
TI's CC3100 websocket camera demo with Arducam mini ov5642 and freertos. Should work with other M3's. Work in progress test demo.
HttpCore
Typedefs | |
typedef struct HttpConnectionData | HttpConnectionData |
This structure holds all information for an HTTP connection. | |
Enumerations | |
enum | HttpConnectionState { Inactive, RequestMethod, RequestHeaders, DropCurrentHeader, RequestData, Processing, ResponseData, ResponseComplete, WebSocketRequest, WebSocketResponse, WebSocketDataRecv, WebSocketDataSend } |
This enumeration defines all possible states for a connection. More... | |
enum | HttpHandler { None, HttpStatic, HttpDynamic } |
This enumeration defines all possible request-handler modules. More... | |
Functions | |
static void | HttpCore_InitWebServer () |
Initialize the server's global state structure. | |
static int | HttpCore_HandleRequestPacket (UINT16 uConnection, struct HttpBlob packet) |
The main state machine to handle an HTTP transaction Every received data packet for a connection is passed to this function for parsing and handling. | |
static int | HttpCore_HandleMethodLine (UINT16 uConnection, struct HttpBlob line) |
This function handles connection initial state Parse the first header line as a method header. | |
static int | HttpCore_HandleHeaderLine (UINT16 uConnection, struct HttpBlob line) |
Handle The HTTP headers (after method) one by one If an empty header is received then the headers section is complete Searches for the headers tokens. | |
static int | WSCore_HandshakeRequest (UINT16 uConnection, struct HttpBlob line) |
Handle The WebSocket headers (after method) one by one If an empty header is received then the headers section is complete Searches for the headers tokens. | |
static int | HttpCore_HandleRequestData (UINT16 uConnection, struct HttpBlob *pData) |
Handles request data for this transaction Behaves the same for POST and GET methods - If content length header was present then read the content for further processing If the content is too long then ignore it. | |
static void | HttpCore_ProcessNotFound (UINT16 uConnection) |
Returns HTTP 404 not found response. | |
void | HttpServerInitAndRun (void *param) |
Initialize and start the HTTP server. | |
static void | HttpCore_ResetConnection (UINT16 uConnection) |
Reset open connection after finishing HTPP transaction. | |
void | HttpCore_CloseConnection (UINT16 uConnection) |
Close a connection and clean up its state. | |
static int | HttpCore_GetNextLine (UINT16 uConnection, struct HttpBlob *pCurrentLocation, struct HttpBlob *pLine) |
Getting the next line in the HTTP headers section This function is called to get the header lines one by one until an empty line is encountered which means the end of the header section The input is the connection and the remaining blob of the received packet. | |
static int | HttpCore_SendPacket (UINT16 uConnection, struct HttpBlob buffer) |
Sends the input blob over the connection socket. | |
void | HttpResponse_AddCharToResponseHeaders (char ch) |
Add char to the response buffer. | |
static void | HttpResponse_AddNumberToResponseHeaders (UINT32 num) |
Add UINT32 number to the response buffer. | |
void | HttpResponse_AddStringToResponseHeaders (char *str, UINT16 len) |
Add a string to the response buffer. | |
static void | HttpResponse_AddHeaderLine (char *headerName, UINT16 headerNameLen, char *headerValue, UINT16 headerValueLen) |
Add header line to response buffer Adds a line to the header with the provided name value pair Precondition to this function is that g_state.packetSendSize and g_state.packetSend are correct. | |
static void | HttpResponse_AddHeaderLineNumValue (char *headerName, UINT16 uHeaderNameLen, UINT32 headerValue) |
Add Header line to response buffer Adds a line to the header with the provided name value pair when the value is numeric precondition to this function is that g_state.packetSendSize and g_state.packetSend are correct. | |
static void | HttpStatusString (UINT16 uHttpStatus, struct HttpBlob *status) |
Returns status string according to status code. | |
int | HttpResponse_Headers (UINT16 uConnection, UINT16 uHttpStatus, UINT16 uFlags, UINT32 uContentLength, struct HttpBlob contentType, struct HttpBlob location) |
Respond with the specified HTTP status and headers. | |
void | HttpResponse_GetPacketSendBuffer (struct HttpBlob *pPacketSendBuffer) |
Retrieves the pointer and size of the packet-send buffer This function should be called by content handlers that wish to use the already-allocated packet-send buffer in calls to HttpResponse_Content() | |
