LIB for resistiv touchscreen connected to 4 mbed pins Use SPI_TFT lib
Dependents: touch LCD_Grapher Mandelbrot Tactile ... more
touch_tft.h
00001 /* mbed library for touchscreen connected to 4 mbed pins 00002 * derive from SPI_TFT lib 00003 * Copyright (c) 2011 Peter Drescher - DC2PD 00004 * 00005 * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR 00006 * IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, 00007 * FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE 00008 * AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER 00009 * LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00010 * OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN 00011 * THE SOFTWARE. 00012 */ 00013 00014 #ifndef MBED_TOUCH_H 00015 #define MBED_TOUCH_H 00016 00017 #include "mbed.h" 00018 #include "SPI_TFT.h" 00019 00020 struct point{ 00021 unsigned short x; 00022 unsigned short y; 00023 }; 00024 00025 00026 /** touchscreen control class, based on SPI_TFT 00027 * 00028 * Example: 00029 * @code 00030 * 00031 * #include "mbed.h" 00032 * #include "SPI_TFT.h" 00033 * #include "Arial12x12.h" 00034 * #include "Arial28x28.h" 00035 * #include "touch_tft.h" 00036 * // the TFT is connected to SPI pin 5-7 00037 * // the touch is connected to 19,20,16,17 00038 * 00039 * touch_tft tt(p19,p20,p16,p17,p5, p6, p7, p8, p15,"TFT"); // x+,x-,y+,y-,mosi, miso, sclk, cs, reset 00040 * 00041 * int main() { 00042 * point p; 00043 * 00044 * tt.claim(stdout); // send stdout to the TFT display 00045 * tt.background(Black); // set background to black 00046 * tt.foreground(White); // set chars to white 00047 * tt.cls(); // clear the screen 00048 * tt.set_font((unsigned char*) Arial12x12); // select the font 00049 * tt.set_orientation(1); 00050 * 00051 * tt.calibrate(); // calibrate the touch 00052 * while (1) { 00053 * p = tt.get_touch(); // read analog pos. 00054 * if (tt.is_touched(p)) { // test if touched 00055 * p = tt.to_pixel(p); // convert to pixel pos 00056 * tt.fillcircle(p.x,p.y,3,Blue); // print a blue dot on the screen 00057 * } 00058 * } 00059 * @endcode 00060 */ 00061 class touch_tft : public SPI_TFT{ 00062 public: 00063 /** create a TFT with touch object connected to the pins: 00064 * 00065 * @param pin xp resistiv touch x+ 00066 * @param pin xm resistiv touch x- 00067 * @param pin yp resistiv touch y+ 00068 * @param pin ym resistiv touch y- 00069 * @param mosi,miso,sclk SPI connection to TFT 00070 * @param cs pin connected to CS of display 00071 * @param reset pin connected to RESET of display 00072 * based on my SPI_TFT lib 00073 */ 00074 touch_tft(PinName xp, PinName xm, PinName yp, PinName ym,PinName mosi, PinName miso, PinName sclk, PinName cs, PinName reset,const char* name ="TFT"); 00075 00076 /** calibrate the touch display 00077 * 00078 * User is asked to touch on two points on the screen 00079 */ 00080 void calibrate(void); 00081 00082 /** read x and y analog samples 00083 * 00084 * @returns point(x,y) 00085 */ 00086 point get_touch(void); 00087 00088 /** calculate coord on screen 00089 * 00090 * @param a_point point(analog x, analog y) 00091 * @returns point(pixel x, pixel y) 00092 * 00093 */ 00094 point to_pixel(point a_point); 00095 00096 /** test if screen is touched 00097 * 00098 * @param point analog x,y 00099 * @returns true is touched 00100 * 00101 */ 00102 bool is_touched(point a); 00103 00104 protected: 00105 DigitalInOut _xp; 00106 DigitalInOut _xm; 00107 DigitalInOut _yp; 00108 DigitalInOut _ym; 00109 AnalogIn _ax; 00110 AnalogIn _ay; 00111 PinName xa; 00112 PinName ya; 00113 00114 00115 unsigned short x_a,y_a; 00116 unsigned short x_off,y_off; 00117 unsigned short pp_tx,pp_ty; 00118 00119 00120 00121 }; 00122 00123 #endif
Generated on Wed Jul 13 2022 06:29:54 by
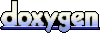