
A rouge-like rpg, heavily inspired on the binding of isaac. Running on a FRDM-K64F Mbed board. C++.
Dependencies: mbed MotionSensor
RoomEngine.h
00001 #ifndef ROOMENGINE_H 00002 #define ROOMENGINE_H 00003 #include "N5110.h" 00004 #include "Gamepad.h" 00005 00006 #include "Player.h" 00007 #include "Health.h" 00008 #include "Room.h" 00009 00010 #include "sprites.h" 00011 #define MAX_ROOMS_MAP_X 11 00012 #define MAX_ROOMS_MAP_Y 11 00013 00014 /**RoomEngine Class 00015 @author Steven Mahasin 00016 @brief Handles all Inter-Class Interactions in the room 00017 @date May 2019 00018 */ 00019 class RoomEngine 00020 { 00021 public: 00022 /** Constructor 00023 * @param global_contrast @details the contrast that the game runs on, set by title screen 00024 */ 00025 RoomEngine(float global_contrast); 00026 /** Destructor */ 00027 ~RoomEngine(); 00028 00029 // Functions 00030 /** 00031 * @brief loads the current player and room into the engine 00032 * @param current_player @details the player we control 00033 * @param current_room @details the room the player is currently in 00034 */ 00035 void load(Player *current_player, Room *current_room); 00036 /** 00037 * @brief plays an entrance_scene of the player into the room 00038 * @param lcd @details the screen that is being drawn on 00039 * @param gamepad @details the gamepad whose controls are being read 00040 */ 00041 void entrance_scene(N5110 &lcd, Gamepad &gamepad); 00042 /** 00043 * @brief increments the room coordinate based on which side the player exits the screen from 00044 */ 00045 void update_current_room(); 00046 00047 /** 00048 * @brief returns 0-4 based on the player's position in the screen 00049 * @return which side of the screen the player is currently at (4 = inside) 00050 */ 00051 int check_player_room_position(); 00052 00053 /** 00054 * @brief reads the input from the gamepad and converts it into member variables 00055 * @param gamepad @details the gamepad whose controls are being read 00056 */ 00057 void read_input(Gamepad &gamepad); 00058 /** 00059 * @brief updates the interaction between entities in room (enemies hp, damage, etc) 00060 * @param number_of_enemies_killed @details for the final score, gets incremented everytime an enemy is killed 00061 */ 00062 void update(int &number_of_enemies_killed); 00063 /** 00064 * @brief to draw all the map, entities, doorway onto the screen 00065 * @param lcd @details the screen that the roomengine draws on 00066 */ 00067 void render(N5110 &lcd, Gamepad &gamepad); 00068 00069 /** 00070 * @brief Plays an exit scene of the player leaving the room 00071 * @param lcd @details the screen that the exit scene is drawn on 00072 * @param gamepad @details the gamepad whose controls are being read 00073 */ 00074 void exit_scene(N5110 &lcd, Gamepad &gamepad); 00075 00076 // Accessor 00077 /** 00078 * @brief reads the member variable _room_x 00079 * @return the current room's x-coordinate 00080 */ 00081 int get_room_x(); 00082 /** 00083 * @brief reads the member variable _room_y 00084 * @return the current room's y-coordinate 00085 */ 00086 int get_room_y(); 00087 00088 private: 00089 // Member variables 00090 /** 00091 * @brief A boolean to indicate if button L is pressed 00092 */ 00093 bool _L; 00094 /** 00095 * @brief A boolean to indicate if button R is pressed 00096 */ 00097 bool _R; 00098 /** 00099 * @brief A boolean to indicate if button A is pressed 00100 */ 00101 bool _A; 00102 /** 00103 * @brief A boolean to indicate if button B is pressed 00104 */ 00105 bool _B; 00106 /** 00107 * @brief A boolean to indicate if button X is pressed 00108 */ 00109 bool _X; 00110 /** 00111 * @brief A boolean to indicate if button Y is pressed 00112 */ 00113 bool _Y; 00114 /** 00115 * @brief A vector2D that stores the x and y of the mapped_coordinates of the joystick 00116 */ 00117 Vector2D mapped_coord; 00118 /** 00119 * @brief The x-coordinate value for the current room's position 00120 */ 00121 int _room_x; 00122 /** 00123 * @brief The y-coordinate value for the current room's position 00124 */ 00125 int _room_y; 00126 /** 00127 * @brief The value of set contrast from title screen, which dictates the contrast of lcd 00128 */ 00129 float _global_contrast; 00130 /** 00131 * @brief A pointer to a Room, used to access the current room class and it's functions 00132 */ 00133 Room *room; 00134 /** 00135 * @brief A pointer to a Player, used to access the current player class and it's functions 00136 */ 00137 Player *player; 00138 00139 // Mutator 00140 /** 00141 * @brief mutates all the user input manually 00142 * @param L @details value to be written onto _L 00143 * @param R @details value to be written onto _R 00144 * @param A @details value to be written onto _A 00145 * @param B @details value to be written onto _B 00146 * @param X @details value to be written onto _X 00147 * @param Y @details value to be written onto _Y 00148 * @param mapped_x @details value to be written onto _mapped_coord.x 00149 * @param mapped_y @details value to be written onto _mapped_coord.y 00150 */ 00151 void set_input(bool L, bool R, bool A, bool B, bool X, bool Y, float mapped_x, float mapped_y); 00152 /** 00153 * @brief mutates _mapped_coord