A very simple software SPI library for the MAX32630FTHR board. It was designed to support the max31865 and RadioHead libraries I ported for my Rocket mbed project.
swspi.h
00001 // Software (bit-banged) SPI Module supporting two CPHA modes and two 00002 // chip select signals. Yields to allow use by different threads. 00003 // 00004 00005 #ifndef sw_spi_h 00006 #define sw_spi_h 00007 00008 #include "mbed.h" 00009 #include <stdint.h> 00010 00011 // This is the bit in the SPI address that marks it as a write 00012 #define SPI_WRITE_MASK 0x80 00013 00014 00015 ///////////////////////////////////////////////////////////////////// 00016 /// \class swspi swspi.h <swspi.h> 00017 /// \brief bit-banged SPI 00018 00019 class swspi 00020 { 00021 public: 00022 /// Constructor 00023 /// \param[in] mosiPin pin number for MOSI 00024 /// \param[in] misoPin pin number for MISO 00025 /// \param[in] sckPin pin number for SCK 00026 /// \param[in] ss1Pin pin number for Slave Select 1 00027 /// \param[in] ss2Pin pin number for Slave Select 2 00028 swspi(PinName mosiPin, PinName misoPin, PinName sckPin, PinName ss1Pin, PinName ss2Pin); 00029 00030 /// Initialise the Driver transport hardware and software. 00031 /// Make sure the Driver is properly configured before calling init(). 00032 void init(); 00033 00034 /// Reads a single register from the SPI device 00035 /// \param[in] reg Register number 00036 /// \return The value of the register 00037 uint8_t spiRead(uint8_t reg, int cpha, int ss); 00038 00039 /// Writes a single byte to the SPI device 00040 /// \param[in] reg Register number 00041 /// \param[in] val The value to write 00042 /// \return Some devices return a status byte during the first data transfer. This byte is returned. 00043 /// it may or may not be meaningfule depending on the the type of device being accessed. 00044 uint8_t spiWrite(uint8_t reg, uint8_t val, int cpha, int ss); 00045 00046 /// Reads a number of consecutive registers from the SPI device using burst read mode 00047 /// \param[in] reg Register number of the first register 00048 /// \param[in] dest Array to write the register values to. Must be at least len bytes 00049 /// \param[in] len Number of bytes to read 00050 /// \return Some devices return a status byte during the first data transfer. This byte is returned. 00051 /// it may or may not be meaningfule depending on the the type of device being accessed. 00052 uint8_t spiBurstRead(uint8_t reg, uint8_t* dest, uint8_t len, int cpha, int ss); 00053 00054 /// Write a number of consecutive registers using burst write mode 00055 /// \param[in] reg Register number of the first register 00056 /// \param[in] src Array of new register values to write. Must be at least len bytes 00057 /// \param[in] len Number of bytes to write 00058 /// \return Some devices return a status byte during the first data transfer. This byte is returned. 00059 /// it may or may not be meaningfule depending on the the type of device being accessed. 00060 uint8_t spiBurstWrite(uint8_t reg, const uint8_t* src, uint8_t len, int cpha, int ss); 00061 00062 protected: 00063 00064 void _setSlaveSelect(int v); 00065 uint8_t _transfer(uint8_t v); 00066 00067 /// Pins 00068 DigitalOut* mosiP; 00069 DigitalIn* misoP; 00070 DigitalOut* sckP; 00071 DigitalOut* ss1P; 00072 DigitalOut* ss2P; 00073 00074 /// The current transfer data phase 00075 int _cpha; 00076 00077 /// The current transfer slave select 00078 uint8_t _ss; 00079 00080 // Access control 00081 Mutex _spi_mutex; 00082 }; 00083 00084 #endif
Generated on Tue Jul 12 2022 13:56:05 by
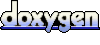