mbed TLS library
Dependents: HTTPClient-SSL WS_SERVER
hmac_drbg.h
00001 /** 00002 * \file hmac_drbg.h 00003 * 00004 * \brief HMAC_DRBG (NIST SP 800-90A) 00005 * 00006 * Copyright (C) 2014, ARM Limited, All Rights Reserved 00007 * 00008 * This file is part of mbed TLS (https://tls.mbed.org) 00009 * 00010 * This program is free software; you can redistribute it and/or modify 00011 * it under the terms of the GNU General Public License as published by 00012 * the Free Software Foundation; either version 2 of the License, or 00013 * (at your option) any later version. 00014 * 00015 * This program is distributed in the hope that it will be useful, 00016 * but WITHOUT ANY WARRANTY; without even the implied warranty of 00017 * MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the 00018 * GNU General Public License for more details. 00019 * 00020 * You should have received a copy of the GNU General Public License along 00021 * with this program; if not, write to the Free Software Foundation, Inc., 00022 * 51 Franklin Street, Fifth Floor, Boston, MA 02110-1301 USA. 00023 */ 00024 #ifndef POLARSSL_HMAC_DRBG_H 00025 #define POLARSSL_HMAC_DRBG_H 00026 00027 #include "md.h" 00028 00029 /* 00030 * Error codes 00031 */ 00032 #define POLARSSL_ERR_HMAC_DRBG_REQUEST_TOO_BIG -0x0003 /**< Too many random requested in single call. */ 00033 #define POLARSSL_ERR_HMAC_DRBG_INPUT_TOO_BIG -0x0005 /**< Input too large (Entropy + additional). */ 00034 #define POLARSSL_ERR_HMAC_DRBG_FILE_IO_ERROR -0x0007 /**< Read/write error in file. */ 00035 #define POLARSSL_ERR_HMAC_DRBG_ENTROPY_SOURCE_FAILED -0x0009 /**< The entropy source failed. */ 00036 00037 /** 00038 * \name SECTION: Module settings 00039 * 00040 * The configuration options you can set for this module are in this section. 00041 * Either change them in config.h or define them on the compiler command line. 00042 * \{ 00043 */ 00044 00045 #if !defined(POLARSSL_HMAC_DRBG_RESEED_INTERVAL) 00046 #define POLARSSL_HMAC_DRBG_RESEED_INTERVAL 10000 /**< Interval before reseed is performed by default */ 00047 #endif 00048 00049 #if !defined(POLARSSL_HMAC_DRBG_MAX_INPUT) 00050 #define POLARSSL_HMAC_DRBG_MAX_INPUT 256 /**< Maximum number of additional input bytes */ 00051 #endif 00052 00053 #if !defined(POLARSSL_HMAC_DRBG_MAX_REQUEST) 00054 #define POLARSSL_HMAC_DRBG_MAX_REQUEST 1024 /**< Maximum number of requested bytes per call */ 00055 #endif 00056 00057 #if !defined(POLARSSL_HMAC_DRBG_MAX_SEED_INPUT) 00058 #define POLARSSL_HMAC_DRBG_MAX_SEED_INPUT 384 /**< Maximum size of (re)seed buffer */ 00059 #endif 00060 00061 /* \} name SECTION: Module settings */ 00062 00063 #define POLARSSL_HMAC_DRBG_PR_OFF 0 /**< No prediction resistance */ 00064 #define POLARSSL_HMAC_DRBG_PR_ON 1 /**< Prediction resistance enabled */ 00065 00066 #ifdef __cplusplus 00067 extern "C" { 00068 #endif 00069 00070 /** 00071 * HMAC_DRBG context. 00072 */ 00073 typedef struct 00074 { 00075 /* Working state: the key K is not stored explicitely, 00076 * but is implied by the HMAC context */ 00077 md_context_t md_ctx ; /*!< HMAC context (inc. K) */ 00078 unsigned char V[POLARSSL_MD_MAX_SIZE]; /*!< V in the spec */ 00079 int reseed_counter ; /*!< reseed counter */ 00080 00081 /* Administrative state */ 00082 size_t entropy_len ; /*!< entropy bytes grabbed on each (re)seed */ 00083 int prediction_resistance; /*!< enable prediction resistance (Automatic 00084 reseed before every random generation) */ 00085 int reseed_interval ; /*!