mbed TLS library
Dependents: HTTPClient-SSL WS_SERVER
hmac_drbg.h File Reference
HMAC_DRBG (NIST SP 800-90A) More...
Go to the source code of this file.
Data Structures | |
struct | hmac_drbg_context |
HMAC_DRBG context. More... | |
Functions | |
int | hmac_drbg_init (hmac_drbg_context *ctx, const md_info_t *md_info, int(*f_entropy)(void *, unsigned char *, size_t), void *p_entropy, const unsigned char *custom, size_t len) |
HMAC_DRBG initialisation. | |
int | hmac_drbg_init_buf (hmac_drbg_context *ctx, const md_info_t *md_info, const unsigned char *data, size_t data_len) |
Initilisation of simpified HMAC_DRBG (never reseeds). | |
void | hmac_drbg_set_prediction_resistance (hmac_drbg_context *ctx, int resistance) |
Enable / disable prediction resistance (Default: Off) | |
void | hmac_drbg_set_entropy_len (hmac_drbg_context *ctx, size_t len) |
Set the amount of entropy grabbed on each reseed (Default: given by the security strength, which depends on the hash used, see hmac_drbg_init() ) | |
void | hmac_drbg_set_reseed_interval (hmac_drbg_context *ctx, int interval) |
Set the reseed interval (Default: POLARSSL_HMAC_DRBG_RESEED_INTERVAL) | |
void | hmac_drbg_update (hmac_drbg_context *ctx, const unsigned char *additional, size_t add_len) |
HMAC_DRBG update state. | |
int | hmac_drbg_reseed (hmac_drbg_context *ctx, const unsigned char *additional, size_t len) |
HMAC_DRBG reseeding (extracts data from entropy source) | |
int | hmac_drbg_random_with_add (void *p_rng, unsigned char *output, size_t output_len, const unsigned char *additional, size_t add_len) |
HMAC_DRBG generate random with additional update input. | |
int | hmac_drbg_random (void *p_rng, unsigned char *output, size_t out_len) |
HMAC_DRBG generate random. | |
void | hmac_drbg_free (hmac_drbg_context *ctx) |
Free an HMAC_DRBG context. | |
int | hmac_drbg_write_seed_file (hmac_drbg_context *ctx, const char *path) |
Write a seed file. | |
int | hmac_drbg_update_seed_file (hmac_drbg_context *ctx, const char *path) |
Read and update a seed file. | |
int | hmac_drbg_self_test (int verbose) |
Checkup routine. |
Detailed Description
HMAC_DRBG (NIST SP 800-90A)
Copyright (C) 2014, ARM Limited, All Rights Reserved
This file is part of mbed TLS (https://tls.mbed.org)
This program is free software; you can redistribute it and/or modify it under the terms of the GNU General Public License as published by the Free Software Foundation; either version 2 of the License, or (at your option) any later version.
This program is distributed in the hope that it will be useful, but WITHOUT ANY WARRANTY; without even the implied warranty of MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU General Public License for more details.
You should have received a copy of the GNU General Public License along with this program; if not, write to the Free Software Foundation, Inc., 51 Franklin Street, Fifth Floor, Boston, MA 02110-1301 USA.
Definition in file hmac_drbg.h.
Function Documentation
void hmac_drbg_free | ( | hmac_drbg_context * | ctx ) |
Free an HMAC_DRBG context.
- Parameters:
-
ctx HMAC_DRBG context to free.
Definition at line 312 of file hmac_drbg.c.
int hmac_drbg_init | ( | hmac_drbg_context * | ctx, |
const md_info_t * | md_info, | ||
int(*)(void *, unsigned char *, size_t) | f_entropy, | ||
void * | p_entropy, | ||
const unsigned char * | custom, | ||
size_t | len | ||
) |
HMAC_DRBG initialisation.
- Parameters:
-
ctx HMAC_DRBG context to be initialised md_info MD algorithm to use for HMAC_DRBG f_entropy Entropy callback (p_entropy, buffer to fill, buffer length) p_entropy Entropy context custom Personalization data (Device specific identifiers) (Can be NULL) len Length of personalization data
- Note:
- The "security strength" as defined by NIST is set to: 128 bits if md_alg is SHA-1, 192 bits if md_alg is SHA-224, 256 bits if md_alg is SHA-256 or higher. Note that SHA-256 is just as efficient as SHA-224.
- Returns:
- 0 if successful, or POLARSSL_ERR_MD_BAD_INPUT_DATA, or POLARSSL_ERR_MD_ALLOC_FAILED, or POLARSSL_ERR_CTR_DRBG_ENTROPY_SOURCE_FAILED.
Definition at line 160 of file hmac_drbg.c.
int hmac_drbg_init_buf | ( | hmac_drbg_context * | ctx, |
const md_info_t * | md_info, | ||
const unsigned char * | data, | ||
size_t | data_len | ||
) |
Initilisation of simpified HMAC_DRBG (never reseeds).
(For use with deterministic ECDSA.)
- Parameters:
-
ctx HMAC_DRBG context to be initialised md_info MD algorithm to use for HMAC_DRBG data Concatenation of entropy string and additional data data_len Length of data in bytes
- Returns:
- 0 if successful, or POLARSSL_ERR_MD_BAD_INPUT_DATA, or POLARSSL_ERR_MD_ALLOC_FAILED.
