mbed TLS library
Dependents: HTTPClient-SSL WS_SERVER
ecdsa.h File Reference
Elliptic curve DSA. More...
Go to the source code of this file.
Data Structures | |
struct | ecdsa_context |
ECDSA context structure. More... | |
Functions | |
int | ecdsa_sign (ecp_group *grp, mpi *r, mpi *s, const mpi *d, const unsigned char *buf, size_t blen, int(*f_rng)(void *, unsigned char *, size_t), void *p_rng) |
Compute ECDSA signature of a previously hashed message. | |
int | ecdsa_sign_det (ecp_group *grp, mpi *r, mpi *s, const mpi *d, const unsigned char *buf, size_t blen, md_type_t md_alg) |
Compute ECDSA signature of a previously hashed message (deterministic version) | |
int | ecdsa_verify (ecp_group *grp, const unsigned char *buf, size_t blen, const ecp_point *Q, const mpi *r, const mpi *s) |
Verify ECDSA signature of a previously hashed message. | |
int | ecdsa_write_signature (ecdsa_context *ctx, const unsigned char *hash, size_t hlen, unsigned char *sig, size_t *slen, int(*f_rng)(void *, unsigned char *, size_t), void *p_rng) |
Compute ECDSA signature and write it to buffer, serialized as defined in RFC 4492 page 20. | |
int | ecdsa_write_signature_det (ecdsa_context *ctx, const unsigned char *hash, size_t hlen, unsigned char *sig, size_t *slen, md_type_t md_alg) |
Compute ECDSA signature and write it to buffer, serialized as defined in RFC 4492 page 20. | |
int | ecdsa_read_signature (ecdsa_context *ctx, const unsigned char *hash, size_t hlen, const unsigned char *sig, size_t slen) |
Read and verify an ECDSA signature. | |
int | ecdsa_genkey (ecdsa_context *ctx, ecp_group_id gid, int(*f_rng)(void *, unsigned char *, size_t), void *p_rng) |
Generate an ECDSA keypair on the given curve. | |
int | ecdsa_from_keypair (ecdsa_context *ctx, const ecp_keypair *key) |
Set an ECDSA context from an EC key pair. | |
void | ecdsa_init (ecdsa_context *ctx) |
Initialize context. | |
void | ecdsa_free (ecdsa_context *ctx) |
Free context. | |
int | ecdsa_self_test (int verbose) |
Checkup routine. |
Detailed Description
Elliptic curve DSA.
Copyright (C) 2006-2013, ARM Limited, All Rights Reserved
This file is part of mbed TLS (https://tls.mbed.org)
This program is free software; you can redistribute it and/or modify it under the terms of the GNU General Public License as published by the Free Software Foundation; either version 2 of the License, or (at your option) any later version.
This program is distributed in the hope that it will be useful, but WITHOUT ANY WARRANTY; without even the implied warranty of MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU General Public License for more details.
You should have received a copy of the GNU General Public License along with this program; if not, write to the Free Software Foundation, Inc., 51 Franklin Street, Fifth Floor, Boston, MA 02110-1301 USA.
Definition in file ecdsa.h.
Function Documentation
void ecdsa_free | ( | ecdsa_context * | ctx ) |
int ecdsa_from_keypair | ( | ecdsa_context * | ctx, |
const ecp_keypair * | key | ||
) |
int ecdsa_genkey | ( | ecdsa_context * | ctx, |
ecp_group_id | gid, | ||
int(*)(void *, unsigned char *, size_t) | f_rng, | ||
void * | p_rng | ||
) |
Generate an ECDSA keypair on the given curve.
- Parameters:
-
ctx ECDSA context in which the keypair should be stored gid Group (elliptic curve) to use. One of the various POLARSSL_ECP_DP_XXX macros depending on configuration. f_rng RNG function p_rng RNG parameter
- Returns:
- 0 on success, or a POLARSSL_ERR_ECP code.
void ecdsa_init | ( | ecdsa_context * | ctx ) |
int ecdsa_read_signature | ( | ecdsa_context * | ctx, |
const unsigned char * | hash, | ||
size_t | hlen, | ||
const unsigned char * | sig, | ||
size_t | slen | ||
) |
Read and verify an ECDSA signature.