int | HttpResponse_Content (UINT16 uConnection, struct HttpBlob content) |
Send response content to the client. | |
int | HttpResponse_CannedRedirect (UINT16 uConnection, struct HttpBlob location, UINT16 bPermanent) |
Sends a canned response, with an HTTP redirect This function should be called *instead* of HttpResponse_Status(), HttpResponse_Headers() and HttpResponse_Content() | |
int | HttpResponse_CannedError (UINT16 uConnection, UINT16 uHttpStatus) |
Sends a canned response, with an error message This function should be called *instead* of HttpResponse_Status(), HttpResponse_Headers() and HttpResponse_Content() | |
Variables | |
int | dataSocket |
The data socket for this connection. Should be INVALID_SOCKET_HANDLE when in Inactive state. | |
struct HttpRequest | request |
The current HTTP request on this connection. | |
enum HttpHandler | handler |
Which handler is going to process the current request. | |
UINT8 | headerStart [HTTP_CORE_MAX_HEADER_LINE_LENGTH] |
An un-parsed chunk of header line. | |
UINT32 | uContentLeft |
Amount of content left to be read in the request or sent in the response. | |
UINT32 | timeout |
timeout count | |
UINT16 | uSavedBufferSize |
If the headers will arrive in several packets the content will be buffered in the headers start buffer until a whole line is available. | |
UINT8 | HttpAuth |
Check weather the authentication is done or not. | |
UINT16 | uOpenConnections |
Number of active connections. | |
struct HttpConnectionData | connections [HTTP_CORE_MAX_CONNECTIONS] |
All possible connections. | |
UINT8 | packetRecv [HTTP_CORE_MAX_PACKET_SIZE_RECEIVED] |
The packet-receive buffer. | |
int | packetRecvSize |
Size of data in the packet-receive buffer. | |
UINT8 | packetSend [HTTP_CORE_MAX_PACKET_SIZE_SEND] |
The packet-send buffer. | |
UINT16 | packetSendSize |
Size of data in the packet-send buffer. | |
struct HttpGlobalState | g_state |
The global state of the HTTP server. |
Typedef Documentation
typedef struct HttpConnectionData HttpConnectionData |
This structure holds all information for an HTTP connection.
Enumeration Type Documentation
enum HttpConnectionState |
This enumeration defines all possible states for a connection.
- Enumerator:
Definition at line 69 of file HttpCore.cpp.
enum HttpHandler |
This enumeration defines all possible request-handler modules.
- Enumerator:
Definition at line 100 of file HttpCore.cpp.
Function Documentation
void HttpCore_CloseConnection | ( | UINT16 | uConnection ) |
Close a connection and clean up its state.
Definition at line 375 of file HttpCore.cpp.
static int HttpCore_GetNextLine | ( | UINT16 | uConnection, |
struct HttpBlob * | pCurrentLocation, | ||
struct HttpBlob * | pLine | ||
) | [static] |
Getting the next line in the HTTP headers section This function is called to get the header lines one by one until an empty line is encountered which means the end of the header section The input is the connection and the remaining blob of the received packet.
- Returns:
- zero if the whole packet was handled, and need to wait for more data (pLine is not set to anything yet) negative if some error occurred, and the connection should be closed. positive if successful. In this case pCurrentLocation is advanced to skip the line and pLine returns the next line, or NULL and 0 if it should be discarded
Definition at line 404 of file HttpCore.cpp.
static int HttpCore_HandleHeaderLine | ( | UINT16 | uConnection, |
struct HttpBlob | line | ||
) | [static] |
Handle The HTTP headers (after method) one by one If an empty header is received then the headers section is complete Searches for the headers tokens.
If important data is found then it is saved in the connection object
returns nonzero if sucessful
Definition at line 798 of file HttpCore.cpp.
static int HttpCore_HandleMethodLine | ( | UINT16 | uConnection, |
struct HttpBlob | line | ||
) | [static] |
This function handles connection initial state Parse the first header line as a method header.
Method line should be in the form: GET /resource.html HTTP/1.1
- Returns:
- nonzero if success
Definition at line 705 of file HttpCore.cpp.
static int HttpCore_HandleRequestData | ( | UINT16 | uConnection, |
struct HttpBlob * | pData | ||
) | [static] |
Handles request data for this transaction Behaves the same for POST and GET methods - If content length header was present then read the content for further processing If the content is too long then ignore it.
