LIB for resistiv touchscreen connected to 4 mbed pins Use SPI_TFT lib
Dependents: touch LCD_Grapher Mandelbrot Tactile ... more
touch_tft.cpp
00001 /* mbed library for resistive touch pads 00002 * uses 4 pins - 2 IO and 2 Analog 00003 00004 * c 2011 Peter Drescher - DC2PD 00005 * 00006 * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR 00007 * IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, 00008 * FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE 00009 * AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER 00010 * LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00011 * OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN 00012 * THE SOFTWARE. 00013 */ 00014 00015 00016 #include "touch_tft.h" 00017 #include "mbed.h" 00018 00019 #define threshold 0x2000 // threshold to detect pressed 00020 00021 touch_tft::touch_tft(PinName xp, PinName xm, PinName yp, PinName ym, 00022 PinName mosi, PinName miso, PinName sclk, PinName cs, PinName reset,const char* name): 00023 _xp(xp),_xm(xm),_yp(yp),_ym(ym),_ax(xp),_ay(yp), 00024 SPI_TFT(mosi,miso,sclk,cs,reset,name) { 00025 xa = xp; 00026 ya = yp; 00027 00028 } 00029 00030 point touch_tft::get_touch() { 00031 unsigned short x1 = 0,x2 = 0, y1 = 0, y2 = 0; 00032 unsigned int s1 = 0,s2 = 0,d1 , d2; 00033 point p; 00034 00035 do { 00036 // read y voltage 00037 _xp.output(); 00038 _xm.output(); 00039 switch (orientation) { 00040 case(0): 00041 case(3): 00042 _xp = 1; 00043 _xm = 0; 00044 break; 00045 case(1): 00046 case(2): 00047 _xp = 0; 00048 _xm = 1; 00049 break; 00050 } 00051 _ym.input(); // y- have to be passive 00052 AnalogIn Ay(ya); // we have to call the constructor to switch to analog mode 00053 wait_us(10); 00054 y1 = Ay.read_u16(); // get y voltage 00055 d1 = (y1 > y2)? (y1-y2) : (y2-y1); 00056 if (((y1 < 8000) && (d1 < 2000)) || ((y1 > 8000) && (d1 < 150))) s1 ++; 00057 else { 00058 if (s1 > 0) s1 --; 00059 } 00060 y2 = y1; 00061 // debug 00062 //locate(1,7); 00063 //printf("d: %4d y: %5d s1: %4d",d1,y1,s1); 00064 00065 // read x voltage 00066 _yp.output(); 00067 _ym.output(); 00068 switch (orientation) { 00069 case(0): 00070 case(1): 00071 _yp = 1; 00072 _ym = 0; 00073 break; 00074 case(2): 00075 case(3): 00076 _yp = 0; 00077 _ym = 1; 00078 break; 00079 } 00080 _xm.input(); // x- have to be passive 00081 AnalogIn Ax(xa); // we have to call the constructor to switch to analog mode 00082 wait_us(10); 00083 x1 = Ax.read_u16(); // get x voltage 00084 d2 = (x1 > x2)? (x1-x2) : (x2-x1); 00085 if (((x1 < 8000) && (d2 < 2000)) || ((x1 > 8000) && (d2 < 150))) s2 ++; 00086 else { 00087 if (s2 > 0) s2 --; 00088 } 00089 x2 = x1; 00090 // debug 00091 //locate(1,8); 00092 //printf("d: %4d x: %5d s2: %4d",d2,x1,s2); 00093 00094 } while (s1 < 3 || s2 < 3); // read until we have three samples close together 00095 switch (orientation) { 00096 case(0): 00097 case(2): 00098 p.y = (x1+x2) / 2; // average of two sample 00099 p.x = (y1+y2) / 2; 00100 break; 00101 case(1): 00102 case(3): 00103 p.x = (x1+x2) / 2; // average of two sample 00104 p.y = (y1+y2) / 2; 00105 break; 00106 } 00107 return(p); 00108 } 00109 00110 void touch_tft::calibrate(void) { 00111 int i; 00112 int a = 0,b = 0,c = 0, d = 0; 00113 int pos_x, pos_y; 00114 point p; 00115 00116 cls(); 00117 line(0,3,6,3,White); 00118 line(3,0,3,6,White); 00119 00120 // get the center of the screen 00121 pos_x = columns() / 2 - 3; 00122 pos_x = pos_x * font[1]; 00123 pos_y = (rows() / 2) - 1; 00124 pos_y = pos_y * font[2]; 00125 00126 locate(pos_x,pos_y); 00127 printf("press cross"); 00128 locate(pos_x,pos_y + font[2]); 00129 printf("to calibrate"); 00130 for (i=0; i<5; i++) { 00131 do { 00132 p = get_touch(); 00133 } while (p.x < 0x2000 | p.y < 0x2000); // wait for touch 00134 a += p.x; 00135 b += p.y; 00136 } 00137 a = a / 5; 00138 b = b / 5; 00139 locate(pos_x,pos_y); 00140 printf("OK "); 00141 do { 00142 p = get_touch(); 00143 } while (p.y > 0x2000 | p.x > 0x2000); // wait for no touch 00144 00145 cls(); 00146 line(width() -5, height() - 8,width() - 5,height() -1,White); // paint cross 00147 line(width() - 8,height() - 5,width() - 1,height() - 5,White); 00148 locate(pos_x,pos_y); 00149 printf("press cross"); 00150 locate(pos_x,pos_y + font[2]); 00151 printf("to calibrate"); 00152 for (i=0; i<5; i++) { 00153 do { 00154 p = get_touch(); 00155 } while (p.y < 0x2000 | p.x < 0x2000); // wait for touch 00156 c+= p.x; 00157 d+= p.y; 00158 } 00159 c = c / 5; 00160 d = d / 5; 00161 00162 locate(pos_x, pos_y); 00163 printf("OK "); 00164 do { 00165 p = get_touch(); 00166 } while (p.y > 0x2000 | p.x > 0x2000); // wait for no touch 00167 00168 cls(); 00169 00170 x_off = a; 00171 y_off = b; 00172 00173 i = c-a; // delta x 00174 pp_tx = i / (width() - 6); 00175 00176 i = d-b; // delta y 00177 pp_ty = i / (height() - 6); 00178 } 00179 00180 00181 point touch_tft::to_pixel(point a_point) { 00182 point p; 00183 00184 p.x = (a_point.x - x_off) / pp_tx; 00185 if (p.x > width()) p.x = width(); 00186 p.y = (a_point.y - y_off) / pp_ty; 00187 if (p.y > height()) p.y = height(); 00188 return (p); 00189 } 00190 00191 bool touch_tft::is_touched(point a) { 00192 if (a.x > threshold & a.y > threshold) return(true); 00193 else return(false); 00194 }
Generated on Wed Jul 13 2022 06:29:54 by
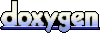