A very simple software SPI library for the MAX32630FTHR board. It was designed to support the max31865 and RadioHead libraries I ported for my Rocket mbed project.
swspi Class Reference
bit-banged SPI More...
#include <swspi.h>
Public Member Functions | |
swspi (PinName mosiPin, PinName misoPin, PinName sckPin, PinName ss1Pin, PinName ss2Pin) | |
Constructor. | |
void | init () |
Initialise the Driver transport hardware and software. | |
uint8_t | spiRead (uint8_t reg, int cpha, int ss) |
Reads a single register from the SPI device. | |
uint8_t | spiWrite (uint8_t reg, uint8_t val, int cpha, int ss) |
Writes a single byte to the SPI device. | |
uint8_t | spiBurstRead (uint8_t reg, uint8_t *dest, uint8_t len, int cpha, int ss) |
Reads a number of consecutive registers from the SPI device using burst read mode. | |
uint8_t | spiBurstWrite (uint8_t reg, const uint8_t *src, uint8_t len, int cpha, int ss) |
Write a number of consecutive registers using burst write mode. | |
Protected Attributes | |
DigitalOut * | mosiP |
Pins. | |
int | _cpha |
The current transfer data phase. | |
uint8_t | _ss |
The current transfer slave select. |
Detailed Description
bit-banged SPI
Definition at line 19 of file swspi.h.
Constructor & Destructor Documentation
swspi | ( | PinName | mosiPin, |
PinName | misoPin, | ||
PinName | sckPin, | ||
PinName | ss1Pin, | ||
PinName | ss2Pin | ||
) |
Member Function Documentation
void init | ( | ) |
uint8_t spiBurstRead | ( | uint8_t | reg, |
uint8_t * | dest, | ||
uint8_t | len, | ||
int | cpha, | ||
int | ss | ||
) |
Reads a number of consecutive registers from the SPI device using burst read mode.
- Parameters:
-
[in] reg Register number of the first register [in] dest Array to write the register values to. Must be at least len bytes [in] len Number of bytes to read
- Returns:
- Some devices return a status byte during the first data transfer. This byte is returned. it may or may not be meaningfule depending on the the type of device being accessed.
uint8_t spiBurstWrite | ( | uint8_t | reg, |
const uint8_t * | src, | ||
uint8_t | len, | ||
int | cpha, | ||
int | ss | ||
) |
Write a number of consecutive registers using burst write mode.
- Parameters:
-
[in] reg Register number of the first register [in] src Array of new register values to write. Must be at least len bytes [in] len Number of bytes to write
- Returns:
- Some devices return a status byte during the first data transfer. This byte is returned. it may or may not be meaningfule depending on the the type of device being accessed.
uint8_t spiRead | ( | uint8_t | reg, |
int | cpha, | ||
int | ss | ||
) |
uint8_t spiWrite | ( | uint8_t | reg, |
uint8_t | val, | ||
int | cpha, | ||
int | ss | ||
) |
Writes a single byte to the SPI device.
- Parameters:
-
[in] reg Register number [in] val The value to write
- Returns:
- Some devices return a status byte during the first data transfer. This byte is returned. it may or may not be meaningfule depending on the the type of device being accessed.
Field Documentation
Generated on Tue Jul 12 2022 13:56:05 by
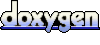