- Returns:
- 1 if successful, pData is updated to skip the handled data 0 if all data is consumed and need to read more data negative if an error occurs and the connection should be closed.
Definition at line 1057 of file HttpCore.cpp.
static int HttpCore_HandleRequestPacket | ( | UINT16 | uConnection, |
struct HttpBlob | packet | ||
) | [static] |
The main state machine to handle an HTTP transaction Every received data packet for a connection is passed to this function for parsing and handling.
If there is an error the connection will be closed in the end of the transaction It will also be closed if "connection: close" header is received or HTTP version is 1.0
- Returns:
- zero to close the connection nonzero if packet was consumed successfully, and the connection can handle more data
Definition at line 570 of file HttpCore.cpp.
static void HttpCore_InitWebServer | ( | ) | [static] |
Initialize the server's global state structure.
Definition at line 352 of file HttpCore.cpp.
static void HttpCore_ProcessNotFound | ( | UINT16 | uConnection ) | [static] |
Returns HTTP 404 not found response.
Definition at line 1100 of file HttpCore.cpp.
static void HttpCore_ResetConnection | ( | UINT16 | uConnection ) | [static] |
Reset open connection after finishing HTPP transaction.
Definition at line 339 of file HttpCore.cpp.
static int HttpCore_SendPacket | ( | UINT16 | uConnection, |
struct HttpBlob | buffer | ||
) | [static] |
Sends the input blob over the connection socket.
Definition at line 1109 of file HttpCore.cpp.
void HttpResponse_AddCharToResponseHeaders | ( | char | ch ) |
Add char to the response buffer.
Definition at line 1160 of file HttpCore.cpp.
static void HttpResponse_AddHeaderLine | ( | char * | headerName, |
UINT16 | headerNameLen, | ||
char * | headerValue, | ||
UINT16 | headerValueLen | ||
) | [static] |
Add header line to response buffer Adds a line to the header with the provided name value pair Precondition to this function is that g_state.packetSendSize and g_state.packetSend are correct.
Definition at line 1193 of file HttpCore.cpp.
static void HttpResponse_AddHeaderLineNumValue | ( | char * | headerName, |
UINT16 | uHeaderNameLen, | ||
UINT32 | headerValue | ||
) | [static] |
Add Header line to response buffer Adds a line to the header with the provided name value pair when the value is numeric precondition to this function is that g_state.packetSendSize and g_state.packetSend are correct.
Definition at line 1207 of file HttpCore.cpp.
static void HttpResponse_AddNumberToResponseHeaders | ( | UINT32 | num ) | [static] |
Add UINT32 number to the response buffer.
Definition at line 1170 of file HttpCore.cpp.
void HttpResponse_AddStringToResponseHeaders | ( | char * | str, |
UINT16 | len | ||
) |
Add a string to the response buffer.
Definition at line 1182 of file HttpCore.cpp.
int HttpResponse_CannedError | ( | UINT16 | uConnection, |
UINT16 | uHttpStatus | ||
) |
Sends a canned response, with an error message This function should be called *instead* of HttpResponse_Status(), HttpResponse_Headers() and HttpResponse_Content()
- Parameters:
-
uConnection The connection number, as it appears in the HttpRequest structure uHttpStatus The HTTP error status. Must be one of HTTP_STATUS_ERROR_*
Definition at line 1541 of file HttpCore.cpp.
int HttpResponse_CannedRedirect | ( | UINT16 | uConnection, |
struct HttpBlob | location, | ||
UINT16 | bPermanent | ||
) |
Sends a canned response, with an HTTP redirect This function should be called *instead* of HttpResponse_Status(), HttpResponse_Headers() and HttpResponse_Content()
- Parameters:
-
uConnection The connection number, as it appears in the HttpRequest structure pLocation The redirect URL bPermanent zero for temporary redirect, nonzero for permanent redirect
Definition at line 1530 of file HttpCore.cpp.
int HttpResponse_Content | ( | UINT16 | uConnection, |
struct HttpBlob | content | ||
) |
Send response content to the client.
This function may be called more than once, until all the content is sent.
- Parameters:
-
uConnection The connection number, as it appears in the HttpRequest structure content Content blob to send to the client.