manually 00154 * @param mapped_x @details value to be written onto _mapped_coord.x 00155 * @param mapped_y @details value to be written onto _mapped_coord.y 00156 */ 00157 void set_mapped_coord(float x, float y); 00158 00159 // Methods 00160 /** 00161 * @brief checks if the two entities a and b collide 00162 * @param a @details entity a to be checked if colliding with entity b 00163 * @param b @details entity b to be checked if colliding with entity a 00164 * @return true if the two entities collide 00165 */ 00166 bool entity_collision(Entity &a, Entity &b); 00167 /** 00168 * @brief checks if the entity a collides with any entities in the entity array "array[]" if entity a moves in the x direction 00169 * @param a @details entity a to be checked if colliding with any entity in the array[] 00170 * @param array[] @details an array of entities to be check if colliding with a 00171 * @param no_of_enemies @details constant length of enemies in the array[] 00172 * @param current_entity @details the index of the enemy to be ignored if entity a is within array[] 00173 * @param valid_enemies[] @details the array that defines which entities in array[] exists 00174 * @return -1 or 1 if the entity a collides with any entities in the entity array "array[]" when entity a moves in the x direction depending if a's x pos is bigger than the colliding entity's x pos or not 00175 */ 00176 float entity_move_check_x(Entity *a, Entity *array[], int no_of_enemies, int current_entity, bool valid_enemies[]); 00177 /** 00178 * @brief checks if the entity a collides with any entities in the entity array "array[]" if entity a moves in the y direction 00179 * @param a @details entity a to be checked if colliding with any entity in the array[] 00180 * @param array[] @details an array of entities to be check if colliding with a 00181 * @param no_of_enemies @details constant length of enemies in the array[] 00182 * @param current_entity @details the index of the enemy to be ignored if entity a is within array[] 00183 * @param valid_enemies[] @details the array that defines which entities in array[] exists 00184 * @return -1 or 1 if the entity a collides with any entities in the entity array "array[]" when entity a moves in the y direction depending if a's x pos is bigger than the colliding entity's x pos or not 00185 */ 00186 float entity_move_check_y(Entity *a, Entity *array[], int no_of_enemies, int current_entity, bool valid_enemies[]); 00187 00188 /** 00189 * @brief inflict any damage happening to any entities in the room 00190 */ 00191 void check_damage(); 00192 /** 00193 * @brief inflict any damage happening to the player in the room 00194 */ 00195 void check_damage_player(); 00196 /** 00197 * @brief inflict any damage happening to any enemies in the room 00198 */ 00199 void check_damage_enemies(); 00200 /** 00201 * @brief delete any enemies that has it's health depleted 00202 * @param number_of_enemies_killed @details increments whenever an enemy is successfully deleted 00203 */ 00204 void check_enemies_death(int &number_of_enemies_killed); 00205 /** 00206 * @brief undo movement of entity if the entity collides with the wall (not used in this version) 00207 */ 00208 void check_walls_collision(); 00209 00210 /** 00211 * @brief updates the positions of all entities 00212 */ 00213 void move(); 00214 /** 00215 * @brief updates the positions of player based on the joystick and wall constraint 00216 */ 00217 void move_player(); 00218 /** 00219 * @brief updates the positions of enemies based on their coded movement patterns, repulsion effect and wall constraint 00220 */ 00221 void move_enemies(); 00222 /** 00223 * @brief updates the position of the player whenever the player has just exit the screen 00224 * @param side @details the side the player exits the room from 00225 */ 00226 void update_player_position(int side); 00227 00228 /** 00229 * @brief holds the loop and detection for when a player pauses 00230 * @param lcd @details the screen where the paused screen is going to be displayed on 00231 * @param gamepad @details the gamepad whose controls are being read 00232 */ 00233 void pause_detection(N5110 &lcd, Gamepad &gamepad); 00234 /** 00235 * @brief draws the pause screen onto lcd 00236 * @param lcd @details the screen where the paused screen is going to be displayed on 00237 * @param paused_screen @details the char array of the frozen screen when paused 00238 * @param pause_timer @details the loop count to check if the pause sprite should appear or not for blink effect 00239 */ 00240 void draw_pause_screen(N5110 &lcd, char * paused_screen, int &pause_timer); 00241 00242 /** 00243 * @brief draws the map, the entities and map overlays in the order of descending j 00244 * @param lcd @details the screen where all entities are being drawn on 00245 */ 00246 void draw(N5110 &lcd); 00247 /** 00248 * @brief draws number of hp as hearts on the top left of the screen whenever the user holds button L 00249 * @param lcd @details the screen where the hearts are being drawn on 00250 */ 00251 void draw_health(N5110 &lcd); 00252 00253 }; 00254 #endif
Generated on Tue Jul 19 2022 23:32:07 by
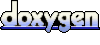