
A rouge-like rpg, heavily inspired on the binding of isaac. Running on a FRDM-K64F Mbed board. C++.
Dependencies: mbed MotionSensor
main.h
00001 #ifndef MAIN_H 00002 #define MAIN_H 00003 00004 // Pre-Processor 00005 #include "mbed.h" 00006 #include "Gamepad.h" 00007 #include "N5110.h" 00008 #include "math.h" 00009 #include "sprites.h" 00010 #include "Entity.h" 00011 #include "Player.h" 00012 #include "Headless.h" 00013 #include "Snake.h" 00014 #include "RoomEngine.h" 00015 #include "Title.h" 00016 00017 #define INSIDE 4 00018 00019 // Variables 00020 float global_contrast = 0.5; 00021 int boss_room_counter = 0; // Counter of how mnay rooms have been explored/spawned 00022 int boss_room_number; // Number of rooms that must be explored for a boss room to spawn 00023 bool boss_room_exist; // Wether or not boss room has been spawned 00024 int number_of_enemies_killed; // For scoring, no of kills 00025 int total_time; // For scoring, time of which the game was played (without pause, without minimap, without room transitions) 00026 int no_of_doorways; // For room generation, number of doorways that room should have 00027 int room_x = MAX_ROOMS_MAP_X; // Room x-coordinate 00028 int room_y = MAX_ROOMS_MAP_Y; // Room y-coordinate 00029 bool have_to[4] = {false, false, false, false}; // Array of which doorways must exist (index order = NESW) 00030 bool cannot[4] = {false, false, false, false}; // Array of which doorways must not exist (index order = NESW) 00031 Player *player; 00032 Room *rooms[MAX_ROOMS_MAP_Y][MAX_ROOMS_MAP_X]; 00033 bool valid_rooms[MAX_ROOMS_MAP_Y][MAX_ROOMS_MAP_X]; 00034 RoomEngine *room_engine; 00035 00036 // Objects 00037 N5110 lcd(PTC9,PTC0,PTC7,PTD2,PTD1,PTC11); 00038 Gamepad gamepad; 00039 Title title; 00040 00041 // Prototypes 00042 void init(); 00043 void game_loop(); 00044 00045 void room_entrance(); 00046 void room_exit(); 00047 void generate_room(); 00048 void update_room_coords(); 00049 void minimap_detection(); 00050 void draw_minimap(int j, int i); 00051 00052 void game_over(); 00053 void win(); 00054 void display_stats(); 00055 void game_unload(); 00056 00057 int opposite(int value); 00058 int count_doorways(); 00059 void update_definite_doorways(); 00060 void update_definite_doorways_up(); 00061 void update_definite_doorways_right(); 00062 void update_definite_doorways_down(); 00063 void update_definite_doorways_left(); 00064 00065 int available_boss_room(); 00066 void set_boss_room(int room_y, int room_x, int side); 00067 00068 #endif
Generated on Tue Jul 19 2022 23:32:07 by
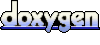