Definition at line 1339 of file HttpCore.cpp.
void HttpResponse_GetPacketSendBuffer | ( | struct HttpBlob * | pPacketSendBuffer ) |
Retrieves the pointer and size of the packet-send buffer This function should be called by content handlers that wish to use the already-allocated packet-send buffer in calls to HttpResponse_Content()
- Parameters:
-
[out] pPacketSendBuffer Returns the pointer and size of the packet-send buffer
Definition at line 1333 of file HttpCore.cpp.
int HttpResponse_Headers | ( | UINT16 | uConnection, |
UINT16 | uHttpStatus, | ||
UINT16 | uFlags, | ||
UINT32 | uContentLength, | ||
struct HttpBlob | contentType, | ||
struct HttpBlob | location | ||
) |
Respond with the specified HTTP status and headers.
- Parameters:
-
uConnection The connection number, as it appears in the HttpRequest structure uHttpStatus The HTTP status number to response with. Must be one of HTTP_STATUS_* uFlags Flags which are manifested in the response headers. See HTTP_RESPONSE_FLAG_* uContentLength The total length of content which will be sent via HttpResponse_Content() contentType The content type string, or NULL to omit the content type location A string which will be used for the Location header, or NULL to omit the Location header
Definition at line 1251 of file HttpCore.cpp.
void HttpServerInitAndRun | ( | void * | param ) |
Initialize and start the HTTP server.
The Wifi interface of the CC3200 chip should be initialized by now, and connected to the network
Definition at line 201 of file HttpCore.cpp.
static void HttpStatusString | ( | UINT16 | uHttpStatus, |
struct HttpBlob * | status | ||
) | [static] |
Returns status string according to status code.
Definition at line 1219 of file HttpCore.cpp.
static int WSCore_HandshakeRequest | ( | UINT16 | uConnection, |
struct HttpBlob | line | ||
) | [static] |
Handle The WebSocket headers (after method) one by one If an empty header is received then the headers section is complete Searches for the headers tokens.
If important data is found then it is saved in the connection object
returns nonzero if sucessful
Definition at line 904 of file HttpCore.cpp.
Variable Documentation
struct HttpConnectionData connections[HTTP_CORE_MAX_CONNECTIONS] [inherited] |
All possible connections.
Definition at line 157 of file HttpCore.cpp.
int dataSocket [inherited] |
The data socket for this connection. Should be INVALID_SOCKET_HANDLE when in Inactive state.
Definition at line 125 of file HttpCore.cpp.
struct HttpGlobalState g_state |
The global state of the HTTP server.
Definition at line 169 of file HttpCore.cpp.
enum HttpHandler handler [inherited] |
Which handler is going to process the current request.
Definition at line 129 of file HttpCore.cpp.
UINT8 headerStart[HTTP_CORE_MAX_HEADER_LINE_LENGTH] [inherited] |
An un-parsed chunk of header line.
Definition at line 131 of file HttpCore.cpp.
UINT8 HttpAuth [inherited] |
Check weather the authentication is done or not.
Definition at line 139 of file HttpCore.cpp.
UINT8 packetRecv[HTTP_CORE_MAX_PACKET_SIZE_RECEIVED] [inherited] |
The packet-receive buffer.
Definition at line 159 of file HttpCore.cpp.
int packetRecvSize [inherited] |
Size of data in the packet-receive buffer.
Definition at line 161 of file HttpCore.cpp.
UINT8 packetSend[HTTP_CORE_MAX_PACKET_SIZE_SEND] [inherited] |
The packet-send buffer.
Definition at line 163 of file HttpCore.cpp.
UINT16 packetSendSize [inherited] |
Size of data in the packet-send buffer.
Definition at line 165 of file HttpCore.cpp.
struct HttpRequest request [inherited] |
The current HTTP request on this connection.
Definition at line 127 of file HttpCore.cpp.
UINT32 timeout [inherited] |
timeout count
Definition at line 135 of file HttpCore.cpp.
UINT32 uContentLeft [inherited] |
Amount of content left to be read in the request or sent in the response.
Definition at line 133 of file HttpCore.cpp.
UINT16 uOpenConnections [inherited] |
Number of active connections.
Definition at line 155 of file HttpCore.cpp.
UINT16 uSavedBufferSize [inherited] |
If the headers will arrive in several packets the content will be buffered in the headers start buffer until a whole line is available.
Definition at line 137 of file HttpCore.cpp.
Generated on Wed Jul 13 2022 15:58:46 by
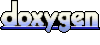