCol(0x82, x+3, page, color); 00894 WriteCharCol(0x00, x+4, page, color); 00895 }break; 00896 00897 case 92: // '\' 00898 { 00899 WriteCharCol(0x04, x, page, color); 00900 WriteCharCol(0x08, x+1, page, color); 00901 WriteCharCol(0x10, x+2, page, color); 00902 WriteCharCol(0x20, x+3, page, color); 00903 WriteCharCol(0x40, x+4, page, color); 00904 }break; 00905 00906 case 93: // ']' 00907 { 00908 WriteCharCol(0x00, x, page, color); 00909 WriteCharCol(0x82, x+1, page, color); 00910 WriteCharCol(0x82, x+2, page, color); 00911 WriteCharCol(0xfe, x+3, page, color); 00912 WriteCharCol(0x00, x+4, page, color); 00913 }break; 00914 00915 case 94: // '^' 00916 { 00917 WriteCharCol(0x08, x, page, color); 00918 WriteCharCol(0x04, x+1, page, color); 00919 WriteCharCol(0x02, x+2, page, color); 00920 WriteCharCol(0x04, x+3, page, color); 00921 WriteCharCol(0x08, x+4, page, color); 00922 }break; 00923 00924 case 95: // '_' 00925 { 00926 WriteCharCol(0x80, x, page, color); 00927 WriteCharCol(0x80, x+1, page, color); 00928 WriteCharCol(0x80, x+2, page, color); 00929 WriteCharCol(0x80, x+3, page, color); 00930 WriteCharCol(0x80, x+4, page, color); 00931 }break; 00932 00933 case 96: // '`' 00934 { 00935 WriteCharCol(0x00, x, page, color); 00936 WriteCharCol(0x02, x+1, page, color); 00937 WriteCharCol(0x04, x+2, page, color); 00938 WriteCharCol(0x08, x+3, page, color); 00939 WriteCharCol(0x00, x+4, page, color); 00940 }break; 00941 00942 case 97: // 'a' 00943 { 00944 WriteCharCol(0x40, x, page, color); 00945 WriteCharCol(0xa8, x+1, page, color); 00946 WriteCharCol(0xa8, x+2, page, color); 00947 WriteCharCol(0xa8, x+3, page, color); 00948 WriteCharCol(0xf0, x+4, page, color); 00949 }break; 00950 00951 case 98: // 'b' 00952 { 00953 WriteCharCol(0xfe, x, page, color); 00954 WriteCharCol(0x90, x+1, page, color); 00955 WriteCharCol(0x88, x+2, page, color); 00956 WriteCharCol(0x88, x+3, page, color); 00957 WriteCharCol(0x70, x+4, page, color); 00958 }break; 00959 00960 case 99: // 'c' 00961 { 00962 WriteCharCol(0x70, x, page, color); 00963 WriteCharCol(0x88, x+1, page, color); 00964 WriteCharCol(0x88, x+2, page, color); 00965 WriteCharCol(0x88, x+3, page, color); 00966 WriteCharCol(0x40, x+4, page, color); 00967 }break; 00968 00969 case 100: // 'd' 00970 { 00971 WriteCharCol(0x70, x, page, color); 00972 WriteCharCol(0x88, x+1, page, color); 00973 WriteCharCol(0x88, x+2, page, color); 00974 WriteCharCol(0x90, x+3, page, color); 00975 WriteCharCol(0xfe, x+4, page, color); 00976 }break; 00977 00978 case 101: // 'e' 00979 { 00980 WriteCharCol(0x70, x, page, color); 00981 WriteCharCol(0xa8, x+1, page, color); 00982 WriteCharCol(0xa8, x+2, page, color); 00983 WriteCharCol(0xa8, x+3, page, color); 00984 WriteCharCol(0x30, x+4, page, color); 00985 }break; 00986 00987 case 102: // 'f' 00988 { 00989 WriteCharCol(0x10, x, page, color); 00990 WriteCharCol(0xfc, x+1, page, color); 00991 WriteCharCol(0x12, x+2, page, color); 00992 WriteCharCol(0x02, x+3, page, color); 00993 WriteCharCol(0x04, x+4, page, color); 00994 }break; 00995 00996 case 103: // 'g' 00997 { 00998 WriteCharCol(0x18, x, page, color); 00999 WriteCharCol(0xa4, x+1, page, color); 01000 WriteCharCol(0xa4, x+2, page, color); 01001 WriteCharCol(0xa4, x+3, page, color); 01002 WriteCharCol(0x7c, x+4, page, color); 01003 }break; 01004 01005 case 104: // 'h' 01006 { 01007 WriteCharCol(0xfe, x, page, color); 