LIB for resistiv touchscreen connected to 4 mbed pins Use SPI_TFT lib
Dependents: touch LCD_Grapher Mandelbrot Tactile ... more
touch_tft Class Reference
touchscreen control class, based on SPI_TFT More...
#include <touch_tft.h>
Public Member Functions | |
touch_tft (PinName xp, PinName xm, PinName yp, PinName ym, PinName mosi, PinName miso, PinName sclk, PinName cs, PinName reset, const char *name="TFT") | |
create a TFT with touch object connected to the pins: | |
void | calibrate (void) |
calibrate the touch display | |
point | get_touch (void) |
read x and y analog samples | |
point | to_pixel (point a_point) |
calculate coord on screen | |
bool | is_touched (point a) |
test if screen is touched |
Detailed Description
touchscreen control class, based on SPI_TFT
Example:
#include "mbed.h" #include "SPI_TFT.h" #include "Arial12x12.h" #include "Arial28x28.h" #include "touch_tft.h" // the TFT is connected to SPI pin 5-7 // the touch is connected to 19,20,16,17 touch_tft tt(p19,p20,p16,p17,p5, p6, p7, p8, p15,"TFT"); // x+,x-,y+,y-,mosi, miso, sclk, cs, reset int main() { point p; tt.claim(stdout); // send stdout to the TFT display tt.background(Black); // set background to black tt.foreground(White); // set chars to white tt.cls(); // clear the screen tt.set_font((unsigned char*) Arial12x12); // select the font tt.set_orientation(1); tt.calibrate(); // calibrate the touch while (1) { p = tt.get_touch(); // read analog pos. if (tt.is_touched(p)) { // test if touched p = tt.to_pixel(p); // convert to pixel pos tt.fillcircle(p.x,p.y,3,Blue); // print a blue dot on the screen } }
Definition at line 61 of file touch_tft.h.
Constructor & Destructor Documentation
touch_tft | ( | PinName | xp, |
PinName | xm, | ||
PinName | yp, | ||
PinName | ym, | ||
PinName | mosi, | ||
PinName | miso, | ||
PinName | sclk, | ||
PinName | cs, | ||
PinName | reset, | ||
const char * | name = "TFT" |
||
) |
create a TFT with touch object connected to the pins:
- Parameters:
-
pin xp resistiv touch x+ pin xm resistiv touch x- pin yp resistiv touch y+ pin ym resistiv touch y- mosi,miso,sclk SPI connection to TFT cs pin connected to CS of display reset pin connected to RESET of display based on my SPI_TFT lib
Definition at line 21 of file touch_tft.cpp.
Member Function Documentation
void calibrate | ( | void | ) |
calibrate the touch display
User is asked to touch on two points on the screen
Definition at line 110 of file touch_tft.cpp.
point get_touch | ( | void | ) |
bool is_touched | ( | point | a ) |
test if screen is touched
- Parameters:
-
point analog x,y
- Returns:
- true is touched
Definition at line 191 of file touch_tft.cpp.
point to_pixel | ( | point | a_point ) |
calculate coord on screen
- Parameters:
-
a_point point(analog x, analog y)
- Returns:
- point(pixel x, pixel y)
Definition at line 181 of file touch_tft.cpp.
Generated on Wed Jul 13 2022 06:29:55 by
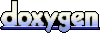