mbed TLS library
Dependents: HTTPClient-SSL WS_SERVER
ctr_drbg.h File Reference
CTR_DRBG based on AES-256 (NIST SP 800-90) More...
Go to the source code of this file.
Data Structures | |
struct | ctr_drbg_context |
CTR_DRBG context structure. More... | |
Functions | |
int | ctr_drbg_init (ctr_drbg_context *ctx, int(*f_entropy)(void *, unsigned char *, size_t), void *p_entropy, const unsigned char *custom, size_t len) |
CTR_DRBG initialization. | |
void | ctr_drbg_free (ctr_drbg_context *ctx) |
Clear CTR_CRBG context data. | |
void | ctr_drbg_set_prediction_resistance (ctr_drbg_context *ctx, int resistance) |
Enable / disable prediction resistance (Default: Off) | |
void | ctr_drbg_set_entropy_len (ctr_drbg_context *ctx, size_t len) |
Set the amount of entropy grabbed on each (re)seed (Default: CTR_DRBG_ENTROPY_LEN) | |
void | ctr_drbg_set_reseed_interval (ctr_drbg_context *ctx, int interval) |
Set the reseed interval (Default: CTR_DRBG_RESEED_INTERVAL) | |
int | ctr_drbg_reseed (ctr_drbg_context *ctx, const unsigned char *additional, size_t len) |
CTR_DRBG reseeding (extracts data from entropy source) | |
void | ctr_drbg_update (ctr_drbg_context *ctx, const unsigned char *additional, size_t add_len) |
CTR_DRBG update state. | |
int | ctr_drbg_random_with_add (void *p_rng, unsigned char *output, size_t output_len, const unsigned char *additional, size_t add_len) |
CTR_DRBG generate random with additional update input. | |
int | ctr_drbg_random (void *p_rng, unsigned char *output, size_t output_len) |
CTR_DRBG generate random. | |
int | ctr_drbg_write_seed_file (ctr_drbg_context *ctx, const char *path) |
Write a seed file. | |
int | ctr_drbg_update_seed_file (ctr_drbg_context *ctx, const char *path) |
Read and update a seed file. | |
int | ctr_drbg_self_test (int verbose) |
Checkup routine. |
Detailed Description
CTR_DRBG based on AES-256 (NIST SP 800-90)
Copyright (C) 2006-2014, ARM Limited, All Rights Reserved
This file is part of mbed TLS (https://tls.mbed.org)
This program is free software; you can redistribute it and/or modify it under the terms of the GNU General Public License as published by the Free Software Foundation; either version 2 of the License, or (at your option) any later version.
This program is distributed in the hope that it will be useful, but WITHOUT ANY WARRANTY; without even the implied warranty of MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU General Public License for more details.
You should have received a copy of the GNU General Public License along with this program; if not, write to the Free Software Foundation, Inc., 51 Franklin Street, Fifth Floor, Boston, MA 02110-1301 USA.
Definition in file ctr_drbg.h.
Function Documentation
void ctr_drbg_free | ( | ctr_drbg_context * | ctx ) |
Clear CTR_CRBG context data.
- Parameters:
-
ctx CTR_DRBG context to clear
Definition at line 105 of file ctr_drbg.c.
int ctr_drbg_init | ( | ctr_drbg_context * | ctx, |
int(*)(void *, unsigned char *, size_t) | f_entropy, | ||
void * | p_entropy, | ||
const unsigned char * | custom, | ||
size_t | len | ||
) |
CTR_DRBG initialization.
Note: Personalization data can be provided in addition to the more generic entropy source to make this instantiation as unique as possible.
- Parameters:
-
ctx CTR_DRBG context to be initialized f_entropy Entropy callback (p_entropy, buffer to fill, buffer length) p_entropy Entropy context custom Personalization data (Device specific identifiers) (Can be NULL) len Length of personalization data
- Returns:
- 0 if successful, or POLARSSL_ERR_CTR_DRBG_ENTROPY_SOURCE_FAILED
Definition at line 95 of file ctr_drbg.c.
int ctr_drbg_random | ( | void * | p_rng, |
unsigned char * | output, | ||
size_t | output_len | ||
) |
CTR_DRBG generate random.
Note: Automatically reseeds if reseed_counter is reached.
