mbed TLS library
Dependents: HTTPClient-SSL WS_SERVER
ecdh.h
00001 /** 00002 * \file ecdh.h 00003 * 00004 * \brief Elliptic curve Diffie-Hellman 00005 * 00006 * Copyright (C) 2006-2013, ARM Limited, All Rights Reserved 00007 * 00008 * This file is part of mbed TLS (https://tls.mbed.org) 00009 * 00010 * This program is free software; you can redistribute it and/or modify 00011 * it under the terms of the GNU General Public License as published by 00012 * the Free Software Foundation; either version 2 of the License, or 00013 * (at your option) any later version. 00014 * 00015 * This program is distributed in the hope that it will be useful, 00016 * but WITHOUT ANY WARRANTY; without even the implied warranty of 00017 * MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the 00018 * GNU General Public License for more details. 00019 * 00020 * You should have received a copy of the GNU General Public License along 00021 * with this program; if not, write to the Free Software Foundation, Inc., 00022 * 51 Franklin Street, Fifth Floor, Boston, MA 02110-1301 USA. 00023 */ 00024 #ifndef POLARSSL_ECDH_H 00025 #define POLARSSL_ECDH_H 00026 00027 #include "ecp.h" 00028 00029 #ifdef __cplusplus 00030 extern "C" { 00031 #endif 00032 00033 /** 00034 * When importing from an EC key, select if it is our key or the peer's key 00035 */ 00036 typedef enum 00037 { 00038 POLARSSL_ECDH_OURS, 00039 POLARSSL_ECDH_THEIRS, 00040 } ecdh_side; 00041 00042 /** 00043 * \brief ECDH context structure 00044 */ 00045 typedef struct 00046 { 00047 ecp_group grp ; /*!< elliptic curve used */ 00048 mpi d ; /*!< our secret value (private key) */ 00049 ecp_point Q ; /*!< our public value (public key) */ 00050 ecp_point Qp ; /*!< peer's public value (public key) */ 00051 mpi z ; /*!< shared secret */ 00052 int point_format ; /*!< format for point export in TLS messages */ 00053 ecp_point Vi ; /*!< blinding value (for later) */ 00054 ecp_point Vf ; /*!< un-blinding value (for later) */ 00055 mpi _d ; /*!< previous d (for later) */ 00056 } 00057 ecdh_context; 00058 00059 /** 00060 * \brief Generate a public key. 00061 * Raw function that only does the core computation. 00062 * 00063 * \param grp ECP group 00064 * \param d Destination MPI (secret exponent, aka private key) 00065 * \param Q Destination point (public key) 00066 * \param f_rng RNG function 00067 * \param p_rng RNG parameter 00068 * 00069 * \return 0 if successful, 00070 * or a POLARSSL_ERR_ECP_XXX or POLARSSL_MPI_XXX error code 00071 */ 00072 int ecdh_gen_public( ecp_group *grp, mpi *d, ecp_point *Q, 00073 int (*f_rng)(void *, unsigned char *, size_t), 00074 void *p_rng ); 00075 00076 /** 00077 * \brief Compute shared secret 00078 * Raw function that only does the core computation. 00079 * 00080 * \param grp ECP group 00081 * \param z Destination MPI (shared secret) 00082 * \param Q Public key from other party 00083 * \param d Our secret exponent (private key) 00084 * \param f_rng RNG function (see notes) 00085 * \param p_rng RNG parameter 00086 * 00087 * \return 0 if successful, 00088 * or a POLARSSL_ERR_ECP_XXX or POLARSSL_MPI_XXX error code 00089 * 00090 * \note If f_rng is not NULL, it is used to implement 00091 * countermeasures against potential elaborate timing 00092 * attacks, see \c ecp_mul() for details. 00093 */ 00094 int ecdh_compute_shared( ecp_group *grp, mpi *z, 00095 const ecp_point *Q, const mpi *d, 00096 int (*f_rng)(void *, unsigned char *, size_t), 00097 void *p_rng ); 00098 00099 /** 00100 * \brief Initialize context 00101 * 00102 * \param ctx Context to initialize 00103 */ 00104 void ecdh_init( ecdh_context *ctx ); 00105 00106 /** 00107 * \brief Free context 00108 * 00109 * \param ctx Context to free 00110 */ 00111 void ecdh_free( ecdh_context *ctx ); 00112 00113 /** 00114 * \brief Generate a public key and a TLS ServerKeyExchange payload. 