
Runlock POC code
Dependencies: BLE_API mbed nRF51822
Fork of BLE_GATT_Example by
main.cpp
00001 #include "mbed.h" 00002 #include "ble/BLE.h" 00003 00004 DigitalOut led(LED1, 1); 00005 uint16_t customServiceUUID = 0xD00D; 00006 uint16_t readCharUUID = 0x2A7D; 00007 uint16_t writeCharUUID = 0x2A87; 00008 00009 const static uint8_t iBeacon[] = { 00010 0x4C, 0x00, 00011 0x02, 0x15, 00012 0xC0, 0x01, 0xBA, 0xBE, 0xB1, 0x6B, 0x00, 0xB5, 0x5E, 0xC5, 0x2B, 0x7E, 0x15, 0x16, 0x28, 0xAE, 00013 0x00, 0x01, 0x00, 0x01, 0xCE 00014 }; 00015 00016 00017 const static char DEVICE_NAME[] = "00010001"; // change this 00018 static const uint16_t uuid16_list[] = {0xD00D}; //Custom UUID, FFFF is reserved for development 00019 00020 /* Set Up custom Characteristics */ 00021 static uint8_t readValue[20] = {0, }; 00022 ReadOnlyArrayGattCharacteristic<uint8_t, sizeof(readValue)> readChar(readCharUUID, readValue); 00023 00024 static uint8_t writeValue[20] = {0, }; 00025 WriteOnlyArrayGattCharacteristic<uint8_t, sizeof(writeValue)> writeChar(writeCharUUID, writeValue); 00026 00027 /* Set up custom service */ 00028 GattCharacteristic *characteristics[] = {&readChar, &writeChar}; 00029 GattService customService(customServiceUUID, characteristics, sizeof(characteristics) / sizeof(GattCharacteristic *)); 00030 00031 00032 /* 00033 * Restart advertising when phone app disconnects 00034 */ 00035 void disconnectionCallback(const Gap::DisconnectionCallbackParams_t *) 00036 { 00037 BLE::Instance(BLE::DEFAULT_INSTANCE).gap().startAdvertising(); 00038 } 00039 00040 /* 00041 * Handle writes to writeCharacteristic 00042 */ 00043 void writeCharCallback(const GattWriteCallbackParams *params) 00044 { 00045 led=true; 00046 printf("led off\n\r"); 00047 /* Check to see what characteristic was written, by handle */ 00048 if(params->handle == writeChar.getValueHandle()) { 00049 /* toggle LED if only if dragos.stoica@qualitance.com#1234#open or #close */ 00050 if(params->len == 4) { 00051 led=false; 00052 printf("led on\n\r"); // print led toggle 00053 } 00054 00055 printf("Data received: length = %d, data = 0x",params->len); 00056 for(int x=0; x < params->len; x++) { 00057 printf("%x", params->data[x]); 00058 } 00059 printf("\n\r"); 00060 00061 /* Update the readChar with the value of writeChar */ 00062 BLE::Instance(BLE::DEFAULT_INSTANCE).gattServer().write(readChar.getValueHandle(), params->data, params->len); 00063 } 00064 } 00065 /* 00066 * Initialization callback 00067 */ 00068 void bleInitComplete(BLE::InitializationCompleteCallbackContext *params) 00069 { 00070 BLE &ble = params->ble; 00071 ble_error_t error = params->error; 00072 00073 if (error != BLE_ERROR_NONE) { 00074 return; 00075 } 00076 00077 ble.gap().onDisconnection(disconnectionCallback); 00078 ble.gattServer().onDataWritten(writeCharCallback); 00079 00080 /* Setup advertising */ 00081 ble.gap().accumulateAdvertisingPayload(GapAdvertisingData::BREDR_NOT_SUPPORTED | GapAdvertisingData::LE_GENERAL_DISCOVERABLE); // BLE only, no classic BT 00082 ble.gap().setAdvertisingType(GapAdvertisingParams::ADV_CONNECTABLE_UNDIRECTED); // advertising type 00083 ble.gap().accumulateAdvertisingPayload(GapAdvertisingData::MANUFACTURER_SPECIFIC_DATA, iBeacon, sizeof(iBeacon)); 00084 ble.gap().accumulateAdvertisingPayload(GapAdvertisingData::COMPLETE_LOCAL_NAME, (uint8_t *)DEVICE_NAME, sizeof(DEVICE_NAME)); 00085 ble.gap().accumulateScanResponse(GapAdvertisingData::COMPLETE_LOCAL_NAME, (uint8_t *)DEVICE_NAME, sizeof(DEVICE_NAME)); // add name 00086 ble.gap().accumulateScanResponse(GapAdvertisingData::COMPLETE_LIST_16BIT_SERVICE_IDS, (uint8_t *)uuid16_list, sizeof(uuid16_list)); // UUID's broadcast in advertising packet 00087 ble.gap().setAdvertisingInterval(100); // 100ms. 00088 00089 /* Add our custom service */ 00090 ble.addService(customService); 00091 00092 /* Start advertising */ 00093 ble.gap().startAdvertising(); 00094 } 00095 00096 /* 00097 * Main loop 00098 */ 00099 int main(void) 00100 { 00101 /* initialize stuff */ 00102 printf("\n\r********* Starting Main Loop *********\n\r"); 00103 00104 BLE& ble = BLE::Instance(BLE::DEFAULT_INSTANCE); 00105 ble.init(bleInitComplete); 00106 00107 /* SpinWait for initialization to complete. This is necessary because the 00108 * BLE object is used in the main loop below. */ 00109 while (ble.hasInitialized() == false) { /* spin loop */ } 00110 00111 /* Infinite loop waiting for BLE interrupt events */ 00112 while (true) { 00113 ble.waitForEvent(); /* Save power */ 00114 } 00115 }
Generated on Mon Jul 18 2022 15:57:42 by
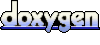