mbed TLS library
Dependents: HTTPClient-SSL WS_SERVER
ecdsa.h
00001 /** 00002 * \file ecdsa.h 00003 * 00004 * \brief Elliptic curve DSA 00005 * 00006 * Copyright (C) 2006-2013, ARM Limited, All Rights Reserved 00007 * 00008 * This file is part of mbed TLS (https://tls.mbed.org) 00009 * 00010 * This program is free software; you can redistribute it and/or modify 00011 * it under the terms of the GNU General Public License as published by 00012 * the Free Software Foundation; either version 2 of the License, or 00013 * (at your option) any later version. 00014 * 00015 * This program is distributed in the hope that it will be useful, 00016 * but WITHOUT ANY WARRANTY; without even the implied warranty of 00017 * MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the 00018 * GNU General Public License for more details. 00019 * 00020 * You should have received a copy of the GNU General Public License along 00021 * with this program; if not, write to the Free Software Foundation, Inc., 00022 * 51 Franklin Street, Fifth Floor, Boston, MA 02110-1301 USA. 00023 */ 00024 #ifndef POLARSSL_ECDSA_H 00025 #define POLARSSL_ECDSA_H 00026 00027 #include "ecp.h" 00028 #include "md.h" 00029 00030 /** 00031 * \brief ECDSA context structure 00032 * 00033 * \note Purposefully begins with the same members as struct ecp_keypair. 00034 */ 00035 typedef struct 00036 { 00037 ecp_group grp ; /*!< elliptic curve used */ 00038 mpi d ; /*!< secret signature key */ 00039 ecp_point Q ; /*!< public signature key */ 00040 mpi r ; /*!< first integer from signature */ 00041 mpi s ; /*!< second integer from signature */ 00042 } 00043 ecdsa_context; 00044 00045 #ifdef __cplusplus 00046 extern "C" { 00047 #endif 00048 00049 /** 00050 * \brief Compute ECDSA signature of a previously hashed message 00051 * 00052 * \param grp ECP group 00053 * \param r First output integer 00054 * \param s Second output integer 00055 * \param d Private signing key 00056 * \param buf Message hash 00057 * \param blen Length of buf 00058 * \param f_rng RNG function 00059 * \param p_rng RNG parameter 00060 * 00061 * \return 0 if successful, 00062 * or a POLARSSL_ERR_ECP_XXX or POLARSSL_MPI_XXX error code 00063 */ 00064 int ecdsa_sign( ecp_group *grp, mpi *r, mpi *s, 00065 const mpi *d, const unsigned char *buf, size_t blen, 00066 int (*f_rng)(void *, unsigned char *, size_t), void *p_rng ); 00067 00068 #if defined(POLARSSL_ECDSA_DETERMINISTIC) 00069 /** 00070 * \brief Compute ECDSA signature of a previously hashed message 00071 * (deterministic version) 00072 * 00073 * \param grp ECP group 00074 * \param r First output integer 00075 * \param s Second output integer 00076 * \param d Private signing key 00077 * \param buf Message hash 00078 * \param blen Length of buf 00079 * \param md_alg MD algorithm used to hash the message 00080 * 00081 * \return 0 if successful, 00082 * or a POLARSSL_ERR_ECP_XXX or POLARSSL_MPI_XXX error code 00083 */ 00084 int ecdsa_sign_det( ecp_group *grp, mpi *r, mpi *s, 00085 const mpi *d, const unsigned char *buf, size_t blen, 00086 md_type_t md_alg ); 00087 #endif /* POLARSSL_ECDSA_DETERMINISTIC */ 00088 00089 /** 00090 * \brief Verify ECDSA signature of a previously hashed message 00091 * 00092 * \param grp ECP group 00093 * \param buf Message hash 00094 * \param blen Length of buf 00095 * \param Q Public key to use for verification 00096 * \param r First integer of the signature 00097 * \param s Second integer of the signature 00098 * 00099 * \return 0 if successful, 00100 * POLARSSL_ERR_ECP_BAD_INPUT_DATA if signature is invalid 00101 * or a POLARSSL_ERR_ECP_XXX or POLARSSL_MPI_XXX error code 00102 */ 00103 int ecdsa_verify( ecp_group *grp, 00104 const unsigned char *buf, size_t blen, 00105 const ecp_point *Q, const mpi *r, const mpi *s); 00106 00107 /** 00108 * \brief Compute ECDSA signature and write it to buffer, 00109 * serialized as defined in RFC 4492 page 20. 