Library for ISL29125 - I2C RGB ambient light sensor
Dependents: ISL29125_demo FinalProject Final_NSR 4180FinalProject_copy ... more
ISL29125 Class Reference
#include <ISL29125.h>
Public Member Functions | |
ISL29125 (PinName sda, PinName scl, PinName irqsync=NC, void(*fptr)(void)=NULL) | |
Create a ISL29125 object connected to I2C bus, irq or sync pin and user-ISR pointer. | |
uint8_t | Status (void) |
Read status register. | |
uint8_t | WhoAmI (void) |
Read the device identifier. | |
bool | Read (uint8_t color, uint16_t *data) |
Read the channel values (12 or 16-bit - depends on resolution). | |
uint16_t | Threshold (uint8_t reg, uint16_t thres=0) |
Read/Write the low/high interrupt threshold value. | |
uint8_t | RGBmode (uint8_t mode=0xff) |
Read/Write the RGB operating mode value (active ADC channels). | |
uint8_t | Range (uint8_t range=0xff) |
Read/Write the sensing range parameter. | |
uint8_t | Resolution (uint8_t resol=0xff) |
Read/Write the ADC resolution parameter. | |
uint8_t | Persist (uint8_t persist=0xff) |
Read/Write the IRQ persistence parameter. | |
uint8_t | IRQonCnvDone (uint8_t irqen=0xff) |
Read/Write the IRQ on conversion done parameter. | |
uint8_t | IRQonColor (uint8_t RGBmode=0xff) |
Read/Write the IRQ threshold to color assignment parameter. | |
uint8_t | IRcomp (uint8_t ircomp=0xff) |
Read/Write the active IR compensation parameter. | |
bool | Run (void) |
Start ADC conversion. |
Detailed Description
ISL29125 class.
Definition at line 46 of file ISL29125.h.
Constructor & Destructor Documentation
ISL29125 | ( | PinName | sda, |
PinName | scl, | ||
PinName | irqsync = NC , |
||
void(*)(void) | fptr = NULL |
||
) |
Create a ISL29125 object connected to I2C bus, irq or sync pin and user-ISR pointer.
- Parameters:
-
sda SDA pin.
scl SCL pin.
irqsync (Optional) Interrupt pin when fptr is also declared OR Sync output when fptr is not declared.
fptr (Optional) Pointer to user-ISR (only used with Interrupt pin).
- Returns:
- none
Definition at line 96 of file ISL29125.cpp.
Member Function Documentation
uint8_t IRcomp | ( | uint8_t | ircomp = 0xff ) |
Read/Write the active IR compensation parameter.
- Parameters:
-
ircomp valid range: between 0..63 or 128..191.
- Returns:
- Written value is returned when called with valid parameter, otherwise 0xff is returned.
Stored value is returned when called without parameter.
Definition at line 295 of file ISL29125.cpp.
uint8_t IRQonCnvDone | ( | uint8_t | irqen = 0xff ) |
Read/Write the IRQ on conversion done parameter.
- Parameters:
-
persist true (enabled).
false (disabled).
- Returns:
- Written value is returned when called with valid parameter, otherwise 0xff is returned.
Stored value is returned when called without parameter.
Definition at line 259 of file ISL29125.cpp.
uint8_t IRQonColor | ( | uint8_t | RGBmode = 0xff ) |
Read/Write the IRQ threshold to color assignment parameter.
- Parameters:
-
RGBmode ISL29125_OFF = No interrupt.
ISL29125_G = Green interrupt.
ISL29125_R = Red interrupt.
ISL29125_B = Blue interrupt.
- Returns:
- Written value is returned when called with valid parameter, otherwise 0xff is returned.
Stored value is returned when called without parameter.
Definition at line 279 of file ISL29125.cpp.
uint8_t Persist | ( | uint8_t | persist = 0xff ) |
Read/Write the IRQ persistence parameter.
- Parameters:
-
persist ISL29125_PERS1 = IRQ occurs when threshold is exceeded once.
ISL29125_PERS2 = IRQ occurs when threshold is exceeded twice.
ISL29125_PERS4 = IRQ occurs when threshold is exceeded 4 times.
ISL29125_PERS8 = IRQ occurs when threshold is exceeded 8 times.
