
TI's CC3100 websocket camera demo with Arducam mini ov5642 and freertos. Should work with other M3's. Work in progress test demo.
HTTP and WebSocket Response modules
This module implements routines to allow content handler modules to build and send HTTP responses back to the client. More...
Functions | |
int | HttpResponse_Headers (UINT16 uConnection, UINT16 uHttpStatus, UINT16 uFlags, UINT32 uContentLength, struct HttpBlob contentType, struct HttpBlob location) |
Respond with the specified HTTP status and headers. | |
void | HttpResponse_GetPacketSendBuffer (struct HttpBlob *pPacketSendBuffer) |
Retrieves the pointer and size of the packet-send buffer This function should be called by content handlers that wish to use the already-allocated packet-send buffer in calls to HttpResponse_Content() | |
int | HttpResponse_Content (UINT16 uConnection, struct HttpBlob content) |
Send response content to the client. | |
int | HttpResponse_CannedRedirect (UINT16 uConnection, struct HttpBlob location, UINT16 bPermanent) |
Sends a canned response, with an HTTP redirect This function should be called *instead* of HttpResponse_Status(), HttpResponse_Headers() and HttpResponse_Content() | |
int | HttpResponse_CannedError (UINT16 uConnection, UINT16 uHttpStatus) |
Sends a canned response, with an error message This function should be called *instead* of HttpResponse_Status(), HttpResponse_Headers() and HttpResponse_Content() |
Detailed Description
This module implements routines to allow content handler modules to build and send HTTP responses back to the client.
There are two layers in this module:
- The lower layer consists of HttpResponse_Headers() and HttpResponse_Content(). These routines allow the caller to specify all details of the response.
- The higher layer consists of HttpResponse_Canned*(). These routines emit canned (pre-made) responses, such as redirects and errors, which are useful in many situations.
Function Documentation
int HttpResponse_CannedError | ( | UINT16 | uConnection, |
UINT16 | uHttpStatus | ||
) |
Sends a canned response, with an error message This function should be called *instead* of HttpResponse_Status(), HttpResponse_Headers() and HttpResponse_Content()
- Parameters:
-
uConnection The connection number, as it appears in the HttpRequest structure uHttpStatus The HTTP error status. Must be one of HTTP_STATUS_ERROR_*
Definition at line 1541 of file HttpCore.cpp.
int HttpResponse_CannedRedirect | ( | UINT16 | uConnection, |
struct HttpBlob | location, | ||
UINT16 | bPermanent | ||
) |
Sends a canned response, with an HTTP redirect This function should be called *instead* of HttpResponse_Status(), HttpResponse_Headers() and HttpResponse_Content()
- Parameters:
-
uConnection The connection number, as it appears in the HttpRequest structure pLocation The redirect URL bPermanent zero for temporary redirect, nonzero for permanent redirect
Definition at line 1530 of file HttpCore.cpp.
int HttpResponse_Content | ( | UINT16 | uConnection, |
struct HttpBlob | content | ||
) |
Send response content to the client.
This function may be called more than once, until all the content is sent.
- Parameters:
-
uConnection The connection number, as it appears in the HttpRequest structure content Content blob to send to the client.
Definition at line 1339 of file HttpCore.cpp.
void HttpResponse_GetPacketSendBuffer | ( | struct HttpBlob * | pPacketSendBuffer ) |
Retrieves the pointer and size of the packet-send buffer This function should be called by content handlers that wish to use the already-allocated packet-send buffer in calls to HttpResponse_Content()
- Parameters:
-
[out] pPacketSendBuffer Returns the pointer and size of the packet-send buffer
Definition at line 1333 of file HttpCore.cpp.
int HttpResponse_Headers | ( | UINT16 | uConnection, |
UINT16 | uHttpStatus, | ||
UINT16 | uFlags, | ||
UINT32 | uContentLength, | ||
struct HttpBlob | contentType, | ||
struct HttpBlob | location | ||
) |
Respond with the specified HTTP status and headers.
- Parameters:
-
uConnection The connection number, as it appears in the HttpRequest structure uHttpStatus The HTTP status number to response with. Must be one of HTTP_STATUS_* uFlags Flags which are manifested in the response headers. See HTTP_RESPONSE_FLAG_* uContentLength The total length of content which will be sent via HttpResponse_Content() contentType The content type string, or NULL to omit the content type location A string which will be used for the Location header, or NULL to omit the Location header
Definition at line 1251 of file HttpCore.cpp.
Generated on Wed Jul 13 2022 15:58:46 by
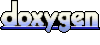