01008 WriteCharCol(0x10, x+1, page, color); 01009 WriteCharCol(0x08, x+2, page, color); 01010 WriteCharCol(0x08, x+3, page, color); 01011 WriteCharCol(0xf0, x+4, page, color); 01012 }break; 01013 01014 case 105: // 'i' 01015 { 01016 WriteCharCol(0x00, x, page, color); 01017 WriteCharCol(0x88, x+1, page, color); 01018 WriteCharCol(0xfa, x+2, page, color); 01019 WriteCharCol(0x80, x+3, page, color); 01020 WriteCharCol(0x00, x+4, page, color); 01021 }break; 01022 01023 case 106: // 'j' 01024 { 01025 WriteCharCol(0x40, x, page, color); 01026 WriteCharCol(0x80, x+1, page, color); 01027 WriteCharCol(0x88, x+2, page, color); 01028 WriteCharCol(0x7a, x+3, page, color); 01029 WriteCharCol(0x00, x+4, page, color); 01030 }break; 01031 01032 case 107: // 'k' 01033 { 01034 WriteCharCol(0xfe, x, page, color); 01035 WriteCharCol(0x20, x+1, page, color); 01036 WriteCharCol(0x50, x+2, page, color); 01037 WriteCharCol(0x88, x+3, page, color); 01038 WriteCharCol(0x00, x+4, page, color); 01039 }break; 01040 01041 case 108: // 'l' 01042 { 01043 WriteCharCol(0x00, x, page, color); 01044 WriteCharCol(0x82, x+1, page, color); 01045 WriteCharCol(0xfe, x+2, page, color); 01046 WriteCharCol(0x80, x+3, page, color); 01047 WriteCharCol(0x00, x+4, page, color); 01048 }break; 01049 01050 case 109: // 'm' 01051 { 01052 WriteCharCol(0xf8, x, page, color); 01053 WriteCharCol(0x08, x+1, page, color); 01054 WriteCharCol(0x30, x+2, page, color); 01055 WriteCharCol(0x08, x+3, page, color); 01056 WriteCharCol(0xf0, x+4, page, color); 01057 }break; 01058 01059 case 110: // 'n' 01060 { 01061 WriteCharCol(0xf8, x, page, color); 01062 WriteCharCol(0x10, x+1, page, color); 01063 WriteCharCol(0x08, x+2, page, color); 01064 WriteCharCol(0x08, x+3, page, color); 01065 WriteCharCol(0xf0, x+4, page, color); 01066 }break; 01067 01068 case 111: // 'o' 01069 { 01070 WriteCharCol(0x70, x, page, color); 01071 WriteCharCol(0x88, x+1, page, color); 01072 WriteCharCol(0x88, x+2, page, color); 01073 WriteCharCol(0x88, x+3, page, color); 01074 WriteCharCol(0x70, x+4, page, color); 01075 }break; 01076 01077 case 112: // 'p' 01078 { 01079 WriteCharCol(0xf8, x, page, color); 01080 WriteCharCol(0x28, x+1, page, color); 01081 WriteCharCol(0x28, x+2, page, color); 01082 WriteCharCol(0x28, x+3, page, color); 01083 WriteCharCol(0x10, x+4, page, color); 01084 }break; 01085 01086 case 113: // 'q' 01087 { 01088 WriteCharCol(0x10, x, page, color); 01089 WriteCharCol(0x28, x+1, page, color); 01090 WriteCharCol(0x28, x+2, page, color); 01091 WriteCharCol(0x30, x+3, page, color); 01092 WriteCharCol(0xf8, x+4, page, color); 01093 }break; 01094 01095 case 114: // 'r' 01096 { 01097 WriteCharCol(0xf8, x, page, color); 01098 WriteCharCol(0x10, x+1, page, color); 01099 WriteCharCol(0x08, x+2, page, color); 01100 WriteCharCol(0x08, x+3, page, color); 01101 WriteCharCol(0x10, x+4, page, color); 01102 }break; 01103 01104 case 115: // 's' 01105 { 01106 WriteCharCol(0x90, x, page, color); 01107 WriteCharCol(0xa8, x+1, page, color); 01108 WriteCharCol(0xa8, x+2, page, color); 01109 WriteCharCol(0xa8, x+3, page, color); 01110 WriteCharCol(0x40, x+4, page, color); 01111 }break; 01112 01113 case 116: // 't' 01114 { 01115 WriteCharCol(0x08, x, page, color); 01116 WriteCharCol(0x7e, x+1, page, color); 01117 WriteCharCol(0x88, x+2, page, color); 01118 WriteCharCol(0x80, x+3, page, color); 01119 WriteCharCol(0x40, x+4, page, color); 