NetServices Stack source
Dependents: HelloWorld ServoInterfaceBoardExample1 4180_Lab4
EthernetNetIf.cpp
00001 00002 /* 00003 Copyright (c) 2010 Donatien Garnier (donatiengar [at] gmail [dot] com) 00004 00005 Permission is hereby granted, free of charge, to any person obtaining a copy 00006 of this software and associated documentation files (the "Software"), to deal 00007 in the Software without restriction, including without limitation the rights 00008 to use, copy, modify, merge, publish, distribute, sublicense, and/or sell 00009 copies of the Software, and to permit persons to whom the Software is 00010 furnished to do so, subject to the following conditions: 00011 00012 The above copyright notice and this permission notice shall be included in 00013 all copies or substantial portions of the Software. 00014 00015 THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR 00016 IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, 00017 FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE 00018 AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER 00019 LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00020 OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN 00021 THE SOFTWARE. 00022 */ 00023 00024 #include "EthernetNetIf.h" 00025 00026 #include "netif/etharp.h" 00027 #include "lwip/dhcp.h " 00028 #include "lwip/dns.h" 00029 #include "lwip/igmp.h" 00030 00031 #include "drv/eth/eth_drv.h" 00032 #include "mbed.h" 00033 00034 #define __DEBUG 00035 #include "dbg/dbg.h" 00036 00037 #include "netCfg.h" 00038 #if NET_ETH 00039 00040 EthernetNetIf::EthernetNetIf() : LwipNetIf(), m_ethArpTimer(), m_dhcpCoarseTimer(), m_dhcpFineTimer(), m_igmpTimer(), m_pNetIf(NULL), 00041 m_netmask(255,255,255,255), m_gateway(), m_hostname(NULL) 00042 { 00043 m_hostname = NULL; 00044 m_pNetIf = new netif; 00045 m_useDhcp = true; 00046 } 00047 00048 EthernetNetIf::EthernetNetIf(IpAddr ip, IpAddr netmask, IpAddr gateway, IpAddr dns) : LwipNetIf(), m_ethArpTimer(), m_dhcpCoarseTimer(), m_dhcpFineTimer(), m_igmpTimer(), m_pNetIf(NULL), m_hostname(NULL) //W/o DHCP 00049 { 00050 m_hostname = NULL; 00051 m_netmask = netmask; 00052 m_gateway = gateway; 00053 m_ip = ip; 00054 m_pNetIf = new netif; 00055 dns_setserver(0, &dns.getStruct()); 00056 m_useDhcp = false; 00057 } 00058 00059 EthernetNetIf::~EthernetNetIf() 00060 { 00061 if(m_pNetIf) 00062 { 00063 igmp_stop(m_pNetIf); //Stop IGMP processing 00064 netif_set_down(m_pNetIf); 00065 netif_remove(m_pNetIf); 00066 delete m_pNetIf; 00067 eth_free(); 00068 } 00069 } 00070 00071 EthernetErr EthernetNetIf::setup(int timeout_ms /*= 15000*/) 00072 { 00073 LwipNetIf::init(); 00074 //m_ethArpTicker.attach_us(ðarp_tmr, ARP_TMR_INTERVAL * 1000); // = 5s in etharp.h 00075 m_ethArpTimer.start(); 00076 if(m_useDhcp) 00077 { 00078 //m_dhcpCoarseTicker.attach(&dhcp_coarse_tmr, DHCP_COARSE_TIMER_SECS); // = 60s in dhcp.h 00079 //m_dhcpFineTicker.attach_us(&dhcp_fine_tmr, DHCP_FINE_TIMER_MSECS * 1000); // = 500ms in dhcp.h 00080 m_dhcpCoarseTimer.start(); 00081 m_dhcpFineTimer.start(); 00082 } 00083 m_pNetIf->hwaddr_len = ETHARP_HWADDR_LEN; //6 00084 eth_address((char *)m_pNetIf->hwaddr); 00085 00086 DBG("HW Addr is : %02x:%02x:%02x:%02x:%02x:%02x.\n", 00087 m_pNetIf->hwaddr[0], m_pNetIf->hwaddr[1], m_pNetIf->hwaddr[2], 00088 m_pNetIf->hwaddr[3], m_pNetIf->hwaddr[4], m_pNetIf->hwaddr[5]); 00089 00090 m_pNetIf = netif_add(m_pNetIf, &(m_ip.getStruct()), &(m_netmask.getStruct()), &(m_gateway.getStruct()), NULL, eth_init, ip_input);//ethernet_input);// ip_input); 00091 //m_pNetIf->hostname = "mbedDG";//(char *)m_hostname; //Not used for now 00092 netif_set_default(m_pNetIf); 00093 00094 //DBG("\r\nStarting DHCP.\r\n"); 00095 00096 if(m_useDhcp) 00097 { 00098 dhcp_start(m_pNetIf); 00099 DBG("DHCP Started, waiting for IP...\n"); 00100 } 00101 else 00102 { 00103 netif_set_up(m_pNetIf); 00104 } 00105 00106 Timer timeout; 00107 timeout.start(); 00108 while( !netif_is_up(m_pNetIf) ) //Wait until device is up 00109 { 00110 if(m_useDhcp) 00111 { 00112 if(m_dhcpFineTimer.read_ms()>=DHCP_FINE_TIMER_MSECS) 00113 { 00114 m_dhcpFineTimer.reset(); 00115 dhcp_fine_tmr(); 00116 } 00117 if(m_dhcpCoarseTimer.read()>=DHCP_COARSE_TIMER_SECS) 00118 { 00119 m_dhcpCoarseTimer.reset(); 00120 dhcp_coarse_tmr(); 00121 } 00122 } 00123 poll(); 00124 if( timeout.read_ms() > timeout_ms ) 00125 { 00126 //Abort 00127 if(m_useDhcp) 00128 dhcp_stop(m_pNetIf); 00129 else 00130 netif_set_down(m_pNetIf); 00131 DBG("\r\nTimeout.\r\n"); 00132 return ETH_TIMEOUT; 00133 } 00134 } 00135 00136 #if LWIP_IGMP 00137 igmp_start(m_pNetIf); //Start IGMP processing 00138 #endif 00139 00140 m_ip = IpAddr(&(m_pNetIf->ip_addr)); 00141 00142 DBG("Connected, IP : %d.%d.%d.%d\n", m_ip[0], m_ip[1], m_ip[2], m_ip[3]); 00143 00144 return ETH_OK; 00145 } 00146 00147 void EthernetNetIf::poll() 00148 { 00149 if(m_ethArpTimer.read_ms()>=ARP_TMR_INTERVAL) 00150 { 00151 m_ethArpTimer.reset(); 00152 etharp_tmr(); 00153 } 00154 #if LWIP_IGMP 00155 if(m_igmpTimer.read_ms()>=IGMP_TMR_INTERVAL) 00156 { 00157 m_igmpTimer.reset(); 00158 igmp_tmr(); 00159 } 00160 #endif 00161 LwipNetIf::poll(); 00162 eth_poll(); 00163 } 00164 00165 #endif
Generated on Tue Jul 12 2022 11:52:57 by
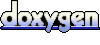