Definition at line 90 of file hmac_drbg.c.
int hmac_drbg_random | ( | void * | p_rng, |
unsigned char * | output, | ||
size_t | out_len | ||
) |
HMAC_DRBG generate random.
Note: Automatically reseeds if reseed_counter is reached or PR is enabled.
- Parameters:
-
p_rng HMAC_DRBG context output Buffer to fill out_len Length of the buffer
- Returns:
- 0 if successful, or POLARSSL_ERR_HMAC_DRBG_ENTROPY_SOURCE_FAILED, or POLARSSL_ERR_HMAC_DRBG_REQUEST_TOO_BIG
Definition at line 304 of file hmac_drbg.c.
int hmac_drbg_random_with_add | ( | void * | p_rng, |
unsigned char * | output, | ||
size_t | output_len, | ||
const unsigned char * | additional, | ||
size_t | add_len | ||
) |
HMAC_DRBG generate random with additional update input.
Note: Automatically reseeds if reseed_counter is reached or PR is enabled.
- Parameters:
-
p_rng HMAC_DRBG context output Buffer to fill output_len Length of the buffer additional Additional data to update with (can be NULL) add_len Length of additional data (can be 0)
- Returns:
- 0 if successful, or POLARSSL_ERR_HMAC_DRBG_ENTROPY_SOURCE_FAILED, or POLARSSL_ERR_HMAC_DRBG_REQUEST_TOO_BIG, or POLARSSL_ERR_HMAC_DRBG_INPUT_TOO_BIG.
Definition at line 244 of file hmac_drbg.c.
int hmac_drbg_reseed | ( | hmac_drbg_context * | ctx, |
const unsigned char * | additional, | ||
size_t | len | ||
) |
HMAC_DRBG reseeding (extracts data from entropy source)
- Parameters:
-
ctx HMAC_DRBG context additional Additional data to add to state (Can be NULL) len Length of additional data
- Returns:
- 0 if successful, or POLARSSL_ERR_HMAC_DRBG_ENTROPY_SOURCE_FAILED
Definition at line 119 of file hmac_drbg.c.
int hmac_drbg_self_test | ( | int | verbose ) |
Checkup routine.
- Returns:
- 0 if successful, or 1 if the test failed
Definition at line 450 of file hmac_drbg.c.
void hmac_drbg_set_entropy_len | ( | hmac_drbg_context * | ctx, |
size_t | len | ||
) |
Set the amount of entropy grabbed on each reseed (Default: given by the security strength, which depends on the hash used, see hmac_drbg_init()
)
- Parameters:
-
ctx HMAC_DRBG context len Amount of entropy to grab, in bytes
Definition at line 227 of file hmac_drbg.c.
void hmac_drbg_set_prediction_resistance | ( | hmac_drbg_context * | ctx, |
int | resistance | ||
) |
Enable / disable prediction resistance (Default: Off)
Note: If enabled, entropy is used for ctx->entropy_len before each call! Only use this if you have ample supply of good entropy!
- Parameters:
-
ctx HMAC_DRBG context resistance POLARSSL_HMAC_DRBG_PR_ON or POLARSSL_HMAC_DRBG_PR_OFF
Definition at line 218 of file hmac_drbg.c.
void hmac_drbg_set_reseed_interval | ( | hmac_drbg_context * | ctx, |
int | interval | ||
) |
Set the reseed interval (Default: POLARSSL_HMAC_DRBG_RESEED_INTERVAL)
- Parameters:
-
ctx HMAC_DRBG context interval Reseed interval
Definition at line 235 of file hmac_drbg.c.
void hmac_drbg_update | ( | hmac_drbg_context * | ctx, |
const unsigned char * | additional, | ||
size_t | add_len | ||
) |
HMAC_DRBG update state.
- Parameters:
-
ctx HMAC_DRBG context additional Additional data to update state with, or NULL add_len Length of additional data, or 0
- Note:
- Additional data is optional, pass NULL and 0 as second third argument if no additional data is being used.
Definition at line 62 of file hmac_drbg.c.
int hmac_drbg_update_seed_file | ( | hmac_drbg_context * | ctx, |
const char * | path | ||
) |
Read and update a seed file.
Seed is added to this instance
- Parameters:
-
ctx HMAC_DRBG context path Name of the file
- Returns:
- 0 if successful, 1 on file error, POLARSSL_ERR_HMAC_DRBG_ENTROPY_SOURCE_FAILED or POLARSSL_ERR_HMAC_DRBG_INPUT_TOO_BIG
Definition at line 348 of file hmac_drbg.c.
int hmac_drbg_write_seed_file | ( | hmac_drbg_context * | ctx, |
const char * | path | ||
) |
Write a seed file.
- Parameters:
-
ctx HMAC_DRBG context path Name of the file
- Returns:
- 0 if successful, 1 on file error, or POLARSSL_ERR_HMAC_DRBG_ENTROPY_SOURCE_FAILED
Definition at line 323 of file hmac_drbg.c.
Generated on Tue Jul 12 2022 13:50:40 by
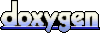