00115 * (First function used by a TLS server for ECDHE.) 00116 * 00117 * \param ctx ECDH context 00118 * \param olen number of chars written 00119 * \param buf destination buffer 00120 * \param blen length of buffer 00121 * \param f_rng RNG function 00122 * \param p_rng RNG parameter 00123 * 00124 * \note This function assumes that ctx->grp has already been 00125 * properly set (for example using ecp_use_known_dp). 00126 * 00127 * \return 0 if successful, or an POLARSSL_ERR_ECP_XXX error code 00128 */ 00129 int ecdh_make_params( ecdh_context *ctx, size_t *olen, 00130 unsigned char *buf, size_t blen, 00131 int (*f_rng)(void *, unsigned char *, size_t), 00132 void *p_rng ); 00133 00134 /** 00135 * \brief Parse and procress a TLS ServerKeyExhange payload. 00136 * (First function used by a TLS client for ECDHE.) 00137 * 00138 * \param ctx ECDH context 00139 * \param buf pointer to start of input buffer 00140 * \param end one past end of buffer 00141 * 00142 * \return 0 if successful, or an POLARSSL_ERR_ECP_XXX error code 00143 */ 00144 int ecdh_read_params( ecdh_context *ctx, 00145 const unsigned char **buf, const unsigned char *end ); 00146 00147 /** 00148 * \brief Setup an ECDH context from an EC key. 00149 * (Used by clients and servers in place of the 00150 * ServerKeyEchange for static ECDH: import ECDH parameters 00151 * from a certificate's EC key information.) 00152 * 00153 * \param ctx ECDH constext to set 00154 * \param key EC key to use 00155 * \param side Is it our key (1) or the peer's key (0) ? 00156 * 00157 * \return 0 if successful, or an POLARSSL_ERR_ECP_XXX error code 00158 */ 00159 int ecdh_get_params( ecdh_context *ctx, const ecp_keypair *key, 00160 ecdh_side side ); 00161 00162 /** 00163 * \brief Generate a public key and a TLS ClientKeyExchange payload. 00164 * (Second function used by a TLS client for ECDH(E).) 00165 * 00166 * \param ctx ECDH context 00167 * \param olen number of bytes actually written 00168 * \param buf destination buffer 00169 * \param blen size of destination buffer 00170 * \param f_rng RNG function 00171 * \param p_rng RNG parameter 00172 * 00173 * \return 0 if successful, or an POLARSSL_ERR_ECP_XXX error code 00174 */ 00175 int ecdh_make_public( ecdh_context *ctx, size_t *olen, 00176 unsigned char *buf, size_t blen, 00177 int (*f_rng)(void *, unsigned char *, size_t), 00178 void *p_rng ); 00179 00180 /** 00181 * \brief Parse and process a TLS ClientKeyExchange payload. 00182 * (Second function used by a TLS server for ECDH(E).) 00183 * 00184 * \param ctx ECDH context 00185 * \param buf start of input buffer 00186 * \param blen length of input buffer 00187 * 00188 * \return 0 if successful, or an POLARSSL_ERR_ECP_XXX error code 00189 */ 00190 int ecdh_read_public( ecdh_context *ctx, 00191 const unsigned char *buf, size_t blen ); 00192 00193 /** 00194 * \brief Derive and export the shared secret. 00195 * (Last function used by both TLS client en servers.) 00196 * 00197 * \param ctx ECDH context 00198 * \param olen number of bytes written 00199 * \param buf destination buffer 00200 * \param blen buffer length 00201 * \param f_rng RNG function, see notes for \c ecdh_compute_shared() 00202 * \param p_rng RNG parameter 00203 * 00204 * \return 0 if successful, or an POLARSSL_ERR_ECP_XXX error code 00205 */ 00206 int ecdh_calc_secret( ecdh_context *ctx, size_t *olen, 00207 unsigned char *buf, size_t blen, 00208 int (*f_rng)(void *, unsigned char *, size_t), 00209 void *p_rng ); 00210 00211 /** 00212 * \brief Checkup routine 00213 * 00214 * \return 0 if successful, or 1 if the test failed 00215 */ 00216 int ecdh_self_test( int verbose ); 00217 00218 #ifdef __cplusplus 00219 } 00220 #endif 00221 00222 #endif /* ecdh.h */ 00223
Generated on Tue Jul 12 2022 13:50:37 by
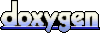