< reseed interval */ 00086 00087 /* Callbacks */ 00088 int (*f_entropy)(void *, unsigned char *, size_t); /*!< entropy function */ 00089 void *p_entropy ; /*!< context for the entropy function */ 00090 } hmac_drbg_context; 00091 00092 /** 00093 * \brief HMAC_DRBG initialisation 00094 * 00095 * \param ctx HMAC_DRBG context to be initialised 00096 * \param md_info MD algorithm to use for HMAC_DRBG 00097 * \param f_entropy Entropy callback (p_entropy, buffer to fill, buffer 00098 * length) 00099 * \param p_entropy Entropy context 00100 * \param custom Personalization data (Device specific identifiers) 00101 * (Can be NULL) 00102 * \param len Length of personalization data 00103 * 00104 * \note The "security strength" as defined by NIST is set to: 00105 * 128 bits if md_alg is SHA-1, 00106 * 192 bits if md_alg is SHA-224, 00107 * 256 bits if md_alg is SHA-256 or higher. 00108 * Note that SHA-256 is just as efficient as SHA-224. 00109 * 00110 * \return 0 if successful, or 00111 * POLARSSL_ERR_MD_BAD_INPUT_DATA, or 00112 * POLARSSL_ERR_MD_ALLOC_FAILED, or 00113 * POLARSSL_ERR_CTR_DRBG_ENTROPY_SOURCE_FAILED. 00114 */ 00115 int hmac_drbg_init( hmac_drbg_context *ctx, 00116 const md_info_t * md_info, 00117 int (*f_entropy)(void *, unsigned char *, size_t), 00118 void *p_entropy, 00119 const unsigned char *custom, 00120 size_t len ); 00121 00122 /** 00123 * \brief Initilisation of simpified HMAC_DRBG (never reseeds). 00124 * (For use with deterministic ECDSA.) 00125 * 00126 * \param ctx HMAC_DRBG context to be initialised 00127 * \param md_info MD algorithm to use for HMAC_DRBG 00128 * \param data Concatenation of entropy string and additional data 00129 * \param data_len Length of data in bytes 00130 * 00131 * \return 0 if successful, or 00132 * POLARSSL_ERR_MD_BAD_INPUT_DATA, or 00133 * POLARSSL_ERR_MD_ALLOC_FAILED. 00134 */ 00135 int hmac_drbg_init_buf( hmac_drbg_context *ctx, 00136 const md_info_t * md_info, 00137 const unsigned char *data, size_t data_len ); 00138 00139 /** 00140 * \brief Enable / disable prediction resistance (Default: Off) 00141 * 00142 * Note: If enabled, entropy is used for ctx->entropy_len before each call! 00143 * Only use this if you have ample supply of good entropy! 00144 * 00145 * \param ctx HMAC_DRBG context 00146 * \param resistance POLARSSL_HMAC_DRBG_PR_ON or POLARSSL_HMAC_DRBG_PR_OFF 00147 */ 00148 void hmac_drbg_set_prediction_resistance( hmac_drbg_context *ctx, 00149 int resistance ); 00150 00151 /** 00152 * \brief Set the amount of entropy grabbed on each reseed 00153 * (Default: given by the security strength, which 00154 * depends on the hash used, see \c hmac_drbg_init() ) 00155 * 00156 * \param ctx HMAC_DRBG context 00157 * \param len Amount of entropy to grab, in bytes 00158 */ 00159 void hmac_drbg_set_entropy_len( hmac_drbg_context *ctx, 00160 size_t len ); 00161 00162 /** 00163 * \brief Set the reseed interval 00164 * (Default: POLARSSL_HMAC_DRBG_RESEED_INTERVAL) 00165 * 00166 * \param ctx HMAC_DRBG context 00167 * \param interval Reseed interval 00168 */ 00169 void hmac_drbg_set_reseed_interval( hmac_drbg_context *ctx, 00170 int interval ); 00171 00172 /** 00173 * \brief HMAC_DRBG update state 00174 * 00175 * \param ctx HMAC_DRBG context 00176 * \param additional Additional data to update state with, or NULL 00177 * \param add_len Length of additional data, or 0 00178 * 00179 * \note Additional data is optional, pass NULL and 0 as second 00180 * third argument if no additional data is being used. 00181 */ 00182 void hmac_drbg_update( hmac_drbg_context *ctx, 00183 const unsigned char *additional, size_t add_len ); 00184 00185 /** 00186 * \brief HMAC_DRBG reseeding (extracts data from entropy source) 00187 * 00188 * \param ctx HMAC_DRBG context 00189 * \param additional Additional data to add to state (Can be NULL) 00190 * \param len Length of additional data 00191 * 00192 * \return 0 if successful, or 00193 * POLARSSL_ERR_HMAC_DRBG_ENTROPY_SOURCE_FAILED 00194 */ 00195 int hmac_drbg_reseed( hmac_drbg_context *ctx, 00196 const unsigned char *additional, size_t len ); 00197 00198 /** 00199 * \brief HMAC_DRBG generate random with additional update input 00200 * 00201 * Note: Automatically reseeds if reseed_counter is reached or PR is enabled. 00202 * 00203 * \param p_rng HMAC_DRBG context 00204 * \param output Buffer to fill 00205 * \param output_len Length of the buffer 00206 * \param additional Additional data to update with (can be NULL) 00207 * \param add_len Length of additional data (can be 0) 00208 * 00209 * \return 0 if successful, or 00210 * POLARSSL_ERR_HMAC_DRBG_ENTROPY_SOURCE_FAILED, or 00211 * POLARSSL_ERR_HMAC_DRBG_REQUEST_TOO_BIG, or 00212 * POLARSSL_ERR_HMAC_DRBG_INPUT_TOO_BIG. 00213 */ 00214 int hmac_drbg_random_with_add( void *p_rng, 00215 unsigned char *output, size_t output_len, 00216 const unsigned char *additional, 00217 size_t add_len ); 00218 00219 /** 00220 * \brief HMAC_DRBG generate random 00221 * 00222 * Note: Automatically reseeds if reseed_counter is reached or PR is enabled. 00223 * 00224 * \param p_rng HMAC_DRBG context 00225 * \param output Buffer to fill 00226 * \param out_len Length of the buffer 00227 * 00228 * \return 0 if successful, or 00229 * POLARSSL_ERR_HMAC_DRBG_ENTROPY_SOURCE_FAILED, or 00230 * POLARSSL_ERR_HMAC_DRBG_REQUEST_TOO_BIG 00231 */ 00232 int hmac_drbg_random( void *p_rng, unsigned char *output, size_t out_len ); 00233 00234 /** 00235 * \brief Free an HMAC_DRBG context 00236 * 00237 * \param ctx HMAC_DRBG context to free. 00238 */ 00239 void hmac_drbg_free( hmac_drbg_context *ctx ); 00240 00241 #if defined(POLARSSL_FS_IO) 00242 /** 00243 * \brief Write a seed file 00244 * 00245 * \param ctx HMAC_DRBG context 00246 * \param path Name of the file 00247 * 00248 * \return 0 if successful, 1 on file error, or 00249 * POLARSSL_ERR_HMAC_DRBG_ENTROPY_SOURCE_FAILED 00250 */ 00251 int hmac_drbg_write_seed_file( hmac_drbg_context *ctx, const char *path ); 00252 00253 /** 00254 * \brief Read and update a seed file. Seed is added to this 00255 * instance 00256 * 00257 * \param ctx HMAC_DRBG context 00258 * \param path Name of the file 00259 * 00260 * \return 0 if successful, 1 on file error, 00261 * POLARSSL_ERR_HMAC_DRBG_ENTROPY_SOURCE_FAILED or 00262 * POLARSSL_ERR_HMAC_DRBG_INPUT_TOO_BIG 00263 */ 00264 int hmac_drbg_update_seed_file( hmac_drbg_context *ctx, const char *path ); 00265 #endif /* POLARSSL_FS_IO */ 00266 00267 00268 #if defined(POLARSSL_SELF_TEST) 00269 /** 00270 * \brief Checkup routine 00271 * 00272 * \return 0 if successful, or 1 if the test failed 00273 */ 00274 int hmac_drbg_self_test( int verbose ); 00275 #endif 00276 00277 #ifdef __cplusplus 00278 } 00279 #endif 00280 00281 #endif /* hmac_drbg.h */ 00282
Generated on Tue Jul 12 2022 13:50:37 by
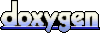