Library for MMA7660FC Accelerometer device
Dependents: TestCode_MMA7660FC 3D_Accelerometer_Tester RTOS-aap-board-modules embed_Grove_3-Axis_Digital_Accelerometer ... more
MMA7660FC Class Reference
Accelerometer MMA7660FC class. More...
#include <MMA7660FC.h>
Public Member Functions | |
MMA7660FC (PinName sda, PinName scl, int addr) | |
Creates an MMA7660FC object connected to the specified I2C object. | |
~MMA7660FC () | |
Destroys an MMA7660FC object. | |
void | init () |
Initialization of device MMA7660FC (required) | |
void | read_Tilt (float *x, float *y, float *z) |
Read the Tilt Angle using Three Axis. | |
int | read_x () |
Reads the x register of the MMA7660FC. | |
int | read_y () |
Reads the y register of the MMA7660FC. | |
int | read_z () |
Reads the z register of the MMA7660FC. | |
char const * | read_Side () |
Reads the Front or Back position of the device. | |
char const * | read_Orientation () |
Reads the Orientation of the device. | |
char | read_reg (char addr) |
Reads from specified MMA7660FC register. | |
void | write_reg (char addr, char data) |
Writes to specified MMA7660FC register. | |
int | check () |
Checks if the address exist on an I2C bus. |
Detailed Description
Accelerometer MMA7660FC class.
Example:
#include "mbed.h" #include "MMA7660FC.h" #define ADDR_MMA7660 0x98 MMA7660FC Acc(p28, p27, ADDR_MMA7660); serial pc(USBTX, USBRX); int main() { Acc.init(); while(1) { int x=0, y=0, z=0; Acc.read_Tilt(&x, &y, &z); pc.printf("Tilt x: %2.2f degree \n", x); // Print the tilt orientation of the X axis pc.printf("Tilt y: %2.2f degree \n", y); // Print the tilt orientation of the Y axis pc.printf("Tilt z: %2.2f degree \n", z); // Print the tilt orientation of the Z axis wait(1); } }
Definition at line 71 of file MMA7660FC.h.
Constructor & Destructor Documentation
MMA7660FC | ( | PinName | sda, |
PinName | scl, | ||
int | addr | ||
) |
Creates an MMA7660FC object connected to the specified I2C object.
- Parameters:
-
sda I2C data port scl I2C clock port addr The address of the MMA7660FC
Definition at line 29 of file MMA7660FC.cpp.
~MMA7660FC | ( | ) |
Destroys an MMA7660FC object.
Definition at line 37 of file MMA7660FC.cpp.
Member Function Documentation
int check | ( | ) |
Checks if the address exist on an I2C bus.
- Returns:
- 0 on success, or non-0 on failure
Definition at line 178 of file MMA7660FC.cpp.
void init | ( | ) |
Initialization of device MMA7660FC (required)
Definition at line 43 of file MMA7660FC.cpp.
char const * read_Orientation | ( | ) |
Reads the Orientation of the device.
- Returns:
- The Left or Right or Down or Up Orientation
Definition at line 125 of file MMA7660FC.cpp.
char read_reg | ( | char | addr ) |
Reads from specified MMA7660FC register.
- Parameters:
-
addr The internal registeraddress of the MMA7660FC
- Returns:
- The value of the register
Definition at line 144 of file MMA7660FC.cpp.
char const * read_Side | ( | ) |
Reads the Front or Back position of the device.
- Returns:
- The Front or Back position
Definition at line 111 of file MMA7660FC.cpp.
void read_Tilt | ( | float * | x, |
float * | y, | ||
float * | z | ||
) |
Read the Tilt Angle using Three Axis.
- Parameters:
-
*x Value of x tilt *y Value of y tilt *z Value of z tilt
Definition at line 51 of file MMA7660FC.cpp.
int read_x | ( | ) |
Reads the x register of the MMA7660FC.
- Returns:
- The value of x acceleration
Definition at line 67 of file MMA7660FC.cpp.
int read_y | ( | ) |
Reads the y register of the MMA7660FC.
- Returns:
- The value of y acceleration
Definition at line 82 of file MMA7660FC.cpp.
int read_z | ( | ) |
Reads the z register of the MMA7660FC.
- Returns:
- The value of z acceleration
Definition at line 97 of file MMA7660FC.cpp.
void write_reg | ( | char | addr, |
char | data | ||
) |
Writes to specified MMA7660FC register.
- Parameters:
-
addr The internal registeraddress of the MMA7660FC data New value of the register
Definition at line 159 of file MMA7660FC.cpp.
Generated on Tue Jul 12 2022 18:21:47 by
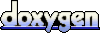