00110 * (Not thread-safe to use same context in multiple threads) 00111 * 00112 * \param ctx ECDSA context 00113 * \param hash Message hash 00114 * \param hlen Length of hash 00115 * \param sig Buffer that will hold the signature 00116 * \param slen Length of the signature written 00117 * \param f_rng RNG function 00118 * \param p_rng RNG parameter 00119 * 00120 * \note The "sig" buffer must be at least as large as twice the 00121 * size of the curve used, plus 7 (eg. 71 bytes if a 256-bit 00122 * curve is used). 00123 * 00124 * \return 0 if successful, 00125 * or a POLARSSL_ERR_ECP, POLARSSL_ERR_MPI or 00126 * POLARSSL_ERR_ASN1 error code 00127 */ 00128 int ecdsa_write_signature( ecdsa_context *ctx, 00129 const unsigned char *hash, size_t hlen, 00130 unsigned char *sig, size_t *slen, 00131 int (*f_rng)(void *, unsigned char *, size_t), 00132 void *p_rng ); 00133 00134 #if defined(POLARSSL_ECDSA_DETERMINISTIC) 00135 /** 00136 * \brief Compute ECDSA signature and write it to buffer, 00137 * serialized as defined in RFC 4492 page 20. 00138 * Deterministic version, RFC 6979. 00139 * (Not thread-safe to use same context in multiple threads) 00140 * 00141 * \param ctx ECDSA context 00142 * \param hash Message hash 00143 * \param hlen Length of hash 00144 * \param sig Buffer that will hold the signature 00145 * \param slen Length of the signature written 00146 * \param md_alg MD algorithm used to hash the message 00147 * 00148 * \note The "sig" buffer must be at least as large as twice the 00149 * size of the curve used, plus 7 (eg. 71 bytes if a 256-bit 00150 * curve is used). 00151 * 00152 * \return 0 if successful, 00153 * or a POLARSSL_ERR_ECP, POLARSSL_ERR_MPI or 00154 * POLARSSL_ERR_ASN1 error code 00155 */ 00156 int ecdsa_write_signature_det( ecdsa_context *ctx, 00157 const unsigned char *hash, size_t hlen, 00158 unsigned char *sig, size_t *slen, 00159 md_type_t md_alg ); 00160 #endif /* POLARSSL_ECDSA_DETERMINISTIC */ 00161 00162 /** 00163 * \brief Read and verify an ECDSA signature 00164 * 00165 * \param ctx ECDSA context 00166 * \param hash Message hash 00167 * \param hlen Size of hash 00168 * \param sig Signature to read and verify 00169 * \param slen Size of sig 00170 * 00171 * \return 0 if successful, 00172 * POLARSSL_ERR_ECP_BAD_INPUT_DATA if signature is invalid, 00173 * POLARSSL_ERR_ECP_SIG_LEN_MISTMATCH if the signature is 00174 * valid but its actual length is less than siglen, 00175 * or a POLARSSL_ERR_ECP or POLARSSL_ERR_MPI error code 00176 */ 00177 int ecdsa_read_signature( ecdsa_context *ctx, 00178 const unsigned char *hash, size_t hlen, 00179 const unsigned char *sig, size_t slen ); 00180 00181 /** 00182 * \brief Generate an ECDSA keypair on the given curve 00183 * 00184 * \param ctx ECDSA context in which the keypair should be stored 00185 * \param gid Group (elliptic curve) to use. One of the various 00186 * POLARSSL_ECP_DP_XXX macros depending on configuration. 00187 * \param f_rng RNG function 00188 * \param p_rng RNG parameter 00189 * 00190 * \return 0 on success, or a POLARSSL_ERR_ECP code. 00191 */ 00192 int ecdsa_genkey( ecdsa_context *ctx, ecp_group_id gid, 00193 int (*f_rng)(void *, unsigned char *, size_t), void *p_rng ); 00194 00195 /** 00196 * \brief Set an ECDSA context from an EC key pair 00197 * 00198 * \param ctx ECDSA context to set 00199 * \param key EC key to use 00200 * 00201 * \return 0 on success, or a POLARSSL_ERR_ECP code. 00202 */ 00203 int ecdsa_from_keypair( ecdsa_context *ctx, const ecp_keypair *key ); 00204 00205 /** 00206 * \brief Initialize context 00207 * 00208 * \param ctx Context to initialize 00209 */ 00210 void ecdsa_init( ecdsa_context *ctx ); 00211 00212 /** 00213 * \brief Free context 00214 * 00215 * \param ctx Context to free 00216 */ 00217 void ecdsa_free( ecdsa_context *ctx ); 00218 00219 /** 00220 * \brief Checkup routine 00221 * 00222 * \return 0 if successful, or 1 if the test failed 00223 */ 00224 int ecdsa_self_test( int verbose ); 00225 00226 #ifdef __cplusplus 00227 } 00228 #endif 00229 00230 #endif /* ecdsa.h */ 00231
Generated on Tue Jul 12 2022 13:50:37 by
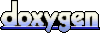