- Returns:
- Written value is returned when called with valid parameter, otherwise 0xff is returned.
Stored value is returned when called without parameter.
Definition at line 243 of file ISL29125.cpp.
uint8_t Range | ( | uint8_t | range = 0xff ) |
Read/Write the sensing range parameter.
- Parameters:
-
range ISL29125_375LX = Max. value corresponds to 375 lux.
ISL29125_10KLX = Max. value corresponds to 10000 lux.
- Returns:
- Written value is returned when called with valid parameter, otherwise 0xff is returned.
Stored value is returned when called without parameter.
Definition at line 211 of file ISL29125.cpp.
bool Read | ( | uint8_t | color, |
uint16_t * | data | ||
) |
Read the channel values (12 or 16-bit - depends on resolution).
- Parameters:
-
color ISL29125_R = Red channel.
ISL29125_G = Green channel.
ISL29125_B = Blue channel.
ISL29125_RGB = Red, Green and Blue channels.
data Pointer to 16-bit array for storing the channel value(s).
Array size: 1 for a single color (Red, Green or Blue) or 3 for all colors.
- Returns:
- bool 1: new data available - 0: no new data available.
Definition at line 145 of file ISL29125.cpp.
uint8_t Resolution | ( | uint8_t | resol = 0xff ) |
Read/Write the ADC resolution parameter.
- Parameters:
-
range ISL29125_16BIT = 16 bit ADC resolution.
ISL29125_12BIT = 12 bit ADC resolution.
- Returns:
- Written value is returned when called with valid parameter, otherwise 0xff is returned.
Stored value is returned when called without parameter.
Definition at line 227 of file ISL29125.cpp.
uint8_t RGBmode | ( | uint8_t | mode = 0xff ) |
Read/Write the RGB operating mode value (active ADC channels).
- Parameters:
-
mode ISL29125_G = G channel only.
ISL29125_R = R channel only.
ISL29125_B = B channel only.
ISL29125_RG = R and G channel.
ISL29125_BG = B and G channel.
ISL29125_RGB = R, G and B channel.
ISL29125_STBY = Standby (No ADC conversion).
ISL29125_OFF = Power down ADC conversion.
- Returns:
- Written value is returned when called with valid parameter, otherwise 0xff is returned.
Stored value is returned when called without parameter.
Definition at line 193 of file ISL29125.cpp.
bool Run | ( | void | ) |
Start ADC conversion.
Only possible when SyncMode is activated.
- Parameters:
-
None.
- Returns:
- bool 1: success - 0: fail.
Definition at line 311 of file ISL29125.cpp.
uint8_t Status | ( | void | ) |
Read status register.
The interrupt status flag is cleared when the status register is read.
- Parameters:
-
NONE
- Returns:
- Content of the entire status register.
bit Description --- --------------------------------------------------------- 5,4 RGB conversion - 00: Inactive 01: Green 10: Red 11: Blue 2 Brownout status - 0: No brownout 1: Power down or brownout occured 1 Conversion status - 0: Conversion is pending or inactive 1: Conversion is completed 0 Interrupt status - 0: no interrupt occured 1: interrupt occured
Definition at line 135 of file ISL29125.cpp.
uint16_t Threshold | ( | uint8_t | reg, |
uint16_t | thres = 0 |
||
) |
Read/Write the low/high interrupt threshold value.
When setIRQonColor is activated, an interrupt will occur when the low or high threshold is exceeded.
- Parameters:
-
reg ISL29125_LTH_W = Write 16-bit low threshold.
ISL29125_HTH_W = Write 16-bit high threshold.
ISL29125_LTH_R = Read 16-bit low threshold.
ISL29125_HTH_R = Read 16-bit high threshold.
thres 16-bit threshold value (only needed when _W parameter is used).
- Returns:
- Written threshold value when called with _W parameter.
Stored threshold value when called with _R parameter only.
Definition at line 175 of file ISL29125.cpp.
uint8_t WhoAmI | ( | void | ) |
Read the device identifier.
- Parameters:
-
none.
- Returns:
- 0x7D on success.
Definition at line 140 of file ISL29125.cpp.
Generated on Sat Jul 16 2022 01:54:41 by
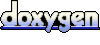