- Parameters:
-
p_rng CTR_DRBG context output Buffer to fill output_len Length of the buffer
- Returns:
- 0 if successful, or POLARSSL_ERR_CTR_DRBG_ENTROPY_SOURCE_FAILED, or POLARSSL_ERR_CTR_DRBG_REQUEST_TOO_BIG
Definition at line 385 of file ctr_drbg.c.
int ctr_drbg_random_with_add | ( | void * | p_rng, |
unsigned char * | output, | ||
size_t | output_len, | ||
const unsigned char * | additional, | ||
size_t | add_len | ||
) |
CTR_DRBG generate random with additional update input.
Note: Automatically reseeds if reseed_counter is reached.
- Parameters:
-
p_rng CTR_DRBG context output Buffer to fill output_len Length of the buffer additional Additional data to update with (Can be NULL) add_len Length of additional data
- Returns:
- 0 if successful, or POLARSSL_ERR_CTR_DRBG_ENTROPY_SOURCE_FAILED, or POLARSSL_ERR_CTR_DRBG_REQUEST_TOO_BIG
Definition at line 319 of file ctr_drbg.c.
int ctr_drbg_reseed | ( | ctr_drbg_context * | ctx, |
const unsigned char * | additional, | ||
size_t | len | ||
) |
CTR_DRBG reseeding (extracts data from entropy source)
- Parameters:
-
ctx CTR_DRBG context additional Additional data to add to state (Can be NULL) len Length of additional data
- Returns:
- 0 if successful, or POLARSSL_ERR_CTR_DRBG_ENTROPY_SOURCE_FAILED
Definition at line 274 of file ctr_drbg.c.
int ctr_drbg_self_test | ( | int | verbose ) |
Checkup routine.
- Returns:
- 0 if successful, or 1 if the test failed
Definition at line 511 of file ctr_drbg.c.
void ctr_drbg_set_entropy_len | ( | ctr_drbg_context * | ctx, |
size_t | len | ||
) |
Set the amount of entropy grabbed on each (re)seed (Default: CTR_DRBG_ENTROPY_LEN)
- Parameters:
-
ctx CTR_DRBG context len Amount of entropy to grab
Definition at line 119 of file ctr_drbg.c.
void ctr_drbg_set_prediction_resistance | ( | ctr_drbg_context * | ctx, |
int | resistance | ||
) |
Enable / disable prediction resistance (Default: Off)
Note: If enabled, entropy is used for ctx->entropy_len before each call! Only use this if you have ample supply of good entropy!
- Parameters:
-
ctx CTR_DRBG context resistance CTR_DRBG_PR_ON or CTR_DRBG_PR_OFF
Definition at line 114 of file ctr_drbg.c.
void ctr_drbg_set_reseed_interval | ( | ctr_drbg_context * | ctx, |
int | interval | ||
) |
Set the reseed interval (Default: CTR_DRBG_RESEED_INTERVAL)
- Parameters:
-
ctx CTR_DRBG context interval Reseed interval
Definition at line 124 of file ctr_drbg.c.
void ctr_drbg_update | ( | ctr_drbg_context * | ctx, |
const unsigned char * | additional, | ||
size_t | add_len | ||
) |
CTR_DRBG update state.
- Parameters:
-
ctx CTR_DRBG context additional Additional data to update state with add_len Length of additional data
- Note:
- If add_len is greater than CTR_DRBG_MAX_SEED_INPUT, only the first CTR_DRBG_MAX_SEED_INPUT bytes are used, the remaining ones are silently discarded.
Definition at line 257 of file ctr_drbg.c.
int ctr_drbg_update_seed_file | ( | ctr_drbg_context * | ctx, |
const char * | path | ||
) |
Read and update a seed file.
Seed is added to this instance
- Parameters:
-
ctx CTR_DRBG context path Name of the file
- Returns:
- 0 if successful, POLARSSL_ERR_CTR_DRBG_FILE_IO_ERROR on file error, POLARSSL_ERR_CTR_DRBG_ENTROPY_SOURCE_FAILED or POLARSSL_ERR_CTR_DRBG_INPUT_TOO_BIG
Definition at line 416 of file ctr_drbg.c.
int ctr_drbg_write_seed_file | ( | ctr_drbg_context * | ctx, |
const char * | path | ||
) |
Write a seed file.
- Parameters:
-
ctx CTR_DRBG context path Name of the file
- Returns:
- 0 if successful, POLARSSL_ERR_CTR_DRBG_FILE_IO_ERROR on file error, or POLARSSL_ERR_CTR_DRBG_ENTROPY_SOURCE_FAILED
Definition at line 391 of file ctr_drbg.c.
Generated on Tue Jul 12 2022 13:50:39 by
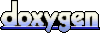