- Parameters:
-
ctx ECDSA context hash Message hash hlen Size of hash sig Signature to read and verify slen Size of sig
- Returns:
- 0 if successful, POLARSSL_ERR_ECP_BAD_INPUT_DATA if signature is invalid, POLARSSL_ERR_ECP_SIG_LEN_MISTMATCH if the signature is valid but its actual length is less than siglen, or a POLARSSL_ERR_ECP or POLARSSL_ERR_MPI error code
int ecdsa_self_test | ( | int | verbose ) |
int ecdsa_sign | ( | ecp_group * | grp, |
mpi * | r, | ||
mpi * | s, | ||
const mpi * | d, | ||
const unsigned char * | buf, | ||
size_t | blen, | ||
int(*)(void *, unsigned char *, size_t) | f_rng, | ||
void * | p_rng | ||
) |
Compute ECDSA signature of a previously hashed message.
- Parameters:
-
grp ECP group r First output integer s Second output integer d Private signing key buf Message hash blen Length of buf f_rng RNG function p_rng RNG parameter
- Returns:
- 0 if successful, or a POLARSSL_ERR_ECP_XXX or POLARSSL_MPI_XXX error code
int ecdsa_sign_det | ( | ecp_group * | grp, |
mpi * | r, | ||
mpi * | s, | ||
const mpi * | d, | ||
const unsigned char * | buf, | ||
size_t | blen, | ||
md_type_t | md_alg | ||
) |
Compute ECDSA signature of a previously hashed message (deterministic version)
- Parameters:
-
grp ECP group r First output integer s Second output integer d Private signing key buf Message hash blen Length of buf md_alg MD algorithm used to hash the message
- Returns:
- 0 if successful, or a POLARSSL_ERR_ECP_XXX or POLARSSL_MPI_XXX error code
int ecdsa_verify | ( | ecp_group * | grp, |
const unsigned char * | buf, | ||
size_t | blen, | ||
const ecp_point * | Q, | ||
const mpi * | r, | ||
const mpi * | s | ||
) |
Verify ECDSA signature of a previously hashed message.
- Parameters:
-
grp ECP group buf Message hash blen Length of buf Q Public key to use for verification r First integer of the signature s Second integer of the signature
- Returns:
- 0 if successful, POLARSSL_ERR_ECP_BAD_INPUT_DATA if signature is invalid or a POLARSSL_ERR_ECP_XXX or POLARSSL_MPI_XXX error code
int ecdsa_write_signature | ( | ecdsa_context * | ctx, |
const unsigned char * | hash, | ||
size_t | hlen, | ||
unsigned char * | sig, | ||
size_t * | slen, | ||
int(*)(void *, unsigned char *, size_t) | f_rng, | ||
void * | p_rng | ||
) |
Compute ECDSA signature and write it to buffer, serialized as defined in RFC 4492 page 20.
(Not thread-safe to use same context in multiple threads)
- Parameters:
-
ctx ECDSA context hash Message hash hlen Length of hash sig Buffer that will hold the signature slen Length of the signature written f_rng RNG function p_rng RNG parameter
- Note:
- The "sig" buffer must be at least as large as twice the size of the curve used, plus 7 (eg. 71 bytes if a 256-bit curve is used).
- Returns:
- 0 if successful, or a POLARSSL_ERR_ECP, POLARSSL_ERR_MPI or POLARSSL_ERR_ASN1 error code
int ecdsa_write_signature_det | ( | ecdsa_context * | ctx, |
const unsigned char * | hash, | ||
size_t | hlen, | ||
unsigned char * | sig, | ||
size_t * | slen, | ||
md_type_t | md_alg | ||
) |
Compute ECDSA signature and write it to buffer, serialized as defined in RFC 4492 page 20.
Deterministic version, RFC 6979. (Not thread-safe to use same context in multiple threads)
- Parameters:
-
ctx ECDSA context hash Message hash hlen Length of hash sig Buffer that will hold the signature slen Length of the signature written md_alg MD algorithm used to hash the message
- Note:
- The "sig" buffer must be at least as large as twice the size of the curve used, plus 7 (eg. 71 bytes if a 256-bit curve is used).
- Returns:
- 0 if successful, or a POLARSSL_ERR_ECP, POLARSSL_ERR_MPI or POLARSSL_ERR_ASN1 error code
Generated on Tue Jul 12 2022 13:50:39 by
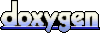