01120 }break; 01121 01122 case 117: // 'u' 01123 { 01124 WriteCharCol(0x78, x, page, color); 01125 WriteCharCol(0x80, x+1, page, color); 01126 WriteCharCol(0x80, x+2, page, color); 01127 WriteCharCol(0x40, x+3, page, color); 01128 WriteCharCol(0xf8, x+4, page, color); 01129 }break; 01130 01131 case 118: // 'v' 01132 { 01133 WriteCharCol(0x38, x, page, color); 01134 WriteCharCol(0x40, x+1, page, color); 01135 WriteCharCol(0x80, x+2, page, color); 01136 WriteCharCol(0x40, x+3, page, color); 01137 WriteCharCol(0x38, x+4, page, color); 01138 }break; 01139 01140 case 119: // 'w' 01141 { 01142 WriteCharCol(0x78, x, page, color); 01143 WriteCharCol(0x80, x+1, page, color); 01144 WriteCharCol(0x60, x+2, page, color); 01145 WriteCharCol(0x80, x+3, page, color); 01146 WriteCharCol(0x78, x+4, page, color); 01147 }break; 01148 01149 case 120: // 'x' 01150 { 01151 WriteCharCol(0x88, x, page, color); 01152 WriteCharCol(0x50, x+1, page, color); 01153 WriteCharCol(0x20, x+2, page, color); 01154 WriteCharCol(0x50, x+3, page, color); 01155 WriteCharCol(0x88, x+4, page, color); 01156 }break; 01157 01158 case 121: // 'y' 01159 { 01160 WriteCharCol(0x18, x, page, color); 01161 WriteCharCol(0xa0, x+1, page, color); 01162 WriteCharCol(0xa0, x+2, page, color); 01163 WriteCharCol(0xa0, x+3, page, color); 01164 WriteCharCol(0x78, x+4, page, color); 01165 }break; 01166 01167 case 122: // 'z' 01168 { 01169 WriteCharCol(0x88, x, page, color); 01170 WriteCharCol(0xc8, x+1, page, color); 01171 WriteCharCol(0xa8, x+2, page, color); 01172 WriteCharCol(0x98, x+3, page, color); 01173 WriteCharCol(0x88, x+4, page, color); 01174 }break; 01175 01176 case 123: // '{' 01177 { 01178 WriteCharCol(0x00, x, page, color); 01179 WriteCharCol(0x10, x+1, page, color); 01180 WriteCharCol(0x6c, x+2, page, color); 01181 WriteCharCol(0x82, x+3, page, color); 01182 WriteCharCol(0x00, x+4, page, color); 01183 }break; 01184 01185 case 124: // '|' 01186 { 01187 WriteCharCol(0x00, x, page, color); 01188 WriteCharCol(0x00, x+1, page, color); 01189 WriteCharCol(0xfe, x+2, page, color); 01190 WriteCharCol(0x00, x+3, page, color); 01191 WriteCharCol(0x00, x+4, page, color); 01192 }break; 01193 01194 case 125: // '}' 01195 { 01196 WriteCharCol(0x00, x, page, color); 01197 WriteCharCol(0x82, x+1, page, color); 01198 WriteCharCol(0x6c, x+2, page, color); 01199 WriteCharCol(0x10, x+3, page, color); 01200 WriteCharCol(0x00, x+4, page, color); 01201 }break; 01202 01203 case 126: // '~' 01204 { 01205 WriteCharCol(0x20, x, page, color); 01206 WriteCharCol(0x10, x+1, page, color); 01207 WriteCharCol(0x10, x+2, page, color); 01208 WriteCharCol(0x20, x+3, page, color); 01209 WriteCharCol(0x10, x+4, page, color); 01210 }break; 01211 /* 01212 01213 case : // '' 01214 { 01215 WriteCharCol(0x, x, page, color); 01216 WriteCharCol(0x, x+1, page, color); 01217 WriteCharCol(0x, x+2, page, color); 01218 WriteCharCol(0x, x+3, page, color); 01219 WriteCharCol(0x, x+4, page, color); 01220 }break; 01221 */ 01222 01223 default: // not supported, print box 01224 { 01225 WriteData(0xfe); 01226 WriteData(0xfe); 01227 WriteData(0xfe); 01228 WriteData(0xfe); 01229 WriteData(0xfe); 01230 } 01231 } 01232 WriteCharCol(0x00, x+5, page, color); //default last blank column of 6 x 8 character 01233 01234 CS1pin = 1; 01235 } 01236
Generated on Thu Jul 14 2022 21:57:33 by
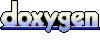